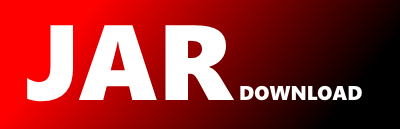
com.yodlee.api.model.provideraccounts.AbstractProviderAccount Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yodlee-api-model-beta Show documentation
Show all versions of yodlee-api-model-beta Show documentation
Yodlee API Model Beta is the stage version
/**
* Copyright (c) 2019 Yodlee, Inc. All Rights Reserved.
*
* Licensed under the MIT License. See LICENSE file in the project root for license information.
*/
package com.yodlee.api.model.provideraccounts;
import java.util.Collections;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.yodlee.api.model.AbstractModelComponent;
import com.yodlee.api.model.AccountDataset;
import com.yodlee.api.model.enums.AggregationSource;
import com.yodlee.api.model.enums.OpenBankingMigrationStatusType;
import com.yodlee.api.model.provideraccounts.enums.ProviderAccountStatus;
import io.swagger.annotations.ApiModelProperty;
@JsonInclude(JsonInclude.Include.NON_NULL)
public abstract class AbstractProviderAccount extends AbstractModelComponent {
@ApiModelProperty(readOnly = true,
value = "Unique identifier for the provider account resource. This is created during account addition."//
+ "
"//
+ "Endpoints:"//
+ ""//
+ "- GET providerAccounts
"//
+ "- POST providerAccounts
"//
+ "- PUT providerAccounts/{providerAccountId}
"//
+ "- GET providerAccounts/{providerAccountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("id")
protected Long id;
@ApiModelProperty(readOnly = true,
value = "The source through which the providerAccount is added in the system."//
+ "
"//
+ "Endpoints:"//
+ ""//
+ "- GET providerAccounts
"//
+ "- POST providerAccounts
"//
+ "- PUT providerAccounts/{providerAccountId}
"//
+ "- GET providerAccounts/{providerAccountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
"//
+ "Applicable Values
"//
)
@JsonProperty("aggregationSource")
protected AggregationSource aggregationSource;
@ApiModelProperty(readOnly = true,
value = "Unique identifier for the provider resource. This denotes the provider for which the provider account id is generated by the user."//
+ "
"//
+ "Endpoints:"//
+ ""//
+ "- GET providerAccounts
"//
+ "- POST providerAccounts
"//
+ "- PUT providerAccounts/{providerAccountId}
"//
+ "- GET providerAccounts/{providerAccountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("providerId")
protected Long providerId;
@ApiModelProperty(readOnly = true,
value = "Indicates whether account is a manual or aggregated provider account."//
+ "
"//
+ "Endpoints:"//
+ ""//
+ "- GET providerAccounts
"//
+ "- POST providerAccounts
"//
+ "- PUT providerAccounts/{providerAccountId}
"//
+ "- GET providerAccounts/{providerAccountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("isManual")
protected Boolean isManual;
@ApiModelProperty(readOnly = true,
value = "Unique id generated to indicate the request."//
+ "
"//
+ "Endpoints:"//
+ ""//
+ "- GET providerAccounts
"//
+ "- POST providerAccounts
"//
+ "- PUT providerAccounts/{providerAccountId}
"//
+ "- GET providerAccounts/{providerAccountId}
"//
+ "
")
@JsonProperty("requestId")
protected String requestId;
@ApiModelProperty(readOnly = true,
value = "The status of last update attempted for the account. "//
+ "
"//
+ "Endpoints:"//
+ ""//
+ "- GET providerAccounts
"//
+ "- POST providerAccounts
"//
+ "- PUT providerAccounts/{providerAccountId}
"//
+ "- GET providerAccounts/{providerAccountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
"//
+ "Applicable Values
"//
)
@JsonProperty("status")
protected ProviderAccountStatus status;
@ApiModelProperty(readOnly = true,
value = "Logical grouping of dataset attributes into datasets such as Basic Aggregation Data, Account Profile and Documents."//
+ "
"//
+ "Endpoints:"//
+ ""//
+ "- GET providerAccounts
"//
+ "- POST providerAccounts
"//
+ "- PUT providerAccounts/{providerAccountId}
"//
+ "- GET providerAccounts/{providerAccountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("dataset")
protected List datasets;
@ApiModelProperty(readOnly = true,
value = "Indicate when the providerAccount is last updated successfully." + "
"//
+ "Account Type: Aggregated
"//
+ "Endpoints:"//
+ ""//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("lastUpdated")
protected String lastUpdated;
/**
* The source through which the providerAccount is added in the system.
*
* Endpoints:
*
* - GET providerAccounts
* - POST providerAccounts
* - PUT providerAccounts/{providerAccountId}
* - GET providerAccounts/{providerAccountId}
*
* Applicable Values
*
* @return aggregationSource
*/
public AggregationSource getAggregationSource() {
return aggregationSource;
}
/**
* Logical grouping of dataset attributes into datasets such as Basic Aggregation Data, Account Profile and
* Documents.
*
* Endpoints:
*
* - GET providerAccounts
* - POST providerAccounts
* - PUT providerAccounts/{providerAccountId}
* - GET providerAccounts/{providerAccountId}
*
*
* @return dataset
*/
@JsonProperty("dataset")
public List getDataset() {
return datasets == null ? null : Collections.unmodifiableList(datasets);
}
/**
* Unique identifier for the provider account resource. This is created during account addition.
*
* Endpoints:
*
* - GET providerAccounts
* - POST providerAccounts
* - PUT providerAccounts/{providerAccountId}
* - GET providerAccounts/{providerAccountId}
*
*
* @return id
*/
public Long getId() {
return id;
}
/**
* Unique identifier for the provider resource. This denotes the provider for which the provider account id is
* generated by the user.
*
* Endpoints:
*
* - GET providerAccounts
* - POST providerAccounts
* - PUT providerAccounts/{providerAccountId}
* - GET providerAccounts/{providerAccountId}
*
*
* @return providerId
*/
public Long getProviderId() {
return providerId;
}
/**
* Indicates whether account is a manual or aggregated provider account.
*
* Endpoints:
*
* - GET providerAccounts
* - POST providerAccounts
* - PUT providerAccounts/{providerAccountId}
* - GET providerAccounts/{providerAccountId}
*
*
* @return isManual
*/
public Boolean getIsManual() {
return isManual;
}
/**
* Unique id generated to indicate the request.
*
* Endpoints:
*
* - GET providerAccounts
* - POST providerAccounts
* - PUT providerAccounts/{providerAccountId}
* - GET providerAccounts/{providerAccountId}
*
*
* @return requestId
*/
public String getRequestId() {
return requestId;
}
/**
* The status of last update attempted for the account.
*
* Endpoints:
*
* - GET providerAccounts
* - POST providerAccounts
* - PUT providerAccounts/{providerAccountId}
* - GET providerAccounts/{providerAccountId}
*
* Applicable Values
*
* @return status
*/
public ProviderAccountStatus getStatus() {
return status;
}
/**
* Indicate when the providerAccount is last updated successfully.
*
* Account Type: Aggregated
* Endpoints:
*
* - GET dataExtracts/userData
*
*
* @return lastUpdated
*/
public String getLastUpdated() {
return lastUpdated;
}
@ApiModelProperty(readOnly = true,
value = "Indicates the migration status of the provider account from screen-scraping provider to the Open Banking provider. "//
+ "
"//
+ "Endpoints:"//
+ ""//
+ "- GET providerAccounts
"//
+ "- GET providerAccounts/{providerAccountId}
"
+ "- PUT providerAccounts/{providerAccountId}
"
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("oauthMigrationStatus")
protected OpenBankingMigrationStatusType openBankingMigrationStatusType;
/**
* Indicates the migration status of the provider account from screen-scraping provider to the Open Banking provider.
*
* Endpoints:
*
* - GET providerAccounts
* - GET providerAccounts/{providerAccountId}
* - PUT providerAccounts/{providerAccountId}
* - GET dataExtracts/userData
*
*
* @return OpenBankingMigrationStatusType
*/
@JsonProperty("oauthMigrationStatus")
public OpenBankingMigrationStatusType getOpenBankingMigrationStatusType() {
return openBankingMigrationStatusType;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy