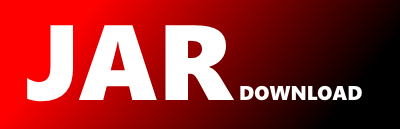
com.yixan.tools.common.files.ImageManager Maven / Gradle / Ivy
package com.yixan.tools.common.files;
import java.io.IOException;
import java.util.regex.Pattern;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.yixan.base.core.model.file.ImageInfo;
import com.yixan.tools.common.util.ImageUtil;
import com.yixan.tools.common.util.StringUtil;
import com.yixan.tools.common.util.VerifyUtil;
/**
* 图片上传工具类
*
* @author zhaohuihua
* @version V1.0 2015年11月25日
*/
public class ImageManager {
/** 日志对象 **/
private static final Logger log = LoggerFactory.getLogger(ImageManager.class);
private static class Size {
private int width;
private int height;
public Size(int size) {
this.width = size;
this.height = size;
}
public Size(int width, int height) {
this.width = width;
this.height = height;
}
}
private static Size SMALL = parseConfig("image.size.small");
private static Size LARGE = parseConfig("image.size.large");
/**
* 上传图片
*
* @param filePath
* @param is
* @return
*/
public static ImageInfo upload(String filePath, byte[] bytes) {
ImageInfo info = new ImageInfo();
String original = FileManager.upload(filePath, bytes);
info.setOriginal(original);
String small = thumbnail(filePath, bytes, SMALL);
String large = thumbnail(filePath, bytes, LARGE);
info.setSmall(small);
info.setLarge(large);
return info;
}
private static Size parseConfig(String key) {
String value = FileManager.getConfigValue(key);
if (VerifyUtil.isBlank(value)) {
return null;
}
if (StringUtil.isDigit(value)) {
return new Size(Integer.valueOf(value));
}
if (value.contains("*")) {
Pattern ptn = Pattern.compile("\\*");
String[] values = ptn.split(value);
if (values.length >= 2) {
String w = values[0].trim();
String h = values[1].trim();
if (StringUtil.isDigit(w) && StringUtil.isDigit(h)) {
int width = Integer.valueOf(w);
int height = Integer.valueOf(h);
return new Size(width, height);
}
}
}
String fmt = "Config '{}' format error, '{}' can't convert to size.";
log.warn(fmt, key, value);
return null;
}
private static String thumbnail(String filePath, byte[] bytes, Size size) {
if (size == null) {
return null;
}
byte[] newer;
try {
newer = ImageUtil.thumbnail(bytes, size.width, size.height);
} catch (IOException e) {
log.error("Failed to image thumbnail. " + filePath, e);
return null;
}
return FileManager.upload(filePath, newer);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy