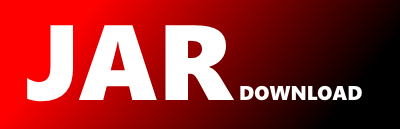
com.yiwowang.intellij.finding.ResultForm Maven / Gradle / Ivy
The newest version!
package com.yiwowang.intellij.finding;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.yiwowang.intellij.finding.util.UIUtils;
import com.yiwowang.intellij.finding.base.bean.FindUsagesParams;
import com.yiwowang.intellij.finding.base.bean.RefResult;
import com.yiwowang.intellij.finding.base.util.Utils;
import org.apache.commons.lang.StringUtils;
import javax.swing.*;
import javax.swing.tree.DefaultMutableTreeNode;
import javax.swing.tree.DefaultTreeModel;
import java.awt.*;
import java.util.*;
import java.util.List;
public class ResultForm {
public JPanel panel1;
public JTree tree1;
private JTabbedPane tabbedPane1;
private JTextArea exportTxt;
private long startTime;
private FindUsagesParams params;
private void init(ProjectContext context, FindUsagesParams params) {
showTip("Searching...");
this.params = params;
startTime = System.currentTimeMillis();
Utils.executeThread(new Utils.AsyncTask>() {
@Override
public java.util.List doInBackground() {
return context.projectFinding.findUsages(params);
}
@Override
public void doUi(List data) {
init(data);
}
});
}
private void init(List data) {
System.out.println("search cost time:" + (System.currentTimeMillis() - startTime));
if (data == null) {
showTip("result size:0");
return;
}
// 按照组件归类,按照类归类
Map> map = Utils.resultToMap(data);
DefaultMutableTreeNode top =
new DefaultMutableTreeNode("");
for (Map.Entry> entry : map.entrySet()) {
String componentName = entry.getKey();
List classList = entry.getValue();
DefaultMutableTreeNode componentNode = new DefaultMutableTreeNode(componentName);
top.add(componentNode);
for (String className : classList) {
DefaultMutableTreeNode classNode = new DefaultMutableTreeNode(className);
componentNode.add(classNode);
}
}
top.setUserObject("result size:" + top.getChildCount());
tree1.setModel(new DefaultTreeModel(top, false));
UIUtils.expandAll(tree1, true);
showExport(map);
}
private void showExport(Map> map) {
Object data = Utils.mergeResult(params, map);
Gson gson = new GsonBuilder().setPrettyPrinting().create();
exportTxt.setText(gson.toJson(data));
}
private String getSpace(int tabNum) {
return StringUtils.repeat(" ", tabNum);
}
private void showTip(String tip) {
DefaultMutableTreeNode top =
new DefaultMutableTreeNode(tip);
tree1.setModel(new DefaultTreeModel(top, false));
}
public static JDialog showDialog(ProjectContext context, FindUsagesParams params) {
JDialog jDialog = new JDialog();
jDialog.setTitle("Find Usages Result");
Dimension dim = Toolkit.getDefaultToolkit().getScreenSize();
double screenWidth = dim.getWidth();
double screenHeight = dim.getHeight();
double size = Math.min(screenWidth, screenHeight);
int width = (int) (size * 0.5);
int height = (int) (size * 0.5);
jDialog.setSize(width, height);
int x = (int) ((screenWidth - width) / 2);
int y = (int) ((screenHeight - height) / 2);
jDialog.setLocation(x, y);
ResultForm form = new ResultForm();
form.init(context, params);
jDialog.setAlwaysOnTop(true);
jDialog.setContentPane(form.panel1);
jDialog.setDefaultCloseOperation(JDialog.DISPOSE_ON_CLOSE);
jDialog.setVisible(true);
return jDialog;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy