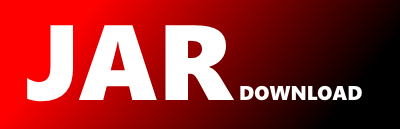
com.yixan.tools.common.sync.Args Maven / Gradle / Ivy
package com.yixan.tools.common.sync;
import java.util.Arrays;
/**
* 调用方法的参数
*
* @author zhh
* @version V1.0 2017年5月26日
*/
public interface Args {
int length();
Arg>[] get();
Class>[] types();
Object[] values();
public static class Arg {
private Class type;
private T value;
public Arg(Class type) {
this.type = type;
}
public Arg(Class type, V value) {
this.type = type;
this.value = value;
}
public Class getType() {
return type;
}
public T getValue() {
return value;
}
}
public static class AnyArgs implements Args {
private final Arg>[] args;
public AnyArgs(Object... objects) {
int length = objects == null ? 0 : objects.length;
this.args = new Arg>[length];
for (int i = 0; i < length; i++) {
Object o = objects[i];
if (o == null) {
throw new IllegalArgumentException("null value[" + i + "] must use new Arg(xxx.class, value)");
} else if (o instanceof Arg) {
Arg> arg = (Arg>) o;
this.args[i] = arg;
} else {
@SuppressWarnings("unchecked")
Class
© 2015 - 2024 Weber Informatics LLC | Privacy Policy