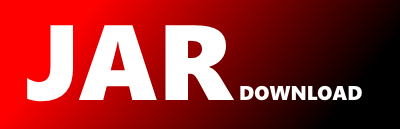
com.yishuifengxiao.common.tool.bean.BeanUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of common-tool Show documentation
Show all versions of common-tool Show documentation
本工具包主要集成了目前在项目开发过程中个人经常会使用到的一些工具类,对工具类进行了一下简单的封装
/**
*
*/
package com.yishuifengxiao.common.tool.bean;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.lang.reflect.Field;
import org.apache.commons.text.StringEscapeUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.BeanUtils;
/**
* 对象转换类
*
* @author yishui
* @date 2018年12月6日
* @Version 0.0.1
*/
public final class BeanUtil {
private final static Logger log = LoggerFactory.getLogger(BeanUtil.class);
/**
* 将源对象里属性值复制给目标对象
*
* @param source 源对象
* @param target 目标对象
* @param converter 目标对象
* @return
*/
public static T copy(S source, T target) {
if (source == null || target == null) {
return null;
}
BeanUtils.copyProperties(source, target);
return target;
}
/**
* 去除对象里非空属性之外的属性和制表符
*
* @param source 原始对象
* @return 过滤后的对象
* @throws IllegalArgumentException
* @throws IllegalAccessException
* @throws SecurityException
*/
public static T setNullValue(T source)
throws IllegalArgumentException, IllegalAccessException, SecurityException {
Field[] fields = source.getClass().getDeclaredFields();
for (Field field : fields) {
if ("class java.lang.String".equals(field.getGenericType().toString())) {
field.setAccessible(true);
Object obj = field.get(source);
if (obj != null && obj.equals("")) {
field.set(source, null);
} else if (obj != null) {
field.set(source,
StringEscapeUtils.escapeJava(obj.toString()).replace("\\", "\\" + "\\").replace("(", "\\(")
.replace(")", "\\)").replace("%", "\\%").replace("*", "\\*").replace("[", "\\[")
.replace("]", "\\]").replace("|", "\\|").replace(".", "\\.").replace("$", "\\$")
.replace("+", "\\+").trim());
}
}
}
return source;
}
/**
* 将Java对象序列化为二进制数据
*
* @param obj 需要序列化的的对象
* @return 二进制数据
*/
public static byte[] objectToByte(Object obj) {
byte[] bytes = null;
try {
// object to bytearray
ByteArrayOutputStream bo = new ByteArrayOutputStream();
ObjectOutputStream oo = new ObjectOutputStream(bo);
oo.writeObject(obj);
bytes = bo.toByteArray();
bo.close();
oo.close();
} catch (Exception e) {
log.info(" 对象序列化出现问题,出现问题的原因为 {}", e.getMessage());
}
return bytes;
}
/**
* 将序列化化后的二进制数据反序列化为对象
*
* @param bytes 序列化化后的二进制数据
* @return 对象
*/
public static Object byteToObject(byte[] bytes) {
Object obj = null;
try {
// bytearray to object
ByteArrayInputStream bi = new ByteArrayInputStream(bytes);
ObjectInputStream oi = new ObjectInputStream(bi);
obj = oi.readObject();
bi.close();
oi.close();
} catch (Exception e) {
log.info("对象反序列化出现问题,出现问题的原因为 {}", e.getMessage());
}
return obj;
}
/**
* 将序列化化后的二进制数据反序列化为对象
*
* @param t 希望序列化成的数据类型,不能为空
*
* @param bytes 序列化化后的二进制数据
* @return 对象
*/
@SuppressWarnings("unchecked")
public static T byteToObject(T t, byte[] bytes) {
try {
// bytearray to object
ByteArrayInputStream bi = new ByteArrayInputStream(bytes);
ObjectInputStream oi = new ObjectInputStream(bi);
t = (T) oi.readObject();
bi.close();
oi.close();
} catch (Exception e) {
log.info(" 对象反序列化出现问题,出现问题的原因为 {}", e.getMessage());
}
return t;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy