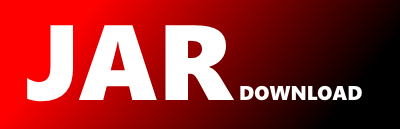
com.yodlee.api.model.consent.Scope Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yodlee-api-model-beta Show documentation
Show all versions of yodlee-api-model-beta Show documentation
Yodlee API Model Beta is the stage version
/**
* Copyright (c) 2019 Yodlee, Inc. All Rights Reserved.
*
* Licensed under the MIT License. See LICENSE file in the project root for license information.
*/
package com.yodlee.api.model.consent;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.yodlee.api.model.AbstractModelComponent;
import com.yodlee.api.model.consent.enums.DataCluster;
import io.swagger.annotations.ApiModelProperty;
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({"scopeId", "title", "titleBody", "datasetAttributes"})
public class Scope extends AbstractModelComponent {
@ApiModelProperty(name = "scopeId",
required = true,
value = "Unique Dataset Cluster name for the consent group like " + "
ACCOUNT_DETAILS"
+ "
STATEMENT_DETAILS" + "
CONTACT_DETAILS" + "
TRANSACTION_DETAILS")
@JsonProperty("scopeId")
private DataCluster scopeId;
@ApiModelProperty(name = "title", required = true, value = "Title for the Data Cluster.")
@JsonProperty("title")
private String title;
@ApiModelProperty(name = "titleBody", required = true, value = "Title body that explains the purpose of the scope.")
@JsonProperty("titleBody")
private List titleBodies;
@ApiModelProperty(name = "datasetAttributes",
required = false,
value = "Permissions that are associated with the Consent group like"
+ "
BASIC_AGG_DATA.BASIC_ACCOUNT_INFO" + "
BASIC_AGG_DATA.ACCOUNT_DETAILS"
+ "
BASIC_AGG_DATA.STATEMENTS" + "
BASIC_AGG_DATA.TRANSACTIONS"
+ "
ACCT_PROFILE.HOLDER_NAME" + "
ACCT_PROFILE.FULL_ACCT_NUMBER"
+ "
ACCT_PROFILE.BANK_TRANSFER_CODE" + "
ACCT_PROFILE.HOLDER_DETAILS")
@JsonProperty("datasetAttributes")
private List datasetAttributes;
/**
* Unique Dataset Cluster name for the consent group like
* ACCOUNT_DETAILS
* STATEMENT_DETAILS
* CONTACT_DETAILS
* TRANSACTION_DETAILS
*
* Endpoints:
*
* - POST Consent
* - GET Consent
*
*
* @return scopeId
*/
public DataCluster getScopeId() {
return scopeId;
}
/**
* Title for the Data Cluster.
*
* Endpoints:
*
* - POST Consent
* - GET Consent
*
*
* @return title
*/
public String getTitle() {
return title;
}
/**
* Title body that explains the purpose of the scope.
*
* Endpoints:
*
* - POST Consent
* - GET Consent
*
*
* @return titleBody
*/
@JsonProperty("titleBody")
public List getTitleBodies() {
return titleBodies == null ? null : Collections.unmodifiableList(titleBodies);
}
/**
* Permissions that are associated with the Consent group like
* BASIC_AGG_DATA.BASIC_ACCOUNT_INFO
* BASIC_AGG_DATA.ACCOUNT_DETAILS
* BASIC_AGG_DATA.STATEMENTS
* BASIC_AGG_DATA.TRANSACTIONS
* ACCT_PROFILE.HOLDER_NAME
* ACCT_PROFILE.FULL_ACCT_NUMBER
* ACCT_PROFILE.BANK_TRANSFER_CODE
* ACCT_PROFILE.HOLDER_DETAILS
*
* Endpoints:
*
* - POST Consent
* - GET Consent
*
*
* @return datasetAttributes
*/
public List getDatasetAttributes() {
return datasetAttributes == null ? null : Collections.unmodifiableList(datasetAttributes);
}
public void setScopeId(DataCluster scopeId) {
this.scopeId = scopeId;
}
public void setTitle(String title) {
this.title = title;
}
@JsonProperty("titleBody")
public void setTitleBodies(List titleBodies) {
if (this.titleBodies == null) {
this.titleBodies = new ArrayList<>();
}
this.titleBodies = titleBodies;
}
public boolean addTitleBodies(String titleBody) {
if (titleBodies == null) {
titleBodies = new ArrayList<>();
}
return titleBodies.add(titleBody);
}
public boolean removeTitleBodies(String titleBody) {
if (titleBodies != null) {
return titleBodies.remove(titleBody);
}
return false;
}
public void clearTitleBodies() {
if (titleBodies != null) {
titleBodies.clear();
}
}
public void setDatasetAttributes(List datasetAttributes) {
if (this.datasetAttributes == null) {
this.datasetAttributes = new ArrayList<>();
}
this.datasetAttributes = datasetAttributes;
}
public boolean addDatasetAttributes(String datasetAttributes) {
if (this.datasetAttributes == null) {
this.datasetAttributes = new ArrayList<>();
}
return this.datasetAttributes.add(datasetAttributes);
}
public boolean removeDatasetAttributes(String datasetAttributes) {
if (this.datasetAttributes != null) {
return this.datasetAttributes.remove(datasetAttributes);
}
return false;
}
public void clearDatasetAttributes() {
if (datasetAttributes != null) {
datasetAttributes.clear();
}
}
@Override
public String toString() {
return "Scope [scopeId=" + scopeId + ", title=" + title + ", titleBodies=" + titleBodies
+ ", datasetAttributes=" + datasetAttributes + "]";
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy