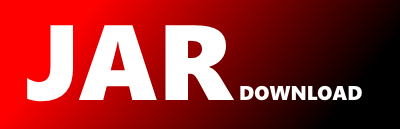
yandex.cloud.sdk.grpc.interceptors.DeadlineClientInterceptor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdk Show documentation
Show all versions of sdk Show documentation
Java SDK for Yandex Cloud
package yandex.cloud.sdk.grpc.interceptors;
import io.grpc.CallOptions;
import io.grpc.Channel;
import io.grpc.ClientCall;
import io.grpc.ClientInterceptor;
import io.grpc.MethodDescriptor;
import java.time.Duration;
import java.util.concurrent.TimeUnit;
/**
* An interceptor that enforces given deadline for a call.
*/
public class DeadlineClientInterceptor implements ClientInterceptor {
/**
* Timeout after which a call fails if it is still not completed
*/
private final Duration timeout;
/**
* Constructs a DeadlineClientInterceptor
object with given timeout
* @param timeout timeout after which a call fails if it is still not completed
*/
private DeadlineClientInterceptor(Duration timeout) {
this.timeout = timeout;
}
@Override
public ClientCall interceptCall(
MethodDescriptor method, CallOptions callOptions, Channel next) {
CallOptions callOptionsWithDeadline = timeout != null ?
callOptions.withDeadlineAfter(getTimeoutInMillis(), TimeUnit.MILLISECONDS) : callOptions;
return next.newCall(method, callOptionsWithDeadline);
}
/**
* Create a DeadlineClientInterceptor
with given timeout
* @param timeout timeout after which a call fails if it is still not completed
* @return DeadlineClientInterceptor
object
*/
public static DeadlineClientInterceptor fromDuration(Duration timeout) {
return new DeadlineClientInterceptor(timeout);
}
/**
* Converts Duration
object to milliseconds value
* @return milliseconds value of {@link DeadlineClientInterceptor#timeout}
*/
private long getTimeoutInMillis() {
return timeout != null ? timeout.toMillis() : 0L;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy