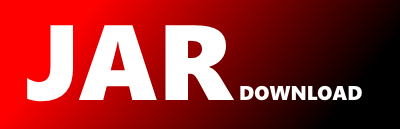
com.yahoo.language.wordpiece.WordPieceConfig Maven / Gradle / Ivy
The newest version!
// ------------ D O N O T E D I T ! ------------
// This file is generated from a config definition file.
package com.yahoo.language.wordpiece;
import java.util.*;
import java.io.File;
import java.nio.file.Path;
import com.yahoo.config.*;
/**
* This class represents the root node of word-piece
*
* Copyright Vespa.ai. Licensed under the terms of the Apache 2.0 license. See LICENSE in the project root.
* Configures com.yahoo.language.wordpiece.WordPieceEmbedder
*/
public final class WordPieceConfig extends ConfigInstance {
public final static String CONFIG_DEF_MD5 = "b1dfd433baa59a6b8a8f0123769d07b1";
public final static String CONFIG_DEF_NAME = "word-piece";
public final static String CONFIG_DEF_NAMESPACE = "language.wordpiece";
public final static String[] CONFIG_DEF_SCHEMA = {
"namespace=language.wordpiece",
"subwordPrefix string default=\"##\"",
"model[].language string",
"model[].path path"
};
public static String getDefMd5() { return CONFIG_DEF_MD5; }
public static String getDefName() { return CONFIG_DEF_NAME; }
public static String getDefNamespace() { return CONFIG_DEF_NAMESPACE; }
public interface Producer extends ConfigInstance.Producer {
void getConfig(Builder builder);
}
public static final class Builder implements ConfigInstance.Builder {
private Set __uninitialized = new HashSet();
private String subwordPrefix = null;
public List model = new ArrayList<>();
public Builder() { }
public Builder(WordPieceConfig config) {
subwordPrefix(config.subwordPrefix());
for (Model m : config.model()) {
model(new Model.Builder(m));
}
}
private Builder override(Builder __superior) {
if (__superior.subwordPrefix != null)
subwordPrefix(__superior.subwordPrefix);
if (!__superior.model.isEmpty())
model.addAll(__superior.model);
return this;
}
public Builder subwordPrefix(String __value) {
if (__value == null) throw new IllegalArgumentException("Null value is not allowed.");
subwordPrefix = __value;
return this;
}
/**
* Add the given builder to this builder's list of Model builders
* @param __builder a builder
* @return this builder
*/
public Builder model(Model.Builder __builder) {
model.add(__builder);
return this;
}
/**
* Make a new builder and run the supplied function on it before adding it to the list
* @param __func lambda that modifies the given builder
* @return this builder
*/
public Builder model(java.util.function.Consumer __func) {
Model.Builder __inner = new Model.Builder();
__func.accept(__inner);
model.add(__inner);
return this;
}
/**
* Set the given list as this builder's list of Model builders
* @param __builders a list of builders
* @return this builder
*/
public Builder model(List __builders) {
model = __builders;
return this;
}
private boolean _applyOnRestart = false;
@java.lang.Override
public final boolean dispatchGetConfig(ConfigInstance.Producer producer) {
if (producer instanceof Producer) {
((Producer)producer).getConfig(this);
return true;
}
return false;
}
@java.lang.Override
public final String getDefMd5() { return CONFIG_DEF_MD5; }
@java.lang.Override
public final String getDefName() { return CONFIG_DEF_NAME; }
@java.lang.Override
public final String getDefNamespace() { return CONFIG_DEF_NAMESPACE; }
@java.lang.Override
public final boolean getApplyOnRestart() { return _applyOnRestart; }
@java.lang.Override
public final void setApplyOnRestart(boolean applyOnRestart) { _applyOnRestart = applyOnRestart; }
public WordPieceConfig build() {
return new WordPieceConfig(this);
}
}
// The prefix to prepend to subword tokens
private final StringNode subwordPrefix;
private final InnerNodeVector model;
public WordPieceConfig(Builder builder) {
this(builder, true);
}
private WordPieceConfig(Builder builder, boolean throwIfUninitialized) {
if (throwIfUninitialized && ! builder.__uninitialized.isEmpty())
throw new IllegalArgumentException("The following builder parameters for " +
"word-piece must be initialized: " + builder.__uninitialized);
subwordPrefix = (builder.subwordPrefix == null) ?
new StringNode("##") : new StringNode(builder.subwordPrefix);
model = Model.createVector(builder.model);
}
/**
* @return word-piece.subwordPrefix
*/
public String subwordPrefix() {
return subwordPrefix.value();
}
/**
* @return word-piece.model[]
*/
public List model() {
return model;
}
/**
* @param i the index of the value to return
* @return word-piece.model[]
*/
public Model model(int i) {
return model.get(i);
}
private ChangesRequiringRestart getChangesRequiringRestart(WordPieceConfig newConfig) {
ChangesRequiringRestart changes = new ChangesRequiringRestart("word-piece");
return changes;
}
private static boolean containsFieldsFlaggedWithRestart() {
return false;
}
/**
* This class represents word-piece.model[]
*/
public final static class Model extends InnerNode {
public static final class Builder implements ConfigBuilder {
private Set __uninitialized = new HashSet(List.of(
"language",
"path"
));
private String language = null;
private FileReference path = null;
public Builder() { }
public Builder(Model config) {
language(config.language());
path(config.path.getFileReference());
}
private Builder override(Builder __superior) {
if (__superior.language != null)
language(__superior.language);
if (__superior.path != null)
path(__superior.path);
return this;
}
public Builder language(String __value) {
if (__value == null) throw new IllegalArgumentException("Null value is not allowed.");
language = __value;
__uninitialized.remove("language");
return this;
}
public Builder path(FileReference __value) {
if (__value == null) throw new IllegalArgumentException("Null value is not allowed.");
path = __value;
__uninitialized.remove("path");
return this;
}
public Model build() {
return new Model(this);
}
}
// The language a model is for, one of the language tags in com.yahoo.language.Language.
// Use "unknown" for a model to be used for any language (i.e by default).
private final StringNode language;
// The path to the model relative to the application package root
private final PathNode path;
public Model(Builder builder) {
this(builder, true);
}
private Model(Builder builder, boolean throwIfUninitialized) {
if (throwIfUninitialized && ! builder.__uninitialized.isEmpty())
throw new IllegalArgumentException("The following builder parameters for " +
"word-piece.model[] must be initialized: " + builder.__uninitialized);
language = (builder.language == null) ?
new StringNode() : new StringNode(builder.language);
path = (builder.path == null) ?
new PathNode() : new PathNode(builder.path);
}
/**
* @return word-piece.model[].language
*/
public String language() {
return language.value();
}
/**
* @return word-piece.model[].path
*/
public Path path() {
return path.value();
}
private ChangesRequiringRestart getChangesRequiringRestart(Model newConfig) {
ChangesRequiringRestart changes = new ChangesRequiringRestart("model");
return changes;
}
private static InnerNodeVector createVector(List builders) {
List elems = new ArrayList<>();
for (Builder b : builders) {
elems.add(new Model(b));
}
return new InnerNodeVector(elems);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy