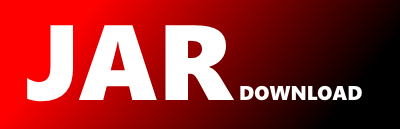
com.yodlee.api.model.account.AccountLatestBalance Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yodlee-api-model-beta Show documentation
Show all versions of yodlee-api-model-beta Show documentation
Yodlee API Model Beta is the stage version
/**
* Copyright (c) 2019 Yodlee, Inc. All Rights Reserved.
*
* Licensed under the MIT License. See LICENSE file in the project root for license information.
*/
package com.yodlee.api.model.account;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.yodlee.api.model.AbstractModelComponent;
import com.yodlee.api.model.Money;
import com.yodlee.api.model.account.enums.AccountLatestBalanceContainer;
import com.yodlee.api.model.account.enums.AccountLatestBalanceFailedReason;
import com.yodlee.api.model.account.enums.AccountLatestBalanceRefreshStatus;
import io.swagger.annotations.ApiModelProperty;
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({"CONTAINER", "providerId", "providerAccountId", "accountId", "providerName", "accountType",
"accountNumber", "accountName", "availableBalance", "currentBalance", "balance", "totalBalance", "cash",
"lastUpdated", "refreshStatus", "failedReason"})
public class AccountLatestBalance extends AbstractModelComponent {
@ApiModelProperty(readOnly = true,
value = "The type of service. E.g., Bank, Investment
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: bank, investment
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts/latestBalances
"//
+ "
"//
+ "Applicable Values
"//
)
@JsonProperty("CONTAINER")
protected AccountLatestBalanceContainer container;
@ApiModelProperty(readOnly = true,
value = "Identifier of the provider site. The primary key of provider resource. "//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: bank, investment
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts/latestBalances
"//
+ "
")
@JsonProperty("providerId")
protected String providerId;
@ApiModelProperty(readOnly = true,
value = "The primary key of the provider account resource."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: bank, investment
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts/latestBalances
"//
+ "
")
@JsonProperty("providerAccountId")
protected Long providerAccountId;
@ApiModelProperty(readOnly = true,
value = "The primary key of the provider account resource."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: bank, investment
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts/latestBalances
"//
+ "
")
@JsonProperty("accountId")
protected Long accountId;
@ApiModelProperty(readOnly = true,
value = "Service provider or institution name where the account originates. This belongs to the provider resource."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: bank, investment
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts/latestBalances
"//
+ "
")
@JsonProperty("providerName")
protected String providerName;
@ApiModelProperty(readOnly = true,
value = "The type of account that is aggregated, i.e., savings, checking, charge, HELOC, etc. "
+ "The account type is derived based on the attributes of the account. " //
+ "
Valid Values:"//
+ "
Aggregated Account Type"//
+ "
bank"//
+ ""//
+ "- CHECKING
"//
+ "- SAVINGS
"//
+ "- CD
"//
+ "- PPF
"//
+ "- RECURRING_DEPOSIT
"//
+ "- FSA
"//
+ "- MONEY_MARKET
"//
+ "- IRA
"//
+ "- PREPAID
"//
+ "
"//
+ "investment (SN 1.0)"//
+ "" + "- BROKERAGE_MARGIN
"//
+ "- HSA
"//
+ "- IRA
"//
+ "- BROKERAGE_CASH
"//
+ "- 401K
"//
+ "- 403B
"//
+ "- TRUST
"//
+ "- ANNUITY
"//
+ "- SIMPLE
"//
+ "- CUSTODIAL
"//
+ "- BROKERAGE_CASH_OPTION
"//
+ "- BROKERAGE_MARGIN_OPTION
"//
+ "- INDIVIDUAL
"//
+ "- CORPORATE
"//
+ "- JTTIC
"//
+ "- JTWROS
"//
+ "- COMMUNITY_PROPERTY
"//
+ "- JOINT_BY_ENTIRETY
"//
+ "- CONSERVATORSHIP
"//
+ "- ROTH
"//
+ "- ROTH_CONVERSION
"//
+ "- ROLLOVER
"//
+ "- EDUCATIONAL
"//
+ "- 529_PLAN
"//
+ "- 457_DEFERRED_COMPENSATION
"//
+ "- 401A
"//
+ "- PSP
"//
+ "- MPP
"//
+ "- STOCK_BASKET
"//
+ "- LIVING_TRUST
"//
+ "- REVOCABLE_TRUST
"//
+ "- IRREVOCABLE_TRUST
"//
+ "- CHARITABLE_REMAINDER
"//
+ "- CHARITABLE_LEAD
"//
+ "- CHARITABLE_GIFT_ACCOUNT
"//
+ "- SEP
"//
+ "- UTMA
"//
+ "- UGMA
"//
+ "- ESOPP
"//
+ "- ADMINISTRATOR
"//
+ "- EXECUTOR
"//
+ "- PARTNERSHIP
"//
+ "- SOLE_PROPRIETORSHIP
"//
+ "- CHURCH
"//
+ "- INVESTMENT_CLUB
"//
+ "- RESTRICTED_STOCK_AWARD
"//
+ "- CMA
"//
+ "- EMPLOYEE_STOCK_PURCHASE_PLAN
"//
+ "- PERFORMANCE_PLAN
"//
+ "- BROKERAGE_LINK_ACCOUNT
"//
+ "- MONEY_MARKET
"//
+ "- SUPER_ANNUATION
"//
+ "- REGISTERED_RETIREMENT_SAVINGS_PLAN
"//
+ "- SPOUSAL_RETIREMENT_SAVINGS_PLAN
"//
+ "- DEFERRED_PROFIT_SHARING_PLAN
"//
+ "- NON_REGISTERED_SAVINGS_PLAN
"//
+ "- REGISTERED_EDUCATION_SAVINGS_PLAN
"//
+ "- GROUP_RETIREMENT_SAVINGS_PLAN
"//
+ "- LOCKED_IN_RETIREMENT_SAVINGS_PLAN
"//
+ "- RESTRICTED_LOCKED_IN_SAVINGS_PLAN
"//
+ "- LOCKED_IN_RETIREMENT_ACCOUNT
"//
+ "- REGISTERED_PENSION_PLAN
"//
+ "- TAX_FREE_SAVINGS_ACCOUNT
"//
+ "- LIFE_INCOME_FUND
"//
+ "- REGISTERED_RETIREMENT_INCOME_FUND
"//
+ "- SPOUSAL_RETIREMENT_INCOME_FUND
"//
+ "- LOCKED_IN_REGISTERED_INVESTMENT_FUND
"//
+ "- PRESCRIBED_REGISTERED_RETIREMENT_INCOME_FUND
"//
+ "- GUARANTEED_INVESTMENT_CERTIFICATES
"//
+ "- REGISTERED_DISABILITY_SAVINGS_PLAN
"//
+ "- DIGITAL_WALLET
"//
+ "- OTHER
"//
+ "
"//
+ "investment (SN 2.0)"//
+ ""//
+ "- BROKERAGE_CASH
"//
+ "- BROKERAGE_MARGIN
"//
+ "- INDIVIDUAL_RETIREMENT_ACCOUNT_IRA
"//
+ "- EMPLOYEE_RETIREMENT_ACCOUNT_401K
"//
+ "- EMPLOYEE_RETIREMENT_SAVINGS_PLAN_403B
"//
+ "- TRUST
"//
+ "- ANNUITY
"//
+ "- SIMPLE_IRA
"//
+ "- CUSTODIAL_ACCOUNT
"//
+ "- BROKERAGE_CASH_OPTION
"//
+ "- BROKERAGE_MARGIN_OPTION
"//
+ "- INDIVIDUAL
"//
+ "- CORPORATE_INVESTMENT_ACCOUNT
"//
+ "- JOINT_TENANTS_TENANCY_IN_COMMON_JTIC
"//
+ "- JOINT_TENANTS_WITH_RIGHTS_OF_SURVIVORSHIP_JTWROS
"//
+ "- JOINT_TENANTS_COMMUNITY_PROPERTY
"//
+ "- JOINT_TENANTS_TENANTS_BY_ENTIRETY
"//
+ "- CONSERVATOR
"//
+ "- ROTH_IRA
"//
+ "- ROTH_CONVERSION
"//
+ "- ROLLOVER_IRA
"//
+ "- EDUCATIONAL
"//
+ "- EDUCATIONAL_SAVINGS_PLAN_529
"//
+ "- DEFERRED_COMPENSATION_PLAN_457
"//
+ "- MONEY_PURCHASE_RETIREMENT_PLAN_401A
"//
+ "- PROFIT_SHARING_PLAN
"//
+ "- MONEY_PURCHASE_PLAN
"//
+ "- STOCK_BASKET_ACCOUNT
"//
+ "- LIVING_TRUST
"//
+ "- REVOCABLE_TRUST
"//
+ "- IRREVOCABLE_TRUST
"//
+ "- CHARITABLE_REMAINDER_TRUST
"//
+ "- CHARITABLE_LEAD_TRUST
"//
+ "- CHARITABLE_GIFT_ACCOUNT
"//
+ "- SEP_IRA
"//
+ "- UNIFORM_TRANSFER_TO_MINORS_ACT_UTMA
"//
+ "- UNIFORM_GIFT_TO_MINORS_ACT_UGMA
"//
+ "- EMPLOYEE_STOCK_OWNERSHIP_PLAN_ESOP
"//
+ "- ADMINISTRATOR
"//
+ "- EXECUTOR
"//
+ "- PARTNERSHIP
"//
+ "- PROPRIETORSHIP
"//
+ "- CHURCH_ACCOUNT
"//
+ "- INVESTMENT_CLUB
"//
+ "- RESTRICTED_STOCK_AWARD
"//
+ "- CASH_MANAGEMENT_ACCOUNT
"//
+ "- EMPLOYEE_STOCK_PURCHASE_PLAN_ESPP
"//
+ "- PERFORMANCE_PLAN
"//
+ "- BROKERAGE_LINK_ACCOUNT
"//
+ "- MONEY_MARKET_ACCOUNT
"//
+ "- SUPERANNUATION
"//
+ "- REGISTERED_RETIREMENT_SAVINGS_PLAN_RRSP
"//
+ "- SPOUSAL_RETIREMENT_SAVINGS_PLAN_SRSP
"//
+ "- DEFERRED_PROFIT_SHARING_PLAN_DPSP
"//
+ "- NON_REGISTERED_SAVINGS_PLAN_NRSP
"//
+ "- REGISTERED_EDUCATION_SAVINGS_PLAN_RESP
"//
+ "- GROUP_RETIREMENT_SAVINGS_PLAN_GRSP
"//
+ "- LOCKED_IN_RETIREMENT_SAVINGS_PLAN_LRSP
"//
+ "- RESTRICTED_LOCKED_IN_SAVINGS_PLAN_RLSP
"//
+ "- LOCKED_IN_RETIREMENT_ACCOUNT_LIRA
"//
+ "- REGISTERED_PENSION_PLAN_RPP
"//
+ "- TAX_FREE_SAVINGS_ACCOUNT_TFSA
"//
+ "- LIFE_INCOME_FUND_LIF
"//
+ "- REGISTERED_RETIREMENT_INCOME_FUND_RIF
"//
+ "- SPOUSAL_RETIREMENT_INCOME_FUND_SRIF
"//
+ "- LOCKED_IN_REGISTERED_INVESTMENT_FUND_LRIF
"//
+ "- PRESCRIBED_REGISTERED_RETIREMENT_INCOME_FUND_PRIF
"//
+ "- GUARANTEED_INVESTMENT_CERTIFICATES_GIC
"//
+ "- REGISTERED_DISABILITY_SAVINGS_PLAN_RDSP
"//
+ "- DEFINED_CONTRIBUTION_PLAN
"//
+ "- DEFINED_BENEFIT_PLAN
"//
+ "- EMPLOYEE_STOCK_OPTION_PLAN
"//
+ "- NONQUALIFIED_DEFERRED_COMPENSATION_PLAN_409A
"//
+ "- KEOGH_PLAN
"//
+ "- EMPLOYEE_RETIREMENT_ACCOUNT_ROTH_401K
"//
+ "- DEFERRED_CONTINGENT_CAPITAL_PLAN_DCCP
"//
+ "- EMPLOYEE_BENEFIT_PLAN
"//
+ "- EMPLOYEE_SAVINGS_PLAN
"//
+ "- HEALTH_SAVINGS_ACCOUNT_HSA
"//
+ "- COVERDELL_EDUCATION_SAVINGS_ACCOUNT_ESA
"//
+ "- TESTAMENTARY_TRUST
"//
+ "- ESTATE
"//
+ "- GRANTOR_RETAINED_ANNUITY_TRUST_GRAT
"//
+ "- ADVISORY_ACCOUNT
"//
+ "- NON_PROFIT_ORGANIZATION_501C
"//
+ "- HEALTH_REIMBURSEMENT_ARRANGEMENT_HRA
"//
+ "- INDIVIDUAL_SAVINGS_ACCOUNT_ISA
"//
+ "- CASH_ISA
"//
+ "- STOCKS_AND_SHARES_ISA
"//
+ "- INNOVATIVE_FINANCE_ISA
"//
+ "- JUNIOR_ISA
"//
+ "- EMPLOYEES_PROVIDENT_FUND_ORGANIZATION_EPFO
"//
+ "- PUBLIC_PROVIDENT_FUND_PPF
"//
+ "- EMPLOYEES_PENSION_SCHEME_EPS
"//
+ "- NATIONAL_PENSION_SYSTEM_NPS
"//
+ "- INDEXED_ANNUITY
"//
+ "- ANNUITIZED_ANNUITY
"//
+ "- VARIABLE_ANNUITY
"//
+ "- ROTH_403B
"//
+ "- SPOUSAL_IRA
"//
+ "- SPOUSAL_ROTH_IRA
"//
+ "- SARSEP_IRA
"//
+ "- SUBSTANTIALLY_EQUAL_PERIODIC_PAYMENTS_SEPP
"//
+ "- OFFSHORE_TRUST
"//
+ "- IRREVOCABLE_LIFE_INSURANCE_TRUST
"//
+ "- INTERNATIONAL_TRUST
"//
+ "- LIFE_INTEREST_TRUST
"//
+ "- EMPLOYEE_BENEFIT_TRUST
"//
+ "- PRECIOUS_METAL_ACCOUNT
"//
+ "- INVESTMENT_LOAN_ACCOUNT
"//
+ "- GRANTOR_RETAINED_INCOME_TRUST
"//
+ "- PENSION_PLAN
"//
+ "- DIGITAL_WALLET
"//
+ "- OTHER
"//
+ "
"//
+ ""//
+ "- GET accounts/latestBalances
"//
+ "
")
@JsonProperty("accountType")
protected String accountType;
@ApiModelProperty(readOnly = true,
value = "The account number as it appears on the site. (The POST accounts service response return this field as number)
"
+ "Additional Details: Bank / Investment:
"
+ " The account number for the bank account as it appears at the site.
"
+ "In most cases, the site does not display the full account number in the account summary page "
+ "and additional navigation is required to aggregate it.
"//
+ "Applicable containers: bank, investment
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Endpoints:
"//
+ ""//
+ "- GET accounts/latestBalances
"//
+ "
")
@JsonProperty("accountNumber")
protected String accountNumber;
@ApiModelProperty(readOnly = true,
value = "The account name as it appears at the site.
"//
+ "(The POST accounts service response return this field as name)
"//
+ "Applicable containers: bank, investment
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Endpoints:
"//
+ ""//
+ "- GET accounts/latestBalances
"//
+ "
")
@JsonProperty("accountName")
protected String accountName;
@ApiModelProperty(readOnly = true,
value = "The balance in the account that is available for spending. "
+ "For checking accounts with overdraft, available balance may include "
+ "overdraft amount, if end site adds overdraft balance to available balance.
"//
+ "Applicable containers: bank, investment
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Endpoints:
"//
+ ""//
+ "- GET accounts/latestBalances
"//
+ "
")
@JsonProperty("availableBalance")
protected Money availableBalance;
@ApiModelProperty(readOnly = true,
value = "The balance in the account that is available at the beginning of the "
+ "business day; it is equal to the ledger balance of the account.
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: bank
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts/latestBalances
"//
+ "
")
@JsonProperty("currentBalance")
protected Money currentBalance;
@ApiModelProperty(readOnly = true,
value = "The total account value. " + "
Additional Details:"
+ "
Bank: available balance or current balance."
+ "
Investment: The total balance of all the investment account, as it appears on the FI site."
+ "Applicable containers: bank, investment
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Endpoints:
"//
+ ""//
+ "- GET accounts/latestBalances
"//
+ "
")
@JsonProperty("balance")
protected Money balance;
@ApiModelProperty(readOnly = true,
value = "The total account value. " + "
Additional Details:"
+ "
Investment: The total balance of all the investment account, as it appears on the FI site."
+ "Applicable containers: investment
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Endpoints:
"//
+ ""//
+ "- GET accounts/latestBalances
"//
+ "
")
@JsonProperty("totalBalance")
protected Money totalBalance;
@ApiModelProperty(readOnly = true,
value = "The date time the account information was last retrieved from the provider site and updated in the Yodlee system.
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: bank, investment
"//
+ "Endpoints:"//
+ "- GET accounts/latestBalances
"//
+ "
")
@JsonProperty("lastUpdated")
protected String lastUpdated;
@ApiModelProperty(readOnly = true,
value = "The amount that is available for immediate withdrawal or the total amount available to purchase securities in a brokerage or investment account."
+ "
Note: The cash balance field is only applicable to brokerage related accounts.
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: investment
"//
+ "Endpoints:"//
+ "- GET accounts/latestBalances
"//
+ "
")
@JsonProperty("cash")
protected Money cash;
@ApiModelProperty(readOnly = true, value = "The status of the account balance refresh request."//
)
@JsonProperty("refreshStatus")
protected AccountLatestBalanceRefreshStatus refreshStatus;
@ApiModelProperty(readOnly = true, value = "The reason the account balance refresh failed.")//
@JsonProperty("failedReason")
protected AccountLatestBalanceFailedReason failedReason;
/**
* The type of service. E.g., Bank, Investment,.
*
* Aggregated / Manual: Aggregated
* Applicable containers: bank, investment
* Endpoints:
*
* - GET accounts/latestBalances
*
* Applicable Values
*
* @return CONTAINER
*/
@JsonProperty("CONTAINER")
public AccountLatestBalanceContainer getContainer() {
return container;
}
/**
* Identifier of the provider site. The primary key of provider resource.
*
* Aggregated / Manual: Aggregated
* Applicable containers: bank, investment
* Endpoints:
*
* - GET accounts/latestBalances
*
*
* @return providerId
*/
public String getProviderId() {
return providerId;
}
/**
* The primary key of the provider account resource.
*
* Aggregated / Manual: Aggregated
* Applicable containers: bank, investment
* Endpoints:
*
* - GET accounts/latestBalances
*
*
* @return providerAccountId
*/
public Long getProviderAccountId() {
return providerAccountId;
}
/**
* The primary key of the provider account resource.
*
* Aggregated / Manual: Aggregated
* Applicable containers: bank, investment
* Endpoints:
*
* - GET accounts/latestBalances
*
*
* @return accountId
*/
public Long getAccountId() {
return accountId;
}
/**
* Service provider or institution name where the account originates. This belongs to the provider resource.
*
* Aggregated / Manual: Aggregated
* Applicable containers: bank, investment
* Endpoints:
*
* - GET accounts/latestBalances
*
*
* @return providerName
*/
public String getProviderName() {
return providerName;
}
/**
* The type of account that is aggregated, i.e., savings, checking, charge, HELOC, etc. The account type is derived
* based on the attributes of the account.
* Valid Values:
* Aggregated Account Type
* bank
*
* - CHECKING
* - SAVINGS
* - CD
* - PPF
* - RECURRING_DEPOSIT
* - FSA
* - MONEY_MARKET
* - IRA
* - PREPAID
*
* investment (SN 1.0)
*
*
* - BROKERAGE_MARGIN
* - HSA
* - IRA
* - BROKERAGE_CASH
* - 401K
* - 403B
* - TRUST
* - ANNUITY
* - SIMPLE
* - CUSTODIAL
* - BROKERAGE_CASH_OPTION
* - BROKERAGE_MARGIN_OPTION
* - INDIVIDUAL
* - CORPORATE
* - JTTIC
* - JTWROS
* - COMMUNITY_PROPERTY
* - JOINT_BY_ENTIRETY
* - CONSERVATORSHIP
* - ROTH
* - ROTH_CONVERSION
* - ROLLOVER
* - EDUCATIONAL
* - 529_PLAN
* - 457_DEFERRED_COMPENSATION
* - 401A
* - PSP
* - MPP
* - STOCK_BASKET
* - LIVING_TRUST
* - REVOCABLE_TRUST
* - IRREVOCABLE_TRUST
* - CHARITABLE_REMAINDER
* - CHARITABLE_LEAD
* - CHARITABLE_GIFT_ACCOUNT
* - SEP
* - UTMA
* - UGMA
* - ESOPP
* - ADMINISTRATOR
* - EXECUTOR
* - PARTNERSHIP
* - SOLE_PROPRIETORSHIP
* - CHURCH
* - INVESTMENT_CLUB
* - RESTRICTED_STOCK_AWARD
* - CMA
* - EMPLOYEE_STOCK_PURCHASE_PLAN
* - PERFORMANCE_PLAN
* - BROKERAGE_LINK_ACCOUNT
* - MONEY_MARKET
* - SUPER_ANNUATION
* - REGISTERED_RETIREMENT_SAVINGS_PLAN
* - SPOUSAL_RETIREMENT_SAVINGS_PLAN
* - DEFERRED_PROFIT_SHARING_PLAN
* - NON_REGISTERED_SAVINGS_PLAN
* - REGISTERED_EDUCATION_SAVINGS_PLAN
* - GROUP_RETIREMENT_SAVINGS_PLAN
* - LOCKED_IN_RETIREMENT_SAVINGS_PLAN
* - RESTRICTED_LOCKED_IN_SAVINGS_PLAN
* - LOCKED_IN_RETIREMENT_ACCOUNT
* - REGISTERED_PENSION_PLAN
* - TAX_FREE_SAVINGS_ACCOUNT
* - LIFE_INCOME_FUND
* - REGISTERED_RETIREMENT_INCOME_FUND
* - SPOUSAL_RETIREMENT_INCOME_FUND
* - LOCKED_IN_REGISTERED_INVESTMENT_FUND
* - PRESCRIBED_REGISTERED_RETIREMENT_INCOME_FUND
* - GUARANTEED_INVESTMENT_CERTIFICATES
* - REGISTERED_DISABILITY_SAVINGS_PLAN
* - OTHER
*
* investment (SN 2.0)
*
* - BROKERAGE_CASH
* - BROKERAGE_MARGIN
* - INDIVIDUAL_RETIREMENT_ACCOUNT_IRA
* - EMPLOYEE_RETIREMENT_ACCOUNT_401K
* - EMPLOYEE_RETIREMENT_SAVINGS_PLAN_403B
* - TRUST
* - ANNUITY
* - SIMPLE_IRA
* - CUSTODIAL_ACCOUNT
* - BROKERAGE_CASH_OPTION
* - BROKERAGE_MARGIN_OPTION
* - INDIVIDUAL
* - CORPORATE_INVESTMENT_ACCOUNT
* - JOINT_TENANTS_TENANCY_IN_COMMON_JTIC
* - JOINT_TENANTS_WITH_RIGHTS_OF_SURVIVORSHIP_JTWROS
* - JOINT_TENANTS_COMMUNITY_PROPERTY
* - JOINT_TENANTS_TENANTS_BY_ENTIRETY
* - CONSERVATOR
* - ROTH_IRA
* - ROTH_CONVERSION
* - ROLLOVER_IRA
* - EDUCATIONAL
* - EDUCATIONAL_SAVINGS_PLAN_529
* - DEFERRED_COMPENSATION_PLAN_457
* - MONEY_PURCHASE_RETIREMENT_PLAN_401A
* - PROFIT_SHARING_PLAN
* - MONEY_PURCHASE_PLAN
* - STOCK_BASKET_ACCOUNT
* - LIVING_TRUST
* - REVOCABLE_TRUST
* - IRREVOCABLE_TRUST
* - CHARITABLE_REMAINDER_TRUST
* - CHARITABLE_LEAD_TRUST
* - CHARITABLE_GIFT_ACCOUNT
* - SEP_IRA
* - UNIFORM_TRANSFER_TO_MINORS_ACT_UTMA
* - UNIFORM_GIFT_TO_MINORS_ACT_UGMA
* - EMPLOYEE_STOCK_OWNERSHIP_PLAN_ESOP
* - ADMINISTRATOR
* - EXECUTOR
* - PARTNERSHIP
* - PROPRIETORSHIP
* - CHURCH_ACCOUNT
* - INVESTMENT_CLUB
* - RESTRICTED_STOCK_AWARD
* - CASH_MANAGEMENT_ACCOUNT
* - EMPLOYEE_STOCK_PURCHASE_PLAN_ESPP
* - PERFORMANCE_PLAN
* - BROKERAGE_LINK_ACCOUNT
* - MONEY_MARKET_ACCOUNT
* - SUPERANNUATION
* - REGISTERED_RETIREMENT_SAVINGS_PLAN_RRSP
* - SPOUSAL_RETIREMENT_SAVINGS_PLAN_SRSP
* - DEFERRED_PROFIT_SHARING_PLAN_DPSP
* - NON_REGISTERED_SAVINGS_PLAN_NRSP
* - REGISTERED_EDUCATION_SAVINGS_PLAN_RESP
* - GROUP_RETIREMENT_SAVINGS_PLAN_GRSP
* - LOCKED_IN_RETIREMENT_SAVINGS_PLAN_LRSP
* - RESTRICTED_LOCKED_IN_SAVINGS_PLAN_RLSP
* - LOCKED_IN_RETIREMENT_ACCOUNT_LIRA
* - REGISTERED_PENSION_PLAN_RPP
* - TAX_FREE_SAVINGS_ACCOUNT_TFSA
* - LIFE_INCOME_FUND_LIF
* - REGISTERED_RETIREMENT_INCOME_FUND_RIF
* - SPOUSAL_RETIREMENT_INCOME_FUND_SRIF
* - LOCKED_IN_REGISTERED_INVESTMENT_FUND_LRIF
* - PRESCRIBED_REGISTERED_RETIREMENT_INCOME_FUND_PRIF
* - GUARANTEED_INVESTMENT_CERTIFICATES_GIC
* - REGISTERED_DISABILITY_SAVINGS_PLAN_RDSP
* - DEFINED_CONTRIBUTION_PLAN
* - DEFINED_BENEFIT_PLAN
* - EMPLOYEE_STOCK_OPTION_PLAN
* - NONQUALIFIED_DEFERRED_COMPENSATION_PLAN_409A
* - KEOGH_PLAN
* - EMPLOYEE_RETIREMENT_ACCOUNT_ROTH_401K
* - DEFERRED_CONTINGENT_CAPITAL_PLAN_DCCP
* - EMPLOYEE_BENEFIT_PLAN
* - EMPLOYEE_SAVINGS_PLAN
* - HEALTH_SAVINGS_ACCOUNT_HSA
* - COVERDELL_EDUCATION_SAVINGS_ACCOUNT_ESA
* - TESTAMENTARY_TRUST
* - ESTATE
* - GRANTOR_RETAINED_ANNUITY_TRUST_GRAT
* - ADVISORY_ACCOUNT
* - NON_PROFIT_ORGANIZATION_501C
* - HEALTH_REIMBURSEMENT_ARRANGEMENT_HRA
* - INDIVIDUAL_SAVINGS_ACCOUNT_ISA
* - CASH_ISA
* - STOCKS_AND_SHARES_ISA
* - INNOVATIVE_FINANCE_ISA
* - JUNIOR_ISA
* - EMPLOYEES_PROVIDENT_FUND_ORGANIZATION_EPFO
* - PUBLIC_PROVIDENT_FUND_PPF
* - EMPLOYEES_PENSION_SCHEME_EPS
* - NATIONAL_PENSION_SYSTEM_NPS
* - INDEXED_ANNUITY
* - ANNUITIZED_ANNUITY
* - VARIABLE_ANNUITY
* - ROTH_403B
* - SPOUSAL_IRA
* - SPOUSAL_ROTH_IRA
* - SARSEP_IRA
* - SUBSTANTIALLY_EQUAL_PERIODIC_PAYMENTS_SEPP
* - OFFSHORE_TRUST
* - IRREVOCABLE_LIFE_INSURANCE_TRUST
* - INTERNATIONAL_TRUST
* - LIFE_INTEREST_TRUST
* - EMPLOYEE_BENEFIT_TRUST
* - PRECIOUS_METAL_ACCOUNT
* - INVESTMENT_LOAN_ACCOUNT
* - GRANTOR_RETAINED_INCOME_TRUST
* - PENSION_PLAN
* - OTHER
*
*
* @return accountType
*/
public String getAccountType() {
return accountType;
}
/**
* The account number as it appears on the site. (The POST accounts service response return this field as
* number)
* Additional Details: Bank/ Investment:
* The account number for the bank account as it appears at the site.
* The account number can be full or partial based on how it is displayed in the account summary page of the site.
* In most cases, the site does not display the full account number in the account summary page and additional
* navigation is required to aggregate it.
* Applicable containers: bank, investment
* Aggregated / Manual: Aggregated
* Endpoints:
*
* - GET accounts/latestBalances
*
*
* @return accountNumber
*/
public String getAccountNumber() {
return accountNumber;
}
/**
* The account name as it appears at the site.
* (The POST accounts service response return this field as name)
* Applicable containers: bank, investment
* Aggregated / Manual: Aggregated
* Endpoints:
*
* - GET accounts/latestBalances
*
*
* @return accountName
*/
public String getAccountName() {
return accountName;
}
/**
* The balance in the account that is available for spending. For checking accounts with overdraft, available
* balance may include overdraft amount, if end site adds overdraft balance to available balance.
* Applicable containers: bank
* Aggregated / Manual: Aggregated
* Endpoints:
* GET accounts/latestBalances
*
* @return availableBalance
*/
public Money getAvailableBalance() {
return availableBalance;
}
/**
* The balance in the account that is available at the beginning of the business day; it is equal to the ledger
* balance of the account.
*
* Aggregated / Manual: Aggregated
* Applicable containers: bank
* Endpoints:
*
* - GET accounts/latestBalances
*
*
* @return currentBalance
*/
public Money getCurrentBalance() {
return currentBalance;
}
/**
* The total account value. *
* Additional Details:
* Bank: available balance or current balance.
* Investment: The total balance of all the investment account, as it appears on the FI site.
* Applicable containers: bank, investment
* Aggregated / Manual: Aggregated
* Endpoints:
*
* - GET accounts/latestBalances
*
*
* @return balance
*/
public Money getBalance() {
return balance;
}
/**
* The total account value. *
* Additional Details:
* Investment: The total balance of all the investment account, as it appears on the FI site.
* Applicable containers: bank, investment
* Aggregated / Manual: Aggregated
* Endpoints:
*
* - GET accounts/latestBalances
*
*
* @return totalBalance
*/
public Money getTotalBalance() {
return totalBalance;
}
/**
* The amount that is available for immediate withdrawal or the total amount available to purchase securities in a
* brokerage or investment account.
* Note: The cash balance field is only applicable to brokerage related accounts.
*
* Aggregated / Manual: Aggregated
* Applicable containers: investment
* Endpoints:
*
* - GET accounts/latestBalances
*
*
* @return cash
*/
public Money getCash() {
return cash;
}
/**
* The date time the account information was last retrieved from the provider site and updated in the Yodlee
* system.
*
* Aggregated / Manual: Aggregated
* Applicable containers: bank, investment
* Endpoints:
*
* - GET accounts/latestBalances
*
*
* @return lastUpdated
*/
public String getLastUpdated() {
return lastUpdated;
}
/**
* The status of the account balance refresh request.
*
* @return refreshStatus
*/
public AccountLatestBalanceRefreshStatus getRefreshStatus() {
return refreshStatus;
}
/**
* The reason the account balance refresh failed.
*
* @return failedReason
*/
public AccountLatestBalanceFailedReason getFailedReason() {
return failedReason;
}
@Override
public String toString() {
return "AccountLatestBalance [container=" + container + ", providerId=" + providerId + ", providerAccountId="
+ providerAccountId + ", accountId=" + accountId + ", providerName=" + providerName + ", accountType="
+ accountType + ", accountNumber=" + accountNumber + ", accountName=" + accountName
+ ", availableBalance=" + availableBalance + ", currentBalance=" + currentBalance + ", balance="
+ balance + ", totalBalance=" + totalBalance + ", lastUpdated=" + lastUpdated + ", cash=" + cash
+ ", refreshStatus=" + refreshStatus + ", failedReason=" + failedReason + "]";
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy