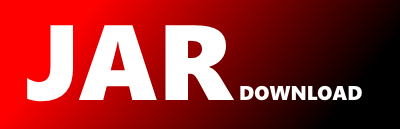
com.yodlee.api.model.derived.DerivedNetworthHistoricalBalance Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yodlee-api-model-beta Show documentation
Show all versions of yodlee-api-model-beta Show documentation
Yodlee API Model Beta is the stage version
/**
* Copyright (c) 2019 Yodlee, Inc. All Rights Reserved.
*
* Licensed under the MIT License. See LICENSE file in the project root for license information.
*/
package com.yodlee.api.model.derived;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.yodlee.api.model.AbstractModelComponent;
import com.yodlee.api.model.Money;
import com.yodlee.api.model.derived.enums.BalanceDataSourceType;
import io.swagger.annotations.ApiModelProperty;
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({"accountId", "date", "asOfDate", "balance", "isAsset", "dataSourceType"})
public class DerivedNetworthHistoricalBalance extends AbstractModelComponent {
@ApiModelProperty(readOnly = true)
@JsonProperty("accountId")
private Long accountId;
@ApiModelProperty(readOnly = true,
value = "Date for which the account balance was provided. This balance could be a carryforward, calculated or a scraped balance. "
+ "AdditIonal Details: scraped: Balance shown in the provider site. This balance gets stored in Yodlee system during system/user account updates. "
+ "carryForward : Balance carried forward from the scraped balance to the days for which the balance was not available in the system. Balance may not be available for all the days in the system due to MFA information required, error in the site, credential changes, etc. "
+ "calculated: Balances that gets calculated for the days that are prior to the account added date."//
+ "
"//
+ "Account Type: Aggregated and Manual
"//
+ "Applicable containers: bank, creditCard, investment, insurance, realEstate, loan
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts/historicalBalances
"//
+ "- GET derived/networth
"//
+ "
")
@JsonProperty("date")
private String date;
@ApiModelProperty(readOnly = true,
value = "Date as of when the balance is last updated due to the auto account updates or user triggered updates. "
+ "This balance will be carry forward for the days where there is no balance available in the system. "//
+ "
"//
+ "Account Type: Aggregated and Manual
"//
+ "Applicable containers: bank, creditCard, investment, insurance, realEstate, loan
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts/historicalBalances
"//
+ "
")
@JsonProperty("asOfDate")
private String asOfDate;
@ApiModelProperty(readOnly = true,
value = "Balance amount of the account."//
+ "
"//
+ "Account Type: Aggregated and Manual
"//
+ "Applicable containers: bank, creditCard, investment, insurance, realEstate, loan
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts/historicalBalances
"//
+ "
")
@JsonProperty("balance")
private Money balance;
@ApiModelProperty(readOnly = true,
value = "Indicates whether the balance is an asset or liability."//
+ "
"//
+ "Account Type: Aggregated and Manual
"//
+ "Applicable containers: bank, creditCard, investment, insurance, realEstate, loan
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts/historicalBalances
"//
+ "
")
@JsonProperty("isAsset")
private Boolean isAsset;
@ApiModelProperty(readOnly = true,
value = "The source of balance information."//
+ "
"//
+ "Account Type: Aggregated and Manual
"//
+ "Applicable containers: bank, creditCard, investment, insurance, realEstate, loan
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts/historicalBalances
"//
+ "
"//
+ "Applicable Values
"//
)
@JsonProperty("dataSourceType")
private BalanceDataSourceType dataSourceType;
public Long getAccountId() {
return accountId;
}
/**
* Date for which the account balance was provided. This balance could be a carryforward, calculated or a scraped
* balance. AdditIonal Details: scraped: Balance shown in the provider site. This balance gets stored in Yodlee
* system during system/user account updates. carryForward : Balance carried forward from the scraped balance to the
* days for which the balance was not available in the system. Balance may not be available for all the days in the
* system due to MFA information required, error in the site, credential changes, etc. calculated: Balances that
* gets calculated for the days that are prior to the account added date.
*
* Account Type: Aggregated and Manual
* Applicable containers: bank, creditCard, investment, insurance, realEstate, loan
* Endpoints:
*
* - GET accounts/historicalBalances
* - GET derived/networth
*
*
* @return date
*/
public String getDate() {
return date;
}
/**
* Date as of when the balance is last updated due to the auto account updates or user triggered updates. This
* balance will be carry forward for the days where there is no balance available in the system.
*
* Account Type: Aggregated and Manual
* Applicable containers: bank, creditCard, investment, insurance, realEstate, loan
* Endpoints:
*
* - GET accounts/historicalBalances
*
*
* @return asOfDate
*/
public String getAsOfDate() {
return asOfDate;
}
/**
* Balance amount of the account.
*
* Account Type: Aggregated and Manual
* Applicable containers: bank, creditCard, investment, insurance, realEstate, loan
* Endpoints:
*
* - GET accounts/historicalBalances
*
*
* @return balance
*/
public Money getBalance() {
return balance;
}
/**
* Indicates whether the balance is an asset or liability.
*
* Account Type: Aggregated and Manual
* Applicable containers: bank, creditCard, investment, insurance, realEstate, loan
* Endpoints:
*
* - GET accounts/historicalBalances
*
*
* @return isAsset
*/
public Boolean getIsAsset() {
return isAsset;
}
/**
* The source of balance information.
*
* Account Type: Aggregated and Manual
* Applicable containers: bank, creditCard, investment, insurance, realEstate, loan
* Endpoints:
*
* - GET accounts/historicalBalances
*
* Applicable Values
*
* @return dataSourceType
*/
public BalanceDataSourceType getDataSourceType() {
return dataSourceType;
}
@Override
public String toString() {
return "DerivedNetworthHistoricalBalance [accountId=" + accountId + ", date=" + date + ", asOfDate=" + asOfDate
+ ", balance=" + balance + ", isAsset=" + isAsset + ", dataSourceType=" + dataSourceType + "]";
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy