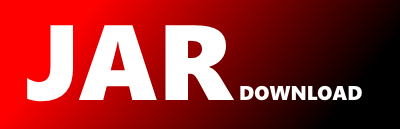
com.yahoo.vespa.config.search.summary.JuniperrcConfig Maven / Gradle / Ivy
// ------------ D O N O T E D I T ! ------------
// This file is generated from a config definition file.
package com.yahoo.vespa.config.search.summary;
import java.util.*;
import java.io.File;
import java.nio.file.Path;
import com.yahoo.config.*;
/**
* This class represents the root node of juniperrc
*
* Copyright Vespa.ai. Licensed under the terms of the Apache 2.0 license. See LICENSE in the project root.
*/
public final class JuniperrcConfig extends ConfigInstance {
public final static String CONFIG_DEF_MD5 = "a16512198c0f1d91f8390f1827386205";
public final static String CONFIG_DEF_NAME = "juniperrc";
public final static String CONFIG_DEF_NAMESPACE = "vespa.config.search.summary";
public final static String[] CONFIG_DEF_SCHEMA = {
"namespace=vespa.config.search.summary",
"length int default=256",
"max_matches int default=3",
"min_length int default=128",
"prefix bool default=true",
"surround_max int default=128",
"winsize int default=200",
"winsize_fallback_multiplier double default=10.0",
"max_match_candidates int default=1000",
"stem_min_length int default=5",
"stem_max_extend int default=3",
"override[].fieldname string",
"override[].length int default=256",
"override[].max_matches int default=3",
"override[].min_length int default=128",
"override[].prefix bool default=true",
"override[].surround_max int default=128",
"override[].winsize int default=200",
"override[].winsize_fallback_multiplier double default=10.0",
"override[].max_match_candidates int default=1000",
"override[].stem_min_length int default=5",
"override[].stem_max_extend int default=3"
};
public static String getDefMd5() { return CONFIG_DEF_MD5; }
public static String getDefName() { return CONFIG_DEF_NAME; }
public static String getDefNamespace() { return CONFIG_DEF_NAMESPACE; }
public interface Producer extends ConfigInstance.Producer {
void getConfig(Builder builder);
}
public static final class Builder implements ConfigInstance.Builder {
private Set __uninitialized = new HashSet();
private Integer length = null;
private Integer max_matches = null;
private Integer min_length = null;
private Boolean prefix = null;
private Integer surround_max = null;
private Integer winsize = null;
private Double winsize_fallback_multiplier = null;
private Integer max_match_candidates = null;
private Integer stem_min_length = null;
private Integer stem_max_extend = null;
public List override = new ArrayList<>();
public Builder() { }
public Builder(JuniperrcConfig config) {
length(config.length());
max_matches(config.max_matches());
min_length(config.min_length());
prefix(config.prefix());
surround_max(config.surround_max());
winsize(config.winsize());
winsize_fallback_multiplier(config.winsize_fallback_multiplier());
max_match_candidates(config.max_match_candidates());
stem_min_length(config.stem_min_length());
stem_max_extend(config.stem_max_extend());
for (Override o : config.override()) {
override(new Override.Builder(o));
}
}
private Builder override(Builder __superior) {
if (__superior.length != null)
length(__superior.length);
if (__superior.max_matches != null)
max_matches(__superior.max_matches);
if (__superior.min_length != null)
min_length(__superior.min_length);
if (__superior.prefix != null)
prefix(__superior.prefix);
if (__superior.surround_max != null)
surround_max(__superior.surround_max);
if (__superior.winsize != null)
winsize(__superior.winsize);
if (__superior.winsize_fallback_multiplier != null)
winsize_fallback_multiplier(__superior.winsize_fallback_multiplier);
if (__superior.max_match_candidates != null)
max_match_candidates(__superior.max_match_candidates);
if (__superior.stem_min_length != null)
stem_min_length(__superior.stem_min_length);
if (__superior.stem_max_extend != null)
stem_max_extend(__superior.stem_max_extend);
if (!__superior.override.isEmpty())
override.addAll(__superior.override);
return this;
}
public Builder length(int __value) {
length = __value;
return this;
}
private Builder length(String __value) {
return length(Integer.valueOf(__value));
}
public Builder max_matches(int __value) {
max_matches = __value;
return this;
}
private Builder max_matches(String __value) {
return max_matches(Integer.valueOf(__value));
}
public Builder min_length(int __value) {
min_length = __value;
return this;
}
private Builder min_length(String __value) {
return min_length(Integer.valueOf(__value));
}
public Builder prefix(boolean __value) {
prefix = __value;
return this;
}
private Builder prefix(String __value) {
return prefix(Boolean.valueOf(__value));
}
public Builder surround_max(int __value) {
surround_max = __value;
return this;
}
private Builder surround_max(String __value) {
return surround_max(Integer.valueOf(__value));
}
public Builder winsize(int __value) {
winsize = __value;
return this;
}
private Builder winsize(String __value) {
return winsize(Integer.valueOf(__value));
}
public Builder winsize_fallback_multiplier(double __value) {
winsize_fallback_multiplier = __value;
return this;
}
private Builder winsize_fallback_multiplier(String __value) {
return winsize_fallback_multiplier(Double.valueOf(__value));
}
public Builder max_match_candidates(int __value) {
max_match_candidates = __value;
return this;
}
private Builder max_match_candidates(String __value) {
return max_match_candidates(Integer.valueOf(__value));
}
public Builder stem_min_length(int __value) {
stem_min_length = __value;
return this;
}
private Builder stem_min_length(String __value) {
return stem_min_length(Integer.valueOf(__value));
}
public Builder stem_max_extend(int __value) {
stem_max_extend = __value;
return this;
}
private Builder stem_max_extend(String __value) {
return stem_max_extend(Integer.valueOf(__value));
}
/**
* Add the given builder to this builder's list of Override builders
* @param __builder a builder
* @return this builder
*/
public Builder override(Override.Builder __builder) {
override.add(__builder);
return this;
}
/**
* Make a new builder and run the supplied function on it before adding it to the list
* @param __func lambda that modifies the given builder
* @return this builder
*/
public Builder override(java.util.function.Consumer __func) {
Override.Builder __inner = new Override.Builder();
__func.accept(__inner);
override.add(__inner);
return this;
}
/**
* Set the given list as this builder's list of Override builders
* @param __builders a list of builders
* @return this builder
*/
public Builder override(List __builders) {
override = __builders;
return this;
}
private boolean _applyOnRestart = false;
@java.lang.Override
public final boolean dispatchGetConfig(ConfigInstance.Producer producer) {
if (producer instanceof Producer) {
((Producer)producer).getConfig(this);
return true;
}
return false;
}
@java.lang.Override
public final String getDefMd5() { return CONFIG_DEF_MD5; }
@java.lang.Override
public final String getDefName() { return CONFIG_DEF_NAME; }
@java.lang.Override
public final String getDefNamespace() { return CONFIG_DEF_NAMESPACE; }
@java.lang.Override
public final boolean getApplyOnRestart() { return _applyOnRestart; }
@java.lang.Override
public final void setApplyOnRestart(boolean applyOnRestart) { _applyOnRestart = applyOnRestart; }
public JuniperrcConfig build() {
return new JuniperrcConfig(this);
}
}
// Set the length (in #characters) of the dynamically generated
// summaries. This is a hint to the module that generates the
// dynamic summary - the actual size will depend on the available text
// and the query. You may find that you need to set this length
// substantially longer than your actual desired average length -
// setting this about twice as long is often appropriate.
private final IntegerNode length;
// The number of (possibly partial) set of keywords
// matching the query, to attempt to include in the summary. The larger this
// value compared is set relative to the length parameter, the more
// dense the keywords may appear in the summary.
private final IntegerNode max_matches;
// Minimal desired length of the generated summary in
// bytes. This is the shortest summary length for which the number of
// matches will be respected. Eg. if
// a summary appear to become shorter than min_length bytes with
// max_matches matches, then additional matches will be used if available.
private final IntegerNode min_length;
// Make sure the prefix (length controlled by 'juniper.dynsum.length')
// of all fields with summary: dynamic are returned in the dynamic
// summary if a query does not hit in those fields
private final BooleanNode prefix;
// The maximal number of bytes of context to prepend and append to
// each of the selected query keyword hits. This parameter defines the
// max size a summary would become if there are few keyword hits
// (max_matches set low or document contained few matches of the keywords).
private final IntegerNode surround_max;
// The size of the sliding window used to determine if
// multiple query terms occur together. The larger the value, the more
// likely the system will find (and present in dynamic summary) complete
// matches containing all the search terms. The downside is a potential
// performance overhead of keeping candidates for matches longer during
// matching, and consequently updating more candidates that eventually
// gets thrown.
private final IntegerNode winsize;
// This value multiplied with the winsize gives the size of a fallback
// window used to break out when searching for phrase term matches.
private final DoubleNode winsize_fallback_multiplier;
// This value specifies the maximum number of match candidates that are
// managed for a non-leaf query node when matching the query against the
// input text.
private final IntegerNode max_match_candidates;
// The minimal number of bytes in a query keyword for
// it to be subject to the simple Juniper stemming algorithm. Keywords
// that are shorter than or equal to this limit will only yield exact
// matches in the dynamic summaries.
private final IntegerNode stem_min_length;
// The maximal number of bytes that a word in the document
// can be longer than the keyword itself to yield a match. Eg. for
// the default values, if the keyword is 7 bytes long, it will match any
// word with length less than or equal to 10 for which the keyword is a prefix.
private final IntegerNode stem_max_extend;
private final InnerNodeVector override;
public JuniperrcConfig(Builder builder) {
this(builder, true);
}
private JuniperrcConfig(Builder builder, boolean throwIfUninitialized) {
if (throwIfUninitialized && ! builder.__uninitialized.isEmpty())
throw new IllegalArgumentException("The following builder parameters for " +
"juniperrc must be initialized: " + builder.__uninitialized);
length = (builder.length == null) ?
new IntegerNode(256) : new IntegerNode(builder.length);
max_matches = (builder.max_matches == null) ?
new IntegerNode(3) : new IntegerNode(builder.max_matches);
min_length = (builder.min_length == null) ?
new IntegerNode(128) : new IntegerNode(builder.min_length);
prefix = (builder.prefix == null) ?
new BooleanNode(true) : new BooleanNode(builder.prefix);
surround_max = (builder.surround_max == null) ?
new IntegerNode(128) : new IntegerNode(builder.surround_max);
winsize = (builder.winsize == null) ?
new IntegerNode(200) : new IntegerNode(builder.winsize);
winsize_fallback_multiplier = (builder.winsize_fallback_multiplier == null) ?
new DoubleNode(10.0D) : new DoubleNode(builder.winsize_fallback_multiplier);
max_match_candidates = (builder.max_match_candidates == null) ?
new IntegerNode(1000) : new IntegerNode(builder.max_match_candidates);
stem_min_length = (builder.stem_min_length == null) ?
new IntegerNode(5) : new IntegerNode(builder.stem_min_length);
stem_max_extend = (builder.stem_max_extend == null) ?
new IntegerNode(3) : new IntegerNode(builder.stem_max_extend);
override = Override.createVector(builder.override);
}
/**
* @return juniperrc.length
*/
public int length() {
return length.value();
}
/**
* @return juniperrc.max_matches
*/
public int max_matches() {
return max_matches.value();
}
/**
* @return juniperrc.min_length
*/
public int min_length() {
return min_length.value();
}
/**
* @return juniperrc.prefix
*/
public boolean prefix() {
return prefix.value();
}
/**
* @return juniperrc.surround_max
*/
public int surround_max() {
return surround_max.value();
}
/**
* @return juniperrc.winsize
*/
public int winsize() {
return winsize.value();
}
/**
* @return juniperrc.winsize_fallback_multiplier
*/
public double winsize_fallback_multiplier() {
return winsize_fallback_multiplier.value();
}
/**
* @return juniperrc.max_match_candidates
*/
public int max_match_candidates() {
return max_match_candidates.value();
}
/**
* @return juniperrc.stem_min_length
*/
public int stem_min_length() {
return stem_min_length.value();
}
/**
* @return juniperrc.stem_max_extend
*/
public int stem_max_extend() {
return stem_max_extend.value();
}
/**
* @return juniperrc.override[]
*/
public List override() {
return override;
}
/**
* @param i the index of the value to return
* @return juniperrc.override[]
*/
public Override override(int i) {
return override.get(i);
}
private ChangesRequiringRestart getChangesRequiringRestart(JuniperrcConfig newConfig) {
ChangesRequiringRestart changes = new ChangesRequiringRestart("juniperrc");
return changes;
}
private static boolean containsFieldsFlaggedWithRestart() {
return false;
}
/**
* This class represents juniperrc.override[]
*/
public final static class Override extends InnerNode {
public static final class Builder implements ConfigBuilder {
private Set __uninitialized = new HashSet(List.of(
"fieldname"
));
private String fieldname = null;
private Integer length = null;
private Integer max_matches = null;
private Integer min_length = null;
private Boolean prefix = null;
private Integer surround_max = null;
private Integer winsize = null;
private Double winsize_fallback_multiplier = null;
private Integer max_match_candidates = null;
private Integer stem_min_length = null;
private Integer stem_max_extend = null;
public Builder() { }
public Builder(Override config) {
fieldname(config.fieldname());
length(config.length());
max_matches(config.max_matches());
min_length(config.min_length());
prefix(config.prefix());
surround_max(config.surround_max());
winsize(config.winsize());
winsize_fallback_multiplier(config.winsize_fallback_multiplier());
max_match_candidates(config.max_match_candidates());
stem_min_length(config.stem_min_length());
stem_max_extend(config.stem_max_extend());
}
private Builder override(Builder __superior) {
if (__superior.fieldname != null)
fieldname(__superior.fieldname);
if (__superior.length != null)
length(__superior.length);
if (__superior.max_matches != null)
max_matches(__superior.max_matches);
if (__superior.min_length != null)
min_length(__superior.min_length);
if (__superior.prefix != null)
prefix(__superior.prefix);
if (__superior.surround_max != null)
surround_max(__superior.surround_max);
if (__superior.winsize != null)
winsize(__superior.winsize);
if (__superior.winsize_fallback_multiplier != null)
winsize_fallback_multiplier(__superior.winsize_fallback_multiplier);
if (__superior.max_match_candidates != null)
max_match_candidates(__superior.max_match_candidates);
if (__superior.stem_min_length != null)
stem_min_length(__superior.stem_min_length);
if (__superior.stem_max_extend != null)
stem_max_extend(__superior.stem_max_extend);
return this;
}
public Builder fieldname(String __value) {
if (__value == null) throw new IllegalArgumentException("Null value is not allowed.");
fieldname = __value;
__uninitialized.remove("fieldname");
return this;
}
public Builder length(int __value) {
length = __value;
return this;
}
private Builder length(String __value) {
return length(Integer.valueOf(__value));
}
public Builder max_matches(int __value) {
max_matches = __value;
return this;
}
private Builder max_matches(String __value) {
return max_matches(Integer.valueOf(__value));
}
public Builder min_length(int __value) {
min_length = __value;
return this;
}
private Builder min_length(String __value) {
return min_length(Integer.valueOf(__value));
}
public Builder prefix(boolean __value) {
prefix = __value;
return this;
}
private Builder prefix(String __value) {
return prefix(Boolean.valueOf(__value));
}
public Builder surround_max(int __value) {
surround_max = __value;
return this;
}
private Builder surround_max(String __value) {
return surround_max(Integer.valueOf(__value));
}
public Builder winsize(int __value) {
winsize = __value;
return this;
}
private Builder winsize(String __value) {
return winsize(Integer.valueOf(__value));
}
public Builder winsize_fallback_multiplier(double __value) {
winsize_fallback_multiplier = __value;
return this;
}
private Builder winsize_fallback_multiplier(String __value) {
return winsize_fallback_multiplier(Double.valueOf(__value));
}
public Builder max_match_candidates(int __value) {
max_match_candidates = __value;
return this;
}
private Builder max_match_candidates(String __value) {
return max_match_candidates(Integer.valueOf(__value));
}
public Builder stem_min_length(int __value) {
stem_min_length = __value;
return this;
}
private Builder stem_min_length(String __value) {
return stem_min_length(Integer.valueOf(__value));
}
public Builder stem_max_extend(int __value) {
stem_max_extend = __value;
return this;
}
private Builder stem_max_extend(String __value) {
return stem_max_extend(Integer.valueOf(__value));
}
public Override build() {
return new Override(this);
}
}
// The parameters above may also be overriden on a per-field basis
// using the following array.
private final StringNode fieldname;
private final IntegerNode length;
private final IntegerNode max_matches;
private final IntegerNode min_length;
private final BooleanNode prefix;
private final IntegerNode surround_max;
private final IntegerNode winsize;
private final DoubleNode winsize_fallback_multiplier;
private final IntegerNode max_match_candidates;
private final IntegerNode stem_min_length;
private final IntegerNode stem_max_extend;
public Override(Builder builder) {
this(builder, true);
}
private Override(Builder builder, boolean throwIfUninitialized) {
if (throwIfUninitialized && ! builder.__uninitialized.isEmpty())
throw new IllegalArgumentException("The following builder parameters for " +
"juniperrc.override[] must be initialized: " + builder.__uninitialized);
fieldname = (builder.fieldname == null) ?
new StringNode() : new StringNode(builder.fieldname);
length = (builder.length == null) ?
new IntegerNode(256) : new IntegerNode(builder.length);
max_matches = (builder.max_matches == null) ?
new IntegerNode(3) : new IntegerNode(builder.max_matches);
min_length = (builder.min_length == null) ?
new IntegerNode(128) : new IntegerNode(builder.min_length);
prefix = (builder.prefix == null) ?
new BooleanNode(true) : new BooleanNode(builder.prefix);
surround_max = (builder.surround_max == null) ?
new IntegerNode(128) : new IntegerNode(builder.surround_max);
winsize = (builder.winsize == null) ?
new IntegerNode(200) : new IntegerNode(builder.winsize);
winsize_fallback_multiplier = (builder.winsize_fallback_multiplier == null) ?
new DoubleNode(10.0D) : new DoubleNode(builder.winsize_fallback_multiplier);
max_match_candidates = (builder.max_match_candidates == null) ?
new IntegerNode(1000) : new IntegerNode(builder.max_match_candidates);
stem_min_length = (builder.stem_min_length == null) ?
new IntegerNode(5) : new IntegerNode(builder.stem_min_length);
stem_max_extend = (builder.stem_max_extend == null) ?
new IntegerNode(3) : new IntegerNode(builder.stem_max_extend);
}
/**
* @return juniperrc.override[].fieldname
*/
public String fieldname() {
return fieldname.value();
}
/**
* @return juniperrc.override[].length
*/
public int length() {
return length.value();
}
/**
* @return juniperrc.override[].max_matches
*/
public int max_matches() {
return max_matches.value();
}
/**
* @return juniperrc.override[].min_length
*/
public int min_length() {
return min_length.value();
}
/**
* @return juniperrc.override[].prefix
*/
public boolean prefix() {
return prefix.value();
}
/**
* @return juniperrc.override[].surround_max
*/
public int surround_max() {
return surround_max.value();
}
/**
* @return juniperrc.override[].winsize
*/
public int winsize() {
return winsize.value();
}
/**
* @return juniperrc.override[].winsize_fallback_multiplier
*/
public double winsize_fallback_multiplier() {
return winsize_fallback_multiplier.value();
}
/**
* @return juniperrc.override[].max_match_candidates
*/
public int max_match_candidates() {
return max_match_candidates.value();
}
/**
* @return juniperrc.override[].stem_min_length
*/
public int stem_min_length() {
return stem_min_length.value();
}
/**
* @return juniperrc.override[].stem_max_extend
*/
public int stem_max_extend() {
return stem_max_extend.value();
}
private ChangesRequiringRestart getChangesRequiringRestart(Override newConfig) {
ChangesRequiringRestart changes = new ChangesRequiringRestart("override");
return changes;
}
private static InnerNodeVector createVector(List builders) {
List elems = new ArrayList<>();
for (Builder b : builders) {
elems.add(new Override(b));
}
return new InnerNodeVector(elems);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy