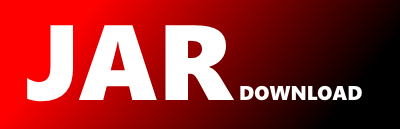
com.yahoo.document.predicate.parser.PredicateParser Maven / Gradle / Ivy
The newest version!
// $ANTLR 3.5.3 com/yahoo/document/predicate/parser/Predicate.g 2024-12-16 15:46:33
package com.yahoo.document.predicate.parser;
import com.yahoo.document.predicate.Conjunction;
import com.yahoo.document.predicate.Disjunction;
import com.yahoo.document.predicate.FeatureRange;
import com.yahoo.document.predicate.FeatureSet;
import com.yahoo.document.predicate.Negation;
import com.yahoo.document.predicate.Predicate;
import com.yahoo.document.predicate.Predicates;
import org.antlr.runtime.*;
import java.util.Stack;
import java.util.List;
import java.util.ArrayList;
@SuppressWarnings("all")
public class PredicateParser extends Parser {
public static final String[] tokenNames = new String[] {
"", "", "", "", "AND", "FALSE", "IN", "INTEGER",
"NOT", "OR", "STRING", "TRUE", "VALUE", "WS", "'('", "')'", "'+['", "','",
"'..'", "'='", "'=-'", "'['", "']'"
};
public static final int EOF=-1;
public static final int T__14=14;
public static final int T__15=15;
public static final int T__16=16;
public static final int T__17=17;
public static final int T__18=18;
public static final int T__19=19;
public static final int T__20=20;
public static final int T__21=21;
public static final int T__22=22;
public static final int AND=4;
public static final int FALSE=5;
public static final int IN=6;
public static final int INTEGER=7;
public static final int NOT=8;
public static final int OR=9;
public static final int STRING=10;
public static final int TRUE=11;
public static final int VALUE=12;
public static final int WS=13;
// delegates
public Parser[] getDelegates() {
return new Parser[] {};
}
// delegators
public PredicateParser(TokenStream input) {
this(input, new RecognizerSharedState());
}
public PredicateParser(TokenStream input, RecognizerSharedState state) {
super(input, state);
}
@Override public String[] getTokenNames() { return PredicateParser.tokenNames; }
@Override public String getGrammarFileName() { return "com/yahoo/document/predicate/parser/Predicate.g"; }
@Override
public void emitErrorMessage(String message) {
throw new IllegalArgumentException(message);
}
// $ANTLR start "predicate"
// com/yahoo/document/predicate/parser/Predicate.g:36:1: predicate returns [Predicate n] : d= disjunction EOF ;
public final Predicate predicate() throws RecognitionException {
Predicate n = null;
Predicate d =null;
try {
// com/yahoo/document/predicate/parser/Predicate.g:37:5: (d= disjunction EOF )
// com/yahoo/document/predicate/parser/Predicate.g:37:7: d= disjunction EOF
{
pushFollow(FOLLOW_disjunction_in_predicate51);
d=disjunction();
state._fsp--;
match(input,EOF,FOLLOW_EOF_in_predicate53);
n = d;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return n;
}
// $ANTLR end "predicate"
// $ANTLR start "disjunction"
// com/yahoo/document/predicate/parser/Predicate.g:40:1: disjunction returns [Predicate n] : c= conjunction ( OR c2= conjunction ( OR c3= conjunction )* )? ;
public final Predicate disjunction() throws RecognitionException {
Predicate n = null;
Predicate c =null;
Predicate c2 =null;
Predicate c3 =null;
try {
// com/yahoo/document/predicate/parser/Predicate.g:41:5: (c= conjunction ( OR c2= conjunction ( OR c3= conjunction )* )? )
// com/yahoo/document/predicate/parser/Predicate.g:41:7: c= conjunction ( OR c2= conjunction ( OR c3= conjunction )* )?
{
pushFollow(FOLLOW_conjunction_in_disjunction78);
c=conjunction();
state._fsp--;
n = c;
// com/yahoo/document/predicate/parser/Predicate.g:42:7: ( OR c2= conjunction ( OR c3= conjunction )* )?
int alt2=2;
int LA2_0 = input.LA(1);
if ( (LA2_0==OR) ) {
alt2=1;
}
switch (alt2) {
case 1 :
// com/yahoo/document/predicate/parser/Predicate.g:42:9: OR c2= conjunction ( OR c3= conjunction )*
{
match(input,OR,FOLLOW_OR_in_disjunction90);
pushFollow(FOLLOW_conjunction_in_disjunction94);
c2=conjunction();
state._fsp--;
n = new Disjunction(n, c2);
// com/yahoo/document/predicate/parser/Predicate.g:43:9: ( OR c3= conjunction )*
loop1:
while (true) {
int alt1=2;
int LA1_0 = input.LA(1);
if ( (LA1_0==OR) ) {
alt1=1;
}
switch (alt1) {
case 1 :
// com/yahoo/document/predicate/parser/Predicate.g:43:11: OR c3= conjunction
{
match(input,OR,FOLLOW_OR_in_disjunction108);
pushFollow(FOLLOW_conjunction_in_disjunction112);
c3=conjunction();
state._fsp--;
((Disjunction)n).addOperand(c3);
}
break;
default :
break loop1;
}
}
}
break;
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return n;
}
// $ANTLR end "disjunction"
// $ANTLR start "conjunction"
// com/yahoo/document/predicate/parser/Predicate.g:46:1: conjunction returns [Predicate n] : u= unary_node ( AND u2= unary_node ( AND u3= unary_node )* )? ;
public final Predicate conjunction() throws RecognitionException {
Predicate n = null;
Predicate u =null;
Predicate u2 =null;
Predicate u3 =null;
try {
// com/yahoo/document/predicate/parser/Predicate.g:47:5: (u= unary_node ( AND u2= unary_node ( AND u3= unary_node )* )? )
// com/yahoo/document/predicate/parser/Predicate.g:47:7: u= unary_node ( AND u2= unary_node ( AND u3= unary_node )* )?
{
pushFollow(FOLLOW_unary_node_in_conjunction143);
u=unary_node();
state._fsp--;
n = u;
// com/yahoo/document/predicate/parser/Predicate.g:48:7: ( AND u2= unary_node ( AND u3= unary_node )* )?
int alt4=2;
int LA4_0 = input.LA(1);
if ( (LA4_0==AND) ) {
alt4=1;
}
switch (alt4) {
case 1 :
// com/yahoo/document/predicate/parser/Predicate.g:48:9: AND u2= unary_node ( AND u3= unary_node )*
{
match(input,AND,FOLLOW_AND_in_conjunction155);
pushFollow(FOLLOW_unary_node_in_conjunction159);
u2=unary_node();
state._fsp--;
n = new Conjunction(n, u2);
// com/yahoo/document/predicate/parser/Predicate.g:49:9: ( AND u3= unary_node )*
loop3:
while (true) {
int alt3=2;
int LA3_0 = input.LA(1);
if ( (LA3_0==AND) ) {
alt3=1;
}
switch (alt3) {
case 1 :
// com/yahoo/document/predicate/parser/Predicate.g:49:11: AND u3= unary_node
{
match(input,AND,FOLLOW_AND_in_conjunction173);
pushFollow(FOLLOW_unary_node_in_conjunction177);
u3=unary_node();
state._fsp--;
((Conjunction)n).addOperand(u3);
}
break;
default :
break loop3;
}
}
}
break;
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return n;
}
// $ANTLR end "conjunction"
// $ANTLR start "unary_node"
// com/yahoo/document/predicate/parser/Predicate.g:52:1: unary_node returns [Predicate n] : (l= leaf | (not= NOT )? '(' d= disjunction ')' );
public final Predicate unary_node() throws RecognitionException {
Predicate n = null;
Token not=null;
Predicate l =null;
Predicate d =null;
try {
// com/yahoo/document/predicate/parser/Predicate.g:53:5: (l= leaf | (not= NOT )? '(' d= disjunction ')' )
int alt6=2;
switch ( input.LA(1) ) {
case AND:
case FALSE:
case IN:
case INTEGER:
case OR:
case STRING:
case TRUE:
case VALUE:
{
alt6=1;
}
break;
case NOT:
{
int LA6_2 = input.LA(2);
if ( (LA6_2==IN||LA6_2==NOT) ) {
alt6=1;
}
else if ( (LA6_2==14) ) {
alt6=2;
}
else {
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 6, 2, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case 14:
{
alt6=2;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 6, 0, input);
throw nvae;
}
switch (alt6) {
case 1 :
// com/yahoo/document/predicate/parser/Predicate.g:53:7: l= leaf
{
pushFollow(FOLLOW_leaf_in_unary_node208);
l=leaf();
state._fsp--;
n = l;
}
break;
case 2 :
// com/yahoo/document/predicate/parser/Predicate.g:54:7: (not= NOT )? '(' d= disjunction ')'
{
// com/yahoo/document/predicate/parser/Predicate.g:54:7: (not= NOT )?
int alt5=2;
int LA5_0 = input.LA(1);
if ( (LA5_0==NOT) ) {
alt5=1;
}
switch (alt5) {
case 1 :
// com/yahoo/document/predicate/parser/Predicate.g:54:9: not= NOT
{
not=(Token)match(input,NOT,FOLLOW_NOT_in_unary_node222);
}
break;
}
match(input,14,FOLLOW_14_in_unary_node227);
pushFollow(FOLLOW_disjunction_in_unary_node231);
d=disjunction();
state._fsp--;
match(input,15,FOLLOW_15_in_unary_node233);
n = d; if (not != null) { n = new Negation(n); }
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return n;
}
// $ANTLR end "unary_node"
// $ANTLR start "leaf"
// com/yahoo/document/predicate/parser/Predicate.g:57:1: leaf returns [Predicate n] : (k= value (not= NOT )? IN (mv= multivalue[k] |r= range[k] ) | TRUE | FALSE );
public final Predicate leaf() throws RecognitionException {
Predicate n = null;
Token not=null;
String k =null;
FeatureSet mv =null;
FeatureRange r =null;
try {
// com/yahoo/document/predicate/parser/Predicate.g:58:5: (k= value (not= NOT )? IN (mv= multivalue[k] |r= range[k] ) | TRUE | FALSE )
int alt9=3;
switch ( input.LA(1) ) {
case AND:
case IN:
case INTEGER:
case NOT:
case OR:
case STRING:
case VALUE:
{
alt9=1;
}
break;
case TRUE:
{
int LA9_2 = input.LA(2);
if ( (LA9_2==IN||LA9_2==NOT) ) {
alt9=1;
}
else if ( (LA9_2==EOF||LA9_2==AND||LA9_2==OR||LA9_2==15) ) {
alt9=2;
}
else {
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 9, 2, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case FALSE:
{
int LA9_3 = input.LA(2);
if ( (LA9_3==IN||LA9_3==NOT) ) {
alt9=1;
}
else if ( (LA9_3==EOF||LA9_3==AND||LA9_3==OR||LA9_3==15) ) {
alt9=3;
}
else {
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 9, 3, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 9, 0, input);
throw nvae;
}
switch (alt9) {
case 1 :
// com/yahoo/document/predicate/parser/Predicate.g:58:7: k= value (not= NOT )? IN (mv= multivalue[k] |r= range[k] )
{
pushFollow(FOLLOW_value_in_leaf258);
k=value();
state._fsp--;
// com/yahoo/document/predicate/parser/Predicate.g:58:15: (not= NOT )?
int alt7=2;
int LA7_0 = input.LA(1);
if ( (LA7_0==NOT) ) {
alt7=1;
}
switch (alt7) {
case 1 :
// com/yahoo/document/predicate/parser/Predicate.g:58:17: not= NOT
{
not=(Token)match(input,NOT,FOLLOW_NOT_in_leaf264);
}
break;
}
match(input,IN,FOLLOW_IN_in_leaf269);
// com/yahoo/document/predicate/parser/Predicate.g:59:9: (mv= multivalue[k] |r= range[k] )
int alt8=2;
int LA8_0 = input.LA(1);
if ( (LA8_0==21) ) {
switch ( input.LA(2) ) {
case AND:
case FALSE:
case IN:
case NOT:
case OR:
case STRING:
case TRUE:
case VALUE:
{
alt8=1;
}
break;
case INTEGER:
{
int LA8_3 = input.LA(3);
if ( (LA8_3==17||LA8_3==22) ) {
alt8=1;
}
else if ( (LA8_3==18) ) {
alt8=2;
}
else {
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 3 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 8, 3, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
break;
case 18:
{
alt8=2;
}
break;
default:
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 8, 1, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
NoViableAltException nvae =
new NoViableAltException("", 8, 0, input);
throw nvae;
}
switch (alt8) {
case 1 :
// com/yahoo/document/predicate/parser/Predicate.g:59:11: mv= multivalue[k]
{
pushFollow(FOLLOW_multivalue_in_leaf283);
mv=multivalue(k);
state._fsp--;
n = mv;
}
break;
case 2 :
// com/yahoo/document/predicate/parser/Predicate.g:60:11: r= range[k]
{
pushFollow(FOLLOW_range_in_leaf300);
r=range(k);
state._fsp--;
n = r;
}
break;
}
if (not != null) { n = new Negation(n); }
}
break;
case 2 :
// com/yahoo/document/predicate/parser/Predicate.g:62:7: TRUE
{
match(input,TRUE,FOLLOW_TRUE_in_leaf323);
n = Predicates.value(true);
}
break;
case 3 :
// com/yahoo/document/predicate/parser/Predicate.g:63:7: FALSE
{
match(input,FALSE,FOLLOW_FALSE_in_leaf333);
n = Predicates.value(false);
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return n;
}
// $ANTLR end "leaf"
// $ANTLR start "multivalue"
// com/yahoo/document/predicate/parser/Predicate.g:66:1: multivalue[String key] returns [FeatureSet n] : '[' v1= value ( ',' v2= value )* ']' ;
public final FeatureSet multivalue(String key) throws RecognitionException {
FeatureSet n = null;
String v1 =null;
String v2 =null;
try {
// com/yahoo/document/predicate/parser/Predicate.g:67:5: ( '[' v1= value ( ',' v2= value )* ']' )
// com/yahoo/document/predicate/parser/Predicate.g:67:7: '[' v1= value ( ',' v2= value )* ']'
{
match(input,21,FOLLOW_21_in_multivalue357);
pushFollow(FOLLOW_value_in_multivalue361);
v1=value();
state._fsp--;
n = new FeatureSet(key, v1);
// com/yahoo/document/predicate/parser/Predicate.g:68:11: ( ',' v2= value )*
loop10:
while (true) {
int alt10=2;
int LA10_0 = input.LA(1);
if ( (LA10_0==17) ) {
alt10=1;
}
switch (alt10) {
case 1 :
// com/yahoo/document/predicate/parser/Predicate.g:68:13: ',' v2= value
{
match(input,17,FOLLOW_17_in_multivalue377);
pushFollow(FOLLOW_value_in_multivalue381);
v2=value();
state._fsp--;
n.addValue(v2);
}
break;
default :
break loop10;
}
}
match(input,22,FOLLOW_22_in_multivalue387);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return n;
}
// $ANTLR end "multivalue"
// $ANTLR start "value"
// com/yahoo/document/predicate/parser/Predicate.g:71:1: value returns [String s] : ( VALUE | STRING | INTEGER |k= keyword );
public final String value() throws RecognitionException {
String s = null;
Token VALUE1=null;
Token STRING2=null;
Token INTEGER3=null;
String k =null;
try {
// com/yahoo/document/predicate/parser/Predicate.g:72:5: ( VALUE | STRING | INTEGER |k= keyword )
int alt11=4;
switch ( input.LA(1) ) {
case VALUE:
{
alt11=1;
}
break;
case STRING:
{
alt11=2;
}
break;
case INTEGER:
{
alt11=3;
}
break;
case AND:
case FALSE:
case IN:
case NOT:
case OR:
case TRUE:
{
alt11=4;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 11, 0, input);
throw nvae;
}
switch (alt11) {
case 1 :
// com/yahoo/document/predicate/parser/Predicate.g:72:7: VALUE
{
VALUE1=(Token)match(input,VALUE,FOLLOW_VALUE_in_value408);
s = (VALUE1!=null?VALUE1.getText():null);
}
break;
case 2 :
// com/yahoo/document/predicate/parser/Predicate.g:73:7: STRING
{
STRING2=(Token)match(input,STRING,FOLLOW_STRING_in_value418);
s = (STRING2!=null?STRING2.getText():null);
}
break;
case 3 :
// com/yahoo/document/predicate/parser/Predicate.g:74:7: INTEGER
{
INTEGER3=(Token)match(input,INTEGER,FOLLOW_INTEGER_in_value428);
s = (INTEGER3!=null?INTEGER3.getText():null);
}
break;
case 4 :
// com/yahoo/document/predicate/parser/Predicate.g:75:7: k= keyword
{
pushFollow(FOLLOW_keyword_in_value440);
k=keyword();
state._fsp--;
s = k;
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return s;
}
// $ANTLR end "value"
// $ANTLR start "range"
// com/yahoo/document/predicate/parser/Predicate.g:78:1: range[String key] returns [FeatureRange r] : '[' (i1= INTEGER )? '..' (i2= INTEGER )? ( '(' partition ( ',' partition )* ')' )? ']' ;
public final FeatureRange range(String key) throws RecognitionException {
FeatureRange r = null;
Token i1=null;
Token i2=null;
try {
// com/yahoo/document/predicate/parser/Predicate.g:79:5: ( '[' (i1= INTEGER )? '..' (i2= INTEGER )? ( '(' partition ( ',' partition )* ')' )? ']' )
// com/yahoo/document/predicate/parser/Predicate.g:79:7: '[' (i1= INTEGER )? '..' (i2= INTEGER )? ( '(' partition ( ',' partition )* ')' )? ']'
{
match(input,21,FOLLOW_21_in_range464);
r = new FeatureRange(key);
// com/yahoo/document/predicate/parser/Predicate.g:80:11: (i1= INTEGER )?
int alt12=2;
int LA12_0 = input.LA(1);
if ( (LA12_0==INTEGER) ) {
alt12=1;
}
switch (alt12) {
case 1 :
// com/yahoo/document/predicate/parser/Predicate.g:80:13: i1= INTEGER
{
i1=(Token)match(input,INTEGER,FOLLOW_INTEGER_in_range482);
r.setFromInclusive(Long.parseLong(i1.getText()));
}
break;
}
match(input,18,FOLLOW_18_in_range499);
// com/yahoo/document/predicate/parser/Predicate.g:82:11: (i2= INTEGER )?
int alt13=2;
int LA13_0 = input.LA(1);
if ( (LA13_0==INTEGER) ) {
alt13=1;
}
switch (alt13) {
case 1 :
// com/yahoo/document/predicate/parser/Predicate.g:82:13: i2= INTEGER
{
i2=(Token)match(input,INTEGER,FOLLOW_INTEGER_in_range515);
r.setToInclusive(Long.parseLong(i2.getText()));
}
break;
}
// com/yahoo/document/predicate/parser/Predicate.g:83:11: ( '(' partition ( ',' partition )* ')' )?
int alt15=2;
int LA15_0 = input.LA(1);
if ( (LA15_0==14) ) {
alt15=1;
}
switch (alt15) {
case 1 :
// com/yahoo/document/predicate/parser/Predicate.g:83:13: '(' partition ( ',' partition )* ')'
{
match(input,14,FOLLOW_14_in_range534);
pushFollow(FOLLOW_partition_in_range536);
partition();
state._fsp--;
// com/yahoo/document/predicate/parser/Predicate.g:83:27: ( ',' partition )*
loop14:
while (true) {
int alt14=2;
int LA14_0 = input.LA(1);
if ( (LA14_0==17) ) {
alt14=1;
}
switch (alt14) {
case 1 :
// com/yahoo/document/predicate/parser/Predicate.g:83:28: ',' partition
{
match(input,17,FOLLOW_17_in_range539);
pushFollow(FOLLOW_partition_in_range541);
partition();
state._fsp--;
}
break;
default :
break loop14;
}
}
match(input,15,FOLLOW_15_in_range545);
}
break;
}
match(input,22,FOLLOW_22_in_range556);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return r;
}
// $ANTLR end "range"
// $ANTLR start "partition"
// com/yahoo/document/predicate/parser/Predicate.g:87:1: partition : value ( '=' | '=-' ) ( INTEGER INTEGER | INTEGER '+[' ( INTEGER )? '..' ( INTEGER )? ']' ) ;
public final void partition() throws RecognitionException {
try {
// com/yahoo/document/predicate/parser/Predicate.g:88:5: ( value ( '=' | '=-' ) ( INTEGER INTEGER | INTEGER '+[' ( INTEGER )? '..' ( INTEGER )? ']' ) )
// com/yahoo/document/predicate/parser/Predicate.g:88:7: value ( '=' | '=-' ) ( INTEGER INTEGER | INTEGER '+[' ( INTEGER )? '..' ( INTEGER )? ']' )
{
pushFollow(FOLLOW_value_in_partition573);
value();
state._fsp--;
if ( (input.LA(1) >= 19 && input.LA(1) <= 20) ) {
input.consume();
state.errorRecovery=false;
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
throw mse;
}
// com/yahoo/document/predicate/parser/Predicate.g:88:24: ( INTEGER INTEGER | INTEGER '+[' ( INTEGER )? '..' ( INTEGER )? ']' )
int alt18=2;
int LA18_0 = input.LA(1);
if ( (LA18_0==INTEGER) ) {
int LA18_1 = input.LA(2);
if ( (LA18_1==INTEGER) ) {
alt18=1;
}
else if ( (LA18_1==16) ) {
alt18=2;
}
else {
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 18, 1, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
NoViableAltException nvae =
new NoViableAltException("", 18, 0, input);
throw nvae;
}
switch (alt18) {
case 1 :
// com/yahoo/document/predicate/parser/Predicate.g:88:25: INTEGER INTEGER
{
match(input,INTEGER,FOLLOW_INTEGER_in_partition582);
match(input,INTEGER,FOLLOW_INTEGER_in_partition584);
}
break;
case 2 :
// com/yahoo/document/predicate/parser/Predicate.g:88:81: INTEGER '+[' ( INTEGER )? '..' ( INTEGER )? ']'
{
match(input,INTEGER,FOLLOW_INTEGER_in_partition590);
match(input,16,FOLLOW_16_in_partition592);
// com/yahoo/document/predicate/parser/Predicate.g:88:94: ( INTEGER )?
int alt16=2;
int LA16_0 = input.LA(1);
if ( (LA16_0==INTEGER) ) {
alt16=1;
}
switch (alt16) {
case 1 :
// com/yahoo/document/predicate/parser/Predicate.g:88:94: INTEGER
{
match(input,INTEGER,FOLLOW_INTEGER_in_partition594);
}
break;
}
match(input,18,FOLLOW_18_in_partition597);
// com/yahoo/document/predicate/parser/Predicate.g:88:108: ( INTEGER )?
int alt17=2;
int LA17_0 = input.LA(1);
if ( (LA17_0==INTEGER) ) {
alt17=1;
}
switch (alt17) {
case 1 :
// com/yahoo/document/predicate/parser/Predicate.g:88:108: INTEGER
{
match(input,INTEGER,FOLLOW_INTEGER_in_partition599);
}
break;
}
match(input,22,FOLLOW_22_in_partition602);
}
break;
}
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "partition"
// $ANTLR start "keyword"
// com/yahoo/document/predicate/parser/Predicate.g:91:1: keyword returns [String s] : ( OR | AND | NOT | IN | TRUE | FALSE );
public final String keyword() throws RecognitionException {
String s = null;
Token OR4=null;
Token AND5=null;
Token NOT6=null;
Token IN7=null;
Token TRUE8=null;
Token FALSE9=null;
try {
// com/yahoo/document/predicate/parser/Predicate.g:92:5: ( OR | AND | NOT | IN | TRUE | FALSE )
int alt19=6;
switch ( input.LA(1) ) {
case OR:
{
alt19=1;
}
break;
case AND:
{
alt19=2;
}
break;
case NOT:
{
alt19=3;
}
break;
case IN:
{
alt19=4;
}
break;
case TRUE:
{
alt19=5;
}
break;
case FALSE:
{
alt19=6;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 19, 0, input);
throw nvae;
}
switch (alt19) {
case 1 :
// com/yahoo/document/predicate/parser/Predicate.g:92:7: OR
{
OR4=(Token)match(input,OR,FOLLOW_OR_in_keyword624);
s = (OR4!=null?OR4.getText():null);
}
break;
case 2 :
// com/yahoo/document/predicate/parser/Predicate.g:93:7: AND
{
AND5=(Token)match(input,AND,FOLLOW_AND_in_keyword635);
s = (AND5!=null?AND5.getText():null);
}
break;
case 3 :
// com/yahoo/document/predicate/parser/Predicate.g:94:7: NOT
{
NOT6=(Token)match(input,NOT,FOLLOW_NOT_in_keyword645);
s = (NOT6!=null?NOT6.getText():null);
}
break;
case 4 :
// com/yahoo/document/predicate/parser/Predicate.g:95:7: IN
{
IN7=(Token)match(input,IN,FOLLOW_IN_in_keyword655);
s = (IN7!=null?IN7.getText():null);
}
break;
case 5 :
// com/yahoo/document/predicate/parser/Predicate.g:96:7: TRUE
{
TRUE8=(Token)match(input,TRUE,FOLLOW_TRUE_in_keyword666);
s = (TRUE8!=null?TRUE8.getText():null);
}
break;
case 6 :
// com/yahoo/document/predicate/parser/Predicate.g:97:7: FALSE
{
FALSE9=(Token)match(input,FALSE,FOLLOW_FALSE_in_keyword676);
s = (FALSE9!=null?FALSE9.getText():null);
}
break;
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return s;
}
// $ANTLR end "keyword"
// Delegated rules
public static final BitSet FOLLOW_disjunction_in_predicate51 = new BitSet(new long[]{0x0000000000000000L});
public static final BitSet FOLLOW_EOF_in_predicate53 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_conjunction_in_disjunction78 = new BitSet(new long[]{0x0000000000000202L});
public static final BitSet FOLLOW_OR_in_disjunction90 = new BitSet(new long[]{0x0000000000005FF0L});
public static final BitSet FOLLOW_conjunction_in_disjunction94 = new BitSet(new long[]{0x0000000000000202L});
public static final BitSet FOLLOW_OR_in_disjunction108 = new BitSet(new long[]{0x0000000000005FF0L});
public static final BitSet FOLLOW_conjunction_in_disjunction112 = new BitSet(new long[]{0x0000000000000202L});
public static final BitSet FOLLOW_unary_node_in_conjunction143 = new BitSet(new long[]{0x0000000000000012L});
public static final BitSet FOLLOW_AND_in_conjunction155 = new BitSet(new long[]{0x0000000000005FF0L});
public static final BitSet FOLLOW_unary_node_in_conjunction159 = new BitSet(new long[]{0x0000000000000012L});
public static final BitSet FOLLOW_AND_in_conjunction173 = new BitSet(new long[]{0x0000000000005FF0L});
public static final BitSet FOLLOW_unary_node_in_conjunction177 = new BitSet(new long[]{0x0000000000000012L});
public static final BitSet FOLLOW_leaf_in_unary_node208 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_NOT_in_unary_node222 = new BitSet(new long[]{0x0000000000004000L});
public static final BitSet FOLLOW_14_in_unary_node227 = new BitSet(new long[]{0x0000000000005FF0L});
public static final BitSet FOLLOW_disjunction_in_unary_node231 = new BitSet(new long[]{0x0000000000008000L});
public static final BitSet FOLLOW_15_in_unary_node233 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_value_in_leaf258 = new BitSet(new long[]{0x0000000000000140L});
public static final BitSet FOLLOW_NOT_in_leaf264 = new BitSet(new long[]{0x0000000000000040L});
public static final BitSet FOLLOW_IN_in_leaf269 = new BitSet(new long[]{0x0000000000200000L});
public static final BitSet FOLLOW_multivalue_in_leaf283 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_range_in_leaf300 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_TRUE_in_leaf323 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_FALSE_in_leaf333 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_21_in_multivalue357 = new BitSet(new long[]{0x0000000000001FF0L});
public static final BitSet FOLLOW_value_in_multivalue361 = new BitSet(new long[]{0x0000000000420000L});
public static final BitSet FOLLOW_17_in_multivalue377 = new BitSet(new long[]{0x0000000000001FF0L});
public static final BitSet FOLLOW_value_in_multivalue381 = new BitSet(new long[]{0x0000000000420000L});
public static final BitSet FOLLOW_22_in_multivalue387 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_VALUE_in_value408 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_STRING_in_value418 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_INTEGER_in_value428 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_keyword_in_value440 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_21_in_range464 = new BitSet(new long[]{0x0000000000040080L});
public static final BitSet FOLLOW_INTEGER_in_range482 = new BitSet(new long[]{0x0000000000040000L});
public static final BitSet FOLLOW_18_in_range499 = new BitSet(new long[]{0x0000000000404080L});
public static final BitSet FOLLOW_INTEGER_in_range515 = new BitSet(new long[]{0x0000000000404000L});
public static final BitSet FOLLOW_14_in_range534 = new BitSet(new long[]{0x0000000000001FF0L});
public static final BitSet FOLLOW_partition_in_range536 = new BitSet(new long[]{0x0000000000028000L});
public static final BitSet FOLLOW_17_in_range539 = new BitSet(new long[]{0x0000000000001FF0L});
public static final BitSet FOLLOW_partition_in_range541 = new BitSet(new long[]{0x0000000000028000L});
public static final BitSet FOLLOW_15_in_range545 = new BitSet(new long[]{0x0000000000400000L});
public static final BitSet FOLLOW_22_in_range556 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_value_in_partition573 = new BitSet(new long[]{0x0000000000180000L});
public static final BitSet FOLLOW_set_in_partition575 = new BitSet(new long[]{0x0000000000000080L});
public static final BitSet FOLLOW_INTEGER_in_partition582 = new BitSet(new long[]{0x0000000000000080L});
public static final BitSet FOLLOW_INTEGER_in_partition584 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_INTEGER_in_partition590 = new BitSet(new long[]{0x0000000000010000L});
public static final BitSet FOLLOW_16_in_partition592 = new BitSet(new long[]{0x0000000000040080L});
public static final BitSet FOLLOW_INTEGER_in_partition594 = new BitSet(new long[]{0x0000000000040000L});
public static final BitSet FOLLOW_18_in_partition597 = new BitSet(new long[]{0x0000000000400080L});
public static final BitSet FOLLOW_INTEGER_in_partition599 = new BitSet(new long[]{0x0000000000400000L});
public static final BitSet FOLLOW_22_in_partition602 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_OR_in_keyword624 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_AND_in_keyword635 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_NOT_in_keyword645 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_IN_in_keyword655 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_TRUE_in_keyword666 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_FALSE_in_keyword676 = new BitSet(new long[]{0x0000000000000002L});
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy