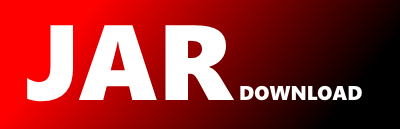
com.yixan.base.web.controller.FileController Maven / Gradle / Ivy
package com.yixan.base.web.controller;
import java.io.IOException;
import javax.annotation.PostConstruct;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.multipart.commons.CommonsMultipartFile;
import com.yixan.base.annotation.OperateRecord;
import com.yixan.base.common.api.file.model.FileAuthorization;
import com.yixan.base.common.api.file.model.FolderName;
import com.yixan.base.common.api.file.service.IFileService;
import com.yixan.base.core.exception.ResultCode;
import com.yixan.base.core.exception.ServiceException;
import com.yixan.base.core.result.ResponseMessage;
import com.yixan.base.web.utils.WebFileUtil;
import com.yixan.tools.common.util.VerifyUtil;
/**
* 文件上传下载控制器
*
* @author zhaohuihua
* @version C01 2015-3-25
*/
@Controller
@RequestMapping("/actions/file")
public class FileController {
@Autowired
private IFileService fileService;
private WebFileUtil uploader;
@PostConstruct
protected void init() {
this.uploader = new WebFileUtil(fileService);
}
/**
* 文件上传
* 文件扩展名必须在allow.upload.suffix配置中
*
* @param file 上传的文件内容
* @param folder 根文件夹, 可为空(为空时由fileService.groupUseDefIfNull决定默认用files还是不要根文件夹)
* @param filename 文件名, 用来获取文件扩展名
* @return
*/
@ResponseBody
@RequestMapping(value = "upload", method = RequestMethod.POST)
@OperateRecord("文件上传")
public ResponseMessage upload(@RequestParam("file") CommonsMultipartFile file, String folder, String filename,
String extra) {
try {
String url = uploader.upload(file, folder, filename, extra);
return new ResponseMessage(url);
} catch (ServiceException e) {
return new ResponseMessage(e);
}
}
/**
* 下载文件
* 文件扩展名必须在allow.download.suffix配置中
* 文件必须位于文件服务配置的文件夹或allow.download.folder配置中
* 如果: download.location.xxx = alias /home/tomcat/runtimelogs/
* 那么: path=xxx/web-user-error.log = /home/tomcat/runtimelogs/web/user-error.log文件
* 如果: download.location.xxx = root /home/tomcat/runtimelogs/
* 那么: path=xxx/web/user-error.log = /home/tomcat/runtimelogs/xxx/web/user-error.log文件
* 完整配置
* download.location.xxx = alias /home/tomcat/runtimelogs/
* allow.download.suffix.location.xxx = .log|.err|.txt
*
* @param path 文件的保存路径
* @param filename 下载时浏览器提示的文件名(可为空,从保存路径中截取)
* @throws IOException
* @throws ServiceException
*/
@RequestMapping("download")
@OperateRecord("文件下载")
public void download(HttpServletRequest request, HttpServletResponse response, String path, String filename)
throws IOException, ServiceException {
if (VerifyUtil.isBlank(path)) {
throw new ServiceException(ResultCode.PARAMTER_NOT_NULL);
}
uploader.download(request, response, path, filename);
}
@ResponseBody
@RequestMapping("authorize")
@OperateRecord(enable = false)
public ResponseMessage authorize(FolderName group) {
try {
FileAuthorization authorization = fileService.authorize(group);
return new ResponseMessage(authorization);
} catch (ServiceException e) {
return new ResponseMessage(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy