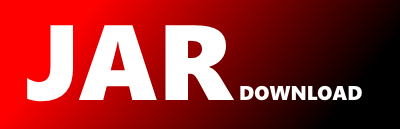
com.yodlee.api.model.enrichData.EnrichDataTransaction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yodlee-api-model-beta Show documentation
Show all versions of yodlee-api-model-beta Show documentation
Yodlee API Model Beta is the stage version
/**
* Copyright (c) 2019 Yodlee, Inc. All Rights Reserved.
*
* Licensed under the MIT License. See LICENSE file in the project root for license information.
*/
package com.yodlee.api.model.enrichData;
import javax.validation.constraints.NotNull;
import javax.validation.constraints.Size;
import org.hibernate.validator.constraints.NotEmpty;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.yodlee.api.model.AbstractModelComponent;
import com.yodlee.api.model.Money;
import com.yodlee.api.model.annotations.AllowedContainer;
import com.yodlee.api.model.enums.BaseType;
import com.yodlee.api.model.enums.Container;
import com.yodlee.api.model.transaction.Description;
import com.yodlee.api.model.transaction.enums.TransactionStatus;
import io.swagger.annotations.ApiModelProperty;
@JsonInclude(JsonInclude.Include.NON_NULL)
public class EnrichDataTransaction extends AbstractModelComponent {
@ApiModelProperty(value = "A unique ID that the provider site has assigned to the transaction. The source ID is only available for the pre-populated accounts."//
+ "
Pre-populated accounts are the accounts that the FI customers shares with Yodlee, so that the user does not have to add or aggregate those accounts."//
)
@Size(min = 1, max = 100, message = "{enrichData.data.invalid}")
@JsonProperty("sourceId")
protected String sourceId;
@JsonProperty("status")
protected TransactionStatus transactionStatus;
@AllowedContainer(value = {Container.bank, Container.creditCard},
message = "{dataEnrich.transaction.container.invalid}")
@ApiModelProperty(value = "The account's container."//
+ "
"//
+ "Applicable containers: bank,creditCard
"//
+ "Applicable Values
"//
)
@NotNull(message = "{enrichData.data.invalid}")
@JsonProperty("container")
protected Container container;
@ApiModelProperty(value = "The amount of the transaction as it appears at the FI site. "//
+ "
"//
+ "Applicable containers: bank,creditCard
"//
)
@NotNull(message = "{enrichData.data.invalid}")
@JsonProperty("amount")
protected Money amount;
@ApiModelProperty(value = "Description details"//
+ "
"//
+ "Applicable containers: bank,creditCard
"//
)
@NotNull(message = "{enrichData.data.invalid}")
@JsonProperty("description")
protected Description description;
@ApiModelProperty(value = "The date on which the transaction is posted to the account."//
+ "
"//
+ "Applicable containers: bank,creditCard
"//
)
@JsonProperty("postDate")
protected String postDate;
@ApiModelProperty(value = "The loginName of the User."//
+ "
"//
+ "Applicable containers: bank,creditCard
"//
)
@NotEmpty(message = "{enrichData.data.invalid}")
@JsonProperty("userLoginName")
protected String userLoginName;
@ApiModelProperty(value = "The account number as it appears on the site. (The POST accounts service response return this field as number)
"
+ "Additional Details: Bank/ Loan/ Insurance/ Investment/Bill:
"
+ " The account number for the bank account as it appears at the site.
"
+ "Credit Card: The account number of the card account as it appears at the site,
"
+ "i.e., the card number.The account number can be full or partial based on how it is displayed in the account summary page of the site."
+ "In most cases, the site does not display the full account number in the account summary page "
+ "and additional navigation is required to aggregate it.
"//
+ "Applicable containers: All Containers
"//
+ "Aggregated / Manual: Both
"//
+ "Endpoints:
"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- POST accounts
"//
+ "- POST dataExtracts/userData
"//
+ "- POST dataEnrich/userData
"//
+ "
")
@JsonProperty("accountNumber")
@NotEmpty(message = "{enrichData.data.invalid}")
protected String accountNumber;
@ApiModelProperty(value = "The date the transaction happens in the account. "//
+ "
"//
+ "Applicable containers: bank,creditCard
"//
)
@JsonProperty("transactionDate")
protected String transactionDate;
@ApiModelProperty(value = "Indicates if the transaction appears as a debit or a credit transaction in the account. "//
+ "
"//
+ "Applicable containers: bank,creditCard
"//
+ "Applicable Values
"//
)
@NotNull(message = "{enrichData.data.invalid}")
@JsonProperty("baseType")
protected BaseType baseType;
public String getSourceId() {
return sourceId;
}
public void setSourceId(String sourceId) {
this.sourceId = sourceId;
}
public Container getContainer() {
return container;
}
public void setContainer(Container container) {
this.container = container;
}
public Money getAmount() {
return amount;
}
public void setAmount(Money amount) {
this.amount = amount;
}
public Description getDescription() {
return description;
}
public void setDescription(Description description) {
this.description = description;
}
public String getPostDate() {
return postDate;
}
public void setPostDate(String postDate) {
this.postDate = postDate;
}
public String getUserLoginName() {
return userLoginName;
}
public void setUserLoginName(String userLoginName) {
this.userLoginName = userLoginName;
}
public String getAccountNumber() {
return accountNumber;
}
public void setAccountNumber(String accountNumber) {
this.accountNumber = accountNumber;
}
public String getTransactionDate() {
return transactionDate;
}
public void setTransactionDate(String transactionDate) {
this.transactionDate = transactionDate;
}
public BaseType getBaseType() {
return baseType;
}
public void setBaseType(BaseType baseType) {
this.baseType = baseType;
}
public TransactionStatus getTransactionStatus() {
return transactionStatus;
}
public void setTransactionStatus(TransactionStatus transactionStatus) {
this.transactionStatus = transactionStatus;
}
@Override
public String toString() {
return "DataEnrichTransaction [sourceId=" + sourceId + ", transactionStatus=" + transactionStatus
+ ", container=" + container + ", amount=" + amount + ", description=" + description + ", postDate="
+ postDate + ", userLoginName=" + userLoginName + ", accountNumber=" + accountNumber
+ ", transactionDate=" + transactionDate + ", baseType=" + baseType + "]";
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy