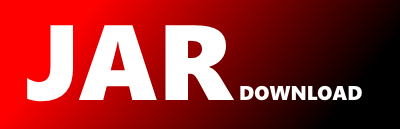
com.yahoo.vespa.hosted.provision.lb.SharedLoadBalancerService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of node-repository Show documentation
Show all versions of node-repository Show documentation
Keeps track of node assignment in a multi-application setup.
// Copyright 2018 Yahoo Holdings. Licensed under the terms of the Apache 2.0 license. See LICENSE in the project root.
package com.yahoo.vespa.hosted.provision.lb;
import com.google.inject.Inject;
import com.yahoo.config.provision.ApplicationId;
import com.yahoo.config.provision.ClusterSpec;
import com.yahoo.config.provision.HostName;
import com.yahoo.config.provision.NodeType;
import com.yahoo.vespa.hosted.provision.Node;
import com.yahoo.vespa.hosted.provision.NodeRepository;
import com.yahoo.vespa.hosted.provision.node.IP;
import java.util.Comparator;
import java.util.Objects;
import java.util.Optional;
import java.util.Set;
import java.util.stream.Collectors;
/**
* This code mimics provisioning load balancers that already exist in the routing layer.
* It will just map the load balancer request to the proxy nodes available in the node repository.
*
* @author ogronnesby
*/
public class SharedLoadBalancerService implements LoadBalancerService {
private static final Comparator hostnameComparator = Comparator.comparing(Node::hostname);
private final NodeRepository nodeRepository;
@Inject
public SharedLoadBalancerService(NodeRepository nodeRepository) {
this.nodeRepository = Objects.requireNonNull(nodeRepository, "Missing nodeRepository value");
}
@Override
public LoadBalancerInstance create(ApplicationId application, ClusterSpec.Id cluster, Set reals, boolean force) {
final var proxyNodes = nodeRepository.getNodes(NodeType.proxy);
proxyNodes.sort(hostnameComparator);
if (proxyNodes.size() == 0) {
throw new IllegalStateException("Missing proxy nodes in nodeRepository");
}
final var firstProxyNode = proxyNodes.get(0);
final var networkNames = proxyNodes.stream()
.flatMap(node -> node.ipAddresses().stream())
.map(SharedLoadBalancerService::addNetworKPrefixLength)
.collect(Collectors.toSet());
return new LoadBalancerInstance(
HostName.from(firstProxyNode.hostname()),
Optional.empty(),
Set.of(4080, 4443),
networkNames,
reals
);
}
@Override
public void remove(ApplicationId application, ClusterSpec.Id cluster) {
// Do nothing, we have no external state to modify
}
@Override
public Protocol protocol() {
return Protocol.dualstack;
}
private static String addNetworKPrefixLength(String address) {
if (IP.isV6(address)) {
return address + "/128";
}
else {
return address + "/32";
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy