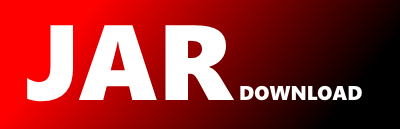
com.yodlee.api.model.account.CoverageAmount Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yodlee-api-model-beta Show documentation
Show all versions of yodlee-api-model-beta Show documentation
Yodlee API Model Beta is the stage version
/**
* Copyright (c) 2019 Yodlee, Inc. All Rights Reserved.
*
* Licensed under the MIT License. See LICENSE file in the project root for license information.
*/
package com.yodlee.api.model.account;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.yodlee.api.model.AbstractModelComponent;
import com.yodlee.api.model.Money;
import com.yodlee.api.model.account.enums.CoverageAmountType;
import com.yodlee.api.model.account.enums.CoverageLimitType;
import com.yodlee.api.model.account.enums.CoverageUnitType;
import io.swagger.annotations.ApiModelProperty;
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({"type", "limitType", "unitType", "limit", "cover", "met"})
public class CoverageAmount extends AbstractModelComponent {
@ApiModelProperty(readOnly = true,
value = "The type of coverage provided to an individual or an entity."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: insurance,investment
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "
"//
+ "Applicable Values:
")
@JsonProperty("type")
private CoverageAmountType type;
@ApiModelProperty(readOnly = true,
value = "The type of coverage limit indicates if the coverage is in-network or out-of-network."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: insurance,investment
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "
"//
+ "Applicable Values:
")
@JsonProperty("limitType")
private CoverageLimitType limitType;
@ApiModelProperty(readOnly = true,
value = "The type of coverage unit indicates if the coverage is for an individual or a family."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: insurance,investment
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "
"//
+ "Applicable Values:
")
@JsonProperty("unitType")
private CoverageUnitType unitType;
@ApiModelProperty(readOnly = true,
value = "The maximum amount that will be paid to an individual or an entity for a covered loss"//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: insurance,investment
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "
")
@JsonProperty("cover")
private Money cover;
@ApiModelProperty(readOnly = true,
value = "The amount the insurance company paid for the incurred medical expenses."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: insurance,investment
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "
")
@JsonProperty("met")
private Money met;
/**
* The type of coverage provided to an individual or an entity.
*
* Aggregated / Manual: Aggregated
* Applicable containers: insurance,investment
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
* Applicable Values:
*
* @return type
*/
public CoverageAmountType getType() {
return type;
}
public void setType(CoverageAmountType type) {
this.type = type;
}
/**
* The type of coverage limit indicates if the coverage is in-network or out-of-network.
*
* Aggregated / Manual: Aggregated
* Applicable containers: insurance,investment
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
* Applicable Values:
*
* @return limitType
*/
public CoverageLimitType getLimitType() {
return limitType;
}
public void setLimitType(CoverageLimitType limitType) {
this.limitType = limitType;
}
/**
* The type of coverage unit indicates if the coverage is for an individual or a family.
*
* Aggregated / Manual: Aggregated
* Applicable containers: insurance,investment
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
* Applicable Values:
*
* @return unitType
*/
public CoverageUnitType getUnitType() {
return unitType;
}
public void setUnitType(CoverageUnitType unitType) {
this.unitType = unitType;
}
/**
* The maximum amount that will be paid to an individual or an entity for a covered loss
*
* Aggregated / Manual: Aggregated
* Applicable containers: insurance,investment
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return cover
*/
public Money getCover() {
return cover;
}
public void setCover(Money cover) {
this.cover = cover;
}
/**
* The amount the insurance company paid for the incurred medical expenses.
*
* Aggregated / Manual: Aggregated
* Applicable containers: insurance,investment
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return met
*/
public Money getMet() {
return met;
}
public void setMet(Money met) {
this.met = met;
}
@Override
public String toString() {
return "CoverageAmount [type=" + type + ", limitType=" + limitType + ", unitType=" + unitType + ", cover="
+ cover + ", met=" + met + "]";
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy