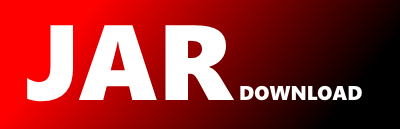
com.yodlee.api.model.account.Account Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yodlee-api-model-beta Show documentation
Show all versions of yodlee-api-model-beta Show documentation
Yodlee API Model Beta is the stage version
/**
* Copyright (c) 2019 Yodlee, Inc. All Rights Reserved.
*
* Licensed under the MIT License. See LICENSE file in the project root for license information.
*/
package com.yodlee.api.model.account;
import java.util.Collections;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.yodlee.api.model.AccountHolder;
import io.swagger.annotations.ApiModelProperty;
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({"fullAccountNumber", "fullAccountNumberList", "CONTAINER", "providerAccountId", "accountName", "accountStatus",
"accountNumber", "aggregationSource", "isAsset", "balance", "id", "userClassification", "includeInNetWorth",
"providerId", "providerName", "isManual", "availableBalance", "currentBalance", "accountType", "displayedName",
"createdDate", "sourceId", "dueDate", "401kLoan", "annuityBalance", "interestPaidYTD", "interestPaidLastYear",
"interestRateType", "collateral", "annualPercentageYield", "premium", "remainingBalance", "policyEffectiveDate",
"policyFromDate", "policyToDate", "deathBenefit", "policyTerm", "policyStatus", "apr", "cashApr",
"availableCash", "availableCredit", "cash", "cashValue", "classification", "expirationDate", "faceAmount",
"interestRate", "lastPayment", "lastPaymentAmount", "lastPaymentDate", "lastUpdated", "marginBalance",
"maturityAmount", "maturityDate", "moneyMarketBalance", "nickname", "runningBalance", "totalCashLimit",
"totalCreditLine", "totalUnvestedBalance", "totalVestedBalance", "escrowBalance", "homeInsuranceType",
"lifeInsuranceType", "originalLoanAmount", "principalBalance", "premiumPaymentTerm", "recurringPayment", "term",
"totalCreditLimit", "enrollmentDate", "primaryRewardUnit", "currentLevel", "nextLevel", "shortBalance",
"lastEmployeeContributionAmount", "lastEmployeeContributionDate", "memo", "originationDate", "overDraftLimit",
"valuationType", "homeValue", "estimatedDate", "address", "historicalBalances", "loanPayoffAmount",
"loanPayByDate", "profile", "bankTransferCode", "rewardBalance", "frequency", "amountDue", "minimumAmountDue",
"dataset", "holder", "associatedProviderAccountId", "loanPayOffDetails", "coverage", "sourceAccountStatus",
"repaymentPlanType", "guarantor", "lender", "paymentProfile", "autoRefresh", "oauthMigrationStatus"})
public class Account extends AbstractAccount {
@Deprecated
@ApiModelProperty(readOnly = true,
value = "Full account number of the account that is included only when include = fullAccountNumber is provided in the request. "
+ "For student loan account the account number that will be used for ACH or fund transfer"//
+ "
"//
+ "Aggregated / Manual: Both
"//
+ "Applicable containers: bank, creditCard, investment, insurance, loan, reward, otherAssets, otherLiabilities
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ " Note : fullAccountNumber is deprecated and is replaced with fullAccountNumberList in include parameter and response."
+ "
")
@JsonProperty("fullAccountNumber")
private String fullAccountNumber;
@ApiModelProperty(readOnly = true,
value = "Full account number List of the account that is included only when include = fullAccountNumberList is provided in the request. "
+ "
"//
+ "Aggregated / Manual: Both
"//
+ "Applicable containers: bank, creditCard, investment, insurance, loan, reward, otherAssets, otherLiabilities
"////
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "
")
@JsonProperty("fullAccountNumberList")
private FullAccountNumberList fullAccountNumberList;
@ApiModelProperty(readOnly = true,
value = "Profile information of the account."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: bank
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "
")
@JsonProperty("profile")
private AccountProfile profile;
@ApiModelProperty(readOnly = true,
value = "Holder details of the account."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: bank
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "
")
@JsonProperty("holder")
private List holders;
@ApiModelProperty(readOnly = true,
value = "The payment profile attribute contains information such as payment address, payment identifier, etc., "//
+ "that are required to set up a payment. "//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: loan
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "
")
@JsonProperty("paymentProfile")
private PaymentProfile paymentProfile;
@ApiModelProperty(readOnly = true,
value = "Auto refresh account-related information."//
+ "
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "
")
@JsonProperty("autoRefresh")
private AutoRefresh autoRefresh;
/**
* Auto refresh account-related information.
*
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return autoRefresh
*/
public AutoRefresh getAutoRefresh() {
return autoRefresh;
}
/**
* Full account number of the account that is included only when include = fullAccountNumber is provided in the
* request. For student loan account the account number that will be used for ACH or fund transfer
*
* Aggregated / Manual: Both
* Applicable containers: bank, creditCard, investment, insurance, loan, reward, otherAssets,
* otherLiabilities
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* Note : fullAccountNumber is deprecated and is replaced with fullAccountNumberList in include parameter and response.
*
* @return fullAccountNumber
*/
@Deprecated
public String getFullAccountNumber() {
return fullAccountNumber;
}
/**
* Full account number List of the account that is included only when include = fullAccountNumberList is provided in the
* request.
*
* Aggregated / Manual: Both
* Applicable containers: bank, creditCard, investment, insurance, loan, reward, otherAssets,
* otherLiabilities
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
*
* @return fullAccountNumberList
*/
public FullAccountNumberList getFullAccountNumberList() {
return fullAccountNumberList;
}
/**
* Holder details of the account.
*
* Aggregated / Manual: Aggregated
* Applicable containers: bank
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return holder
*/
@JsonProperty("holder")
public List getHolders() {
return holders == null ? null : Collections.unmodifiableList(holders);
}
/**
* Profile information of the account.
*
* Aggregated / Manual: Aggregated
* Applicable containers: bank
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return profile
*/
public AccountProfile getProfile() {
return profile;
}
/**
* The payment profile attribute contains information such as payment address, payment identifier, etc., that are
* required to set up a payment.
*
* Aggregated / Manual: Aggregated
* Applicable containers: loan
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return paymentProfile
*/
public PaymentProfile getPaymentProfile() {
return paymentProfile;
}
@Override
public String toString() {
return "Account [fullAccountNumber=" + fullAccountNumber + ", fullAccountNumberList=" + fullAccountNumberList + ", profile=" + profile + ", holders=" + holders
+ ", paymentProfile=" + paymentProfile + ", autoRefresh=" + autoRefresh + ", container=" + container
+ ", providerAccountId=" + providerAccountId + ", accountName=" + accountName + ", accountStatus="
+ accountStatus + ", accountNumber=" + accountNumber + ", aggregationSource=" + aggregationSource
+ ", isAsset=" + isAsset + ", balance=" + balance + ", id=" + id + ", userClassification="
+ userClassification + ", includeInNetWorth=" + includeInNetWorth + ", providerId=" + providerId
+ ", providerName=" + providerName + ", isManual=" + isManual + ", availableBalance=" + availableBalance
+ ", currentBalance=" + currentBalance + ", accountType=" + accountType + ", displayedName="
+ displayedName + ", createdDate=" + createdDate + ", sourceId=" + sourceId + ", dueDate=" + dueDate
+ ", loan401k=" + loan401k + ", annuityBalance=" + annuityBalance + ", interestPaidYTD="
+ interestPaidYTD + ", interestPaidLastYear=" + interestPaidLastYear + ", interestRateType="
+ interestRateType + ", collateral=" + collateral + ", annualPercentageYield=" + annualPercentageYield
+ ", premium=" + premium + ", remainingBalance=" + remainingBalance + ", policyEffectiveDate="
+ policyEffectiveDate + ", policyFromDate=" + policyFromDate + ", policyToDate=" + policyToDate
+ ", deathBenefit=" + deathBenefit + ", policyTerm=" + policyTerm + ", policyStatus=" + policyStatus
+ ", apr=" + apr + ", derivedApr =" + derivedApr + ", cashApr=" + cashApr + ", availableCash="
+ availableCash + ", availableCredit=" + availableCredit + ", cash=" + cash + ", cashValue=" + cashValue
+ ", classification=" + classification + ", expirationDate=" + expirationDate + ", faceAmount="
+ faceAmount + ", interestRate=" + interestRate + ", lastPayment=" + lastPayment
+ ", lastPaymentAmount=" + lastPaymentAmount + ", lastPaymentDate=" + lastPaymentDate + ", lastUpdated="
+ lastUpdated + ", marginBalance=" + marginBalance + ", maturityAmount=" + maturityAmount
+ ", maturityDate=" + maturityDate + ", moneyMarketBalance=" + moneyMarketBalance + ", nickname="
+ nickname + ", runningBalance=" + runningBalance + ", totalCashLimit=" + totalCashLimit
+ ", totalCreditLine=" + totalCreditLine + ", totalUnvestedBalance=" + totalUnvestedBalance
+ ", totalVestedBalance=" + totalVestedBalance + ", escrowBalance=" + escrowBalance
+ ", homeInsuranceType=" + homeInsuranceType + ", lifeInsuranceType=" + lifeInsuranceType
+ ", originalLoanAmount=" + originalLoanAmount + ", principalBalance=" + principalBalance
+ ", premiumPaymentTerm=" + premiumPaymentTerm + ", recurringPayment=" + recurringPayment + ", term="
+ term + ", totalCreditLimit=" + totalCreditLimit + ", enrollmentDate=" + enrollmentDate
+ ", primaryRewardUnit=" + primaryRewardUnit + ", currentLevel=" + currentLevel + ", nextLevel="
+ nextLevel + ", shortBalance=" + shortBalance + ", lastEmployeeContributionAmount="
+ lastEmployeeContributionAmount + ", lastEmployeeContributionDate=" + lastEmployeeContributionDate
+ ", memo=" + memo + ", originationDate=" + originationDate + ", overDraftLimit=" + overDraftLimit
+ ", valuationType=" + valuationType + ", homeValue=" + homeValue + ", estimatedDate=" + estimatedDate
+ ", address=" + address + ", loanPayoffAmount=" + loanPayoffAmount + ", loanPayByDate=" + loanPayByDate
+ ", bankTransferCode=" + bankTransferCode + ", rewardBalance=" + rewardBalance + ", frequency="
+ frequency + ", amountDue=" + amountDue + ", minimumAmountDue=" + minimumAmountDue + ", datasets="
+ datasets + ", associatedProviderAccountIds=" + associatedProviderAccountIds + ", loanPayoffDetails="
+ loanPayOffDetails + ", coverage=" + coverage + ", sourceAccountStatus=" + sourceAccountStatus
+ ", repaymentPlanType=" + repaymentPlanType + ", guarantor=" + guarantor + ", lender=" + lender
+ ", oauthMigrationStatus=" + openBankingMigrationStatusType +"]";
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy