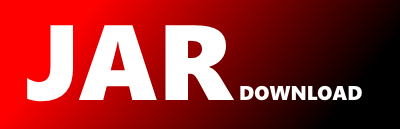
com.yodlee.api.model.AbstractProfile Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yodlee-api-model-beta Show documentation
Show all versions of yodlee-api-model-beta Show documentation
Yodlee API Model Beta is the stage version
/**
* Copyright (c) 2019 Yodlee, Inc. All Rights Reserved.
*
* Licensed under the MIT License. See LICENSE file in the project root for license information.
*/
package com.yodlee.api.model;
import java.util.Collections;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.yodlee.api.model.account.AccountAddress;
import io.swagger.annotations.ApiModelProperty;
public abstract class AbstractProfile extends AbstractModelComponent {
@ApiModelProperty(readOnly = true,
value = "Address available in the profile page of the account."//
+ "
"//
+ "Account Type: Aggregated
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "
")
@JsonProperty("address")
protected List addresses;
@ApiModelProperty(readOnly = true,
value = "Phone number available in the profile page of the account."//
+ "
"//
+ "Account Type: Aggregated
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "
")
@JsonProperty("phoneNumber")
protected List phoneNumbers;
@ApiModelProperty(readOnly = true,
value = "Email Id available in the profile page of the account."//
+ "
"//
+ "Account Type: Aggregated
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "
")
@JsonProperty("email")
protected List emails;
@ApiModelProperty(readOnly = true,
value = "Identifiers available in the profile page of the account."//
+ "
"//
+ "Account Type: Aggregated
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "
")
@JsonProperty("identifier")
protected List identifiers;
public AbstractProfile() {
super();
}
/**
* Email Id available in the profile page of the account.
*
* Aggregated / Manual: Aggregated
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
* - GET providerAccounts/profile
*
*
* @return email
*/
@JsonProperty("email")
public List getEmails() {
return emails == null ? null : Collections.unmodifiableList(emails);
}
/**
* Address available in the profile page of the account.
*
* Aggregated / Manual: Aggregated
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
* - GET providerAccounts/profile
*
*
* @return address
*/
@JsonProperty("address")
public List getAddresses() {
return addresses == null ? null : Collections.unmodifiableList(addresses);
}
/**
* Phone number available in the profile page of the account.
*
* Aggregated / Manual: Aggregated
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
* - GET providerAccounts/profile
*
*
* @return phoneNumber
*/
@JsonProperty("phoneNumber")
public List getPhoneNumbers() {
return phoneNumbers == null ? null : Collections.unmodifiableList(phoneNumbers);
}
/**
* Identifiers available in the profile page of the account.
*
* Aggregated / Manual: Aggregated
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
* - GET providerAccounts/profile
*
*
* @return identifier
*/
@JsonProperty("identifier")
public List getIdentifier() {
return identifiers == null ? null : Collections.unmodifiableList(identifiers);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy