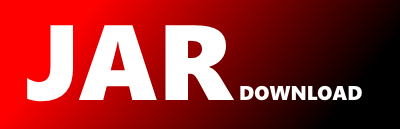
com.yodlee.api.model.provideraccounts.DataSetInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yodlee-api-model-beta Show documentation
Show all versions of yodlee-api-model-beta Show documentation
Yodlee API Model Beta is the stage version
/**
* Copyright (c) 2019 Yodlee, Inc. All Rights Reserved.
*
* Licensed under the MIT License. See LICENSE file in the project root for license information.
*/
package com.yodlee.api.model.provideraccounts;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.yodlee.api.model.AbstractModelComponent;
import com.yodlee.api.model.enums.AdditionalStatusType;
import com.yodlee.api.model.enums.DatasetNameType;
import com.yodlee.api.model.enums.EligibilityStatus;
import io.swagger.annotations.ApiModelProperty;
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({"name", "additionalStatus", "updateEligibility", "lastUpdated", "lastUpdateAttempt", "attribute"})
public class DataSetInfo extends AbstractModelComponent {
@ApiModelProperty(value = "The name of the dataset requested from the provider site" + "
"//
+ "Account Type: Manual
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET providers/{providerId}
"//
+ "- GET providers
"//
+ "
"//
+ "Applicable Values
"//
)
@JsonProperty("name")
private DatasetNameType name;
@ApiModelProperty(value = "The status of last update attempted for the dataset. " + "
"//
+ "Account Type: Aggregated
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "
"//
+ "Applicable Values
"//
)
@JsonProperty("additionalStatus")
private AdditionalStatusType additionalStatus;
@ApiModelProperty(value = "Indicate whether the dataset is eligible for update or not." + "
"//
+ "Account Type: Aggregated
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "
"//
+ "Applicable Values
"//
)
@JsonProperty("updateEligibility")
private EligibilityStatus updateEligibility;
@ApiModelProperty(value = "Indicate when the dataset is last updated successfully for the given provider account."
+ "
"//
+ "Account Type: Aggregated
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "
")
@JsonProperty("lastUpdated")
private String lastUpdated;
@ApiModelProperty(value = "Indicate when the last attempt was performed to update the dataset for the given provider account"
+ "
"//
+ "Account Type: Aggregated
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "
")
@JsonProperty("lastUpdateAttempt")
private String lastUpdateAttempt;
@ApiModelProperty(value = "Indicate when the next attempt is scheduled to update the dataset for the given provider account"
+ "
"//
+ "Account Type: Aggregated
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "
")
@JsonProperty("nextUpdateScheduled")
private String nextUpdateScheduled;
/**
* Indicate when the last attempt was performed to update the dataset for the given provider account
*
* Account Type: Aggregated
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return lastUpdateAttempt
*/
public String getLastUpdateAttempt() {
return lastUpdateAttempt;
}
public void setLastUpdateAttempt(String lastUpdateAttempt) {
this.lastUpdateAttempt = lastUpdateAttempt;
}
/**
* Indicate whether the dataset is eligible for update or not. *
*
* Account Type: Aggregated
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
* Applicable Values
*
* @return updateEligibility
*/
public EligibilityStatus getUpdateEligibility() {
return updateEligibility;
}
public void setUpdateEligibility(EligibilityStatus updateEligibility) {
this.updateEligibility = updateEligibility;
}
/**
* The name of the dataset requested from the provider site *
*
* Account Type: Manual
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
* - GET providers/{providerId}
* - GET providers
*
* Applicable Values
*
* @return name
*/
public DatasetNameType getName() {
return name;
}
public void setName(DatasetNameType name) {
this.name = name;
}
/**
* The status of last update attempted for the dataset. *
*
* Account Type: Aggregated
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
* Applicable Values
*
* @return additionalStatus
*/
public AdditionalStatusType getAdditionalStatus() {
return additionalStatus;
}
public void setAdditionalStatus(AdditionalStatusType additionalStatus) {
this.additionalStatus = additionalStatus;
}
@Override
public String toString() {
return "DataSetInfo [name=" + name + ", additionalStatus=" + additionalStatus + ", updateEligibility="
+ updateEligibility + ", lastUpdated=" + lastUpdated + ", lastUpdateAttempt=" + lastUpdateAttempt
+ ", nextUpdateScheduled=" + nextUpdateScheduled + "]";
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy