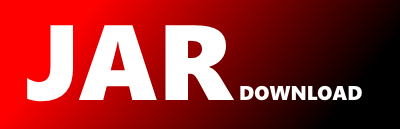
com.yahoo.routing.config.ZoneConfig Maven / Gradle / Ivy
/**
* This file is generated from a config definition file.
* ------------ D O N O T E D I T ! ------------
*/
package com.yahoo.routing.config;
import java.util.*;
import java.io.File;
import java.nio.file.Path;
import com.yahoo.config.*;
/**
* This class represents the root node of zone
*
* Copyright Yahoo. Licensed under the terms of the Apache 2.0 license. See LICENSE in the project root.
*/
public final class ZoneConfig extends ConfigInstance {
public final static String CONFIG_DEF_MD5 = "5d3b9e317eec1d697032825439272841";
public final static String CONFIG_DEF_NAME = "zone";
public final static String CONFIG_DEF_NAMESPACE = "routing.config";
public final static String CONFIG_DEF_VERSION = "";
public final static String[] CONFIG_DEF_SCHEMA = {
"namespace=routing.config",
"configserverVipUrl string",
"configserverAthenzDomain string",
"configserverAthenzServiceName string",
"configserver[] string"
};
public static String getDefMd5() { return CONFIG_DEF_MD5; }
public static String getDefName() { return CONFIG_DEF_NAME; }
public static String getDefNamespace() { return CONFIG_DEF_NAMESPACE; }
public static String getDefVersion() { return CONFIG_DEF_VERSION; }
public interface Producer extends ConfigInstance.Producer {
void getConfig(Builder builder);
}
public static class Builder implements ConfigInstance.Builder {
private Set __uninitialized = new HashSet(Arrays.asList(
"configserverVipUrl",
"configserverAthenzDomain",
"configserverAthenzServiceName"
));
private String configserverVipUrl = null;
private String configserverAthenzDomain = null;
private String configserverAthenzServiceName = null;
public List configserver = new ArrayList<>();
public Builder() { }
public Builder(ZoneConfig config) {
configserverVipUrl(config.configserverVipUrl());
configserverAthenzDomain(config.configserverAthenzDomain());
configserverAthenzServiceName(config.configserverAthenzServiceName());
configserver(config.configserver());
}
private Builder override(Builder __superior) {
if (__superior.configserverVipUrl != null)
configserverVipUrl(__superior.configserverVipUrl);
if (__superior.configserverAthenzDomain != null)
configserverAthenzDomain(__superior.configserverAthenzDomain);
if (__superior.configserverAthenzServiceName != null)
configserverAthenzServiceName(__superior.configserverAthenzServiceName);
if (!__superior.configserver.isEmpty())
configserver.addAll(__superior.configserver);
return this;
}
public Builder configserverVipUrl(String __value) {
if (__value == null) throw new IllegalArgumentException("Null value is not allowed.");
configserverVipUrl = __value;
__uninitialized.remove("configserverVipUrl");
return this;
}
public Builder configserverAthenzDomain(String __value) {
if (__value == null) throw new IllegalArgumentException("Null value is not allowed.");
configserverAthenzDomain = __value;
__uninitialized.remove("configserverAthenzDomain");
return this;
}
public Builder configserverAthenzServiceName(String __value) {
if (__value == null) throw new IllegalArgumentException("Null value is not allowed.");
configserverAthenzServiceName = __value;
__uninitialized.remove("configserverAthenzServiceName");
return this;
}
public Builder configserver(String __value) {
configserver.add(__value);
return this;
}
public Builder configserver(Collection __values) {
configserver.addAll(__values);
return this;
}
private boolean _applyOnRestart = false;
@java.lang.Override
public final boolean dispatchGetConfig(ConfigInstance.Producer producer) {
if (producer instanceof Producer) {
((Producer)producer).getConfig(this);
return true;
}
return false;
}
@java.lang.Override
public final String getDefMd5() { return CONFIG_DEF_MD5; }
@java.lang.Override
public final String getDefName() { return CONFIG_DEF_NAME; }
@java.lang.Override
public final String getDefNamespace() { return CONFIG_DEF_NAMESPACE; }
@java.lang.Override
public final boolean getApplyOnRestart() { return _applyOnRestart; }
@java.lang.Override
public final void setApplyOnRestart(boolean applyOnRestart) { _applyOnRestart = applyOnRestart; }
public ZoneConfig build() {
return new ZoneConfig(this);
}
}
// URL to config server load balancer
private final StringNode configserverVipUrl;
// Athenz domain/service name of config server
private final StringNode configserverAthenzDomain;
private final StringNode configserverAthenzServiceName;
// Tenant config server endpoint, used to fetch tenant (mapping) info
// Auto detected if empty (from VESPA_CONFIG_SOURCES)
// E.g.: tcp/cfg1.example.com:19070
private final LeafNodeVector configserver;
public ZoneConfig(Builder builder) {
this(builder, true);
}
private ZoneConfig(Builder builder, boolean throwIfUninitialized) {
if (throwIfUninitialized && ! builder.__uninitialized.isEmpty())
throw new IllegalArgumentException("The following builder parameters for " +
"zone must be initialized: " + builder.__uninitialized);
configserverVipUrl = (builder.configserverVipUrl == null) ?
new StringNode() : new StringNode(builder.configserverVipUrl);
configserverAthenzDomain = (builder.configserverAthenzDomain == null) ?
new StringNode() : new StringNode(builder.configserverAthenzDomain);
configserverAthenzServiceName = (builder.configserverAthenzServiceName == null) ?
new StringNode() : new StringNode(builder.configserverAthenzServiceName);
configserver = new LeafNodeVector<>(builder.configserver, new StringNode());
}
/**
* @return zone.configserverVipUrl
*/
public String configserverVipUrl() {
return configserverVipUrl.value();
}
/**
* @return zone.configserverAthenzDomain
*/
public String configserverAthenzDomain() {
return configserverAthenzDomain.value();
}
/**
* @return zone.configserverAthenzServiceName
*/
public String configserverAthenzServiceName() {
return configserverAthenzServiceName.value();
}
/**
* @return zone.configserver[]
*/
public List configserver() {
return configserver.asList();
}
/**
* @param i the index of the value to return
* @return zone.configserver[]
*/
public String configserver(int i) {
return configserver.get(i).value();
}
private ChangesRequiringRestart getChangesRequiringRestart(ZoneConfig newConfig) {
ChangesRequiringRestart changes = new ChangesRequiringRestart("zone");
return changes;
}
private static boolean containsFieldsFlaggedWithRestart() {
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy