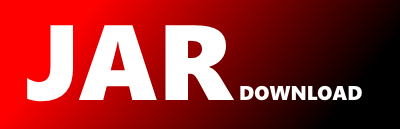
com.yodlee.api.model.dataextracts.DataExtractsProviderAccount Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yodlee-api-model-beta Show documentation
Show all versions of yodlee-api-model-beta Show documentation
Yodlee API Model Beta is the stage version
/**
* Copyright (c) 2019 Yodlee, Inc. All Rights Reserved.
*
* Licensed under the MIT License. See LICENSE file in the project root for license information.
*/
package com.yodlee.api.model.dataextracts;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.yodlee.api.model.provideraccounts.AbstractProviderAccount;
import io.swagger.annotations.ApiModelProperty;
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({"id", "aggregationSource", "providerId", "isManual", "createdDate", "requestId", "status",
"dataset", "loginForm", "isAutoRefreshPreferred", "isDataExtractsPreferred", "preferences", "isDeleted", "oauthMigrationStatus",
"destinationProviderAccountId", "sourceProviderAccountIds"})
public class DataExtractsProviderAccount extends AbstractProviderAccount {
@ApiModelProperty(readOnly = true,
value = "The date on when the provider account is created in the system."//
+ "
"//
+ "Endpoints:"//
+ ""//
+ "- GET providerAccounts
"//
+ "- POST providerAccounts
"//
+ "- PUT providerAccounts/{providerAccountId}
"//
+ "- GET providerAccounts/{providerAccountId}
"//
+ "
")
@JsonProperty("createdDate")
private String createdDate;
@ApiModelProperty(readOnly = true,
value = "Indicates if the provider account is deleted from the system."//
+ "Applicable containers: All Containers
"//
+ "Aggregated / Manual: Both
"//
+ "Endpoints:
"//
+ ""//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("isDeleted")
protected Boolean isDeleted;
@ApiModelProperty(readOnly = true,
value = "The providerAccountId that is retained as part of the many-to-one OAuth migration process.
"//
+ "Endpoints:"//
+ ""//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("destinationProviderAccountId")
protected Long destinationProviderAccountId;
@ApiModelProperty(readOnly = true,
value = "The providerAccountIds that are deleted and merged into the destinationProviderAccountId as part of the many-to-one OAuth migration process.
"//
+ "Endpoints:"//
+ ""//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("sourceProviderAccountIds")
protected List sourceProviderAccountIds;
/**
* The date on when the provider account is created in the system.
*
* Endpoints:
*
* - GET providerAccounts
* - POST providerAccounts
* - PUT providerAccounts/{providerAccountId}
* - GET providerAccounts/{providerAccountId}
*
*
* @return createdDate
*/
public String getCreatedDate() {
return createdDate;
}
/**
* Indicates if the account is marked as deleted. Applicable containers: All Containers
* Aggregated / Manual: Both
* Endpoints:
*
* - GET dataExtracts/userData
*
*
* @return isDeleted
*/
public Boolean getIsDeleted() {
return isDeleted;
}
/**
* The providerAccountId that is retained as part of the many-to-one OAuth migration process.
* Endpoints:
*
* - GET dataExtracts/userData
*
*
* @return the destinationProviderAccountId
*/
public Long getDestinationProviderAccountId() {
return destinationProviderAccountId;
}
/**
* The providerAccountIds that are deleted and merged into the destinationProviderAccountId as part of the many-to-one OAuth migration process.
* Endpoints:
*
* - GET dataExtracts/userData
*
*
* @return the sourceProviderAccountIds
*/
public List getSourceProviderAccountIds() {
return sourceProviderAccountIds;
}
@Override
public String toString() {
return "DataExtractsProviderAccount [createdDate=" + createdDate + ", id=" + id + ", aggregationSource="
+ aggregationSource + ", providerId=" + providerId + ", isManual=" + isManual + ", requestId="
+ requestId + ", status=" + status + ", datasets=" + datasets + ", isDeleted=" + isDeleted
+ ", oauthMigrationStatus=" + openBankingMigrationStatusType
+ ", destinationProviderAccountId=" + destinationProviderAccountId + ", sourceProviderAccountIds=" + sourceProviderAccountIds +"]";
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy