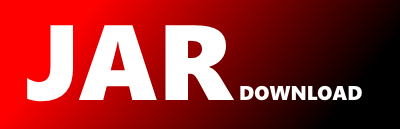
com.yodlee.api.model.transaction.Merchant Maven / Gradle / Ivy
/**
* Copyright (c) 2019 Yodlee, Inc. All Rights Reserved.
*
* Licensed under the MIT License. See LICENSE file in the project root for license information.
*/
package com.yodlee.api.model.transaction;
import java.util.Collections;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.yodlee.api.model.AbstractModelComponent;
import com.yodlee.api.model.account.AccountAddress;
import com.yodlee.api.model.transaction.enums.TransactionMerchantSource;
import io.swagger.annotations.ApiModelProperty;
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({"id", "source", "address", "categoryLabel", "name", "coordinates", "contact", "website","logoURL"})
public class Merchant extends AbstractModelComponent {
@ApiModelProperty(readOnly = true,
value = "Identifier of the merchant."//
+ "
"//
+ "Applicable containers: bank,creditCard,investment,insurance,loan
"//
)
@JsonProperty("id")
private String id;
@ApiModelProperty(readOnly = true,
value = "The source through which merchant information is retrieved."//
+ "
"//
+ "Applicable containers: bank,creditCard,investment,insurance,loan
"//
+ "Applicable Values
"//
)
@JsonProperty("source")
private TransactionMerchantSource source;
@ApiModelProperty(readOnly = true,
value = "The address of the merchant associated with the transaction is populated in the merchant address field."
+ "
Note: The merchant address field is not available by default and customers will have to specifically request the merchant's address (that includes city, state, and ZIP of the merchant). The merchant address field is available only for merchants in the United States."//
+ "
"//
+ "Applicable containers: bank,creditCard
"//
)
@JsonProperty("address")
private AccountAddress address;
@ApiModelProperty(readOnly = true,
value = "The business categories of the merchant."//
+ "
"//
+ "Applicable containers: bank,creditCard
"//
+ "Applicable Values
"//
)
@JsonProperty("categoryLabel")
private List categoryLabel;
@ApiModelProperty(readOnly = true,
value = "The name of the merchant."//
+ "
"//
+ "Applicable containers: bank,creditCard,investment,insurance,loan
"//
)
@JsonProperty("name")
private String name;
@ApiModelProperty(value = "The merchant geolocation coordinates like latitude and longitude."//
+ "
"//
+ "Applicable containers: bank,creditCard,loan
"//
)
@JsonProperty("coordinates")
private Coordinates coordinates;
@ApiModelProperty(value = "The merchant contact information like phone and email."//
+ "
"//
+ "Applicable containers: bank,creditCard,investment,loan
"//
)
@JsonProperty("contact")
private Contact contact;
@ApiModelProperty(readOnly = true,
value = "The website of the merchant."//
+ "
"//
+ "Applicable containers: bank,creditCard,investment,loan
"//
)
@JsonProperty("website")
private String website;
@ApiModelProperty(readOnly = true,
value = "The logoURL of the merchant."//
+ "
"//
+ "Applicable containers: bank,creditCard,investment,loan
"//
)
@JsonProperty("logoURL")
private String logoURL;
/**
* Identifier of the merchant.
*
* Applicable containers: bank,creditCard,investment,insurance,loan
*
* @return id
*/
public String getId() {
return id;
}
/**
* The source through which merchant information is retrieved.
*
* Applicable containers: bank,creditCard,investment,insurance,loan
* Applicable Values
*
* @return source
*/
public TransactionMerchantSource getSource() {
return source;
}
/**
* The address of the merchant associated with the transaction is populated in the merchant address field.
* Note: The merchant address field is not available by default and customers will have to specifically
* request the merchant's address (that includes city, state, and ZIP of the merchant). The merchant address field
* is available only for merchants in the United States.
*
* Applicable containers: bank,creditCard
*
* @return address
*/
public AccountAddress getAddress() {
return address;
}
/**
* The business categories of the merchant.
*
* Applicable containers: bank,creditCard
* Applicable Values
*
* @return categoryLabel
*/
@JsonProperty("categoryLabel")
public List getCategoryLabel() {
return categoryLabel == null ? null : Collections.unmodifiableList(categoryLabel);
}
/**
* The name of the merchant.
*
* Applicable containers: bank,creditCard,investment,insurance,loan
*
* @return name
*/
public String getName() {
return name;
}
/**
* The Coordinates of merchant which includes merchant longitude and latitude information
*
* Applicable containers: bank,creditCard,investment,loan
* Applicable Values
*
* @return coordinates
*/
public Coordinates getCoordinates() {
return coordinates;
}
/**
* The Contact information of merchant which includes phone number and email id information
*
* Applicable containers: bank,creditCard,investment,loan
* Applicable Values
*
* @return contact
*/
public Contact getContact() {
return contact;
}
/**
* The website of the merchant
*
* Applicable containers: bank,creditCard,investment,loan
* Applicable Values
*
* @return website
*/
public String getWebsite() {
return website;
}
/**
* The logoURL of the merchant
*
* Applicable containers: bank,creditCard,investment,loan
* Applicable Values
*
* @return logoURL
*/
public String getLogoURL() {
return logoURL;
}
@Override
public String toString() {
return "Merchant [id=" + id + ", source=" + source + ", address=" + address + ", categoryLabel=" + categoryLabel
+ ", name=" + name + ", coordinates=" + coordinates + ", contact=" + contact + ", website=" + website +",logoURL="+logoURL
+ "]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy