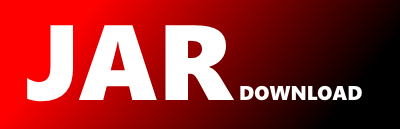
ai.vespa.metricsproxy.metric.ExternalMetrics Maven / Gradle / Ivy
// Copyright Vespa.ai. Licensed under the terms of the Apache 2.0 license. See LICENSE in the project root.
package ai.vespa.metricsproxy.metric;
import ai.vespa.metricsproxy.core.ConfiguredMetric;
import ai.vespa.metricsproxy.core.MetricsConsumers;
import ai.vespa.metricsproxy.metric.model.DimensionId;
import ai.vespa.metricsproxy.metric.model.MetricId;
import ai.vespa.metricsproxy.metric.model.MetricsPacket;
import ai.vespa.metricsproxy.metric.model.ServiceId;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.logging.Logger;
import static ai.vespa.metricsproxy.metric.model.DimensionId.toDimensionId;
import static java.util.logging.Level.FINE;
import static java.util.stream.Collectors.toCollection;
/**
* Handling of metrics received from external processes.
*
* @author gjoranv
*/
public class ExternalMetrics {
private static final Logger log = Logger.getLogger(ExternalMetrics.class.getName());
// NOTE: node service id must be kept in sync with the same constant _value_ used in node-admin:Metrics.java
public static final ServiceId VESPA_NODE_SERVICE_ID = ServiceId.toServiceId("vespa.node");
public static final DimensionId ROLE_DIMENSION = toDimensionId("role");
public static final DimensionId STATE_DIMENSION = toDimensionId("state");
private volatile List metrics = new ArrayList<>();
private final MetricsConsumers consumers;
public ExternalMetrics(MetricsConsumers consumers) {
this.consumers = consumers;
}
public List getMetrics() {
return metrics;
}
public void setExtraMetrics(List externalPackets) {
// TODO: Metrics filtering per consumer is not yet implemented.
// Split each packet per metric, and re-aggregate based on the metrics each consumer wants.
// Then filter out all packages with no consumers.
log.log(FINE, () -> "Setting new external metrics with " + externalPackets.size() + " metrics packets.");
externalPackets.forEach(packet -> packet.addConsumers(consumers.getAllConsumers())
.retainMetrics(metricsToRetain())
.applyOutputNames(outputNamesById()));
metrics = List.copyOf(externalPackets);
}
private Set metricsToRetain() {
return consumers.getConsumersByMetric().keySet().stream()
.map(ConfiguredMetric::id)
.collect(toCollection(LinkedHashSet::new));
}
/**
* Returns a mapping from metric id to a list of the metric's output names.
* Metrics that only have their id as output name are included in the output.
*/
private Map> outputNamesById() {
Map> outputNamesById = new LinkedHashMap<>();
for (ConfiguredMetric metric : consumers.getConsumersByMetric().keySet()) {
outputNamesById.computeIfAbsent(metric.id(), unused -> new ArrayList<>())
.add(metric.outputname());
}
return outputNamesById;
}
/**
* Extracts the node repository dimensions (role, state etc.) from the given packets.
* If the same dimension exists in multiple packets, this implementation gives no guarantees
* about which value is returned.
*/
public static Map extractConfigserverDimensions(Collection packets) {
Map dimensions = new HashMap<>();
for (MetricsPacket.Builder packet : packets) {
dimensions.putAll(packet.build().dimensions());
}
dimensions.keySet().retainAll(Set.of(ROLE_DIMENSION, STATE_DIMENSION));
return dimensions;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy