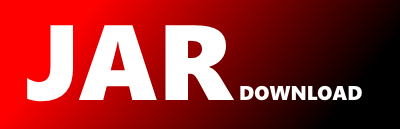
com.yodlee.api.model.enrichData.EnrichDataAccount Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yodlee-api-model-beta Show documentation
Show all versions of yodlee-api-model-beta Show documentation
Yodlee API Model Beta is the stage version
/**
* Copyright (c) 2019 Yodlee, Inc. All Rights Reserved.
*
* Licensed under the MIT License. See LICENSE file in the project root for license information.
*/
package com.yodlee.api.model.enrichData;
import javax.validation.constraints.NotNull;
import org.hibernate.validator.constraints.NotEmpty;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.yodlee.api.model.AbstractModelComponent;
import com.yodlee.api.model.Money;
import com.yodlee.api.model.account.enums.ItemAccountStatus;
import com.yodlee.api.model.enums.Container;
import io.swagger.annotations.ApiModelProperty;
@JsonInclude(JsonInclude.Include.NON_NULL)
public class EnrichDataAccount extends AbstractModelComponent {
@ApiModelProperty(value = "The type of service. E.g., Bank, Credit Card, Investment, Insurance, etc.
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: All containers
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "- POST dataEnrich/userData
"//
+ "
"//
+ "Applicable Values
"//
)
@NotNull(message = "{enrichData.data.invalid}")
@JsonProperty("container")
protected Container container;
@ApiModelProperty(value = "The amount that is available for an ATM withdrawal, i.e., the cash available after deducting the amount that is already withdrawn from the total cash limit. (totalCashLimit-cashAdvance= availableCash)"
+ "
Additional Details: The available cash amount at the account-level can differ from the available cash at the statement-level, as the information in the aggregated card account data provides more up-to-date information.
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: creditCard
"//
+ "Endpoints:
"//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "- POST dataEnrich/userData
"//
+ "
")
@JsonProperty("availableCash")
@NotNull(message = "{enrichData.data.invalid}")
protected Money availableCash;
@ApiModelProperty(value = "
Credit Card: Amount that is available to spend on the credit card. It is usually "
+ "the Total credit line- Running balance- pending charges. "
+ "
Loan: The unused portion of line of credit, on a revolving loan (such as a home-equity line of credit)."
+ "
Additional Details:"
+ "
Note: The available credit amount at the account-level can differ from the available credit "
+ "field at the statement-level, as the information in the aggregated card account data provides more up-to-date information.
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: creditCard, loan
"//
+ "Endpoints:"//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "- POST dataEnrich/userData
"//
+ "
")
@JsonProperty("availableCredit")
@NotNull(message = "{enrichData.data.invalid}")
protected Money availableCredit;
@ApiModelProperty(value = "The account name as it appears at the site.
"//
+ "(The POST accounts service response return this field as name)
"//
+ "Applicable containers: All Containers
"//
+ "Aggregated / Manual: Both
"//
+ "Endpoints:
"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "- POST dataEnrich/userData
"//
+ "
")
@JsonProperty("accountName")
protected String accountName;
@ApiModelProperty(value = "The name or identification of the account owner, as it appears at the FI site. "
+ "
Note: The account holder name can be full or partial based on how it is displayed in the account "
+ "summary page of the FI site. In most cases, the FI site does not display the full account holder "
+ "name in the account summary page."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: bank, creditCard, investment, insurance, loan, bill, reward
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "- POST dataEnrich/userData
"//
+ "
")
@JsonProperty("displayedName")
protected String displayedName;
@ApiModelProperty(value = "The type of account that is aggregated, i.e., savings, checking, credit card, charge, HELOC, etc. "
+ "The account type is derived based on the attributes of the account. " //
+ "
Valid Values:"//
+ "
Aggregated Account Type"//
+ "
bank"//
+ ""//
+ "- CHECKING
"//
+ "- SAVINGS
"//
+ "- CD
"//
+ "- PPF
"//
+ "- RECURRING_DEPOSIT
"//
+ "- FSA
"//
+ "- MONEY_MARKET
"//
+ "- IRA
"//
+ "- PREPAID
"//
+ "
"//
+ "creditCard"//
+ ""//
+ "- OTHER
"//
+ "- CREDIT
"//
+ "- STORE
"//
+ "- CHARGE
"//
+ "- OTHER
"//
+ "
"//
+ "investment (SN 1.0)"//
+ "" + "- BROKERAGE_MARGIN
"//
+ "- HSA
"//
+ "- IRA
"//
+ "- BROKERAGE_CASH
"//
+ "- 401K
"//
+ "- 403B
"//
+ "- TRUST
"//
+ "- ANNUITY
"//
+ "- SIMPLE
"//
+ "- CUSTODIAL
"//
+ "- BROKERAGE_CASH_OPTION
"//
+ "- BROKERAGE_MARGIN_OPTION
"//
+ "- INDIVIDUAL
"//
+ "- CORPORATE
"//
+ "- JTTIC
"//
+ "- JTWROS
"//
+ "- COMMUNITY_PROPERTY
"//
+ "- JOINT_BY_ENTIRETY
"//
+ "- CONSERVATORSHIP
"//
+ "- ROTH
"//
+ "- ROTH_CONVERSION
"//
+ "- ROLLOVER
"//
+ "- EDUCATIONAL
"//
+ "- 529_PLAN
"//
+ "- 457_DEFERRED_COMPENSATION
"//
+ "- 401A
"//
+ "- PSP
"//
+ "- MPP
"//
+ "- STOCK_BASKET
"//
+ "- LIVING_TRUST
"//
+ "- REVOCABLE_TRUST
"//
+ "- IRREVOCABLE_TRUST
"//
+ "- CHARITABLE_REMAINDER
"//
+ "- CHARITABLE_LEAD
"//
+ "- CHARITABLE_GIFT_ACCOUNT
"//
+ "- SEP
"//
+ "- UTMA
"//
+ "- UGMA
"//
+ "- ESOPP
"//
+ "- ADMINISTRATOR
"//
+ "- EXECUTOR
"//
+ "- PARTNERSHIP
"//
+ "- SOLE_PROPRIETORSHIP
"//
+ "- CHURCH
"//
+ "- INVESTMENT_CLUB
"//
+ "- RESTRICTED_STOCK_AWARD
"//
+ "- CMA
"//
+ "- EMPLOYEE_STOCK_PURCHASE_PLAN
"//
+ "- PERFORMANCE_PLAN
"//
+ "- BROKERAGE_LINK_ACCOUNT
"//
+ "- MONEY_MARKET
"//
+ "- SUPER_ANNUATION
"//
+ "- REGISTERED_RETIREMENT_SAVINGS_PLAN
"//
+ "- SPOUSAL_RETIREMENT_SAVINGS_PLAN
"//
+ "- DEFERRED_PROFIT_SHARING_PLAN
"//
+ "- NON_REGISTERED_SAVINGS_PLAN
"//
+ "- REGISTERED_EDUCATION_SAVINGS_PLAN
"//
+ "- GROUP_RETIREMENT_SAVINGS_PLAN
"//
+ "- LOCKED_IN_RETIREMENT_SAVINGS_PLAN
"//
+ "- RESTRICTED_LOCKED_IN_SAVINGS_PLAN
"//
+ "- LOCKED_IN_RETIREMENT_ACCOUNT
"//
+ "- REGISTERED_PENSION_PLAN
"//
+ "- TAX_FREE_SAVINGS_ACCOUNT
"//
+ "- LIFE_INCOME_FUND
"//
+ "- REGISTERED_RETIREMENT_INCOME_FUND
"//
+ "- SPOUSAL_RETIREMENT_INCOME_FUND
"//
+ "- LOCKED_IN_REGISTERED_INVESTMENT_FUND
"//
+ "- PRESCRIBED_REGISTERED_RETIREMENT_INCOME_FUND
"//
+ "- GUARANTEED_INVESTMENT_CERTIFICATES
"//
+ "- REGISTERED_DISABILITY_SAVINGS_PLAN
"//
+ "- DIGITAL_WALLET
"//
+ "- OTHER
"//
+ "
"//
+ "investment (SN 2.0)"//
+ ""//
+ "- BROKERAGE_CASH
"//
+ "- BROKERAGE_MARGIN
"//
+ "- INDIVIDUAL_RETIREMENT_ACCOUNT_IRA
"//
+ "- EMPLOYEE_RETIREMENT_ACCOUNT_401K
"//
+ "- EMPLOYEE_RETIREMENT_SAVINGS_PLAN_403B
"//
+ "- TRUST
"//
+ "- ANNUITY
"//
+ "- SIMPLE_IRA
"//
+ "- CUSTODIAL_ACCOUNT
"//
+ "- BROKERAGE_CASH_OPTION
"//
+ "- BROKERAGE_MARGIN_OPTION
"//
+ "- INDIVIDUAL
"//
+ "- CORPORATE_INVESTMENT_ACCOUNT
"//
+ "- JOINT_TENANTS_TENANCY_IN_COMMON_JTIC
"//
+ "- JOINT_TENANTS_WITH_RIGHTS_OF_SURVIVORSHIP_JTWROS
"//
+ "- JOINT_TENANTS_COMMUNITY_PROPERTY
"//
+ "- JOINT_TENANTS_TENANTS_BY_ENTIRETY
"//
+ "- CONSERVATOR
"//
+ "- ROTH_IRA
"//
+ "- ROTH_CONVERSION
"//
+ "- ROLLOVER_IRA
"//
+ "- EDUCATIONAL
"//
+ "- EDUCATIONAL_SAVINGS_PLAN_529
"//
+ "- DEFERRED_COMPENSATION_PLAN_457
"//
+ "- MONEY_PURCHASE_RETIREMENT_PLAN_401A
"//
+ "- PROFIT_SHARING_PLAN
"//
+ "- MONEY_PURCHASE_PLAN
"//
+ "- STOCK_BASKET_ACCOUNT
"//
+ "- LIVING_TRUST
"//
+ "- REVOCABLE_TRUST
"//
+ "- IRREVOCABLE_TRUST
"//
+ "- CHARITABLE_REMAINDER_TRUST
"//
+ "- CHARITABLE_LEAD_TRUST
"//
+ "- CHARITABLE_GIFT_ACCOUNT
"//
+ "- SEP_IRA
"//
+ "- UNIFORM_TRANSFER_TO_MINORS_ACT_UTMA
"//
+ "- UNIFORM_GIFT_TO_MINORS_ACT_UGMA
"//
+ "- EMPLOYEE_STOCK_OWNERSHIP_PLAN_ESOP
"//
+ "- ADMINISTRATOR
"//
+ "- EXECUTOR
"//
+ "- PARTNERSHIP
"//
+ "- PROPRIETORSHIP
"//
+ "- CHURCH_ACCOUNT
"//
+ "- INVESTMENT_CLUB
"//
+ "- RESTRICTED_STOCK_AWARD
"//
+ "- CASH_MANAGEMENT_ACCOUNT
"//
+ "- EMPLOYEE_STOCK_PURCHASE_PLAN_ESPP
"//
+ "- PERFORMANCE_PLAN
"//
+ "- BROKERAGE_LINK_ACCOUNT
"//
+ "- MONEY_MARKET_ACCOUNT
"//
+ "- SUPERANNUATION
"//
+ "- REGISTERED_RETIREMENT_SAVINGS_PLAN_RRSP
"//
+ "- SPOUSAL_RETIREMENT_SAVINGS_PLAN_SRSP
"//
+ "- DEFERRED_PROFIT_SHARING_PLAN_DPSP
"//
+ "- NON_REGISTERED_SAVINGS_PLAN_NRSP
"//
+ "- REGISTERED_EDUCATION_SAVINGS_PLAN_RESP
"//
+ "- GROUP_RETIREMENT_SAVINGS_PLAN_GRSP
"//
+ "- LOCKED_IN_RETIREMENT_SAVINGS_PLAN_LRSP
"//
+ "- RESTRICTED_LOCKED_IN_SAVINGS_PLAN_RLSP
"//
+ "- LOCKED_IN_RETIREMENT_ACCOUNT_LIRA
"//
+ "- REGISTERED_PENSION_PLAN_RPP
"//
+ "- TAX_FREE_SAVINGS_ACCOUNT_TFSA
"//
+ "- LIFE_INCOME_FUND_LIF
"//
+ "- REGISTERED_RETIREMENT_INCOME_FUND_RIF
"//
+ "- SPOUSAL_RETIREMENT_INCOME_FUND_SRIF
"//
+ "- LOCKED_IN_REGISTERED_INVESTMENT_FUND_LRIF
"//
+ "- PRESCRIBED_REGISTERED_RETIREMENT_INCOME_FUND_PRIF
"//
+ "- GUARANTEED_INVESTMENT_CERTIFICATES_GIC
"//
+ "- REGISTERED_DISABILITY_SAVINGS_PLAN_RDSP
"//
+ "- DEFINED_CONTRIBUTION_PLAN
"//
+ "- DEFINED_BENEFIT_PLAN
"//
+ "- EMPLOYEE_STOCK_OPTION_PLAN
"//
+ "- NONQUALIFIED_DEFERRED_COMPENSATION_PLAN_409A
"//
+ "- KEOGH_PLAN
"//
+ "- EMPLOYEE_RETIREMENT_ACCOUNT_ROTH_401K
"//
+ "- DEFERRED_CONTINGENT_CAPITAL_PLAN_DCCP
"//
+ "- EMPLOYEE_BENEFIT_PLAN
"//
+ "- EMPLOYEE_SAVINGS_PLAN
"//
+ "- HEALTH_SAVINGS_ACCOUNT_HSA
"//
+ "- COVERDELL_EDUCATION_SAVINGS_ACCOUNT_ESA
"//
+ "- TESTAMENTARY_TRUST
"//
+ "- ESTATE
"//
+ "- GRANTOR_RETAINED_ANNUITY_TRUST_GRAT
"//
+ "- ADVISORY_ACCOUNT
"//
+ "- NON_PROFIT_ORGANIZATION_501C
"//
+ "- HEALTH_REIMBURSEMENT_ARRANGEMENT_HRA
"//
+ "- INDIVIDUAL_SAVINGS_ACCOUNT_ISA
"//
+ "- CASH_ISA
"//
+ "- STOCKS_AND_SHARES_ISA
"//
+ "- INNOVATIVE_FINANCE_ISA
"//
+ "- JUNIOR_ISA
"//
+ "- EMPLOYEES_PROVIDENT_FUND_ORGANIZATION_EPFO
"//
+ "- PUBLIC_PROVIDENT_FUND_PPF
"//
+ "- EMPLOYEES_PENSION_SCHEME_EPS
"//
+ "- NATIONAL_PENSION_SYSTEM_NPS
"//
+ "- INDEXED_ANNUITY
"//
+ "- ANNUITIZED_ANNUITY
"//
+ "- VARIABLE_ANNUITY
"//
+ "- ROTH_403B
"//
+ "- SPOUSAL_IRA
"//
+ "- SPOUSAL_ROTH_IRA
"//
+ "- SARSEP_IRA
"//
+ "- SUBSTANTIALLY_EQUAL_PERIODIC_PAYMENTS_SEPP
"//
+ "- OFFSHORE_TRUST
"//
+ "- IRREVOCABLE_LIFE_INSURANCE_TRUST
"//
+ "- INTERNATIONAL_TRUST
"//
+ "- LIFE_INTEREST_TRUST
"//
+ "- EMPLOYEE_BENEFIT_TRUST
"//
+ "- PRECIOUS_METAL_ACCOUNT
"//
+ "- INVESTMENT_LOAN_ACCOUNT
"//
+ "- GRANTOR_RETAINED_INCOME_TRUST
"//
+ "- PENSION_PLAN
"//
+ "- DIGITAL_WALLET
"//
+ "- OTHER
"//
+ "
"//
+ "bill"//
+ ""//
+ "- TELEPHONE
"//
+ "- UTILITY
"//
+ "- CABLE
"//
+ "- WIRELESS
"//
+ "- BILLS
"//
+ "
"//
+ "loan"//
+ ""//
+ "- MORTGAGE
"//
+ "- INSTALLMENT_LOAN
"//
+ "- PERSONAL_LOAN
"//
+ "- HOME_EQUITY_LINE_OF_CREDIT
"//
+ "- LINE_OF_CREDIT
"//
+ "- AUTO_LOAN
"//
+ "- STUDENT_LOAN
"//
+ "- HOME_LOAN
"//
+ "
"//
+ "insurance"//
+ ""//
+ "- AUTO_INSURANCE
"//
+ "- HEALTH_INSURANCE
"//
+ "- HOME_INSURANCE
"//
+ "- LIFE_INSURANCE
"//
+ "- ANNUITY
"//
+ "- TRAVEL_INSURANCE
"//
+ "- INSURANCE
"//
+ "
"//
+ "realEstate"//
+ " " + "- REAL_ESTATE
"//
+ "
"//
+ "reward"//
+ ""//
+ "- REWARD_POINTS
"//
+ "
"//
+ "Manual Account Type
"//
+ "bank"//
+ ""//
+ "- CHECKING
"//
+ "- SAVINGS
"//
+ "- CD
"//
+ "- PREPAID
"//
+ "
"//
+ "credit"//
+ " " + "- CREDIT
"//
+ "
"//
+ "loan"//
+ " " + "- PERSONAL_LOAN
"//
+ "- HOME_LOAN
"//
+ "
"//
+ "bill"//
+ ""//
+ "- BILLS
"//
+ "
"//
+ "insurance"//
+ ""//
+ "- INSURANCE
"//
+ "- ANNUITY
"//
+ "
"//
+ "investment"//
+ ""//
+ "- BROKERAGE_CASH
"//
+ "
"//
+ "
"//
+ "Aggregated / Manual: Both
"//
+ "Applicable containers: All containers
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "- POST dataEnrich/userData
"//
+ "
")
@NotEmpty(message = "{enrichData.data.invalid}")
@JsonProperty("accountType")
protected String accountType;
@ApiModelProperty(value = "The account number as it appears on the site. (The POST accounts service response return this field as number)
"
+ "Additional Details: Bank/ Loan/ Insurance/ Investment/Bill:
"
+ " The account number for the bank account as it appears at the site.
"
+ "Credit Card: The account number of the card account as it appears at the site,
"
+ "i.e., the card number.The account number can be full or partial based on how it is displayed in the account summary page of the site."
+ "In most cases, the site does not display the full account number in the account summary page "
+ "and additional navigation is required to aggregate it.
"//
+ "Applicable containers: All Containers
"//
+ "Aggregated / Manual: Both
"//
+ "Endpoints:
"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- POST accounts
"//
+ "- GET dataExtracts/userData
"//
+ "- POST dataEnrich/userData
"//
+ "
")
@NotEmpty(message = "{enrichData.data.invalid}")
@JsonProperty("accountNumber")
protected String accountNumber;
// dluna- check with Ahila
@ApiModelProperty(value = "The loginName of the User."//
+ "
"//
+ "Applicable containers: bank,creditCard
"//
)
@NotEmpty(message = "{enrichData.data.invalid}")
@JsonProperty("userLoginName")
protected String userLoginName;
@JsonProperty("asOfDate")
protected String asOfDate;
@JsonProperty("accountHolderName")
protected String accountHolderName;
@ApiModelProperty(value = "The status of the account that is updated by the consumer through an application or an API. "
+ "Valid Values: AccountStatus" //
+ "
Additional Details:"
+ "
ACTIVE: All the added manual and aggregated accounts status will be made \"ACTIVE\" by default. "
+ "
TO_BE_CLOSED: If the aggregated accounts are not found or closed in the data provider site, Yodlee system marks the status as TO_BE_CLOSED"
+ "
INACTIVE: Users can update the status as INACTIVE to stop updating and to stop considering the account in other services"
+ "
CLOSED: Users can update the status as CLOSED, if the account is closed with the provider. "
+ "
"//
+ "Aggregated / Manual: Both
"//
+ "Applicable containers: All containers
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "- POST dataEnrich/userData
"//
+ "
"//
+ "Applicable Values
"//
)
@JsonProperty("accountStatus")
protected ItemAccountStatus accountStatus;
@ApiModelProperty(value = "The amount due to be paid for the account.
"//
+ "Additional Details:"
+ "Credit Card: The total amount due for the purchase of goods or services that must be paid by the due date.
"
+ "Loan: The amount due to be paid on the due date.
"
+ "Note: The amount due at the account-level can differ from the amount due at the statement-level, as the information in the aggregated card account data provides more up-to-date information.
"//
+ "Applicable containers: creditCard, loan, insurance, bill
"//
+ "Aggregated / Manual: Both
"//
+ "Endpoints:
"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "- POST dataEnrich/userData
"//
+ "
")
@JsonProperty("amountDue")
protected Money amountDue;
@ApiModelProperty(value = "The date on which the due amount has to be paid. " + "
Additional Details:"
+ "
Credit Card: The monthly date by when the minimum payment is due to be paid on the credit card account. "
+ "
Loan: The date on or before which the due amount should be paid."
+ "
Note: The due date at the account-level can differ from the due date field at the statement-level, as the "
+ "information in the aggregated card account data provides an up-to-date information to the consumer."
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: creditCard, loan, insurance, bill
"//
+ "Endpoints:"//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "- POST dataEnrich/userData
"//
+ "
")
@JsonProperty("dueDate")
protected String dueDate;
@ApiModelProperty(value = "The minimum amount due is the lowest amount of money that a consumer is required to pay each month."
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: creditCard, insurance, bill, loan
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "- POST dataEnrich/userData
"//
+ "
")
@JsonProperty("minimumAmountDue")
protected Money minimumAmountDue;
@ApiModelProperty(value = "The balance in the account that is available at the beginning of the "
+ "business day; it is equal to the ledger balance of the account.
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: bank
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@NotNull(message = "{enrichData.data.invalid}")
@JsonProperty("currentBalance")
protected Money currentBalance;
public Container getContainer() {
return container;
}
public void setContainer(Container container) {
this.container = container;
}
public Money getAvailableCash() {
return availableCash;
}
public void setAvailableCash(Money availableCash) {
this.availableCash = availableCash;
}
public Money getAvailableCredit() {
return availableCredit;
}
public void setAvailableCredit(Money availableCredit) {
this.availableCredit = availableCredit;
}
public String getAccountName() {
return accountName;
}
public void setAccountName(String accountName) {
this.accountName = accountName;
}
public String getDisplayedName() {
return displayedName;
}
public void setDisplayedName(String displayedName) {
this.displayedName = displayedName;
}
public String getAccountType() {
return accountType;
}
public void setAccountType(String accountType) {
this.accountType = accountType;
}
public String getAccountNumber() {
return accountNumber;
}
public void setAccountNumber(String accountNumber) {
this.accountNumber = accountNumber;
}
public String getUserLoginName() {
return userLoginName;
}
public void setUserLoginName(String userLoginName) {
this.userLoginName = userLoginName;
}
public String getAsOfDate() {
return asOfDate;
}
public void setAsOfDate(String asOfDate) {
this.asOfDate = asOfDate;
}
public String getAccountHolderName() {
return accountHolderName;
}
public void setAccountHolderName(String accountHolderName) {
this.accountHolderName = accountHolderName;
}
public ItemAccountStatus getAccountStatus() {
return accountStatus;
}
public void setAccountStatus(ItemAccountStatus accountStatus) {
this.accountStatus = accountStatus;
}
public Money getAmountDue() {
return amountDue;
}
public void setAmountDue(Money amountDue) {
this.amountDue = amountDue;
}
public String getDueDate() {
return dueDate;
}
public void setDueDate(String dueDate) {
this.dueDate = dueDate;
}
public Money getMinimumAmountDue() {
return minimumAmountDue;
}
public void setMinimumAmountDue(Money minimumAmountDue) {
this.minimumAmountDue = minimumAmountDue;
}
public Money getCurrentBalance() {
return currentBalance;
}
public void setCurrentBalance(Money currentBalance) {
this.currentBalance = currentBalance;
}
@Override
public String toString() {
return "EnrichDataAccount [container=" + container + ", availableCash=" + availableCash + ", availableCredit="
+ availableCredit + ", accountName=" + accountName + ", displayedName=" + displayedName
+ ", accountType=" + accountType + ", accountNumber=" + accountNumber + ", userLoginName="
+ userLoginName + ", asOfDate=" + asOfDate + ", accountHolderName=" + accountHolderName
+ ", accountStatus=" + accountStatus + ", amountDue=" + amountDue + ", dueDate=" + dueDate
+ ", minimumAmountDue=" + minimumAmountDue + ", currentBalance=" + currentBalance + "]";
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy