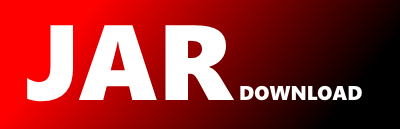
com.yixan.base.web.utils.FileIoUtil Maven / Gradle / Ivy
package com.yixan.base.web.utils;
import java.io.IOException;
import java.net.URLEncoder;
import java.util.ArrayList;
import java.util.List;
import javax.mail.internet.MimeUtility;
import javax.servlet.http.HttpServletRequest;
import org.springframework.web.multipart.MultipartFile;
import com.yixan.base.core.exception.ResultCode;
import com.yixan.base.core.exception.ServiceException;
import com.yixan.base.core.model.file.InputData;
import com.yixan.tools.common.util.VerifyUtil;
import eu.bitwalker.useragentutils.Browser;
import eu.bitwalker.useragentutils.UserAgent;
/**
* 文件上传处理
*
* @author zhaohuihua
* @version V1.0 2016年2月18日
*/
public abstract class FileIoUtil {
public static InputData getInputData(MultipartFile file) throws ServiceException {
if (file == null || file.getSize() == 0 || VerifyUtil.isBlank(file.getName())) {
return null;
}
try {
return new InputData(file.getOriginalFilename(), file.getInputStream(), file.getSize());
} catch (IOException e) {
throw new ServiceException(ResultCode.SERVER_INNER_ERROR, e);
}
}
public static List getInputData(MultipartFile[] files) throws ServiceException {
try {
List list = null;
if (files != null && files.length > 0) {
list = new ArrayList<>();
for (MultipartFile file : files) {
if (file == null || file.getSize() == 0 || VerifyUtil.isBlank(file.getName())) {
continue;
}
list.add(new InputData(file.getOriginalFilename(), file.getInputStream(), file.getSize()));
}
}
return list;
} catch (IOException e) {
throw new ServiceException(ResultCode.SERVER_INNER_ERROR, e);
}
}
/**
* 转换为附件下载描述, 防止中文文件名乱码
* response.setHeader("content-disposition", toAttachmentDesc(request, filename));
*
* @param request
* @param filename
* @return
* @throws ServiceException
* @throws IOException
*/
public static String toAttachmentDesc(HttpServletRequest request, String filename)
throws ServiceException, IOException {
String prefix = "attachment;filename";
String utf8name = URLEncoder.encode(filename, "UTF-8");
// 如果没有UA,则默认使用IE的方式进行编码,因为毕竟IE还是占多数的
String newname = prefix + "=\"" + utf8name + "\"";
String userAgent = request.getHeader("User-Agent");
if (userAgent != null) {
UserAgent ua = new UserAgent(userAgent);
Browser group = ua.getBrowser().getGroup();
if (group == Browser.OPERA || group == Browser.FIREFOX) {
// Opera浏览器只能采用filename*
// FireFox浏览器,可以使用MimeUtility或filename*或ISO编码的中文输出
newname = prefix + "*=UTF-8''" + utf8name;
} else if (group == Browser.SAFARI) {
// Safari浏览器,只能采用ISO编码的中文输出
newname = prefix + "=\"" + new String(filename.getBytes("UTF-8"), "ISO8859-1") + "\"";
} else if (group == Browser.CHROME) {
// Chrome浏览器,只能采用MimeUtility编码或ISO编码的中文输出
String name = MimeUtility.encodeText(filename, "UTF8", "B");
newname = prefix + "=\"" + name + "\"";
}
}
return newname;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy