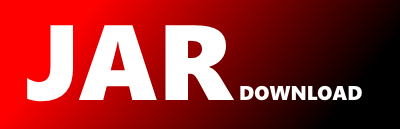
com.yixan.tools.common.util.ZipUtil Maven / Gradle / Ivy
package com.yixan.tools.common.util;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.URL;
import java.util.ArrayList;
import java.util.List;
import java.util.zip.ZipEntry;
import java.util.zip.ZipOutputStream;
/**
* zip压缩工具
*
* @author zhaohuihua
* @version V1.0 2016年2月23日
*/
public class ZipUtil {
/**
* URL配置
*
* @author zhaohuihua
* @version V1.0 2016年2月23日
*/
public static class UrlItem {
private String name;
private String url;
public UrlItem() {
}
public UrlItem(String name, String url) {
this.name = name;
this.url = url;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getUrl() {
return url;
}
public void setUrl(String url) {
this.url = url;
}
}
/**
* 通过URL下载文件并压缩
*
* @param urls
* @return
* @throws IOException
*/
public static byte[] compression(List urls) throws IOException {
try (ByteArrayOutputStream baos = new ByteArrayOutputStream();
ZipOutputStream zos = new ZipOutputStream(baos);
BufferedOutputStream output = new BufferedOutputStream(zos);) {
for (UrlItem i : urls) {
zos.putNextEntry(new ZipEntry(i.getName()));
URL url = new URL(i.getUrl());
try (BufferedInputStream input = new BufferedInputStream(url.openStream());) {
copy(input, output);
}
output.flush();
}
output.close();
return baos.toByteArray();
}
}
/**
* 通过URL下载文件并压缩保存到指定位置
*
* @param urls
* @param savePath
* @throws IOException
*/
public static void compression(List urls, String savePath) throws IOException {
byte[] bytes = compression(urls);
try (ByteArrayInputStream input = new ByteArrayInputStream(bytes);
FileOutputStream fos = new FileOutputStream(savePath);
BufferedOutputStream output = new BufferedOutputStream(fos)) {
copy(input, output);
output.flush();
output.close();
}
}
private static void copy(InputStream input, OutputStream output) throws IOException {
int length;
int bz = 2048;
byte[] buffer = new byte[bz];
while ((length = input.read(buffer, 0, buffer.length)) > 0) {
output.write(buffer, 0, length);
}
}
public static void main(String[] args) throws IOException {
List urls = new ArrayList<>();
urls.add(new UrlItem("百度.png", "http://www.baidu.com/img/bd_logo.png"));
urls.add(new UrlItem("腾讯.png", "http://mat1.gtimg.com/www/images/qq2012/qqlogo.png"));
compression(urls, "D:/测试.zip");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy