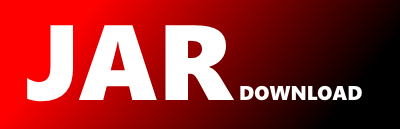
com.yodlee.api.model.account.Coverage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yodlee-api-model-beta Show documentation
Show all versions of yodlee-api-model-beta Show documentation
Yodlee API Model Beta is the stage version
/**
* Copyright (c) 2019 Yodlee, Inc. All Rights Reserved.
*
* Licensed under the MIT License. See LICENSE file in the project root for license information.
*/
package com.yodlee.api.model.account;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.yodlee.api.model.AbstractModelComponent;
import com.yodlee.api.model.account.enums.CoveragePlanType;
import com.yodlee.api.model.account.enums.CoverageType;
import io.swagger.annotations.ApiModelProperty;
public class Coverage extends AbstractModelComponent {
@ApiModelProperty(readOnly = true,
value = "The type of coverage provided to an individual or an entity."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: insurance,investment
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "
"//
+ "Applicable Values:
")
@JsonProperty("type")
private CoverageType type;
@ApiModelProperty(readOnly = true,
value = "The date on which the coverage for the account starts."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: insurance,investment
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "
")
@JsonProperty("startDate")
private String startDate;
@ApiModelProperty(readOnly = true,
value = "The date on which the coverage for the account ends or expires."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: insurance,investment
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "
")
@JsonProperty("endDate")
private String endDate;
@ApiModelProperty(readOnly = true,
value = "The coverage amount-related details."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: insurance,investment
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "
")
@JsonProperty("amount")
private List amount;
@ApiModelProperty(readOnly = true,
value = "The plan type for an insurance provided to an individual or an entity."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: insurance
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "
"//
+ "Applicable Values:
")
@JsonProperty("planType")
private CoveragePlanType planType;
/**
* The type of coverage provided to an individual or an entity.
*
* Aggregated / Manual: Aggregated
* Applicable containers: insurance,investment
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
* Applicable Values:
*
* @return type
*/
public CoverageType getType() {
return type;
}
public void setType(CoverageType type) {
this.type = type;
}
/**
* The date on which the coverage for the account starts.
*
* Aggregated / Manual: Aggregated
* Applicable containers: insurance,investment
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return startDate
*/
public String getStartDate() {
return startDate;
}
public void setStartDate(String startDate) {
this.startDate = startDate;
}
/**
* The date on which the coverage for the account ends or expires.
*
* Aggregated / Manual: Aggregated
* Applicable containers: insurance,investment
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return endDate
*/
public String getEndDate() {
return endDate;
}
public void setEndDate(String endDate) {
this.endDate = endDate;
}
/**
* The coverage amount-related details.
*
* Aggregated / Manual: Aggregated
* Applicable containers: insurance,investment
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return amount
*/
public List getAmount() {
return amount;
}
public void setAmount(List amount) {
this.amount = amount;
}
/**
* The plan type for an insurance provided to an individual or an entity.
*
* Aggregated / Manual: Aggregated
* Applicable containers: insurance
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
* Applicable Values:
*
* @return planType
*/
public CoveragePlanType getPlanType() {
return planType;
}
public void setPlanType(CoveragePlanType planType) {
this.planType = planType;
}
@Override
public String toString() {
return "Coverage [type=" + type + ", startDate=" + startDate + ", endDate=" + endDate + ", amount=" + amount
+ ", planType=" + planType + "]";
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy