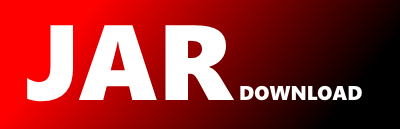
com.yodlee.api.model.consent.AbstractConsent Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yodlee-api-model-beta Show documentation
Show all versions of yodlee-api-model-beta Show documentation
Yodlee API Model Beta is the stage version
/**
* Copyright (c) 2019 Yodlee, Inc. All Rights Reserved.
*
* Licensed under the MIT License. See LICENSE file in the project root for license information.
*/
package com.yodlee.api.model.consent;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.yodlee.api.model.AbstractModelComponent;
import com.yodlee.api.model.consent.enums.ConsentStatus;
import com.yodlee.api.model.consent.enums.DataAccessFrequency;
import io.swagger.annotations.ApiModelProperty;
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({"consentId", "title", "titleBody", "startDate","expirationDate","applicationDisplayName", "dataAccessFrequency", "consentStatus",
"providerId", "renewal", "scope"})
public abstract class AbstractConsent extends AbstractModelComponent {
@ApiModelProperty(name = "consentId", required = true, value = "Consent Id generated through POST Consent.")
@JsonProperty("consentId")
protected Long consentId;
@ApiModelProperty(name = "title", required = true, value = "Title for the consent form.")
@JsonProperty("title")
protected String title;
@ApiModelProperty(name = "titleBody", required = true, value = "Description for the title.")
@JsonProperty("titleBody")
protected String titleBody;
@ApiModelProperty(name = "expirationDate", required = true, value = "Consent expiry date.")
@JsonProperty("expirationDate")
protected String expirationDate;
@ApiModelProperty(name = "startDate", required = true, value = "Consent start date.")
@JsonProperty("startDate")
protected String startDate;
@ApiModelProperty(name = "applicationDisplayName", required = true, value = "Application display name.")
@JsonProperty("applicationDisplayName")
protected String applicationDisplayName;
@ApiModelProperty(name = "dataAccessFrequency",
required = false,
value = "Data Access Frequency explains the number of times that this consent can be used."
+ "
"//
+ " Otherwise called as consent frequency type.") //
@JsonProperty("dataAccessFrequency")
protected DataAccessFrequency dataAccessFrequency;
@ApiModelProperty(name = "consentStatus", required = true, value = "Status of the consent.")
@JsonProperty("consentStatus")
protected ConsentStatus consentStatus;
@ApiModelProperty(name = "providerId",
required = true,
value = "Provider Id for which the consent needs to be generated.")
@JsonProperty("providerId")
protected Long providerId;
@ApiModelProperty(name = "renewal",
required = false,
value = "Renewal describes the sharing duration and reauthorization required.")
@JsonProperty("renewal")
protected Renewal renewal;
@ApiModelProperty(name = "scope",
required = true,
value = "Scope describes about the consent permissions and their purpose.")
@JsonProperty("scope")
protected List scopes;
/**
* Consent Id generated through POST Consent.
*
* Endpoints:
*
* - POST Consent
*
*
* @return consentId
*/
public Long getConsentId() {
return consentId;
}
/**
* Title for the consent form.
*
* Endpoints:
*
* - POST Consent
*
*
* @return title
*/
public String getTitle() {
return title;
}
/**
* Description for the title.
*
* Endpoints:
*
* - POST Consent
*
*
* @return titleBody
*/
public String getTitleBody() {
return titleBody;
}
/**
* Scope describes about the consent permissions and their purpose.
*
* Endpoints:
*
* - POST Consent
*
*
* @return scope
*/
@JsonProperty("scope")
public List getScopes() {
return scopes == null ? null : Collections.unmodifiableList(scopes);
}
/**
* Consent expiry date.
*
* Endpoints:
*
* - POST Consent
*
*
* @return expirationDate
*/
public String getExpirationDate() {
return expirationDate;
}
/**
* Data Access Frequency explains the number of times that this consent can be used.
* Otherwise called as consent frequency type.
*
* Endpoints:
*
* - POST Consent
*
*
* @return dataAccessFrequency
*/
public DataAccessFrequency getDataAccessFrequency() {
return dataAccessFrequency;
}
/**
* Status of the consent.
*
* Endpoints:
*
* - POST Consent
*
*
* @return consentStatus
*/
public ConsentStatus getConsentStatus() {
return consentStatus;
}
/**
* Unique identifier for the provider site. (e.g., financial institution sites, biller sites, lender sites, etc.).
*
*
* Endpoints:
*
* - POST Consent
*
*
* @return providerId
*/
public Long getProviderId() {
return providerId;
}
public void setConsentId(Long consentId) {
this.consentId = consentId;
}
public void setTitle(String title) {
this.title = title;
}
public void setTitleBody(String titleBody) {
this.titleBody = titleBody;
}
public void setExpirationDate(String expirationDate) {
this.expirationDate = expirationDate;
}
public void setDataAccessFrequency(DataAccessFrequency dataAccessFrequency) {
this.dataAccessFrequency = dataAccessFrequency;
}
public void setConsentStatus(ConsentStatus consentStatus) {
this.consentStatus = consentStatus;
}
public void setProviderId(Long providerId) {
this.providerId = providerId;
}
@JsonProperty("scope") public void setScopes(List scope) {
if (scopes == null) {
scopes = new ArrayList<>();
}
scopes = scope;
}
public boolean addScope(Scope scope) {
if (scopes == null) {
scopes = new ArrayList<>();
}
return this.scopes.add(scope);
}
public boolean removeScopeId(Scope scope) {
if (scopes != null) {
return this.scopes.remove(scope);
}
return false;
}
public void clearScopeIds() {
if (scopes != null) {
scopes.clear();
}
}
/**
* Consent start date.
*
* Endpoints:
*
* - GET Consent
*
*
* @return startDate
*/
public String getStartDate() {
return startDate;
}
public void setStartDate(String startDate) {
this.startDate = startDate;
}
/**
* application display name.
*
* Endpoints:
*
* - GET Consent
*
*
* @return applicationDisplayName
*/
public String getApplicationDisplayName() {
return applicationDisplayName;
}
public void setApplicationDisplayName(String applicationDisplayName) {
this.applicationDisplayName = applicationDisplayName;
}
/**
* containing default consent duration and reauthorization eligibility.
*
* Endpoints:
*
* - GET Consent
*
*
* @return renewal
*/
public Renewal getRenewal() {
return renewal;
}
public void setRenewal(Renewal renewal) {
this.renewal = renewal;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy