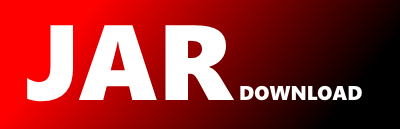
com.yixan.base.web.utils.CookieUtil Maven / Gradle / Ivy
package com.yixan.base.web.utils;
import java.io.UnsupportedEncodingException;
import java.net.URLDecoder;
import java.net.URLEncoder;
import java.util.HashMap;
import java.util.Map;
import javax.servlet.http.Cookie;
import javax.servlet.http.HttpServletResponse;
import com.yixan.tools.common.util.StringUtil;
/**
* Cookie操作工具类
*
* 实现对cookie的新增、查询、删除等功能
*
*
* @author 史怀周
* @version C01 2014年11月15日
* @since v1.0
*/
public class CookieUtil {
/**
* 增加cookie,生命周期为默认值
*
* @author 史怀周
* @param key cookie键值
* @param value cookie值
* @param path cookie路径
* @param response 响应对象
* @throws UnsupportedEncodingException
*/
public static void addCookie(String key, String value, String path, HttpServletResponse response) {
if (StringUtil.isNotEmpty(getCookie(key))) {
removeCookie(key, path, response);
}
addCookie(key, value, null, path, response);
}
/**
* 增加cookie,可设置生命周期
*
* @author 史怀周
* @param key cookie键值
* @param value cookie值
* @param maxAge 生命周期
* @param path cookie路径
* @param response 响应对象
* @throws UnsupportedEncodingException
*/
public static void addCookie(String key, String value, Integer maxAge, String path, HttpServletResponse response) {
Cookie cookie = new Cookie(key, encode(value));
cookie.setPath(path);
if (maxAge != null) {
cookie.setMaxAge(maxAge);
}
response.addCookie(cookie);
}
/**
* 移除指定Cookie
*
* @author 史怀周
* @param key cookie键值
* @param response 响应对象
*/
public static void removeCookie(String key, String path, HttpServletResponse response) {
Cookie cookie = new Cookie(key, null);
cookie.setMaxAge(0);
cookie.setPath(path);
response.addCookie(cookie);
}
/**
* 获取指定名称的cookie
*
* @author 史怀周
* @param key cookie键值
* @return cookie值
* @throws UnsupportedEncodingException
*/
public static String getCookie(String key) {
// 判断key是否为空
if (StringUtil.isEmpty(key)) {
return null;
}
// 判断客户端cookie列表是否为空
Cookie[] cookies = WebUtil.getInstance().getRequest().getCookies();
if (cookies == null || cookies.length == 0) {
return null;
}
// 从cookie列表中查询需要的cookie
for (Cookie c : cookies) {
if (c.getName().equals(key)) {
return decode(c.getValue());
}
}
return null;
}
/**
* 根据正则表达式获取key符合条件的Cookie列表并组装成Map返回
*
* @author 史怀周
* @param keyRegular 正则表达式
* @return
* @throws UnsupportedEncodingException
*/
public static Map getCookies(String keyRegular) {
// 判断key是否为空
if (StringUtil.isEmpty(keyRegular)) {
return null;
}
// 判断客户端cookie列表是否为空
Cookie[] cookies = WebUtil.getInstance().getRequest().getCookies();
if (cookies == null || cookies.length == 0) {
return null;
}
Map result = new HashMap();
for (Cookie c : cookies) {
if (c.getName().matches(keyRegular)) {
result.put(c.getName(), decode(c.getValue()));
}
}
return result;
}
/**
* 根据正则表达式删除所有名称匹配的cookie
*
* @author 史怀周
* @param keyRegular 正则表达式
* @param response
* @throws UnsupportedEncodingException
*/
public static void removeCookies(String keyRegular, HttpServletResponse response) {
// 判断key是否为空
if (StringUtil.isEmpty(keyRegular)) {
return;
}
// 判断客户端cookie列表是否为空
Cookie[] cookies = WebUtil.getInstance().getRequest().getCookies();
if (cookies.length == 0) {
return;
}
for (Cookie c : cookies) {
if (c.getName().matches(keyRegular)) {
removeCookie(c.getName(), null, response);
}
}
}
private static String encode(String value) {
try {
return URLEncoder.encode(value, "UTF-8");
} catch (UnsupportedEncodingException e) {
return value;
}
}
private static String decode(String value) {
try {
return URLDecoder.decode(value, "UTF-8");
} catch (UnsupportedEncodingException e) {
return value;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy