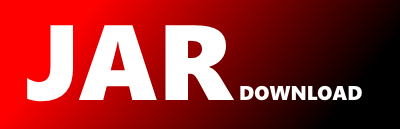
com.yodlee.api.model.transaction.TransactionCategory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yodlee-api-model-beta Show documentation
Show all versions of yodlee-api-model-beta Show documentation
Yodlee API Model Beta is the stage version
/**
* Copyright (c) 2019 Yodlee, Inc. All Rights Reserved.
*
* Licensed under the MIT License. See LICENSE file in the project root for license information.
*/
package com.yodlee.api.model.transaction;
import java.util.Collections;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.yodlee.api.model.AbstractModelComponent;
import com.yodlee.api.model.transaction.enums.TransactionCategoryClassification;
import com.yodlee.api.model.transaction.enums.TransactionCategorySource;
import com.yodlee.api.model.transaction.enums.TransactionCategoryType;
import io.swagger.annotations.ApiModelProperty;
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({"id", "highLevelCategoryName", "category", "source", "highLevelCategoryId", "type",
"detailCategory", "defaultCategoryName", "defaultHighLevelCategoryName"})
public class TransactionCategory extends AbstractModelComponent {
@ApiModelProperty(readOnly = true,
value = "Unique identifier of the category."//
+ "
"//
+ "Applicable containers: creditCard, investment, insurance, loan
"//
)
@JsonProperty("id")
private Long id;
@ApiModelProperty(readOnly = true,
value = "The name of the high level category. A group of similar transaction categories are clubbed together to form a high-level category."//
+ "
"//
+ "Applicable containers: creditCard, investment, insurance, loan
"//
)
@JsonProperty("highLevelCategoryName")
private String highLevelCategoryName;
@ApiModelProperty(readOnly = true,
value = "The name of the category."
+ "
Note: Transaction categorization is one of the core features offered by Yodlee and the categories are assigned to the transactions by the system. Transactions can be clubbed together by the category that is assigned to them. "//
+ "
"//
+ "Applicable containers: creditCard, investment, insurance, loan
"//
)
@JsonProperty("category")
private String category;
@ApiModelProperty(readOnly = true,
value = "Source used to identify whether the transaction category is user defined category or system created category."//
+ "
"//
+ "Applicable containers: creditCard, investment, insurance, loan
"//
+ "Applicable Values
"//
)
@JsonProperty("source")
private TransactionCategorySource source;
@ApiModelProperty(readOnly = true,
value = "The unique identifier of the high level category."//
+ "
"//
+ "Applicable containers: creditCard, investment, insurance, loan
"//
)
@JsonProperty("highLevelCategoryId")
private Long highLevelCategoryId;
@ApiModelProperty(readOnly = true,
value = "Transaction categories and high-level categories are further mapped to five transaction category types. Customers, based on their needs can either use the transaction categories, the high-level categories, or the transaction category types. "//
+ "
"//
+ "Applicable containers: creditCard, investment, insurance, loan
"//
+ "Applicable Values
"//
)
@JsonProperty("type")
private TransactionCategoryType type;
@ApiModelProperty(readOnly = true,
value = "Entity that provides detail category attributes"//
+ "
"//
+ "Applicable containers: creditCard, investment, insurance, loan
"//
)
@JsonProperty("detailCategory")
private List detailCategory;
@ApiModelProperty(readOnly = true,
value = "Category Classification."//
+ "
"//
+ "Applicable containers: creditCard, investment, insurance, loan
"//
+ "Applicable Values
"//
)
@JsonProperty("classification")
private TransactionCategoryClassification classification;
@ApiModelProperty(readOnly = true,
value = "A attribute which will always hold the first value(initial name) of Yodlee defined category attribute."//
+ "
"//
+ "Applicable containers: creditCard, investment, insurance, loan
"//
)
@JsonProperty("defaultCategoryName")
private String defaultCategoryName;
@ApiModelProperty(readOnly = true,
value = "A attribute which will always hold the first value(initial name) of Yodlee defined highLevelCategoryName attribute."//
+ "
"//
+ "Applicable containers: creditCard, investment, insurance, loan
"//
)
@JsonProperty("defaultHighLevelCategoryName")
private String defaultHighLevelCategoryName;
/**
* Unique identifier of the category.
*
* Applicable containers: creditCard, investment, insurance, loan
*
* @return id
*/
public Long getId() {
return id;
}
/**
* The name of the high level category. A group of similar transaction categories are clubbed together to form a
* high-level category.
*
* Applicable containers: creditCard, investment, insurance, loan
*
* @return highLevelCategoryName
*/
public String getHighLevelCategoryName() {
return highLevelCategoryName;
}
/**
* The name of the category.
* Note: Transaction categorization is one of the core features offered by Yodlee and the categories are
* assigned to the transactions by the system. Transactions can be clubbed together by the category that is assigned
* to them.
*
* Applicable containers: creditCard, investment, insurance, loan
*
* @return category
*/
public String getCategory() {
return category;
}
/**
* Source used to identify whether the transaction category is user defined category or system created category.
*
*
* Applicable containers: creditCard, investment, insurance, loan
* Applicable Values
*
* @return source
*/
public TransactionCategorySource getSource() {
return source;
}
/**
* The unique identifier of the high level category.
*
* Applicable containers: creditCard, investment, insurance, loan
*
* @return highLevelCategoryId
*/
public Long getHighLevelCategoryId() {
return highLevelCategoryId;
}
/**
* Transaction categories and high-level categories are further mapped to five transaction category types.
* Customers, based on their needs can either use the transaction categories, the high-level categories, or the
* transaction category types.
*
* Applicable containers: creditCard, investment, insurance, loan
* Applicable Values
*
* @return type
*/
public TransactionCategoryType getType() {
return type;
}
/**
* Entity that provides detail category attributes
*
* Applicable containers: creditCard, investment, insurance, loan
*
* @return detailCategory
*/
@JsonProperty("detailCategory")
public List getDetailCategories() {
return detailCategory == null ? null : Collections.unmodifiableList(detailCategory);
}
/**
* Category Classification.
*
* Applicable containers: creditCard, investment, insurance, loan
* Applicable Values
*
* @return classification
*/
public TransactionCategoryClassification getClassification() {
return classification;
}
/**
* A attribute which will always hold the first value(initial name) of Yodlee defined category attribute.
*
* Applicable containers: creditCard, investment, insurance, loan
*
* @return defaultCategoryName
*/
public String getDefaultCategoryName() {
return defaultCategoryName;
}
/**
* A attribute which will always hold the first value(initial name) of Yodlee defined highLevelCategoryName
* attribute.
*
* Applicable containers: creditCard, investment, insurance, loan
*
* @return defaultHighLevelCategoryName
*/
public String getDefaultHighLevelCategoryName() {
return defaultHighLevelCategoryName;
}
@Override
public String toString() {
return "TransactionCategory [id=" + id + ", highLevelCategoryName=" + highLevelCategoryName + ", category="
+ category + ", source=" + source + ", highLevelCategoryId=" + highLevelCategoryId + ", type=" + type
+ ", detailCategory=" + detailCategory + ", classification=" + classification + ", defaultCategoryName="
+ defaultCategoryName + ", defaultHighLevelCategoryName=" + defaultHighLevelCategoryName + "]";
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy