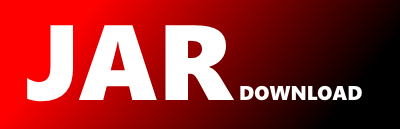
com.yahoo.document.predicate.parser.PredicateLexer Maven / Gradle / Ivy
The newest version!
// $ANTLR 3.5.3 com/yahoo/document/predicate/parser/Predicate.g 2024-12-16 15:46:34
package com.yahoo.document.predicate.parser;
import com.yahoo.document.predicate.Predicate;
import org.antlr.runtime.*;
import java.util.Stack;
import java.util.List;
import java.util.ArrayList;
@SuppressWarnings("all")
public class PredicateLexer extends Lexer {
public static final int EOF=-1;
public static final int T__14=14;
public static final int T__15=15;
public static final int T__16=16;
public static final int T__17=17;
public static final int T__18=18;
public static final int T__19=19;
public static final int T__20=20;
public static final int T__21=21;
public static final int T__22=22;
public static final int AND=4;
public static final int FALSE=5;
public static final int IN=6;
public static final int INTEGER=7;
public static final int NOT=8;
public static final int OR=9;
public static final int STRING=10;
public static final int TRUE=11;
public static final int VALUE=12;
public static final int WS=13;
@Override
public void emitErrorMessage(String message) {
throw new IllegalArgumentException(message);
}
// delegates
// delegators
public Lexer[] getDelegates() {
return new Lexer[] {};
}
public PredicateLexer() {}
public PredicateLexer(CharStream input) {
this(input, new RecognizerSharedState());
}
public PredicateLexer(CharStream input, RecognizerSharedState state) {
super(input,state);
}
@Override public String getGrammarFileName() { return "com/yahoo/document/predicate/parser/Predicate.g"; }
// $ANTLR start "T__14"
public final void mT__14() throws RecognitionException {
try {
int _type = T__14;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/yahoo/document/predicate/parser/Predicate.g:14:7: ( '(' )
// com/yahoo/document/predicate/parser/Predicate.g:14:9: '('
{
match('(');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "T__14"
// $ANTLR start "T__15"
public final void mT__15() throws RecognitionException {
try {
int _type = T__15;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/yahoo/document/predicate/parser/Predicate.g:15:7: ( ')' )
// com/yahoo/document/predicate/parser/Predicate.g:15:9: ')'
{
match(')');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "T__15"
// $ANTLR start "T__16"
public final void mT__16() throws RecognitionException {
try {
int _type = T__16;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/yahoo/document/predicate/parser/Predicate.g:16:7: ( '+[' )
// com/yahoo/document/predicate/parser/Predicate.g:16:9: '+['
{
match("+[");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "T__16"
// $ANTLR start "T__17"
public final void mT__17() throws RecognitionException {
try {
int _type = T__17;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/yahoo/document/predicate/parser/Predicate.g:17:7: ( ',' )
// com/yahoo/document/predicate/parser/Predicate.g:17:9: ','
{
match(',');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "T__17"
// $ANTLR start "T__18"
public final void mT__18() throws RecognitionException {
try {
int _type = T__18;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/yahoo/document/predicate/parser/Predicate.g:18:7: ( '..' )
// com/yahoo/document/predicate/parser/Predicate.g:18:9: '..'
{
match("..");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "T__18"
// $ANTLR start "T__19"
public final void mT__19() throws RecognitionException {
try {
int _type = T__19;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/yahoo/document/predicate/parser/Predicate.g:19:7: ( '=' )
// com/yahoo/document/predicate/parser/Predicate.g:19:9: '='
{
match('=');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "T__19"
// $ANTLR start "T__20"
public final void mT__20() throws RecognitionException {
try {
int _type = T__20;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/yahoo/document/predicate/parser/Predicate.g:20:7: ( '=-' )
// com/yahoo/document/predicate/parser/Predicate.g:20:9: '=-'
{
match("=-");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "T__20"
// $ANTLR start "T__21"
public final void mT__21() throws RecognitionException {
try {
int _type = T__21;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/yahoo/document/predicate/parser/Predicate.g:21:7: ( '[' )
// com/yahoo/document/predicate/parser/Predicate.g:21:9: '['
{
match('[');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "T__21"
// $ANTLR start "T__22"
public final void mT__22() throws RecognitionException {
try {
int _type = T__22;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/yahoo/document/predicate/parser/Predicate.g:22:7: ( ']' )
// com/yahoo/document/predicate/parser/Predicate.g:22:9: ']'
{
match(']');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "T__22"
// $ANTLR start "INTEGER"
public final void mINTEGER() throws RecognitionException {
try {
int _type = INTEGER;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/yahoo/document/predicate/parser/Predicate.g:100:9: ( ( '-' | '+' )? ( '1' .. '9' ( '0' .. '9' )* | '0' ) )
// com/yahoo/document/predicate/parser/Predicate.g:100:11: ( '-' | '+' )? ( '1' .. '9' ( '0' .. '9' )* | '0' )
{
// com/yahoo/document/predicate/parser/Predicate.g:100:11: ( '-' | '+' )?
int alt1=2;
int LA1_0 = input.LA(1);
if ( (LA1_0=='+'||LA1_0=='-') ) {
alt1=1;
}
switch (alt1) {
case 1 :
// com/yahoo/document/predicate/parser/Predicate.g:
{
if ( input.LA(1)=='+'||input.LA(1)=='-' ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
}
// com/yahoo/document/predicate/parser/Predicate.g:100:26: ( '1' .. '9' ( '0' .. '9' )* | '0' )
int alt3=2;
int LA3_0 = input.LA(1);
if ( ((LA3_0 >= '1' && LA3_0 <= '9')) ) {
alt3=1;
}
else if ( (LA3_0=='0') ) {
alt3=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 3, 0, input);
throw nvae;
}
switch (alt3) {
case 1 :
// com/yahoo/document/predicate/parser/Predicate.g:100:27: '1' .. '9' ( '0' .. '9' )*
{
matchRange('1','9');
// com/yahoo/document/predicate/parser/Predicate.g:100:36: ( '0' .. '9' )*
loop2:
while (true) {
int alt2=2;
int LA2_0 = input.LA(1);
if ( ((LA2_0 >= '0' && LA2_0 <= '9')) ) {
alt2=1;
}
switch (alt2) {
case 1 :
// com/yahoo/document/predicate/parser/Predicate.g:
{
if ( (input.LA(1) >= '0' && input.LA(1) <= '9') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
break loop2;
}
}
}
break;
case 2 :
// com/yahoo/document/predicate/parser/Predicate.g:100:52: '0'
{
match('0');
}
break;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "INTEGER"
// $ANTLR start "OR"
public final void mOR() throws RecognitionException {
try {
int _type = OR;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/yahoo/document/predicate/parser/Predicate.g:102:5: ( 'OR' | 'or' )
int alt4=2;
int LA4_0 = input.LA(1);
if ( (LA4_0=='O') ) {
alt4=1;
}
else if ( (LA4_0=='o') ) {
alt4=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 4, 0, input);
throw nvae;
}
switch (alt4) {
case 1 :
// com/yahoo/document/predicate/parser/Predicate.g:102:7: 'OR'
{
match("OR");
}
break;
case 2 :
// com/yahoo/document/predicate/parser/Predicate.g:102:14: 'or'
{
match("or");
}
break;
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "OR"
// $ANTLR start "AND"
public final void mAND() throws RecognitionException {
try {
int _type = AND;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/yahoo/document/predicate/parser/Predicate.g:103:5: ( 'AND' | 'and' )
int alt5=2;
int LA5_0 = input.LA(1);
if ( (LA5_0=='A') ) {
alt5=1;
}
else if ( (LA5_0=='a') ) {
alt5=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 5, 0, input);
throw nvae;
}
switch (alt5) {
case 1 :
// com/yahoo/document/predicate/parser/Predicate.g:103:7: 'AND'
{
match("AND");
}
break;
case 2 :
// com/yahoo/document/predicate/parser/Predicate.g:103:15: 'and'
{
match("and");
}
break;
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "AND"
// $ANTLR start "NOT"
public final void mNOT() throws RecognitionException {
try {
int _type = NOT;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/yahoo/document/predicate/parser/Predicate.g:104:5: ( 'NOT' | 'not' )
int alt6=2;
int LA6_0 = input.LA(1);
if ( (LA6_0=='N') ) {
alt6=1;
}
else if ( (LA6_0=='n') ) {
alt6=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 6, 0, input);
throw nvae;
}
switch (alt6) {
case 1 :
// com/yahoo/document/predicate/parser/Predicate.g:104:7: 'NOT'
{
match("NOT");
}
break;
case 2 :
// com/yahoo/document/predicate/parser/Predicate.g:104:15: 'not'
{
match("not");
}
break;
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "NOT"
// $ANTLR start "IN"
public final void mIN() throws RecognitionException {
try {
int _type = IN;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/yahoo/document/predicate/parser/Predicate.g:105:5: ( 'IN' | 'in' )
int alt7=2;
int LA7_0 = input.LA(1);
if ( (LA7_0=='I') ) {
alt7=1;
}
else if ( (LA7_0=='i') ) {
alt7=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 7, 0, input);
throw nvae;
}
switch (alt7) {
case 1 :
// com/yahoo/document/predicate/parser/Predicate.g:105:7: 'IN'
{
match("IN");
}
break;
case 2 :
// com/yahoo/document/predicate/parser/Predicate.g:105:14: 'in'
{
match("in");
}
break;
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "IN"
// $ANTLR start "TRUE"
public final void mTRUE() throws RecognitionException {
try {
int _type = TRUE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/yahoo/document/predicate/parser/Predicate.g:106:6: ( 'TRUE' | 'true' )
int alt8=2;
int LA8_0 = input.LA(1);
if ( (LA8_0=='T') ) {
alt8=1;
}
else if ( (LA8_0=='t') ) {
alt8=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 8, 0, input);
throw nvae;
}
switch (alt8) {
case 1 :
// com/yahoo/document/predicate/parser/Predicate.g:106:8: 'TRUE'
{
match("TRUE");
}
break;
case 2 :
// com/yahoo/document/predicate/parser/Predicate.g:106:17: 'true'
{
match("true");
}
break;
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "TRUE"
// $ANTLR start "FALSE"
public final void mFALSE() throws RecognitionException {
try {
int _type = FALSE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/yahoo/document/predicate/parser/Predicate.g:107:7: ( 'FALSE' | 'false' )
int alt9=2;
int LA9_0 = input.LA(1);
if ( (LA9_0=='F') ) {
alt9=1;
}
else if ( (LA9_0=='f') ) {
alt9=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 9, 0, input);
throw nvae;
}
switch (alt9) {
case 1 :
// com/yahoo/document/predicate/parser/Predicate.g:107:9: 'FALSE'
{
match("FALSE");
}
break;
case 2 :
// com/yahoo/document/predicate/parser/Predicate.g:107:19: 'false'
{
match("false");
}
break;
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "FALSE"
// $ANTLR start "VALUE"
public final void mVALUE() throws RecognitionException {
try {
int _type = VALUE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/yahoo/document/predicate/parser/Predicate.g:109:7: ( ( 'a' .. 'z' | 'A' .. 'Z' | '0' .. '9' | '_' )+ )
// com/yahoo/document/predicate/parser/Predicate.g:109:9: ( 'a' .. 'z' | 'A' .. 'Z' | '0' .. '9' | '_' )+
{
// com/yahoo/document/predicate/parser/Predicate.g:109:9: ( 'a' .. 'z' | 'A' .. 'Z' | '0' .. '9' | '_' )+
int cnt10=0;
loop10:
while (true) {
int alt10=2;
int LA10_0 = input.LA(1);
if ( ((LA10_0 >= '0' && LA10_0 <= '9')||(LA10_0 >= 'A' && LA10_0 <= 'Z')||LA10_0=='_'||(LA10_0 >= 'a' && LA10_0 <= 'z')) ) {
alt10=1;
}
switch (alt10) {
case 1 :
// com/yahoo/document/predicate/parser/Predicate.g:
{
if ( (input.LA(1) >= '0' && input.LA(1) <= '9')||(input.LA(1) >= 'A' && input.LA(1) <= 'Z')||input.LA(1)=='_'||(input.LA(1) >= 'a' && input.LA(1) <= 'z') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
if ( cnt10 >= 1 ) break loop10;
EarlyExitException eee = new EarlyExitException(10, input);
throw eee;
}
cnt10++;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "VALUE"
// $ANTLR start "STRING"
public final void mSTRING() throws RecognitionException {
try {
int _type = STRING;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/yahoo/document/predicate/parser/Predicate.g:112:5: ( ( '\\'' (~ ( '\\'' ) | '\\\\\\'' )* '\\'' | '\\\"' (~ ( '\\\"' ) | '\\\\\\\"' )* '\\\"' ) )
// com/yahoo/document/predicate/parser/Predicate.g:112:7: ( '\\'' (~ ( '\\'' ) | '\\\\\\'' )* '\\'' | '\\\"' (~ ( '\\\"' ) | '\\\\\\\"' )* '\\\"' )
{
// com/yahoo/document/predicate/parser/Predicate.g:112:7: ( '\\'' (~ ( '\\'' ) | '\\\\\\'' )* '\\'' | '\\\"' (~ ( '\\\"' ) | '\\\\\\\"' )* '\\\"' )
int alt13=2;
int LA13_0 = input.LA(1);
if ( (LA13_0=='\'') ) {
alt13=1;
}
else if ( (LA13_0=='\"') ) {
alt13=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 13, 0, input);
throw nvae;
}
switch (alt13) {
case 1 :
// com/yahoo/document/predicate/parser/Predicate.g:112:9: '\\'' (~ ( '\\'' ) | '\\\\\\'' )* '\\''
{
match('\'');
// com/yahoo/document/predicate/parser/Predicate.g:112:14: (~ ( '\\'' ) | '\\\\\\'' )*
loop11:
while (true) {
int alt11=3;
int LA11_0 = input.LA(1);
if ( (LA11_0=='\\') ) {
int LA11_2 = input.LA(2);
if ( (LA11_2=='\'') ) {
int LA11_4 = input.LA(3);
if ( ((LA11_4 >= '\u0000' && LA11_4 <= '\uFFFF')) ) {
alt11=2;
}
else {
alt11=1;
}
}
else if ( ((LA11_2 >= '\u0000' && LA11_2 <= '&')||(LA11_2 >= '(' && LA11_2 <= '\uFFFF')) ) {
alt11=1;
}
}
else if ( ((LA11_0 >= '\u0000' && LA11_0 <= '&')||(LA11_0 >= '(' && LA11_0 <= '[')||(LA11_0 >= ']' && LA11_0 <= '\uFFFF')) ) {
alt11=1;
}
switch (alt11) {
case 1 :
// com/yahoo/document/predicate/parser/Predicate.g:112:16: ~ ( '\\'' )
{
if ( (input.LA(1) >= '\u0000' && input.LA(1) <= '&')||(input.LA(1) >= '(' && input.LA(1) <= '\uFFFF') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
case 2 :
// com/yahoo/document/predicate/parser/Predicate.g:112:26: '\\\\\\''
{
match("\\'");
}
break;
default :
break loop11;
}
}
match('\'');
}
break;
case 2 :
// com/yahoo/document/predicate/parser/Predicate.g:113:9: '\\\"' (~ ( '\\\"' ) | '\\\\\\\"' )* '\\\"'
{
match('\"');
// com/yahoo/document/predicate/parser/Predicate.g:113:14: (~ ( '\\\"' ) | '\\\\\\\"' )*
loop12:
while (true) {
int alt12=3;
int LA12_0 = input.LA(1);
if ( (LA12_0=='\\') ) {
int LA12_2 = input.LA(2);
if ( (LA12_2=='\"') ) {
int LA12_4 = input.LA(3);
if ( ((LA12_4 >= '\u0000' && LA12_4 <= '\uFFFF')) ) {
alt12=2;
}
else {
alt12=1;
}
}
else if ( ((LA12_2 >= '\u0000' && LA12_2 <= '!')||(LA12_2 >= '#' && LA12_2 <= '\uFFFF')) ) {
alt12=1;
}
}
else if ( ((LA12_0 >= '\u0000' && LA12_0 <= '!')||(LA12_0 >= '#' && LA12_0 <= '[')||(LA12_0 >= ']' && LA12_0 <= '\uFFFF')) ) {
alt12=1;
}
switch (alt12) {
case 1 :
// com/yahoo/document/predicate/parser/Predicate.g:113:16: ~ ( '\\\"' )
{
if ( (input.LA(1) >= '\u0000' && input.LA(1) <= '!')||(input.LA(1) >= '#' && input.LA(1) <= '\uFFFF') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
case 2 :
// com/yahoo/document/predicate/parser/Predicate.g:113:26: '\\\\\\\"'
{
match("\\\"");
}
break;
default :
break loop12;
}
}
match('\"');
}
break;
}
setText(Predicate.asciiDecode(getText().substring(1, getText().length() - 1)));
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "STRING"
// $ANTLR start "WS"
public final void mWS() throws RecognitionException {
try {
int _type = WS;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/yahoo/document/predicate/parser/Predicate.g:117:5: ( ( ' ' | '\\t' )+ )
// com/yahoo/document/predicate/parser/Predicate.g:117:7: ( ' ' | '\\t' )+
{
// com/yahoo/document/predicate/parser/Predicate.g:117:7: ( ' ' | '\\t' )+
int cnt14=0;
loop14:
while (true) {
int alt14=2;
int LA14_0 = input.LA(1);
if ( (LA14_0=='\t'||LA14_0==' ') ) {
alt14=1;
}
switch (alt14) {
case 1 :
// com/yahoo/document/predicate/parser/Predicate.g:
{
if ( input.LA(1)=='\t'||input.LA(1)==' ' ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
if ( cnt14 >= 1 ) break loop14;
EarlyExitException eee = new EarlyExitException(14, input);
throw eee;
}
cnt14++;
}
_channel = HIDDEN;
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "WS"
@Override
public void mTokens() throws RecognitionException {
// com/yahoo/document/predicate/parser/Predicate.g:1:8: ( T__14 | T__15 | T__16 | T__17 | T__18 | T__19 | T__20 | T__21 | T__22 | INTEGER | OR | AND | NOT | IN | TRUE | FALSE | VALUE | STRING | WS )
int alt15=19;
alt15 = dfa15.predict(input);
switch (alt15) {
case 1 :
// com/yahoo/document/predicate/parser/Predicate.g:1:10: T__14
{
mT__14();
}
break;
case 2 :
// com/yahoo/document/predicate/parser/Predicate.g:1:16: T__15
{
mT__15();
}
break;
case 3 :
// com/yahoo/document/predicate/parser/Predicate.g:1:22: T__16
{
mT__16();
}
break;
case 4 :
// com/yahoo/document/predicate/parser/Predicate.g:1:28: T__17
{
mT__17();
}
break;
case 5 :
// com/yahoo/document/predicate/parser/Predicate.g:1:34: T__18
{
mT__18();
}
break;
case 6 :
// com/yahoo/document/predicate/parser/Predicate.g:1:40: T__19
{
mT__19();
}
break;
case 7 :
// com/yahoo/document/predicate/parser/Predicate.g:1:46: T__20
{
mT__20();
}
break;
case 8 :
// com/yahoo/document/predicate/parser/Predicate.g:1:52: T__21
{
mT__21();
}
break;
case 9 :
// com/yahoo/document/predicate/parser/Predicate.g:1:58: T__22
{
mT__22();
}
break;
case 10 :
// com/yahoo/document/predicate/parser/Predicate.g:1:64: INTEGER
{
mINTEGER();
}
break;
case 11 :
// com/yahoo/document/predicate/parser/Predicate.g:1:72: OR
{
mOR();
}
break;
case 12 :
// com/yahoo/document/predicate/parser/Predicate.g:1:75: AND
{
mAND();
}
break;
case 13 :
// com/yahoo/document/predicate/parser/Predicate.g:1:79: NOT
{
mNOT();
}
break;
case 14 :
// com/yahoo/document/predicate/parser/Predicate.g:1:83: IN
{
mIN();
}
break;
case 15 :
// com/yahoo/document/predicate/parser/Predicate.g:1:86: TRUE
{
mTRUE();
}
break;
case 16 :
// com/yahoo/document/predicate/parser/Predicate.g:1:91: FALSE
{
mFALSE();
}
break;
case 17 :
// com/yahoo/document/predicate/parser/Predicate.g:1:97: VALUE
{
mVALUE();
}
break;
case 18 :
// com/yahoo/document/predicate/parser/Predicate.g:1:103: STRING
{
mSTRING();
}
break;
case 19 :
// com/yahoo/document/predicate/parser/Predicate.g:1:110: WS
{
mWS();
}
break;
}
}
protected DFA15 dfa15 = new DFA15(this);
static final String DFA15_eotS =
"\6\uffff\1\35\3\uffff\2\11\14\30\6\uffff\1\11\2\53\4\30\2\60\4\30\1\uffff"+
"\2\65\2\66\1\uffff\4\30\2\uffff\2\73\2\30\1\uffff\2\76\1\uffff";
static final String DFA15_eofS =
"\77\uffff";
static final String DFA15_minS =
"\1\11\2\uffff\1\60\2\uffff\1\55\3\uffff\2\60\1\122\1\162\1\116\1\156\1"+
"\117\1\157\1\116\1\156\1\122\1\162\1\101\1\141\6\uffff\3\60\1\104\1\144"+
"\1\124\1\164\2\60\1\125\1\165\1\114\1\154\1\uffff\4\60\1\uffff\1\105\1"+
"\145\1\123\1\163\2\uffff\2\60\1\105\1\145\1\uffff\2\60\1\uffff";
static final String DFA15_maxS =
"\1\172\2\uffff\1\133\2\uffff\1\55\3\uffff\2\172\1\122\1\162\1\116\1\156"+
"\1\117\1\157\1\116\1\156\1\122\1\162\1\101\1\141\6\uffff\3\172\1\104\1"+
"\144\1\124\1\164\2\172\1\125\1\165\1\114\1\154\1\uffff\4\172\1\uffff\1"+
"\105\1\145\1\123\1\163\2\uffff\2\172\1\105\1\145\1\uffff\2\172\1\uffff";
static final String DFA15_acceptS =
"\1\uffff\1\1\1\2\1\uffff\1\4\1\5\1\uffff\1\10\1\11\1\12\16\uffff\1\21"+
"\1\22\1\23\1\3\1\7\1\6\15\uffff\1\13\4\uffff\1\16\4\uffff\1\14\1\15\4"+
"\uffff\1\17\2\uffff\1\20";
static final String DFA15_specialS =
"\77\uffff}>";
static final String[] DFA15_transitionS = {
"\1\32\26\uffff\1\32\1\uffff\1\31\4\uffff\1\31\1\1\1\2\1\uffff\1\3\1\4"+
"\1\11\1\5\1\uffff\1\13\11\12\3\uffff\1\6\3\uffff\1\16\4\30\1\26\2\30"+
"\1\22\4\30\1\20\1\14\4\30\1\24\6\30\1\7\1\uffff\1\10\1\uffff\1\30\1\uffff"+
"\1\17\4\30\1\27\2\30\1\23\4\30\1\21\1\15\4\30\1\25\6\30",
"",
"",
"\12\11\41\uffff\1\33",
"",
"",
"\1\34",
"",
"",
"",
"\12\36\7\uffff\32\30\4\uffff\1\30\1\uffff\32\30",
"\12\30\7\uffff\32\30\4\uffff\1\30\1\uffff\32\30",
"\1\37",
"\1\40",
"\1\41",
"\1\42",
"\1\43",
"\1\44",
"\1\45",
"\1\46",
"\1\47",
"\1\50",
"\1\51",
"\1\52",
"",
"",
"",
"",
"",
"",
"\12\36\7\uffff\32\30\4\uffff\1\30\1\uffff\32\30",
"\12\30\7\uffff\32\30\4\uffff\1\30\1\uffff\32\30",
"\12\30\7\uffff\32\30\4\uffff\1\30\1\uffff\32\30",
"\1\54",
"\1\55",
"\1\56",
"\1\57",
"\12\30\7\uffff\32\30\4\uffff\1\30\1\uffff\32\30",
"\12\30\7\uffff\32\30\4\uffff\1\30\1\uffff\32\30",
"\1\61",
"\1\62",
"\1\63",
"\1\64",
"",
"\12\30\7\uffff\32\30\4\uffff\1\30\1\uffff\32\30",
"\12\30\7\uffff\32\30\4\uffff\1\30\1\uffff\32\30",
"\12\30\7\uffff\32\30\4\uffff\1\30\1\uffff\32\30",
"\12\30\7\uffff\32\30\4\uffff\1\30\1\uffff\32\30",
"",
"\1\67",
"\1\70",
"\1\71",
"\1\72",
"",
"",
"\12\30\7\uffff\32\30\4\uffff\1\30\1\uffff\32\30",
"\12\30\7\uffff\32\30\4\uffff\1\30\1\uffff\32\30",
"\1\74",
"\1\75",
"",
"\12\30\7\uffff\32\30\4\uffff\1\30\1\uffff\32\30",
"\12\30\7\uffff\32\30\4\uffff\1\30\1\uffff\32\30",
""
};
static final short[] DFA15_eot = DFA.unpackEncodedString(DFA15_eotS);
static final short[] DFA15_eof = DFA.unpackEncodedString(DFA15_eofS);
static final char[] DFA15_min = DFA.unpackEncodedStringToUnsignedChars(DFA15_minS);
static final char[] DFA15_max = DFA.unpackEncodedStringToUnsignedChars(DFA15_maxS);
static final short[] DFA15_accept = DFA.unpackEncodedString(DFA15_acceptS);
static final short[] DFA15_special = DFA.unpackEncodedString(DFA15_specialS);
static final short[][] DFA15_transition;
static {
int numStates = DFA15_transitionS.length;
DFA15_transition = new short[numStates][];
for (int i=0; i
© 2015 - 2024 Weber Informatics LLC | Privacy Policy