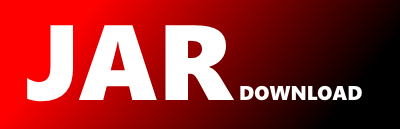
com.yodlee.api.model.holdings.AbstractHolding Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yodlee-api-model-beta Show documentation
Show all versions of yodlee-api-model-beta Show documentation
Yodlee API Model Beta is the stage version
/**
* Copyright (c) 2019 Yodlee, Inc. All Rights Reserved.
*
* Licensed under the MIT License. See LICENSE file in the project root for license information.
*/
package com.yodlee.api.model.holdings;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.yodlee.api.model.AbstractModelComponent;
import com.yodlee.api.model.Money;
import com.yodlee.api.model.enums.HoldingType;
import com.yodlee.api.model.enums.OptionType;
import io.swagger.annotations.ApiModelProperty;
@JsonInclude(JsonInclude.Include.NON_NULL)
public abstract class AbstractHolding extends AbstractModelComponent {
@ApiModelProperty(readOnly = true,
value = "Unique identifier for the security added in the system. This is the primary key of the holding resource."//
+ "
"//
+ "Applicable containers: investment, insurance
"//
)
@JsonProperty("id")
protected Long id;
@ApiModelProperty(readOnly = true,
value = "Unique identifier of the account to which the security is linked."//
+ "
"//
+ "Applicable containers: investment, insurance
"//
)
@JsonProperty("accountId")
protected Long accountId;
@ApiModelProperty(readOnly = true,
value = "Unique identifier for the user's association with the provider."//
+ "
"//
+ "Applicable containers: investment, insurance
"//
)
@JsonProperty("providerAccountId")
protected Long providerAccountId;
@ApiModelProperty(readOnly = true,
value = "In a one-off security purchase, the cost basis is the quantity acquired multiplied by the price per unit paid plus any commission paid. In case, the same position is acquired in different lots on different days at different prices, the sum total of the cost incurred is divided by the total units acquired to arrive at the average cost basis."//
+ "
"//
+ "Applicable containers: investment
"//
)
@JsonProperty("costBasis")
protected Money costBasis;
@ApiModelProperty(readOnly = true,
value = "The CUSIP (Committee on Uniform Securities Identification Procedures) identifies most the financial instruments in the United States and Canada."//
+ "
"//
+ "Applicable containers: investment
"//
)
@JsonProperty("cusipNumber")
protected String cusipNumber;
@ApiModelProperty(readOnly = true,
value = "Indicates the security type of holding identified through the security service."//
+ "
"//
+ "Applicable containers: investment, insurance
"//
)
@JsonProperty("securityType")
protected String securityType;
@ApiModelProperty(readOnly = true,
value = "Indicates the security style of holding identified through the security service."//
+ "
"//
+ "Applicable containers: investment, insurance
"//
)
@JsonProperty("securityStyle")
protected String securityStyle;
@ApiModelProperty(readOnly = true,
value = "Indicates the security match status id of the investment option identified during security normalization."//
+ "
"//
+ "Applicable containers: investment
"//
)
@JsonProperty("matchStatus")
protected String matchStatus;
@ApiModelProperty(readOnly = true,
value = "The description (name) for the holding (E.g., Cisco Systems)"
+ "
For insurance container, the field is only applicable for insurance annuity and variable life insurance types. "//
+ "
"//
+ "Applicable containers: investment, insurance
"//
)
@JsonProperty("description")
protected String description;
@ApiModelProperty(readOnly = true,
value = "The enrichedDescription is the security description of the normalized holding"
+ "
"//
+ "Applicable containers: investment, insurance
"//
)
@JsonProperty("enrichedDescription")
protected String enrichedDescription;
@ApiModelProperty(readOnly = true,
value = "The current price of the security."
+ "
Note: Only for bonds the price field indicates the normalized price and not the price aggregated from the site. For insurance container, the field is only applicable for insurance annuity and variable life insurance types."//
+ "
"//
+ "Applicable containers: investment, insurance
"//
)
@JsonProperty("price")
protected Money price;
@ApiModelProperty(readOnly = true,
value = "The quantity held for the holding."
+ "
Note: Only for bonds the quantity field indicates the normalized quantity and not the quantity aggregated from the site. The quantity field is only applicable to restricted stock units/awards, performance units, currency, and commodity."
+ "
For insurance container, the field is only applicable for insurance annuity and variable life insurance types."//
+ "
"//
+ "Applicable containers: investment, insurance
"//
)
@JsonProperty("quantity")
protected Double quantity;
@ApiModelProperty(readOnly = true,
value = "The symbol of the security."//
+ "
"//
+ "Applicable containers: investment
"//
)
@JsonProperty("symbol")
protected String symbol;
@ApiModelProperty(readOnly = true,
value = "The total market value of the security. For insurance container, the field is only applicable for insurance annuity and variable life insurance types."//
+ "
"//
+ "Applicable containers: investment, insurance
"//
)
@JsonProperty("value")
protected Money value;
//
@ApiModelProperty(readOnly = true,
value = "The ISIN (International Securities Identification Number) is used worldwide to identify specific securities. It is equivalent to CUSIP for international markets.
"
+ "
Note: The ISIN field is only applicable to the trade related transactions"//
+ "
"//
+ "Applicable containers: investment
"//
)
@JsonProperty("isin")
protected String isin;
@ApiModelProperty(readOnly = true,
value = "The SEDOL (Stock Exchange Daily Official List) is a set of security identifiers used in the United Kingdom and Ireland for clearing purposes."
+ "
Note: The SEDOL field is only applicable to the trade related transactions"//
+ "
"//
+ "Applicable containers: investment
"//
)
@JsonProperty("sedol")
protected String sedol;
@ApiModelProperty(readOnly = true,
value = "Indicates that the holding is a short trading."//
+ "
"//
+ "Applicable containers: investment
"//
)
@JsonProperty("isShort")
protected Boolean isShort;
@ApiModelProperty(readOnly = true,
value = "Indicates the number of unvested quantity or units."
+ "
Note: The unvested quantity field is only applicable to employee stock options, restricted stock units/awards, performance units, etc."//
+ "
"//
+ "Applicable containers: investment
"//
)
@JsonProperty("unvestedQuantity")
protected Double unvestedQuantity;
@ApiModelProperty(readOnly = true,
value = "Indicates the estimated market value of the unvested units."
+ "
Note: FIs usually calculates the unvested value as the market price unvested quantity. The unvested value field is only applicable to employee stock options, restricted stock units/awards, performance units, etc."//
+ "
"//
+ "Applicable containers: investment
"//
)
@JsonProperty("unvestedValue")
protected Money unvestedValue;
@ApiModelProperty(readOnly = true,
value = "The quantity of units or shares that are already vested on a vest date."
+ "
Note: The vested quantity field is only applicable to employee stock options, restricted stock units/awards, performance units, etc."//
+ "
"//
+ "Applicable containers: investment
"//
)
@JsonProperty("vestedQuantity")
protected Double vestedQuantity;
@ApiModelProperty(readOnly = true,
value = "The number of vested shares that can be exercised by the employee. It is usually equal to the vested quantity."
+ "
Note: The vested shares exercisable field is only applicable to employee stock options, restricted stock units/awards, performance units, etc."//
+ "
"//
+ "Applicable containers: investment
"//
)
@JsonProperty("vestedSharesExercisable")
protected Double vestedSharesExercisable;
@ApiModelProperty(readOnly = true,
value = "Indicates the estimated market value of the vested units."
+ "
Note: FIs usually calculates the vested value as the market price vested quantity. The vested value field is only applicable to employee stock options, restricted stock units/awards, performance units, etc."//
+ "
"//
+ "Applicable containers: investment
"//
)
@JsonProperty("vestedValue")
protected Money vestedValue;
@ApiModelProperty(readOnly = true,
value = "The date on which a RSU, RSA, or an employee stock options become vested."
+ "
Note: The vesting date field is only applicable to employee stock options, restricted stock units/awards, performance units, etc."//
+ "
"//
+ "Applicable containers: investment
"//
)
@JsonProperty("vestingDate")
protected String vestingDate;
@ApiModelProperty(readOnly = true,
value = "The quantity of tradeable units in a contract."
+ "
Note: The contract quantity field is only applicable to commodity and currency."//
+ "
"//
+ "Applicable containers: investment
"//
)
@JsonProperty("contractQuantity")
protected Double contractQuantity;
@ApiModelProperty(readOnly = true,
value = "The stated interest rate for a bond."//
+ "
"//
+ "Applicable containers: investment
"//
)
@JsonProperty("couponRate")
protected Double couponRate;
@ApiModelProperty(readOnly = true,
value = "The quantity of the employee stock options that are already exercised or bought by the employee."
+ "
Note: Once the employee stock options is exercised, they are either converted to cash value or equity positions depending on the FI. The exercised quantity field is only applicable to employee stock options."//
+ "
"//
+ "Applicable containers: investment
"//
)
@JsonProperty("exercisedQuantity")
protected Double exercisedQuantity;
@ApiModelProperty(readOnly = true,
value = "The date on which an option, right or warrant expires."
+ "
Note: The expiration date field is only applicable to options and employee stock options."//
+ "
"//
+ "Applicable containers: investment
"//
)
@JsonProperty("expirationDate")
protected String expirationDate;
@ApiModelProperty(readOnly = true,
value = "The date on which equity awards like ESOP, RSU, etc., are issued or granted."
+ "
Note: The grant date field is only applicable to employee stock options, restricted stock units/awards, performance units, etc."//
+ "
"//
+ "Applicable containers: investment
"//
)
@JsonProperty("grantDate")
protected String grantDate;
@ApiModelProperty(readOnly = true,
value = "The interest rate on a CD."
+ "
Note: The interest rate field is only applicable to CD."//
+ "
"//
+ "Applicable containers: investment
"//
)
@JsonProperty("interestRate")
protected Double interestRate;
@ApiModelProperty(readOnly = true,
value = "The stated maturity date of a bond or CD."//
+ "
"//
+ "Applicable containers: investment
"//
)
@JsonProperty("maturityDate")
protected String maturityDate;
@ApiModelProperty(readOnly = true,
value = "The type of the option position (i.e., put or call)."
+ "
Note: The option type field is only applicable to options."//
+ "
"//
+ "Applicable containers: investment
"//
+ "Applicable Values
"//
)
@JsonProperty("optionType")
protected OptionType optionType;
@ApiModelProperty(readOnly = true,
value = "The difference between the current market value of a stock and the strike price of the employee stock option, when the market value of the shares are greater than the stock price."
+ "
Note: The spread field is only applicable to employee stock options."//
+ "
"//
+ "Applicable containers: investment
"//
)
@JsonProperty("spread")
protected Money spread;
@ApiModelProperty(readOnly = true,
value = "The strike (exercise) price for the option position."
+ "
Note: The strike price field is only applicable to options and employee stock options."//
+ "
"//
+ "Applicable containers: investment
"//
)
@JsonProperty("strikePrice")
protected Money strikePrice;
@ApiModelProperty(readOnly = true,
value = "The fixed duration for which the bond or CD is issued."
+ "
Note: The term field is only applicable to CD."//
+ "
"//
+ "Applicable containers: investment
"//
)
@JsonProperty("term")
protected String term;
@ApiModelProperty(readOnly = true,
value = "The accruedInterest of the holding."//
+ "
"//
+ "Applicable containers: investment
"//
)
@JsonProperty("accruedInterest")
protected Money accruedInterest;
@ApiModelProperty(readOnly = true,
value = "The accruedIncome of the holding."//
+ "
"//
+ "Applicable containers: investment
"//
)
@JsonProperty("accruedIncome")
protected Money accruedIncome;
@ApiModelProperty(readOnly = true,
value = "The date on which the holding is created in the Yodlee system."//
+ "
"//
+ "Applicable containers: investment, insurance
"//
)
@JsonProperty("createdDate")
protected String createdDate;
@ApiModelProperty(readOnly = true,
value = "The date when the information was last updated in the system."//
+ "
"//
+ "Applicable containers: investment, insurance
"//
)
@JsonProperty("lastUpdated")
protected String lastUpdated;
@ApiModelProperty(readOnly = true,
value = "Type of holding"//
+ "
"//
+ "Applicable containers: investment, insurance
"//
)
@JsonProperty("holdingType")
protected HoldingType holdingType;
/**
* The CUSIP (Committee on Uniform Securities Identification Procedures) identifies most the financial instruments
* in the United States and Canada.
*
* Applicable containers: investment
*
* @return cusipNumber
*/
public String getCusipNumber() {
return cusipNumber;
}
/**
* The symbol of the security.
*
* Applicable containers: investment
*
* @return symbol
*/
public String getSymbol() {
return symbol;
}
/**
* The current price of the security.
* Note: Only for bonds the price field indicates the normalized price and not the price aggregated from the
* site. For insurance container, the field is only applicable for insurance annuity and variable life insurance
* types.
*
* Applicable containers: investment, insurance
*
* @return price
*/
public Money getPrice() {
return price;
}
/**
* Indicates the security type of holding identified through the security service.
*
* Applicable containers: investment, insurance
*
* @return securityType
*/
public String getSecurityType() {
return securityType;
}
/**
* Indicates the security style of holding identified through the security service.
*
* Applicable containers: investment, insurance
*
* @return securityStyle
*/
public String getSecurityStyle() {
return securityStyle;
}
/**
* The description (name) for the holding (E.g., Cisco Systems)
* For insurance container, the field is only applicable for insurance annuity and variable life insurance types.
*
*
* Applicable containers: investment, insurance
*
* @return description
*/
public String getDescription() {
return description;
}
/**
* The enrichedDescription is the security description of the normalized holding
*
*
* Applicable containers: investment, insurance
*
* @return enrichedDescription
*/
public String getEnrichedDescription() {
return enrichedDescription;
}
/**
* Indicates the security match status id of the investment option identified during security normalization.
*
* Applicable containers: investment
*
* @return matchStatus
*/
public String getMatchStatus() {
return matchStatus;
}
/**
* The total market value of the security. For insurance container, the field is only applicable for insurance
* annuity and variable life insurance types.
*
* Applicable containers: investment, insurance
*
* @return value
*/
public Money getValue() {
return value;
}
/**
* In a one-off security purchase, the cost basis is the quantity acquired multiplied by the price per unit paid
* plus any commission paid. In case, the same position is acquired in different lots on different days at different
* prices, the sum total of the cost incurred is divided by the total units acquired to arrive at the average cost
* basis.
*
* Applicable containers: investment
*
* @return costBasis
*/
public Money getCostBasis() {
return costBasis;
}
/**
* Unique identifier for the security added in the system. This is the primary key of the holding resource.
*
* Applicable containers: investment, insurance
*
* @return id
*/
public Long getId() {
return id;
}
/**
* Unique identifier of the account to which the security is linked.
*
* Applicable containers: investment, insurance
*
* @return accountId
*/
public Long getAccountId() {
return accountId;
}
/**
* Unique identifier for the user's association with the provider.
*
* Applicable containers: investment, insurance
*
* @return providerAccountId
*/
public Long getProviderAccountId() {
return providerAccountId;
}
/**
* The quantity held for the holding.
* Note: Only for bonds the quantity field indicates the normalized quantity and not the quantity aggregated
* from the site. The quantity field is only applicable to restricted stock units/awards, performance units,
* currency, and commodity.
* For insurance container, the field is only applicable for insurance annuity and variable life insurance types.
*
*
* Applicable containers: investment, insurance
*
* @return quantity
*/
public Double getQuantity() {
return quantity;
}
/**
* The ISIN (International Securities Identification Number) is used worldwide to identify specific securities. It
* is equivalent to CUSIP for international markets.
*
* Note: The ISIN field is only applicable to the trade related transactions
*
* Applicable containers: investment
*
* @return isin
*/
public String getIsin() {
return isin;
}
/**
* The SEDOL (Stock Exchange Daily Official List) is a set of security identifiers used in the United Kingdom and
* Ireland for clearing purposes.
* Note: The SEDOL field is only applicable to the trade related transactions
*
* Applicable containers: investment
*
* @return sedol
*/
public String getSedol() {
return sedol;
}
/**
* Indicates that the holding is a short trading.
*
* Applicable containers: investment
*
* @return isShort
*/
public Boolean getIsShort() {
return isShort;
}
/**
* Indicates the number of unvested quantity or units.
* Note: The unvested quantity field is only applicable to employee stock options, restricted stock
* units/awards, performance units, etc.
*
* Applicable containers: investment
*
* @return unvestedQuantity
*/
public Double getUnvestedQuantity() {
return unvestedQuantity;
}
/**
* Indicates the estimated market value of the unvested units.
* Note: FIs usually calculates the unvested value as the market price unvested quantity. The unvested value
* field is only applicable to employee stock options, restricted stock units/awards, performance units, etc.
*
* Applicable containers: investment
*
* @return unvestedValue
*/
public Money getUnvestedValue() {
return unvestedValue;
}
/**
* The quantity of units or shares that are already vested on a vest date.
* Note: The vested quantity field is only applicable to employee stock options, restricted stock
* units/awards, performance units, etc.
*
* Applicable containers: investment
*
* @return vestedQuantity
*/
public Double getVestedQuantity() {
return vestedQuantity;
}
/**
* The number of vested shares that can be exercised by the employee. It is usually equal to the vested quantity.
*
* Note: The vested shares exercisable field is only applicable to employee stock options, restricted stock
* units/awards, performance units, etc.
*
* Applicable containers: investment
*
* @return vestedSharesExercisable
*/
public Double getVestedSharesExercisable() {
return vestedSharesExercisable;
}
/**
* Indicates the estimated market value of the vested units.
* Note: FIs usually calculates the vested value as the market price vested quantity. The vested value field
* is only applicable to employee stock options, restricted stock units/awards, performance units, etc.
*
* Applicable containers: investment
*
* @return vestedValue
*/
public Money getVestedValue() {
return vestedValue;
}
/**
* The date on which a RSU, RSA, or an employee stock options become vested.
* Note: The vesting date field is only applicable to employee stock options, restricted stock units/awards,
* performance units, etc.
*
* Applicable containers: investment
*
* @return vestingDate
*/
public String getVestingDate() {
return vestingDate;
}
/**
* The quantity of tradeable units in a contract.
* Note: The contract quantity field is only applicable to commodity and currency.
*
* Applicable containers: investment
*
* @return contractQuantity
*/
public Double getContractQuantity() {
return contractQuantity;
}
/**
* The stated interest rate for a bond.
*
* Applicable containers: investment
*
* @return couponRate
*/
public Double getCouponRate() {
return couponRate;
}
/**
* The quantity of the employee stock options that are already exercised or bought by the employee.
* Note: Once the employee stock options is exercised, they are either converted to cash value or equity
* positions depending on the FI. The exercised quantity field is only applicable to employee stock options.
*
* Applicable containers: investment
*
* @return exercisedQuantity
*/
public Double getExercisedQuantity() {
return exercisedQuantity;
}
/**
* The date on which an option, right or warrant expires.
* Note: The expiration date field is only applicable to options and employee stock options.
*
* Applicable containers: investment
*
* @return expirationDate
*/
public String getExpirationDate() {
return expirationDate;
}
/**
* The date on which equity awards like ESOP, RSU, etc., are issued or granted.
* Note: The grant date field is only applicable to employee stock options, restricted stock units/awards,
* performance units, etc.
*
* Applicable containers: investment
*
* @return grantDate
*/
public String getGrantDate() {
return grantDate;
}
/**
* The interest rate on a CD.
* Note: The interest rate field is only applicable to CD.
*
* Applicable containers: investment
*
* @return interestRate
*/
public Double getInterestRate() {
return interestRate;
}
/**
* The stated maturity date of a bond or CD.
*
* Applicable containers: investment
*
* @return maturityDate
*/
public String getMaturityDate() {
return maturityDate;
}
/**
* The type of the option position (i.e., put or call).
* Note: The option type field is only applicable to options.
*
* Applicable containers: investment
* Applicable Values
*
* @return optionType
*/
public OptionType getOptionType() {
return optionType;
}
/**
* The difference between the current market value of a stock and the strike price of the employee stock option,
* when the market value of the shares are greater than the stock price.
* Note: The spread field is only applicable to employee stock options.
*
* Applicable containers: investment
*
* @return spread
*/
public Money getSpread() {
return spread;
}
/**
* The strike (exercise) price for the option position.
* Note: The strike price field is only applicable to options and employee stock options.
*
* Applicable containers: investment
*
* @return strikePrice
*/
public Money getStrikePrice() {
return strikePrice;
}
/**
* The fixed duration for which the bond or CD is issued.
* Note: The term field is only applicable to CD.
*
* Applicable containers: investment
*
* @return term
*/
public String getTerm() {
return term;
}
/**
* The accruedInterest of the holding.
*
* Applicable containers: investment
*
* @return accruedInterest
*/
public Money getAccruedInterest() {
return accruedInterest;
}
/**
* The accruedIncome of the holding.
*
* Applicable containers: investment
*
* @return accruedIncome
*/
public Money getAccruedIncome() {
return accruedIncome;
}
/**
* The date on which the holding is created in the Yodlee system.
*
* Applicable containers: investment, insurance
*
* @return createdDate
*/
public String getCreatedDate() {
return createdDate;
}
/**
* The date when the information was last updated in the system.
*
* Applicable containers: investment, insurance
*
* @return lastUpdated
*/
public String getLastUpdated() {
return lastUpdated;
}
/**
* Type of holding
*
* Applicable containers: investment, insurance
*
* @return holdingType
*/
public HoldingType getHoldingType() {
return holdingType;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy