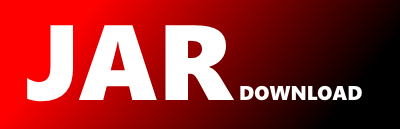
com.yodlee.api.model.AccountDataset Maven / Gradle / Ivy
/**
* Copyright (c) 2019 Yodlee, Inc. All Rights Reserved.
*
* Licensed under the MIT License. See LICENSE file in the project root for license information.
*/
package com.yodlee.api.model;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.yodlee.api.model.enums.AdditionalStatusType;
import com.yodlee.api.model.enums.EligibilityStatus;
import io.swagger.annotations.ApiModelProperty;
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({"additionalStatus", "updateEligibility", "lastUpdated", "nextUpdateScheduled"})
public class AccountDataset extends AbstractDataset {
@ApiModelProperty(readOnly = true,
value = "The status of last update attempted for the dataset. "//
+ "
"//
+ "Account Type: Aggregated
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET providerAccounts
"//
+ "- POST providerAccounts
"//
+ "- PUT providerAccounts/{providerAccountId}
"//
+ "- GET providerAccounts/{providerAccountId}
"//
+ "
"//
+ "Applicable Values
"//
)
@JsonProperty("additionalStatus")
private AdditionalStatusType additionalStatus;
@ApiModelProperty(readOnly = true,
value = "Indicate whether the dataset is eligible for update or not." + "
"//
+ "Account Type: Aggregated
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET providerAccounts
"//
+ "- POST providerAccounts
"//
+ "- PUT providerAccounts/{providerAccountId}
"//
+ "- GET providerAccounts/{providerAccountId}
"//
+ "
"//
+ "Applicable Values
"//
)
@JsonProperty("updateEligibility")
private EligibilityStatus updateEligibility;
@ApiModelProperty(readOnly = true,
value = "Indicate when the dataset is last updated successfully for the given provider account."
+ "
"//
+ "Account Type: Aggregated
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET providerAccounts
"//
+ "- POST providerAccounts
"//
+ "- PUT providerAccounts/{providerAccountId}
"//
+ "- GET providerAccounts/{providerAccountId}
"//
+ "
")
@JsonProperty("lastUpdated")
private String lastUpdated;
@ApiModelProperty(readOnly = true,
value = "Indicate when the last attempt was performed to update the dataset for the given provider account"
+ "
"//
+ "Account Type: Aggregated
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET providerAccounts
"//
+ "- POST providerAccounts
"//
+ "- PUT providerAccounts/{providerAccountId}
"//
+ "- GET providerAccounts/{providerAccountId}
"//
+ "
")
@JsonProperty("lastUpdateAttempt")
private String lastUpdateAttempt;
@ApiModelProperty(readOnly = true,
value = "Indicates when the next attempt to update the dataset is scheduled."//
+ "
"//
+ "Account Type: Aggregated
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET providerAccounts
"//
+ "- POST providerAccounts
"//
+ "- PUT providerAccounts/{providerAccountId}
"//
+ "- GET providerAccounts/{providerAccountId}
"//
+ "
")
@JsonProperty("nextUpdateScheduled")
private String nextUpdateScheduled;
/**
* The status of last update attempted for the dataset.
*
* Account Type: Aggregated
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
* - GET providerAccounts
* - POST providerAccounts
* - PUT providerAccounts/{providerAccountId}
* - GET providerAccounts/{providerAccountId}
*
* Applicable Values
*
* @return additional status
*/
public AdditionalStatusType getAdditionalStatus() {
return additionalStatus;
}
/**
* Indicate whether the dataset is eligible for update or not. *
*
* Account Type: Aggregated
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
* - GET providerAccounts
* - POST providerAccounts
* - PUT providerAccounts/{providerAccountId}
* - GET providerAccounts/{providerAccountId}
*
* Applicable Values
*
* @return eligibility status
*/
public EligibilityStatus getUpdateEligibility() {
return updateEligibility;
}
/**
* Indicate when the dataset is last updated successfully for the given provider account.
*
* Account Type: Aggregated
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
* - GET providerAccounts
* - POST providerAccounts
* - PUT providerAccounts/{providerAccountId}
* - GET providerAccounts/{providerAccountId}
*
*
* @return lastUpdated
*/
public String getLastUpdated() {
return lastUpdated;
}
/**
* Indicate when the last attempt was performed to update the dataset for the given provider account
*
* Account Type: Aggregated
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
* - GET providerAccounts
* - POST providerAccounts
* - PUT providerAccounts/{providerAccountId}
* - GET providerAccounts/{providerAccountId}
*
*
* @return lastUpdateAttempt
*/
public String getLastUpdateAttempt() {
return lastUpdateAttempt;
}
/**
* Indicates when the next attempt to update the dataset is scheduled.
*
* Account Type: Aggregated
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
* - GET providerAccounts
* - POST providerAccounts
* - PUT providerAccounts/{providerAccountId}
* - GET providerAccounts/{providerAccountId}
*
*
* @return nextUpdateScheduled
*/
public String getNextUpdateScheduled() {
return nextUpdateScheduled;
}
@Override
public String toString() {
return "AccountDataset [additionalStatus=" + additionalStatus + ", updateEligibility=" + updateEligibility
+ ", lastUpdated=" + lastUpdated + ", lastUpdateAttempt=" + lastUpdateAttempt + ", nextUpdateScheduled="
+ nextUpdateScheduled + "]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy