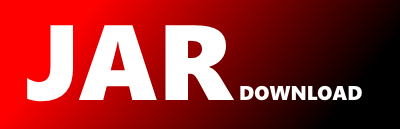
com.yixan.tools.common.files.FileManager Maven / Gradle / Ivy
The newest version!
package com.yixan.tools.common.files;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.RandomAccessFile;
import java.nio.MappedByteBuffer;
import java.nio.channels.FileChannel;
import java.nio.channels.FileChannel.MapMode;
import java.util.Properties;
import org.csource.fastdfs.ClientGlobal;
import org.csource.fastdfs.ProtoCommon;
import org.csource.fastdfs.ServerInfo;
import org.csource.fastdfs.StorageClient;
import org.csource.fastdfs.StorageServer;
import org.csource.fastdfs.TrackerClient;
import org.csource.fastdfs.TrackerServer;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.core.io.ClassPathResource;
import org.springframework.core.io.Resource;
import com.yixan.tools.common.util.FileUtil;
/**
* 文件上传工具类
*
* @author luyujian
* @author zhaohuihua
* @version C01 2015-3-26
*/
public class FileManager {
/** 日志对象 **/
private static final Logger log = LoggerFactory.getLogger(FileManager.class);
/** 配置文件相对路径 **/
private static final String PROPERTIES = "WEB-INF/classes/settings/store/fastdfs.cfg";
private static TrackerClient trackerClient;
private static TrackerServer trackerServer;
private static StorageServer storageServer;
private static StorageClient storageClient;
private static String serverUrl;
private static Properties properties = new Properties();
static { // Initialize Fast DFS Client configurations
Resource res = new ClassPathResource(PROPERTIES);
try (InputStream input = res.getInputStream();
InputStreamReader is = new InputStreamReader(input, "UTF-8")) {
properties.load(is);
serverUrl = properties.getProperty("http.server_url");
if (serverUrl == null) {
throw new IllegalStateException("properties 'http.server_url' not found");
}
if (!serverUrl.endsWith("/")) {
serverUrl += "/";
}
log.trace("Fast DFS configuration file path: " + PROPERTIES);
ClientGlobal.init(properties);
trackerClient = new TrackerClient();
trackerServer = trackerClient.getConnection();
if (trackerServer != null) {
storageClient = new StorageClient(trackerServer, storageServer);
//发送测试连接
ProtoCommon.activeTest(trackerServer.getSocket());
}
} catch (Exception e) {
log.error("", e);
}
}
/**
* 上传文件
*
* @param file
* @return
*/
public static String upload(File file) {
try {
String fileName = file.getName();
byte[] bytes = toByteArray(file);
return upload(fileName, bytes);
} catch (IOException e) {
log.error("IO Exception when uploading the file: " + file.getAbsolutePath(), e);
return null;
}
}
/**
* 上传文件
*
* @author luyujian
* @param file
* @return
*/
public static String upload(String filePath, InputStream is) {
try {
byte[] bytes = toByteArray(is);
return upload(filePath, bytes);
} catch (IOException e) {
log.error("IO Exception when uploading the file: " + filePath, e);
return null;
}
}
/**
* 上传文件
*
* @author luyujian
* @param file
* @return
*/
public static String upload(String filePath, byte[] bytes) {
log.trace("File:" + filePath + ", Length:" + bytes.length);
long startTime = System.currentTimeMillis();
String[] uploadResults = null;
try {
if (storageClient == null) {
storageClient = new StorageClient(trackerServer, storageServer);
trackerServer = trackerClient.getConnection();
if (trackerServer != null) {
// 发送测试连接
ProtoCommon.activeTest(trackerServer.getSocket());
}
}
String extension = FileUtil.getExtension(filePath, false);
uploadResults = storageClient.upload_file(bytes, extension, null);
} catch (IOException e) {
log.error("IO Exception when uploading the file: " + filePath, e);
} catch (Exception e) {
log.error("Non IO Exception when uploading the file: " + filePath, e);
}
if (uploadResults == null) {
log.error("upload file fail, error code: " + storageClient.getErrorCode());
return null;
}
String groupName = uploadResults[0];
String fileName = uploadResults[1];
String fileUrl = serverUrl + groupName + "/" + fileName;
if (log.isTraceEnabled()) {
long time = System.currentTimeMillis() - startTime;
log.trace("Upload file successfully!!! Time:{}ms, {}", time, fileUrl);
}
return fileUrl;
}
/**
* 将文件转为字节数组 Mapped File way MappedByteBuffer 可以在处理大文件时,提升性能
*
* @param filename
* @return
* @throws IOException
*/
public static byte[] toByteArray(String filename) throws IOException {
return toByteArray(new File(filename));
}
/**
* 将文件转为字节数组 Mapped File way MappedByteBuffer 可以在处理大文件时,提升性能
*
* @param file
* @return
* @throws IOException
*/
public static byte[] toByteArray(File file) throws IOException {
try (RandomAccessFile raf = new RandomAccessFile(file, "r")) {
FileChannel fc = raf.getChannel();
MappedByteBuffer byteBuffer = fc.map(MapMode.READ_ONLY, 0, fc.size()).load();
byte[] result = new byte[(int) fc.size()];
if (byteBuffer.remaining() > 0) {
// System.out.println("remain");
byteBuffer.get(result, 0, byteBuffer.remaining());
}
return result;
}
}
/**
* 将文件转为字节数组 Mapped File way MappedByteBuffer 可以在处理大文件时,提升性能
*
* @param file
* @return
* @throws IOException
*/
public static byte[] toByteArray(InputStream is) throws IOException {
try (ByteArrayOutputStream baos = new ByteArrayOutputStream();) {
int size = 1024;
byte[] buffer = new byte[size];
int index = 0;
while ((index = is.read(buffer, 0, size)) > 0) {
baos.write(buffer, 0, index);
}
return baos.toByteArray();
}
}
/**
* 删除文件
*
* @author luyujian
* @param groupName
* @param remoteFileName
* @throws Exception
*/
public static void deleteFile(String groupName, String remoteFileName) throws Exception {
storageClient.delete_file(groupName, remoteFileName);
}
public static String getConfigValue(String key) {
String value = properties.getProperty(key);
if (value == null) {
String fmt = "Config '{}' not found. file is '{}'.";
log.warn(fmt, key, PROPERTIES);
}
return value;
}
public static StorageServer[] getStoreStorages(String groupName) throws IOException {
return trackerClient.getStoreStorages(trackerServer, groupName);
}
public static ServerInfo[] getFetchStorages(String groupName, String remoteFileName) throws IOException {
return trackerClient.getFetchStorages(trackerServer, groupName, remoteFileName);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy