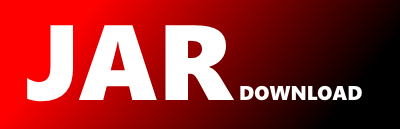
com.yixan.tools.common.util.ImageUtil Maven / Gradle / Ivy
package com.yixan.tools.common.util;
import java.awt.image.BufferedImage;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.Iterator;
import javax.imageio.ImageIO;
import javax.imageio.ImageReader;
import javax.imageio.stream.ImageInputStream;
import net.coobird.thumbnailator.Thumbnails;
import net.coobird.thumbnailator.Thumbnails.Builder;
import net.coobird.thumbnailator.geometry.Positions;
/**
* 图片工具
*
* @author zhaohuihua
*/
public class ImageUtil {
/**
* 生成缩略图
* 截取图片的中间部分(不产生变形)
* 如3000x2000生成100x100的缩略图
* 先截取中间的2000x2000的部分, 再缩小为100x100
*
* @param width
* @param height
* @throws IOException
*/
public static byte[] thumbnail(byte[] bytes, int width, int height) throws IOException {
ByteArrayInputStream is = new ByteArrayInputStream(bytes);
return thumbnail(is, width, height);
}
/**
* 生成缩略图
* 截取图片的中间部分(不产生变形)
* 如3000x2000生成100x100的缩略图
* 先截取中间的2000x2000的部分, 再缩小为100x100
*
* @param width
* @param height
* @throws IOException
*/
public static byte[] thumbnail(InputStream is, int width, int height) throws IOException {
ByteArrayOutputStream os = new ByteArrayOutputStream();
thumbnail(is, os, width, height);
return os.toByteArray();
}
/**
* 生成缩略图
* 截取图片的中间部分(不产生变形)
* 如3000x2000生成100x100的缩略图
* 先截取中间的2000x2000的部分, 再缩小为100x100
*
* @param width 缩放后的宽度, 0表示自动计算
* @param height 缩放后的宽度, 0表示自动计算
* @throws IOException
*/
public static void thumbnail(InputStream is, OutputStream os, int width, int height) throws IOException {
ImageInputStream iis = ImageIO.createImageInputStream(is);
Iterator iterator = ImageIO.getImageReaders(iis);
ImageReader reader = iterator.next();
reader.setInput(iis, true);
String imageType = reader.getFormatName();
BufferedImage image = reader.read(0);
int w = image.getWidth();
int h = image.getHeight();
if (width > 0 && height <= 0) {
height = (int) (1.0 * h / w * width);
} else if (width <= 0 && height > 0) {
width = (int) (1.0 * w / h * height);
} else if (width <= 0 && height <= 0) {
width = w;
height = h;
}
double wscale = 1.0 * width / w;
double hscale = 1.0 * height / h;
double scale = height / wscale > h ? hscale : wscale;
int nw = (int) (width / scale);
int nh = (int) (height / scale);
Builder> builder = Thumbnails.of(image);
builder.scale(scale);
builder.sourceRegion(Positions.CENTER, nw, nh);
builder.outputFormat(imageType).toOutputStream(os);
}
public static void main(String[] args) throws IOException {
int width = 400;
int height = 400;
String folder = "D:/image/";
// System.out.println(Arrays.toString(ImageIO.getWriterFormatNames()));
String[] files = new String[] { "1.png", "2.png", "he.jpg", "hw.jpg" };
for (String src : files) {
int index = src.lastIndexOf('.');
String dest = src.substring(0, index) + "." + width + "x" + height + src.substring(index);
try (InputStream is = new FileInputStream(new File(folder + src));
OutputStream os = new FileOutputStream(new File(folder + dest))) {
thumbnail(is, os, width, height);
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy