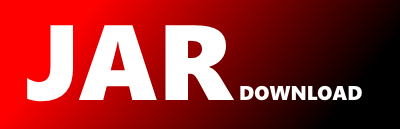
com.yiwowang.intellij.finding.SearchForm Maven / Gradle / Ivy
The newest version!
package com.yiwowang.intellij.finding;
import com.intellij.openapi.project.Project;
import com.yiwowang.intellij.finding.base.bean.FindUsagesParams;
import com.yiwowang.intellij.finding.base.util.ProjectFinding;
import com.yiwowang.intellij.finding.util.ProjectUtils;
import com.yiwowang.intellij.finding.util.UIUtils;
import org.apache.commons.lang.StringUtils;
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class SearchForm {
public JPanel panel;
public JTabbedPane tabPane;
public JTree classTree;
public JList memberList;
public JLabel memberComponentLabel;
public JButton memberSearchBtn;
public JTextField componentInput;
public JSplitPane jSplitPane;
public JCheckBox memberIgnoreCaseChk;
public ButtonGroup buttonGroup;
public JRadioButton classRbtn;
public JRadioButton methodRbtn;
public JRadioButton fieldRbtn;
public JRadioButton constantRbtn;
public JRadioButton packageRbtn;
public JLabel classLabel;
public JTextField classInput;
public JTextField methodInput;
public JLabel methodLabel;
public JTextField fieldInput;
public JLabel fieldLabel;
public JTextField constantInput;
public JLabel constantLabel;
public JCheckBox androidClassChk;
public JTextField packageInput;
public JLabel packageLabel;
public JTree componentTree;
public JCheckBox dependentChk;
public JRadioButton depCodeRbtn;
public JRadioButton depAllRbtn;
public JRadioButton depFirstRbtn;
public JCheckBox depExpandAllChk;
private SearchComponent mSearchComponent;
private SearchMember mSearchMember;
public int width;
public int height;
private JDialog jDialog;
private Project project;
public ProjectContext context;
private FindUsagesParams params;
public void show(Project project, FindUsagesParams params) {
this.params = params;
if (isShowing()) {
jDialog.setVisible(true);
mSearchMember.formFindUsagesParams(params);
return;
}
this.project = project;
this.context = new ProjectContext();
Dimension dim = Toolkit.getDefaultToolkit().getScreenSize();
double screenWidth = dim.getWidth();
double screenHeight = dim.getHeight();
double size = Math.min(screenWidth, screenHeight);
this.width = (int) (size * 0.8);
this.height = (int) (size * 0.8);
TipDialog dialog = new TipDialog();
String text = "Initializing. Please wait...";
dialog.setText(text);
dialog.show();
context.projectFinding.initAsync(ProjectUtils.getProjectDir(project), new ProjectFinding.InitProgressCallback() {
@Override
public void onProgress(int value, int max) {
UIUtils.runOnUi(new Runnable() {
@Override
public void run() {
dialog.setText(text + " " + (int) (value * 1.0 / max * 100) + "%");
}
});
}
@Override
public void onFinish(String error) {
UIUtils.runOnUi(new Runnable() {
@Override
public void run() {
if (!StringUtils.isEmpty(error)) {
dialog.setText(error);
return;
}
dialog.close();
initRbtn();
initSearchComponentUI();
setClassMethodMode();
mSearchComponent = new SearchComponent(SearchForm.this);
mSearchMember = new SearchMember(project, SearchForm.this);
mSearchMember.formFindUsagesParams(params);
jDialog = new JDialog();
jDialog.setTitle("Finding");
jDialog.setSize(width, height);
int x = (int) ((screenWidth - width) / 2);
int y = (int) ((screenHeight - height) / 2);
jDialog.setLocation(x, y);
jDialog.add(panel);
jDialog.setDefaultCloseOperation(JDialog.DISPOSE_ON_CLOSE);
jDialog.setVisible(true);
}
});
}
});
}
private void initRbtn() {
buttonGroup = new ButtonGroup();
buttonGroup.add(methodRbtn);
buttonGroup.add(fieldRbtn);
buttonGroup.add(classRbtn);
buttonGroup.add(constantRbtn);
buttonGroup.add(packageRbtn);
classRbtn.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
setClassMode();
}
});
methodRbtn.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
setClassMethodMode();
}
});
fieldRbtn.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
setClassFieldMode();
}
});
constantRbtn.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
setClassConstantMode();
}
});
packageRbtn.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
setPackageMode();
}
});
}
void initSearchComponentUI() {
ButtonGroup buttonGroup = new ButtonGroup();
buttonGroup.add(depCodeRbtn);
buttonGroup.add(depAllRbtn);
buttonGroup.add(depFirstRbtn);
ActionListener actionListener = new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
mSearchComponent.refreshData();
}
};
depCodeRbtn.addActionListener(actionListener);
depAllRbtn.addActionListener(actionListener);
depFirstRbtn.addActionListener(actionListener);
depAllRbtn.setSelected(true);
depExpandAllChk.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
UIUtils.expandAll(componentTree, depExpandAllChk.isSelected());
}
});
}
public void openSplitPanel() {
jSplitPane.setDividerLocation((int) (width * 0.7));
}
public void closeSplitPanel() {
jSplitPane.setDividerLocation(width);
}
public void setClassMode() {
classLabel.setVisible(true);
classInput.setVisible(true);
methodLabel.setVisible(false);
methodInput.setVisible(false);
fieldLabel.setVisible(false);
fieldInput.setVisible(false);
constantLabel.setVisible(false);
constantInput.setVisible(false);
packageLabel.setVisible(false);
packageInput.setVisible(false);
closeSplitPanel();
}
public void setClassMethodMode() {
classLabel.setVisible(true);
classInput.setVisible(true);
methodLabel.setVisible(true);
methodInput.setVisible(true);
fieldLabel.setVisible(false);
fieldInput.setVisible(false);
constantLabel.setVisible(false);
constantInput.setVisible(false);
packageLabel.setVisible(false);
packageInput.setVisible(false);
openSplitPanel();
}
public void setClassFieldMode() {
classLabel.setVisible(true);
classInput.setVisible(true);
methodLabel.setVisible(false);
methodInput.setVisible(false);
fieldLabel.setVisible(true);
fieldInput.setVisible(true);
constantLabel.setVisible(false);
constantInput.setVisible(false);
packageLabel.setVisible(false);
packageInput.setVisible(false);
openSplitPanel();
}
public void setClassConstantMode() {
classLabel.setVisible(true);
classInput.setVisible(true);
methodLabel.setVisible(false);
methodInput.setVisible(false);
fieldLabel.setVisible(false);
fieldInput.setVisible(false);
constantLabel.setVisible(true);
constantInput.setVisible(true);
packageLabel.setVisible(false);
packageInput.setVisible(false);
closeSplitPanel();
}
public void setPackageMode() {
classLabel.setVisible(false);
classInput.setVisible(false);
methodLabel.setVisible(false);
methodInput.setVisible(false);
fieldLabel.setVisible(false);
fieldInput.setVisible(false);
constantLabel.setVisible(false);
constantInput.setVisible(false);
packageLabel.setVisible(true);
packageInput.setVisible(true);
closeSplitPanel();
}
public boolean isShowing() {
return jDialog != null && jDialog.isShowing();
}
public void close() {
if (jDialog != null) {
jDialog.dispose();
}
}
public Project getProject() {
return project;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy