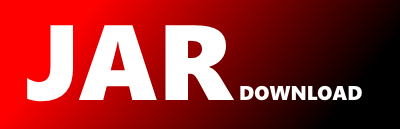
divkit.dsl.ActionVideo.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kotlin-json-builder Show documentation
Show all versions of kotlin-json-builder Show documentation
DivKit is an open source Server-Driven UI (SDUI) framework. SDUI is a an emerging technique that leverage the server to build the user interfaces of their mobile app.
@file:Suppress(
"unused",
"UNUSED_PARAMETER",
)
package divkit.dsl
import com.fasterxml.jackson.annotation.JsonAnyGetter
import com.fasterxml.jackson.annotation.JsonIgnore
import com.fasterxml.jackson.annotation.JsonValue
import divkit.dsl.annotation.*
import divkit.dsl.core.*
import divkit.dsl.scope.*
import kotlin.Any
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
* Controls given video.
*
* Can be created using the method [actionVideo].
*
* Required parameters: `type, id, action`.
*/
@Generated
class ActionVideo internal constructor(
@JsonIgnore
val properties: Properties,
) : ActionTyped {
@JsonAnyGetter
internal fun getJsonProperties(): Map = properties.mergeWith(
mapOf("type" to "video")
)
operator fun plus(additive: Properties): ActionVideo = ActionVideo(
Properties(
action = additive.action ?: properties.action,
id = additive.id ?: properties.id,
)
)
class Properties internal constructor(
/**
* Defines video action:`start` - play if it is ready or plans to play when video becomes ready; `pause` - pauses video playback.
*/
val action: Property?,
/**
* Video identifier.
*/
val id: Property?,
) {
internal fun mergeWith(properties: Map): Map {
val result = mutableMapOf()
result.putAll(properties)
result.tryPutProperty("action", action)
result.tryPutProperty("id", id)
return result
}
}
/**
* Defines video action:`start` - play if it is ready or plans to play when video becomes ready; `pause` - pauses video playback.
*
* Possible values: [start], [pause].
*/
@Generated
sealed interface Action
}
/**
* @param action Defines video action:`start` - play if it is ready or plans to play when video becomes ready; `pause` - pauses video playback.
* @param id Video identifier.
*/
@Generated
fun DivScope.actionVideo(
`use named arguments`: Guard = Guard.instance,
action: ActionVideo.Action? = null,
id: String? = null,
): ActionVideo = ActionVideo(
ActionVideo.Properties(
action = valueOrNull(action),
id = valueOrNull(id),
)
)
/**
* @param action Defines video action:`start` - play if it is ready or plans to play when video becomes ready; `pause` - pauses video playback.
* @param id Video identifier.
*/
@Generated
fun DivScope.actionVideoProps(
`use named arguments`: Guard = Guard.instance,
action: ActionVideo.Action? = null,
id: String? = null,
) = ActionVideo.Properties(
action = valueOrNull(action),
id = valueOrNull(id),
)
/**
* @param action Defines video action:`start` - play if it is ready or plans to play when video becomes ready; `pause` - pauses video playback.
* @param id Video identifier.
*/
@Generated
fun TemplateScope.actionVideoRefs(
`use named arguments`: Guard = Guard.instance,
action: ReferenceProperty? = null,
id: ReferenceProperty? = null,
) = ActionVideo.Properties(
action = action,
id = id,
)
/**
* @param action Defines video action:`start` - play if it is ready or plans to play when video becomes ready; `pause` - pauses video playback.
* @param id Video identifier.
*/
@Generated
fun ActionVideo.override(
`use named arguments`: Guard = Guard.instance,
action: ActionVideo.Action? = null,
id: String? = null,
): ActionVideo = ActionVideo(
ActionVideo.Properties(
action = valueOrNull(action) ?: properties.action,
id = valueOrNull(id) ?: properties.id,
)
)
/**
* @param action Defines video action:`start` - play if it is ready or plans to play when video becomes ready; `pause` - pauses video playback.
* @param id Video identifier.
*/
@Generated
fun ActionVideo.defer(
`use named arguments`: Guard = Guard.instance,
action: ReferenceProperty? = null,
id: ReferenceProperty? = null,
): ActionVideo = ActionVideo(
ActionVideo.Properties(
action = action ?: properties.action,
id = id ?: properties.id,
)
)
/**
* @param action Defines video action:`start` - play if it is ready or plans to play when video becomes ready; `pause` - pauses video playback.
* @param id Video identifier.
*/
@Generated
fun ActionVideo.evaluate(
`use named arguments`: Guard = Guard.instance,
action: ExpressionProperty? = null,
id: ExpressionProperty? = null,
): ActionVideo = ActionVideo(
ActionVideo.Properties(
action = action ?: properties.action,
id = id ?: properties.id,
)
)
@Generated
fun ActionVideo.asList() = listOf(this)
@Generated
fun ActionVideo.Action.asList() = listOf(this)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy