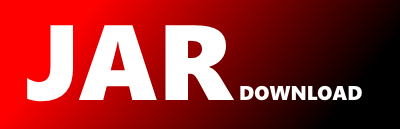
com.yodlee.api.model.derived.DerivedTransactionsSummary Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yodlee-api-model-beta Show documentation
Show all versions of yodlee-api-model-beta Show documentation
Yodlee API Model Beta is the stage version
/**
* Copyright (c) 2019 Yodlee, Inc. All Rights Reserved.
*
* Licensed under the MIT License. See LICENSE file in the project root for license information.
*/
package com.yodlee.api.model.derived;
import java.util.Collections;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.yodlee.api.model.AbstractModelComponent;
import com.yodlee.api.model.Money;
import com.yodlee.api.model.derived.enums.TransactionCategoryType;
import io.swagger.annotations.ApiModelProperty;
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({"categoryType", "creditTotal", "debitTotal", "categorySummary", "links"})
public class DerivedTransactionsSummary extends AbstractModelComponent {
@ApiModelProperty(readOnly = true,
value = "Type of categories provided by transactions/categories service."//
+ "
"//
+ "Applicable containers: creditCard, bank, investment
"//
+ "Applicable Values
"//
)
@JsonProperty("categoryType")
private TransactionCategoryType categoryType;
@ApiModelProperty(readOnly = true,
value = "The total of credit transactions for the category type."//
+ "
"//
+ "Applicable containers: creditCard, bank, investment
"//
)
@JsonProperty("creditTotal")
private Money creditTotal;
@ApiModelProperty(readOnly = true,
value = "The total of debit transactions for the category type."//
+ "
"//
+ "Applicable containers: creditCard, bank, investment
"//
)
@JsonProperty("debitTotal")
private Money debitTotal;
@ApiModelProperty(readOnly = true,
value = "Summary of transaction amouts at category level."//
+ "
"//
+ "Applicable containers: creditCard, bank, investment
"//
)
@JsonProperty("categorySummary")
private List categorySummaries;
@ApiModelProperty(readOnly = true,
value = "Link of the API services that corresponds to the value derivation."//
+ "
"//
+ "Applicable containers: creditCard, bank, investment
"//
)
@JsonProperty("links")
private DerivedTransactionsLinks links;
/**
* The total of debit transactions for the category type.
*
* Applicable containers: creditCard, bank, investment
*
* @return debitTotal
*/
public Money getDebitTotal() {
return debitTotal;
}
/**
* The total of credit transactions for the category type.
*
* Applicable containers: creditCard, bank, investment
*
* @return creditTotal
*/
public Money getCreditTotal() {
return creditTotal;
}
/**
* Type of categories provided by transactions/categories service.
*
* Applicable containers: creditCard, bank, investment
* Applicable Values
*
* @return categoryType
*/
public TransactionCategoryType getCategoryType() {
return categoryType;
}
/**
* Link of the API services that corresponds to the value derivation.
*
* Applicable containers: creditCard, bank, investment
*
* @return links
*/
public DerivedTransactionsLinks getLinks() {
return links;
}
/**
* Summary of transaction amounts at category level.
*
* Applicable containers: creditCard, bank, investment
*
* @return list of category summary
*/
public List getCategorySummaries() {
return categorySummaries == null ? null : Collections.unmodifiableList(categorySummaries);
}
@Override
public String toString() {
return "DerivedTransactionsSummary [categoryType=" + categoryType + ", creditTotal=" + creditTotal
+ ", debitTotal=" + debitTotal + ", categorySummaries=" + categorySummaries + ", links=" + links + "]";
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy