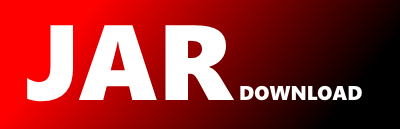
com.yixan.tools.common.util.KeyValue Maven / Gradle / Ivy
package com.yixan.tools.common.util;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
/**
* KeyValue类
*
* @author 赵卉华
* @version C01 2014年5月21日
*/
public class KeyValue implements Comparable>, Entry {
/** KEY **/
private String key;
/** VALUE **/
private V value;
/** 构造函数 **/
public KeyValue() {
}
/**
* 构造函数
*
* @param key KEY
* @param value VALUE
*/
public KeyValue(String key, V value) {
this.key = key;
this.value = value;
}
/** 获取KEY **/
@Override
public String getKey() {
return key;
}
/** 设置KEY **/
public void setKey(String key) {
this.key = key;
}
/** 获取VALUE **/
@Override
public V getValue() {
return value;
}
/** 设置VALUE **/
@Override
public V setValue(V value) {
V original = this.value;
this.value = value;
return original;
}
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + ((key == null) ? 0 : key.hashCode());
result = prime * result + ((value == null) ? 0 : value.hashCode());
return result;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (getClass() != obj.getClass()) {
return false;
}
@SuppressWarnings("rawtypes")
KeyValue other = (KeyValue) obj;
if (key == null) {
if (other.key != null) {
return false;
}
} else {
if (!key.equals(other.key)) {
return false;
}
}
if (value == null) {
if (other.value != null) {
return false;
}
} else {
if (!value.equals(other.value)) {
return false;
}
}
return true;
}
/**
* List转换为Map
*
* @author 赵卉华
* @param list KeyValue列表
* @return Map
*/
public static Map toMap(List> list) {
if (list == null) {
return null;
}
Map map = new HashMap();
for (KeyValue i : list) {
map.put(i.key, i.value);
}
return map;
}
/**
* Map转换为List
*
* @author 赵卉华
* @param map Map对象
* @return List
*/
public static List> toList(Map map) {
List> entries = new ArrayList>();
Set> original = map.entrySet();
for (Map.Entry entry : original) {
String key = entry.getKey();
V value = entry.getValue();
entries.add(new KeyValue(key, value));
}
return entries;
}
@Override
public String toString() {
return String.format("{%s=%s}", key, value);
}
@Override
public int compareTo(KeyValue o) {
return key == null ? 0 : key.compareTo(o.getKey());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy