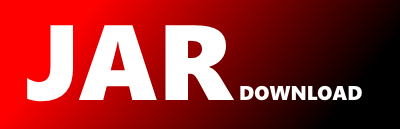
ai.vespa.schemals.parser.rankingexpression.Token Maven / Gradle / Ivy
/*
* Generated by: CongoCC Parser Generator. Token.java
*/
package ai.vespa.schemals.parser.rankingexpression;
import ai.vespa.schemals.parser.rankingexpression.ast.*;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
public class Token implements CharSequence, Node.TerminalNode {
public enum TokenType implements Node.NodeType {
EOF, LBRACE, RBRACE, LSQUARE, RSQUARE, LCURLY, RCURLY, ADD, SUB, DIV, MUL,
DOT, MOD, POWOP, DOLLAR, COMMA, COLON, GREATEREQUAL, GREATER, LESSEQUAL, LESS,
APPROX, NOTEQUAL, EQUAL, IF, IN, F, NOT, AND, OR, ABS, ACOS, ASIN, ATAN, CEIL,
COS, COSH, ELU, EXP, FABS, FLOOR, ISNAN, LOG, LOG10, RELU, ROUND, SIGMOID,
SIGN, SIN, SINH, SQUARE, SQRT, TAN, TANH, ERF, ATAN2, FMOD, LDEXP, POW, BIT,
HAMMING, MAP, MAP_SUBSPACES, UNPACK_BITS, REDUCE, JOIN, MERGE, RENAME, CONCAT,
TENSOR, RANGE, DIAG, RANDOM, L1_NORMALIZE, L2_NORMALIZE, EUCLIDEAN_DISTANCE,
COSINE_SIMILARITY, MATMUL, SOFTMAX, XW_PLUS_B, ARGMAX, ARGMIN, CELL_CAST,
EXPAND, AVG, COUNT, MAX, MEDIAN, MIN, PROD, SUM, TRUE, FALSE, _TOKEN_93, INTEGER,
FLOAT, STRING, IDENTIFIER, SINGLE_LINE_COMMENT, DUMMY, INVALID;
public boolean isUndefined() {
return this == DUMMY;
}
public boolean isInvalid() {
return this == INVALID;
}
public boolean isEOF() {
return this == EOF;
}
}
private RankingExpressionParserLexer tokenSource;
private TokenType type = TokenType.DUMMY;
private int beginOffset;
private int endOffset;
private boolean unparsed;
private Node parent;
/**
* It would be extremely rare that an application
* programmer would use this method. It needs to
* be public because it is part of the ai.vespa.schemals.parser.rankingexpression.Node interface.
*/
public void setBeginOffset(int beginOffset) {
this.beginOffset = beginOffset;
}
/**
* It would be extremely rare that an application
* programmer would use this method. It needs to
* be public because it is part of the ai.vespa.schemals.parser.rankingexpression.Node interface.
*/
public void setEndOffset(int endOffset) {
this.endOffset = endOffset;
}
/**
* @return the RankingExpressionParserLexer object that handles
* location info for the tokens.
*/
public RankingExpressionParserLexer getTokenSource() {
return this.tokenSource;
}
/**
* It should be exceedingly rare that an application
* programmer needs to use this method.
*/
public void setTokenSource(TokenSource tokenSource) {
this.tokenSource = (RankingExpressionParserLexer) tokenSource;
}
public boolean isInvalid() {
return getType().isInvalid();
}
/**
* Return the TokenType of this Token object
*/
@Override
public TokenType getType() {
return type;
}
protected void setType(TokenType type) {
this.type = type;
}
/**
* @return whether this Token represent actual input or was it inserted somehow?
*/
public boolean isVirtual() {
return virtual || type == TokenType.EOF;
}
/**
* @return Did we skip this token in parsing?
*/
public boolean isSkipped() {
return skipped;
}
private boolean virtual;
private boolean skipped;
private boolean dirty;
void setVirtual(boolean virtual) {
this.virtual = virtual;
if (virtual) dirty = true;
}
void setSkipped(boolean skipped) {
this.skipped = skipped;
if (skipped) dirty = true;
}
public boolean isDirty() {
return dirty;
}
public void setDirty(boolean dirty) {
this.dirty = dirty;
}
public int getBeginOffset() {
return beginOffset;
}
public int getEndOffset() {
return endOffset;
}
/**
* @return the string image of the token.
*/
@Override
/**
* @return the next _cached_ regular (i.e. parsed) token
* or null
*/
public final Token getNext() {
return getNextParsedToken();
}
/**
* @return the previous regular (i.e. parsed) token
* or null
*/
public final Token getPrevious() {
Token result = previousCachedToken();
while (result != null && result.isUnparsed()) {
result = result.previousCachedToken();
}
return result;
}
/**
* @return the next regular (i.e. parsed) token
*/
private Token getNextParsedToken() {
Token result = nextCachedToken();
while (result != null && result.isUnparsed()) {
result = result.nextCachedToken();
}
return result;
}
/**
* @return the next token of any sort (parsed or unparsed or invalid)
*/
public Token nextCachedToken() {
if (getType() == TokenType.EOF) return null;
RankingExpressionParserLexer tokenSource = getTokenSource();
return tokenSource != null ? (Token) tokenSource.nextCachedToken(getEndOffset()) : null;
}
public Token previousCachedToken() {
if (getTokenSource() == null) return null;
return (Token) getTokenSource().previousCachedToken(getBeginOffset());
}
Token getPreviousToken() {
return previousCachedToken();
}
public Token replaceType(TokenType type) {
Token result = newToken(type, getTokenSource(), getBeginOffset(), getEndOffset());
getTokenSource().cacheToken(result);
return result;
}
public String getSource() {
if (type == TokenType.EOF) return "";
RankingExpressionParserLexer ts = getTokenSource();
return ts == null ? null : ts.getText(getBeginOffset(), getEndOffset());
}
protected Token() {
}
public Token(TokenType type, RankingExpressionParserLexer tokenSource, int beginOffset, int endOffset) {
this.type = type;
this.tokenSource = tokenSource;
this.beginOffset = beginOffset;
this.endOffset = endOffset;
}
public boolean isUnparsed() {
return unparsed;
}
public void setUnparsed(boolean unparsed) {
this.unparsed = unparsed;
}
/**
* @return An iterator of the tokens preceding this one.
*/
public Iterator precedingTokens() {
return new Iterator() {
Token currentPoint = Token.this;
public boolean hasNext() {
return currentPoint.previousCachedToken() != null;
}
public Token next() {
Token previous = currentPoint.previousCachedToken();
if (previous == null) throw new java.util.NoSuchElementException("No previous token!");
return currentPoint = previous;
}
};
}
/**
* @return a list of the unparsed tokens preceding this one in the order they appear in the input
*/
public List precedingUnparsedTokens() {
List result = new ArrayList<>();
Token t = this.previousCachedToken();
while (t != null && t.isUnparsed()) {
result.add(t);
t = t.previousCachedToken();
}
Collections.reverse(result);
return result;
}
/**
* @return An iterator of the (cached) tokens that follow this one.
*/
public Iterator followingTokens() {
return new java.util.Iterator() {
Token currentPoint = Token.this;
public boolean hasNext() {
return currentPoint.nextCachedToken() != null;
}
public Token next() {
Token next = currentPoint.nextCachedToken();
if (next == null) throw new java.util.NoSuchElementException("No next token!");
return currentPoint = next;
}
};
}
public void copyLocationInfo(Token from) {
setTokenSource(from.getTokenSource());
setBeginOffset(from.getBeginOffset());
setEndOffset(from.getEndOffset());
}
public void copyLocationInfo(Token start, Token end) {
setTokenSource(start.getTokenSource());
if (tokenSource == null) setTokenSource(end.getTokenSource());
setBeginOffset(start.getBeginOffset());
setEndOffset(end.getEndOffset());
}
public static Token newToken(TokenType type, RankingExpressionParserLexer tokenSource, int beginOffset, int endOffset) {
switch(type) {
case CELL_CAST :
return new CELL_CAST(TokenType.CELL_CAST, tokenSource, beginOffset, endOffset);
case SQRT :
return new SQRT(TokenType.SQRT, tokenSource, beginOffset, endOffset);
case JOIN :
return new JOIN(TokenType.JOIN, tokenSource, beginOffset, endOffset);
case LOG10 :
return new LOG10(TokenType.LOG10, tokenSource, beginOffset, endOffset);
case RSQUARE :
return new RSQUARE(TokenType.RSQUARE, tokenSource, beginOffset, endOffset);
case TENSOR :
return new TENSOR(TokenType.TENSOR, tokenSource, beginOffset, endOffset);
case FLOOR :
return new FLOOR(TokenType.FLOOR, tokenSource, beginOffset, endOffset);
case CEIL :
return new CEIL(TokenType.CEIL, tokenSource, beginOffset, endOffset);
case IF :
return new IF(TokenType.IF, tokenSource, beginOffset, endOffset);
case LESSEQUAL :
return new LESSEQUAL(TokenType.LESSEQUAL, tokenSource, beginOffset, endOffset);
case SUB :
return new SUB(TokenType.SUB, tokenSource, beginOffset, endOffset);
case EXPAND :
return new EXPAND(TokenType.EXPAND, tokenSource, beginOffset, endOffset);
case SQUARE :
return new SQUARE(TokenType.SQUARE, tokenSource, beginOffset, endOffset);
case IN :
return new IN(TokenType.IN, tokenSource, beginOffset, endOffset);
case LOG :
return new LOG(TokenType.LOG, tokenSource, beginOffset, endOffset);
case DOT :
return new DOT(TokenType.DOT, tokenSource, beginOffset, endOffset);
case ATAN2 :
return new ATAN2(TokenType.ATAN2, tokenSource, beginOffset, endOffset);
case SUM :
return new SUM(TokenType.SUM, tokenSource, beginOffset, endOffset);
case SIGMOID :
return new SIGMOID(TokenType.SIGMOID, tokenSource, beginOffset, endOffset);
case MIN :
return new MIN(TokenType.MIN, tokenSource, beginOffset, endOffset);
case ERF :
return new ERF(TokenType.ERF, tokenSource, beginOffset, endOffset);
case POW :
return new POW(TokenType.POW, tokenSource, beginOffset, endOffset);
case DOLLAR :
return new DOLLAR(TokenType.DOLLAR, tokenSource, beginOffset, endOffset);
case COUNT :
return new COUNT(TokenType.COUNT, tokenSource, beginOffset, endOffset);
case MAP :
return new MAP(TokenType.MAP, tokenSource, beginOffset, endOffset);
case LBRACE :
return new LBRACE(TokenType.LBRACE, tokenSource, beginOffset, endOffset);
case MATMUL :
return new MATMUL(TokenType.MATMUL, tokenSource, beginOffset, endOffset);
case MERGE :
return new MERGE(TokenType.MERGE, tokenSource, beginOffset, endOffset);
case MAX :
return new MAX(TokenType.MAX, tokenSource, beginOffset, endOffset);
case F :
return new F(TokenType.F, tokenSource, beginOffset, endOffset);
case DIAG :
return new DIAG(TokenType.DIAG, tokenSource, beginOffset, endOffset);
case XW_PLUS_B :
return new XW_PLUS_B(TokenType.XW_PLUS_B, tokenSource, beginOffset, endOffset);
case COSINE_SIMILARITY :
return new COSINE_SIMILARITY(TokenType.COSINE_SIMILARITY, tokenSource, beginOffset, endOffset);
case ABS :
return new ABS(TokenType.ABS, tokenSource, beginOffset, endOffset);
case MEDIAN :
return new MEDIAN(TokenType.MEDIAN, tokenSource, beginOffset, endOffset);
case RCURLY :
return new RCURLY(TokenType.RCURLY, tokenSource, beginOffset, endOffset);
case COMMA :
return new COMMA(TokenType.COMMA, tokenSource, beginOffset, endOffset);
case L1_NORMALIZE :
return new L1_NORMALIZE(TokenType.L1_NORMALIZE, tokenSource, beginOffset, endOffset);
case COS :
return new COS(TokenType.COS, tokenSource, beginOffset, endOffset);
case RANDOM :
return new RANDOM(TokenType.RANDOM, tokenSource, beginOffset, endOffset);
case GREATER :
return new GREATER(TokenType.GREATER, tokenSource, beginOffset, endOffset);
case LCURLY :
return new LCURLY(TokenType.LCURLY, tokenSource, beginOffset, endOffset);
case FABS :
return new FABS(TokenType.FABS, tokenSource, beginOffset, endOffset);
case APPROX :
return new APPROX(TokenType.APPROX, tokenSource, beginOffset, endOffset);
case DIV :
return new DIV(TokenType.DIV, tokenSource, beginOffset, endOffset);
case PROD :
return new PROD(TokenType.PROD, tokenSource, beginOffset, endOffset);
case STRING :
return new STRING(TokenType.STRING, tokenSource, beginOffset, endOffset);
case TAN :
return new TAN(TokenType.TAN, tokenSource, beginOffset, endOffset);
case ADD :
return new ADD(TokenType.ADD, tokenSource, beginOffset, endOffset);
case UNPACK_BITS :
return new UNPACK_BITS(TokenType.UNPACK_BITS, tokenSource, beginOffset, endOffset);
case ELU :
return new ELU(TokenType.ELU, tokenSource, beginOffset, endOffset);
case L2_NORMALIZE :
return new L2_NORMALIZE(TokenType.L2_NORMALIZE, tokenSource, beginOffset, endOffset);
case POWOP :
return new POWOP(TokenType.POWOP, tokenSource, beginOffset, endOffset);
case ACOS :
return new ACOS(TokenType.ACOS, tokenSource, beginOffset, endOffset);
case SIGN :
return new SIGN(TokenType.SIGN, tokenSource, beginOffset, endOffset);
case INTEGER :
return new INTEGER(TokenType.INTEGER, tokenSource, beginOffset, endOffset);
case MUL :
return new MUL(TokenType.MUL, tokenSource, beginOffset, endOffset);
case TRUE :
return new TRUE(TokenType.TRUE, tokenSource, beginOffset, endOffset);
case SOFTMAX :
return new SOFTMAX(TokenType.SOFTMAX, tokenSource, beginOffset, endOffset);
case NOTEQUAL :
return new NOTEQUAL(TokenType.NOTEQUAL, tokenSource, beginOffset, endOffset);
case NOT :
return new NOT(TokenType.NOT, tokenSource, beginOffset, endOffset);
case AVG :
return new AVG(TokenType.AVG, tokenSource, beginOffset, endOffset);
case ARGMIN :
return new ARGMIN(TokenType.ARGMIN, tokenSource, beginOffset, endOffset);
case RBRACE :
return new RBRACE(TokenType.RBRACE, tokenSource, beginOffset, endOffset);
case SINH :
return new SINH(TokenType.SINH, tokenSource, beginOffset, endOffset);
case AND :
return new AND(TokenType.AND, tokenSource, beginOffset, endOffset);
case SIN :
return new SIN(TokenType.SIN, tokenSource, beginOffset, endOffset);
case LESS :
return new LESS(TokenType.LESS, tokenSource, beginOffset, endOffset);
case ISNAN :
return new ISNAN(TokenType.ISNAN, tokenSource, beginOffset, endOffset);
case ATAN :
return new ATAN(TokenType.ATAN, tokenSource, beginOffset, endOffset);
case MAP_SUBSPACES :
return new MAP_SUBSPACES(TokenType.MAP_SUBSPACES, tokenSource, beginOffset, endOffset);
case REDUCE :
return new REDUCE(TokenType.REDUCE, tokenSource, beginOffset, endOffset);
case RANGE :
return new RANGE(TokenType.RANGE, tokenSource, beginOffset, endOffset);
case TANH :
return new TANH(TokenType.TANH, tokenSource, beginOffset, endOffset);
case ARGMAX :
return new ARGMAX(TokenType.ARGMAX, tokenSource, beginOffset, endOffset);
case FLOAT :
return new FLOAT(TokenType.FLOAT, tokenSource, beginOffset, endOffset);
case HAMMING :
return new HAMMING(TokenType.HAMMING, tokenSource, beginOffset, endOffset);
case ROUND :
return new ROUND(TokenType.ROUND, tokenSource, beginOffset, endOffset);
case CONCAT :
return new CONCAT(TokenType.CONCAT, tokenSource, beginOffset, endOffset);
case ASIN :
return new ASIN(TokenType.ASIN, tokenSource, beginOffset, endOffset);
case LDEXP :
return new LDEXP(TokenType.LDEXP, tokenSource, beginOffset, endOffset);
case SINGLE_LINE_COMMENT :
return new SINGLE_LINE_COMMENT(TokenType.SINGLE_LINE_COMMENT, tokenSource, beginOffset, endOffset);
case RELU :
return new RELU(TokenType.RELU, tokenSource, beginOffset, endOffset);
case IDENTIFIER :
return new IDENTIFIER(TokenType.IDENTIFIER, tokenSource, beginOffset, endOffset);
case COSH :
return new COSH(TokenType.COSH, tokenSource, beginOffset, endOffset);
case RENAME :
return new RENAME(TokenType.RENAME, tokenSource, beginOffset, endOffset);
case MOD :
return new MOD(TokenType.MOD, tokenSource, beginOffset, endOffset);
case OR :
return new OR(TokenType.OR, tokenSource, beginOffset, endOffset);
case EQUAL :
return new EQUAL(TokenType.EQUAL, tokenSource, beginOffset, endOffset);
case COLON :
return new COLON(TokenType.COLON, tokenSource, beginOffset, endOffset);
case BIT :
return new BIT(TokenType.BIT, tokenSource, beginOffset, endOffset);
case LSQUARE :
return new LSQUARE(TokenType.LSQUARE, tokenSource, beginOffset, endOffset);
case EUCLIDEAN_DISTANCE :
return new EUCLIDEAN_DISTANCE(TokenType.EUCLIDEAN_DISTANCE, tokenSource, beginOffset, endOffset);
case GREATEREQUAL :
return new GREATEREQUAL(TokenType.GREATEREQUAL, tokenSource, beginOffset, endOffset);
case FALSE :
return new FALSE(TokenType.FALSE, tokenSource, beginOffset, endOffset);
case EXP :
return new EXP(TokenType.EXP, tokenSource, beginOffset, endOffset);
case FMOD :
return new FMOD(TokenType.FMOD, tokenSource, beginOffset, endOffset);
case INVALID :
return new InvalidToken(tokenSource, beginOffset, endOffset);
default :
return new Token(type, tokenSource, beginOffset, endOffset);
}
}
public String getLocation() {
return getInputSource() + ":" + getBeginLine() + ":" + getBeginColumn();
}
public Node getParent() {
return parent;
}
public void setParent(Node parent) {
this.parent = parent;
}
public boolean isEmpty() {
return length() == 0;
}
public int length() {
return endOffset - beginOffset;
}
public CharSequence subSequence(int start, int end) {
return getTokenSource().subSequence(beginOffset + start, beginOffset + end);
}
public char charAt(int offset) {
return getTokenSource().charAt(beginOffset + offset);
}
/**
* @deprecated Use toString() instead
*/
@Deprecated
public String getImage() {
return getSource();
}
@Override
public String toString() {
return getSource();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy