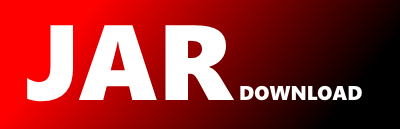
com.yodlee.api.model.account.AbstractAccount Maven / Gradle / Ivy
/**
* Copyright (c) 2019 Yodlee, Inc. All Rights Reserved.
*
* Licensed under the MIT License. See LICENSE file in the project root for license information.
*/
package com.yodlee.api.model.account;
import java.util.Collections;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.yodlee.api.model.AbstractModelComponent;
import com.yodlee.api.model.AccountDataset;
import com.yodlee.api.model.Money;
import com.yodlee.api.model.account.enums.AccountClassification;
import com.yodlee.api.model.account.enums.FrequencyType;
import com.yodlee.api.model.account.enums.HomeInsuranceType;
import com.yodlee.api.model.account.enums.ItemAccountStatus;
import com.yodlee.api.model.account.enums.LifeInsuranceType;
import com.yodlee.api.model.account.enums.LoanInterestRateType;
import com.yodlee.api.model.account.enums.LoanRepaymentPlanType;
import com.yodlee.api.model.account.enums.PolicyStatus;
import com.yodlee.api.model.account.enums.SourceAccountStatus;
import com.yodlee.api.model.account.enums.UserClassification;
import com.yodlee.api.model.account.enums.ValuationType;
import com.yodlee.api.model.enums.AggregationSource;
import com.yodlee.api.model.enums.Container;
import com.yodlee.api.model.enums.OpenBankingMigrationStatusType;
import io.swagger.annotations.ApiModelProperty;
@JsonInclude(JsonInclude.Include.NON_NULL)
public abstract class AbstractAccount extends AbstractModelComponent {
@ApiModelProperty(readOnly = true,
value = "The type of service. E.g., Bank, Credit Card, Investment, Insurance, etc.
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: All containers
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
"//
+ "Applicable Values
"//
)
@JsonProperty("CONTAINER")
protected Container container;
@ApiModelProperty(readOnly = true,
value = "The primary key of the provider account resource."//
+ "
"//
+ "Aggregated / Manual: Both
"//
+ "Applicable containers: All containers
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("providerAccountId")
protected Long providerAccountId;
@ApiModelProperty(readOnly = true,
value = "List of Loan accountId(s) to which the real-estate account is linked"//
+ "
"//
+ "Aggregated / Manual: Both
"//
+ "Applicable containers: realEstate
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("linkedAccountIds")
protected List linkedAccountIds;
@ApiModelProperty(readOnly = true,
value = "The account name as it appears at the site.
"//
+ "(The POST accounts service response return this field as name)
"//
+ "Applicable containers: All Containers
"//
+ "Aggregated / Manual: Both
"//
+ "Endpoints:
"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("accountName")
protected String accountName;
@ApiModelProperty(readOnly = true,
value = "The status of the account that is updated by the consumer through an application or an API. "
+ "Valid Values: AccountStatus" //
+ "
Additional Details:"
+ "
ACTIVE: All the added manual and aggregated accounts status will be made \"ACTIVE\" by default. "
+ "
TO_BE_CLOSED: If the aggregated accounts are not found or closed in the data provider site, Yodlee system marks the status as TO_BE_CLOSED"
+ "
INACTIVE: Users can update the status as INACTIVE to stop updating and to stop considering the account in other services"
+ "
CLOSED: Users can update the status as CLOSED, if the account is closed with the provider. "
+ "
"//
+ "Aggregated / Manual: Both
"//
+ "Applicable containers: All containers
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
"//
+ "Applicable Values
"//
)
@JsonProperty("accountStatus")
protected ItemAccountStatus accountStatus;
@ApiModelProperty(readOnly = true,
value = "The account number as it appears on the site. (The POST accounts service response return this field as number)
"
+ "Additional Details: Bank/ Loan/ Insurance/ Investment:
"
+ " The account number for the bank account as it appears at the site.
"
+ "Credit Card: The account number of the card account as it appears at the site,
"
+ "i.e., the card number.The account number can be full or partial based on how it is displayed in the account summary page of the site."
+ "In most cases, the site does not display the full account number in the account summary page "
+ "and additional navigation is required to aggregate it.
"//
+ "Applicable containers: All Containers
"//
+ "Aggregated / Manual: Both
"//
+ "Endpoints:
"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- POST accounts
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("accountNumber")
protected String accountNumber;
@ApiModelProperty(readOnly = true,
value = "The source through which the account(s) are added in the system.
"
+ "Valid Values: SYSTEM, USER
"//
+ "Applicable containers: All Containers
"//
+ "Aggregated / Manual: Both
"//
+ "Endpoints:
"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
"//
+ "Applicable Values
"//
)
@JsonProperty("aggregationSource")
protected AggregationSource aggregationSource;
@ApiModelProperty(readOnly = true,
value = "The account to be considered as an asset or liability.
"//
+ "Applicable containers: All Containers
"//
+ "Aggregated / Manual: Both
"//
+ "Endpoints:
"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("isAsset")
protected Boolean isAsset;
@ApiModelProperty(readOnly = true,
value = "The total account value. " + "
Additional Details:"
+ "
Bank: available balance or current balance."
+ "
Credit Card: running Balance."
+ "
Investment: The total balance of all the investment account, as it appears on the FI site."
+ "
Insurance: CashValue or amountDue" + "
Loan: principalBalance
"
+ "Applicable containers: bank, creditCard, investment, insurance, loan, otherAssets, otherLiabilities, realEstate
"//
+ "Aggregated / Manual: Both
"//
+ "Endpoints:
"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("balance")
protected Money balance;
@ApiModelProperty(readOnly = true,
value = "The primary key of the account resource and the unique identifier for the account.
"//
+ "Aggregated / Manual: Both
"//
+ "Applicable containers: All containers
"//
+ "Endpoints:"//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET investmentOptions
"//
+ "- GET accounts/historicalBalances
"//
+ "- POST accounts
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("id")
protected Long id;
@ApiModelProperty(readOnly = true,
value = ""//
+ "Applicable containers: reward, bank, creditCard, investment, loan, insurance, realEstate, otherLiabilities
"//
+ "Endpoints:"//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- POST accounts
"//
+ "GET dataExtracts/userData "//
+ "Applicable Values
"//
)
@JsonProperty("userClassification")
protected UserClassification userClassification;
@ApiModelProperty(readOnly = true,
value = "Used to determine whether an account to be considered in the networth calculation."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: bank,creditCard,loan,investment,insurance,realEstate,otherAssets,otherLiabilities
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("includeInNetWorth")
protected Boolean includeInNetWorth;
@ApiModelProperty(readOnly = true,
value = "Identifier of the provider site. The primary key of provider resource. "//
+ "
"//
+ "Aggregated / Manual: Both
"//
+ "Applicable containers: All containers
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("providerId")
protected String providerId;
@ApiModelProperty(readOnly = true,
value = "Service provider or institution name where the account originates. This belongs to the provider resource."//
+ "
"//
+ "Aggregated / Manual: Both
"//
+ "Applicable containers: All containers
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("providerName")
protected String providerName;
@ApiModelProperty(readOnly = true,
value = "Indicates if an account is aggregated from a site or it is a "
+ "manual account i.e. account information manually provided by the user.
"//
+ "Aggregated / Manual: Both
"//
+ "Applicable containers: All containers
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("isManual")
protected Boolean isManual;
@ApiModelProperty(readOnly = true,
value = "The balance in the account that is available for spending. "
+ "For checking accounts with overdraft, available balance may include "
+ "overdraft amount, if end site adds overdraft balance to available balance.
"//
+ "Applicable containers: bank, investment
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Endpoints:
"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("availableBalance")
protected Money availableBalance;
@ApiModelProperty(readOnly = true,
value = "The balance in the account that is available at the beginning of the "
+ "business day; it is equal to the ledger balance of the account.
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: bank
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("currentBalance")
protected Money currentBalance;
@ApiModelProperty(readOnly = true,
value = "The type of account that is aggregated, i.e., savings, checking, credit card, charge, HELOC, etc. "
+ "The account type is derived based on the attributes of the account. " //
+ "
Valid Values:"//
+ "
Aggregated Account Type"//
+ "
bank"//
+ ""//
+ "- CHECKING
"//
+ "- SAVINGS
"//
+ "- CD
"//
+ "- PPF
"//
+ "- RECURRING_DEPOSIT
"//
+ "- FSA
"//
+ "- MONEY_MARKET
"//
+ "- IRA
"//
+ "- PREPAID
"//
+ "
"//
+ "creditCard"//
+ ""//
+ "- OTHER
"//
+ "- CREDIT
"//
+ "- STORE
"//
+ "- CHARGE
"//
+ "- OTHER
"//
+ "
"//
+ "investment (SN 1.0)"//
+ "" + "- BROKERAGE_MARGIN
"//
+ "- HSA
"//
+ "- IRA
"//
+ "- BROKERAGE_CASH
"//
+ "- 401K
"//
+ "- 403B
"//
+ "- TRUST
"//
+ "- ANNUITY
"//
+ "- SIMPLE
"//
+ "- CUSTODIAL
"//
+ "- BROKERAGE_CASH_OPTION
"//
+ "- BROKERAGE_MARGIN_OPTION
"//
+ "- INDIVIDUAL
"//
+ "- CORPORATE
"//
+ "- JTTIC
"//
+ "- JTWROS
"//
+ "- COMMUNITY_PROPERTY
"//
+ "- JOINT_BY_ENTIRETY
"//
+ "- CONSERVATORSHIP
"//
+ "- ROTH
"//
+ "- ROTH_CONVERSION
"//
+ "- ROLLOVER
"//
+ "- EDUCATIONAL
"//
+ "- 529_PLAN
"//
+ "- 457_DEFERRED_COMPENSATION
"//
+ "- 401A
"//
+ "- PSP
"//
+ "- MPP
"//
+ "- STOCK_BASKET
"//
+ "- LIVING_TRUST
"//
+ "- REVOCABLE_TRUST
"//
+ "- IRREVOCABLE_TRUST
"//
+ "- CHARITABLE_REMAINDER
"//
+ "- CHARITABLE_LEAD
"//
+ "- CHARITABLE_GIFT_ACCOUNT
"//
+ "- SEP
"//
+ "- UTMA
"//
+ "- UGMA
"//
+ "- ESOPP
"//
+ "- ADMINISTRATOR
"//
+ "- EXECUTOR
"//
+ "- PARTNERSHIP
"//
+ "- SOLE_PROPRIETORSHIP
"//
+ "- CHURCH
"//
+ "- INVESTMENT_CLUB
"//
+ "- RESTRICTED_STOCK_AWARD
"//
+ "- CMA
"//
+ "- EMPLOYEE_STOCK_PURCHASE_PLAN
"//
+ "- PERFORMANCE_PLAN
"//
+ "- BROKERAGE_LINK_ACCOUNT
"//
+ "- MONEY_MARKET
"//
+ "- SUPER_ANNUATION
"//
+ "- REGISTERED_RETIREMENT_SAVINGS_PLAN
"//
+ "- SPOUSAL_RETIREMENT_SAVINGS_PLAN
"//
+ "- DEFERRED_PROFIT_SHARING_PLAN
"//
+ "- NON_REGISTERED_SAVINGS_PLAN
"//
+ "- REGISTERED_EDUCATION_SAVINGS_PLAN
"//
+ "- GROUP_RETIREMENT_SAVINGS_PLAN
"//
+ "- LOCKED_IN_RETIREMENT_SAVINGS_PLAN
"//
+ "- RESTRICTED_LOCKED_IN_SAVINGS_PLAN
"//
+ "- LOCKED_IN_RETIREMENT_ACCOUNT
"//
+ "- REGISTERED_PENSION_PLAN
"//
+ "- TAX_FREE_SAVINGS_ACCOUNT
"//
+ "- LIFE_INCOME_FUND
"//
+ "- REGISTERED_RETIREMENT_INCOME_FUND
"//
+ "- SPOUSAL_RETIREMENT_INCOME_FUND
"//
+ "- LOCKED_IN_REGISTERED_INVESTMENT_FUND
"//
+ "- PRESCRIBED_REGISTERED_RETIREMENT_INCOME_FUND
"//
+ "- GUARANTEED_INVESTMENT_CERTIFICATES
"//
+ "- REGISTERED_DISABILITY_SAVINGS_PLAN
"//
+ "- DIGITAL_WALLET
"//
+ "- OTHER
"//
+ "
"//
+ "investment (SN 2.0)"//
+ ""//
+ "- BROKERAGE_CASH
"//
+ "- BROKERAGE_MARGIN
"//
+ "- INDIVIDUAL_RETIREMENT_ACCOUNT_IRA
"//
+ "- EMPLOYEE_RETIREMENT_ACCOUNT_401K
"//
+ "- EMPLOYEE_RETIREMENT_SAVINGS_PLAN_403B
"//
+ "- TRUST
"//
+ "- ANNUITY
"//
+ "- SIMPLE_IRA
"//
+ "- CUSTODIAL_ACCOUNT
"//
+ "- BROKERAGE_CASH_OPTION
"//
+ "- BROKERAGE_MARGIN_OPTION
"//
+ "- INDIVIDUAL
"//
+ "- CORPORATE_INVESTMENT_ACCOUNT
"//
+ "- JOINT_TENANTS_TENANCY_IN_COMMON_JTIC
"//
+ "- JOINT_TENANTS_WITH_RIGHTS_OF_SURVIVORSHIP_JTWROS
"//
+ "- JOINT_TENANTS_COMMUNITY_PROPERTY
"//
+ "- JOINT_TENANTS_TENANTS_BY_ENTIRETY
"//
+ "- CONSERVATOR
"//
+ "- ROTH_IRA
"//
+ "- ROTH_CONVERSION
"//
+ "- ROLLOVER_IRA
"//
+ "- EDUCATIONAL
"//
+ "- EDUCATIONAL_SAVINGS_PLAN_529
"//
+ "- DEFERRED_COMPENSATION_PLAN_457
"//
+ "- MONEY_PURCHASE_RETIREMENT_PLAN_401A
"//
+ "- PROFIT_SHARING_PLAN
"//
+ "- MONEY_PURCHASE_PLAN
"//
+ "- STOCK_BASKET_ACCOUNT
"//
+ "- LIVING_TRUST
"//
+ "- REVOCABLE_TRUST
"//
+ "- IRREVOCABLE_TRUST
"//
+ "- CHARITABLE_REMAINDER_TRUST
"//
+ "- CHARITABLE_LEAD_TRUST
"//
+ "- CHARITABLE_GIFT_ACCOUNT
"//
+ "- SEP_IRA
"//
+ "- UNIFORM_TRANSFER_TO_MINORS_ACT_UTMA
"//
+ "- UNIFORM_GIFT_TO_MINORS_ACT_UGMA
"//
+ "- EMPLOYEE_STOCK_OWNERSHIP_PLAN_ESOP
"//
+ "- ADMINISTRATOR
"//
+ "- EXECUTOR
"//
+ "- PARTNERSHIP
"//
+ "- PROPRIETORSHIP
"//
+ "- CHURCH_ACCOUNT
"//
+ "- INVESTMENT_CLUB
"//
+ "- RESTRICTED_STOCK_AWARD
"//
+ "- CASH_MANAGEMENT_ACCOUNT
"//
+ "- EMPLOYEE_STOCK_PURCHASE_PLAN_ESPP
"//
+ "- PERFORMANCE_PLAN
"//
+ "- BROKERAGE_LINK_ACCOUNT
"//
+ "- MONEY_MARKET_ACCOUNT
"//
+ "- SUPERANNUATION
"//
+ "- REGISTERED_RETIREMENT_SAVINGS_PLAN_RRSP
"//
+ "- SPOUSAL_RETIREMENT_SAVINGS_PLAN_SRSP
"//
+ "- DEFERRED_PROFIT_SHARING_PLAN_DPSP
"//
+ "- NON_REGISTERED_SAVINGS_PLAN_NRSP
"//
+ "- REGISTERED_EDUCATION_SAVINGS_PLAN_RESP
"//
+ "- GROUP_RETIREMENT_SAVINGS_PLAN_GRSP
"//
+ "- LOCKED_IN_RETIREMENT_SAVINGS_PLAN_LRSP
"//
+ "- RESTRICTED_LOCKED_IN_SAVINGS_PLAN_RLSP
"//
+ "- LOCKED_IN_RETIREMENT_ACCOUNT_LIRA
"//
+ "- REGISTERED_PENSION_PLAN_RPP
"//
+ "- TAX_FREE_SAVINGS_ACCOUNT_TFSA
"//
+ "- LIFE_INCOME_FUND_LIF
"//
+ "- REGISTERED_RETIREMENT_INCOME_FUND_RIF
"//
+ "- SPOUSAL_RETIREMENT_INCOME_FUND_SRIF
"//
+ "- LOCKED_IN_REGISTERED_INVESTMENT_FUND_LRIF
"//
+ "- PRESCRIBED_REGISTERED_RETIREMENT_INCOME_FUND_PRIF
"//
+ "- GUARANTEED_INVESTMENT_CERTIFICATES_GIC
"//
+ "- REGISTERED_DISABILITY_SAVINGS_PLAN_RDSP
"//
+ "- DEFINED_CONTRIBUTION_PLAN
"//
+ "- DEFINED_BENEFIT_PLAN
"//
+ "- EMPLOYEE_STOCK_OPTION_PLAN
"//
+ "- NONQUALIFIED_DEFERRED_COMPENSATION_PLAN_409A
"//
+ "- KEOGH_PLAN
"//
+ "- EMPLOYEE_RETIREMENT_ACCOUNT_ROTH_401K
"//
+ "- DEFERRED_CONTINGENT_CAPITAL_PLAN_DCCP
"//
+ "- EMPLOYEE_BENEFIT_PLAN
"//
+ "- EMPLOYEE_SAVINGS_PLAN
"//
+ "- HEALTH_SAVINGS_ACCOUNT_HSA
"//
+ "- COVERDELL_EDUCATION_SAVINGS_ACCOUNT_ESA
"//
+ "- TESTAMENTARY_TRUST
"//
+ "- ESTATE
"//
+ "- GRANTOR_RETAINED_ANNUITY_TRUST_GRAT
"//
+ "- ADVISORY_ACCOUNT
"//
+ "- NON_PROFIT_ORGANIZATION_501C
"//
+ "- HEALTH_REIMBURSEMENT_ARRANGEMENT_HRA
"//
+ "- INDIVIDUAL_SAVINGS_ACCOUNT_ISA
"//
+ "- CASH_ISA
"//
+ "- STOCKS_AND_SHARES_ISA
"//
+ "- INNOVATIVE_FINANCE_ISA
"//
+ "- JUNIOR_ISA
"//
+ "- EMPLOYEES_PROVIDENT_FUND_ORGANIZATION_EPFO
"//
+ "- PUBLIC_PROVIDENT_FUND_PPF
"//
+ "- EMPLOYEES_PENSION_SCHEME_EPS
"//
+ "- NATIONAL_PENSION_SYSTEM_NPS
"//
+ "- INDEXED_ANNUITY
"//
+ "- ANNUITIZED_ANNUITY
"//
+ "- VARIABLE_ANNUITY
"//
+ "- ROTH_403B
"//
+ "- SPOUSAL_IRA
"//
+ "- SPOUSAL_ROTH_IRA
"//
+ "- SARSEP_IRA
"//
+ "- SUBSTANTIALLY_EQUAL_PERIODIC_PAYMENTS_SEPP
"//
+ "- OFFSHORE_TRUST
"//
+ "- IRREVOCABLE_LIFE_INSURANCE_TRUST
"//
+ "- INTERNATIONAL_TRUST
"//
+ "- LIFE_INTEREST_TRUST
"//
+ "- EMPLOYEE_BENEFIT_TRUST
"//
+ "- PRECIOUS_METAL_ACCOUNT
"//
+ "- INVESTMENT_LOAN_ACCOUNT
"//
+ "- GRANTOR_RETAINED_INCOME_TRUST
"//
+ "- PENSION_PLAN
"//
+ "- DIGITAL_WALLET
"//
+ "- OTHER
"//
+ "
"//
+ "loan"//
+ ""//
+ "- MORTGAGE
"//
+ "- INSTALLMENT_LOAN
"//
+ "- PERSONAL_LOAN
"//
+ "- HOME_EQUITY_LINE_OF_CREDIT
"//
+ "- LINE_OF_CREDIT
"//
+ "- AUTO_LOAN
"//
+ "- STUDENT_LOAN
"//
+ "- HOME_LOAN
"//
+ "
"//
+ "insurance"//
+ ""//
+ "- AUTO_INSURANCE
"//
+ "- HEALTH_INSURANCE
"//
+ "- HOME_INSURANCE
"//
+ "- LIFE_INSURANCE
"//
+ "- ANNUITY
"//
+ "- TRAVEL_INSURANCE
"//
+ "- INSURANCE
"//
+ "
"//
+ "realEstate"//
+ " " + "- REAL_ESTATE
"//
+ "
"//
+ "reward"//
+ ""//
+ "- REWARD_POINTS
"//
+ "
"//
+ "Manual Account Type
"//
+ "bank"//
+ ""//
+ "- CHECKING
"//
+ "- SAVINGS
"//
+ "- CD
"//
+ "- PREPAID
"//
+ "
"//
+ "credit"//
+ " " + "- CREDIT
"//
+ "
"//
+ "loan"//
+ " " + "- PERSONAL_LOAN
"//
+ "- HOME_LOAN
"//
+ "
"//
+ "insurance"//
+ ""//
+ "- INSURANCE
"//
+ "- ANNUITY
"//
+ "
"//
+ "investment"//
+ ""//
+ "- BROKERAGE_CASH
"//
+ "
"//
+ "
"//
+ "Aggregated / Manual: Both
"//
+ "Applicable containers: All containers
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("accountType")
protected String accountType;
@ApiModelProperty(readOnly = true,
value = "The name or identification of the account owner, as it appears at the FI site. "
+ "
Note: The account holder name can be full or partial based on how it is displayed in the account "
+ "summary page of the FI site. In most cases, the FI site does not display the full account holder "
+ "name in the account summary page."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: bank, creditCard, investment, insurance, loan, reward
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("displayedName")
protected String displayedName;
@ApiModelProperty(readOnly = true,
value = "The date on which the account is created in the Yodlee system."
+ "
Additional Details: It is the date when the user links or aggregates the account(s) that "
+ "are held with the provider to the Yodlee system."//
+ "
"//
+ "Aggregated / Manual: Both
"//
+ "Applicable containers: All containers
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("createdDate")
protected String createdDate;
@ApiModelProperty(readOnly = true,
value = "A unique ID that the provider site has assigned to the account. "
+ "The source ID is only available for the HELD accounts." + "
"//
+ "Applicable containers: bank, creditCard, investment, insurance, loan, reward
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("sourceId")
protected String sourceId;
@ApiModelProperty(readOnly = true,
value = "The date on which the due amount has to be paid. " + "
Additional Details:"
+ "
Credit Card: The monthly date by when the minimum payment is due to be paid on the credit card account. "
+ "
Loan: The date on or before which the due amount should be paid."
+ "
Note: The due date at the account-level can differ from the due date field at the statement-level, as the "
+ "information in the aggregated card account data provides an up-to-date information to the consumer."
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: creditCard, loan, insurance
"//
+ "Endpoints:"//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("dueDate")
protected String dueDate;
@ApiModelProperty(readOnly = true,
value = "The amount borrowed from the 401k account.
"
+ "Note: The 401k loan field is only applicable to the 401k account type.
"//
+ "Applicable containers: investment
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Endpoints:"//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("401kLoan")
protected Money loan401k;
@ApiModelProperty(readOnly = true,
value = "Indicates the contract value of the annuity.
"
+ "Note: The annuity balance field is applicable only to annuities.
"//
+ "Applicable containers: insurance, investment
"//
+ "Aggregated / Manual: Both
"//
+ "Endpoints:"//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("annuityBalance")
protected Money annuityBalance;
@ApiModelProperty(readOnly = true,
value = "Interest paid from the start of the year to date.
"//
+ "Applicable containers: loan
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Endpoints:
"//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("interestPaidYTD")
protected Money interestPaidYTD;
@ApiModelProperty(readOnly = true,
value = "Interest paid in last calendar year.
"//
+ "Applicable containers: loan
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Endpoints:
"//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("interestPaidLastYear")
protected Money interestPaidLastYear;
@ApiModelProperty(readOnly = true,
value = "The type of the interest rate, for example, fixed or variable.
"//
+ "Applicable containers: loan
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Endpoints:
"//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
"//
+ "Applicable Values
"//
)
@JsonProperty("interestRateType")
protected LoanInterestRateType interestRateType;
@ApiModelProperty(readOnly = true,
value = "Property or possession offered to support a loan that can be seized on a default.
"//
+ "Applicable containers: loan
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Endpoints:
"//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("collateral")
protected String collateral;
@ApiModelProperty(readOnly = true,
value = "Annual percentage yield (APY) is a normalized representation of an interest rate, "
+ "based on a compounding period of one year. "
+ "APY generally refers to the rate paid to a depositor by a financial institution on an account.
"//
+ "Applicable containers: bank
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Endpoints:
"//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("annualPercentageYield")
protected Double annualPercentageYield;
@ApiModelProperty(readOnly = true,
value = "The financial cost that the policyholder pays to the insurance company to obtain an insurance cover."
+ "The premium is paid as a lump sum or in installments during the duration of the policy.
"//
+ "Applicable containers: insurance
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Endpoints:
"//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("premium")
protected Money premium;
@ApiModelProperty(readOnly = true,
value = "The sum of the future payments due to be paid to the insurance company during a policy year. "
+ "It is the policy rate minus the payments made till date."
+ "
Note: The remaining balance field is applicable only to auto insurance and home insurance.
"//
+ "Applicable containers: insurance
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Endpoints:
"//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("remainingBalance")
protected Money remainingBalance;
@ApiModelProperty(readOnly = true,
value = "The date on which the insurance policy coverage commences.
"//
+ "Applicable containers: insurance
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Endpoints:
"//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("policyEffectiveDate")
protected String policyEffectiveDate;
@ApiModelProperty(readOnly = true,
value = "The date the insurance policy began.
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: insurance
"//
+ "Endpoints:
"//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("policyFromDate")
protected String policyFromDate;
@ApiModelProperty(readOnly = true,
value = "The date to which the policy exists.
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: insurance
"//
+ "Endpoints:
"//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("policyToDate")
protected String policyToDate;
@ApiModelProperty(readOnly = true,
value = "The death benefit amount on a life insurance policy and annuity. "
+ "It is usually equal to the face amount of the policy, but sometimes can vary for a whole life and universal life insurance policies."
+ "
Note: The death benefit amount field is applicable only to annuities and life insurance.
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: insurance
"//
+ "Endpoints:
"//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("deathBenefit")
protected Money deathBenefit;
@ApiModelProperty(readOnly = true,
value = "The duration for which the policy is valid or in effect. For example, one year, five years, etc.
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: insurance
"//
+ "Endpoints:
"//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("policyTerm")
protected String policyTerm;
@ApiModelProperty(readOnly = true,
value = "The status of the policy.
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: insurance
"//
+ "Endpoints:
"//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
"//
+ "Applicable Values
"//
)
@JsonProperty("policyStatus")
protected PolicyStatus policyStatus;
@ApiModelProperty(readOnly = true,
value = "The annual percentage rate (APR) is the yearly rate of interest on the credit card account.
"
+ "Additional Details: The yearly percentage rate charged when a balance is held on a credit card. This rate of interest is applied every month on the outstanding credit card balance.
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: creditCard
"//
+ "Endpoints:
"//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("apr")
protected Double apr;
@ApiModelProperty(readOnly = true,
value = "Derived APR will be an estimated purchase APR based on consumers credit card transactions and credit card purchase.
"
+ "Aggregated / Manual / Derived: Derived
"//
+ "Applicable containers: creditCard
"//
+ "Endpoints:
"//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("derivedApr")
protected Double derivedApr;
@ApiModelProperty(value = "Annual percentage rate applied to cash withdrawals on the card.
"//
+ "Account Type: Aggregated
"//
+ "Applicable containers: creditCard
"//
+ "Endpoints:"//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("cashApr")
protected Double cashApr;
@ApiModelProperty(readOnly = true,
value = "The amount that is available for an ATM withdrawal, i.e., the cash available after deducting the amount that is already withdrawn from the total cash limit. (totalCashLimit-cashAdvance= availableCash)"
+ "
Additional Details: The available cash amount at the account-level can differ from the available cash at the statement-level, as the information in the aggregated card account data provides more up-to-date information.
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: creditCard
"//
+ "Endpoints:
"//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("availableCash")
protected Money availableCash;
@ApiModelProperty(readOnly = true,
value = "
Credit Card: Amount that is available to spend on the credit card. It is usually "
+ "the Total credit line- Running balance- pending charges. "
+ "
Loan: The unused portion of line of credit, on a revolving loan (such as a home-equity line of credit)."
+ "
Additional Details:"
+ "
Note: The available credit amount at the account-level can differ from the available credit "
+ "field at the statement-level, as the information in the aggregated card account data provides more up-to-date information.
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: creditCard, loan
"//
+ "Endpoints:"//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("availableCredit")
protected Money availableCredit;
@ApiModelProperty(readOnly = true,
value = "The amount that is available for immediate withdrawal or the total amount available to purchase securities in a brokerage or investment account."
+ "
Note: The cash balance field is only applicable to brokerage related accounts.
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: investment
"//
+ "Endpoints:"//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("cash")
protected Money cash;
@ApiModelProperty(readOnly = true,
value = "The amount of cash value available in the consumer's life insurance policy account - except for term "
+ "insurance policy - for withdrawals, loans, etc. This field is also used to capture the cash value on the home "
+ "insurance policy.It is the standard that the insurance company generally prefer to reimburse the policyholder for "
+ "his or her loss, i.e., the cash value is equal to the replacement cost minus depreciation. The cash value is also "
+ "referred to as surrender value in India for life insurance policies."
+ "
Note: The cash value field is applicable to all types of life insurance (except for term life) and home insurance.
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: insurance
"//
+ "Endpoints:"//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("cashValue")
protected Money cashValue;
@ApiModelProperty(readOnly = true,
value = "The classification of the account such as personal, corporate, etc.
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: bank, creditCard, investment, reward, loan, insurance
"//
+ "Endpoints:"//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
"//
+ "Applicable Values
"//
)
@JsonProperty("classification")
protected AccountClassification classification;
@ApiModelProperty(readOnly = true,
value = "The date on which the insurance policy expires or matures."
+ "
Additional Details: The due date at the account-level can differ from the due date field at the "
+ "statement-level, as the information in the aggregated card account data provides "
+ "an up-to-date information to the consumer.
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: insurance
"//
+ "Endpoints:"//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("expirationDate")
protected String expirationDate;
@ApiModelProperty(readOnly = true,
value = "The amount stated on the face of a consumer's policy that will be paid "
+ "in the event of his or her death or any other event as stated in the insurance policy. "
+ "The face amount is also referred to as the sum insured or maturity value in India."
+ "
Note: The face amount field is applicable only to life insurance.
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: insurance
"//
+ "Endpoints:"//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("faceAmount")
protected Money faceAmount;
@ApiModelProperty(readOnly = true,
value = "
Bank: The interest rate offered by a FI to its depositors on a bank account."
+ "
Loan: Interest rate applied on the loan." + "
Additional Details:"
+ "
Note: The Interest Rate field is only applicable for the following account types: savings, checking, money market, and certificate of deposit.
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: bank, loan
"//
+ "Endpoints:"//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("interestRate")
protected Double interestRate;
@ApiModelProperty(readOnly = true,
value = "The last payment made for the account."//
+ "
Aggregated / Manual: Aggregated
"//
+ "Applicable containers: bill
"//
+ "Endpoints:"//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("lastPayment")
protected Money lastPayment;
@ApiModelProperty(readOnly = true,
value = "Last/Previous payment amount on the account. Portion of the principal "
+ "and interest paid on previous month or period to satisfy a loan."
+ "
Additional Details: If the payment is already done for the current billing cycle, then the "
+ "field indicates the payment of the current billing cycle. "
+ "If payment is yet to be done for the current billing cycle, then the "
+ "field indicates the payment that was made for any of the previous billing cycles.
"
+ "
Aggregated / Manual: Aggregated
"//
+ "Applicable containers: creditCard, loan, insurance
"//
+ "Endpoints:"//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("lastPaymentAmount")
protected Money lastPaymentAmount;
@ApiModelProperty(readOnly = true,
value = "The date on which the payment for the previous or current billing cycle is done."
+ "
Additional Details: If the payment is already done for the current billing cycle, "
+ "then the field indicates the payment date of the current billing cycle. "
+ "If payment is yet to be done for the current billing cycle, then the field "
+ "indicates the date on which the payment was made for any of the previous billing cycles. "
+ "The last payment date at the account-level can differ from the last payment date at the "
+ "statement-level, as the information in the aggregated card account data provides an "
+ "up-to-date information to the consumer.
"//
+ "
Aggregated / Manual: Aggregated
"//
+ "Applicable containers: creditCard, loan, insurance
"//
+ "Endpoints:"//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("lastPaymentDate")
protected String lastPaymentDate;
@ApiModelProperty(readOnly = true,
value = "The date time the account information was last retrieved from the provider site and updated in the Yodlee system.
"//
+ "
Aggregated / Manual: Both
"//
+ "Applicable containers: All containers
"//
+ "Endpoints:"//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("lastUpdated")
protected String lastUpdated;
@ApiModelProperty(readOnly = true,
value = "The amount of borrowed funds used to purchase securities."
+ "
Note: Margin balance is displayed only if the brokerage account is approved for margin. "
+ "The margin balance field is only applicable to brokerage related accounts."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: investment
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("marginBalance")
protected Money marginBalance;
@ApiModelProperty(readOnly = true,
value = "The maturity amount on the CD is the amount(principal and interest) paid on or after the maturity date."
+ "
Additional Details: The Maturity Amount field is only applicable for the account type CD(Fixed Deposits)."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: bank
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("maturityAmount")
protected Money maturityAmount;
@ApiModelProperty(readOnly = true,
value = "The date when a certificate of deposit (CD/FD) matures or the final payment date of a loan "
+ "at which point the principal amount (including pending interest) is due to be paid."
+ "
Additional Details: The date when a certificate of deposit (CD) matures, i.e., the money in the "
+ "CD can be withdrawn without paying an early withdrawal penalty."
+ "The final payment date of a loan, i.e., the principal amount (including pending interest) is due to be paid."
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: bank, loan
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("maturityDate")
protected String maturityDate;
@ApiModelProperty(readOnly = true,
value = "The amount in the money market fund or its equivalent such as bank deposit programs."
+ "
Note: The money market balance field is only applicable to brokerage related accounts."
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: investment
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("moneyMarketBalance")
protected Money moneyMarketBalance;
@ApiModelProperty(readOnly = true,
value = "The nickname of the account as provided by the consumer to identify an account. "
+ "The account nickname can be used instead of the account name." + "
"//
+ "Aggregated / Manual: Both
"//
+ "Applicable containers: All containers
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("nickname")
protected String nickname;
@ApiModelProperty(readOnly = true,
value = "The amount that is currently owed on the credit card account."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: creditCard
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("runningBalance")
protected Money runningBalance;
@ApiModelProperty(readOnly = true,
value = "The maximum amount that can be withdrawn from an ATM using the credit card. "
+ "Credit cards issuer allow cardholders to withdraw cash using their cards - the cash limit is a percent of the overall credit limit."
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: creditCard
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("totalCashLimit")
protected Money totalCashLimit;
@ApiModelProperty(readOnly = true,
value = "Total credit line is the amount of money that can be charged to a credit card. "
+ "If credit limit of $5,000 is issued on a credit card, the total charges on the card cannot exceed this amount."
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: creditCard
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("totalCreditLine")
protected Money totalCreditLine;
@ApiModelProperty(readOnly = true,
value = "The total unvested balance that appears in an investment account."
+ "Such as the 401k account or the equity award account that includes employer provided funding. "//
+ "
Note: The amount the employer contributes is generally subject to vesting and remain "
+ "unvested for a specific period of time or until fulfillment of certain conditions. "
+ "The total unvested balance field is only applicable to the retirement related accounts "
+ "such as 401k, equity awards, etc."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: investment
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("totalUnvestedBalance")
protected Money totalUnvestedBalance;
@ApiModelProperty(readOnly = true,
value = "The total vested balance that appears in an investment account. Such as the "
+ "401k account or the equity award account that includes employer provided funding. "
+ "
Note: The amount an employee can claim after he or she leaves the organization. The total "
+ "vested balance field is only applicable to the retirement related accounts such as 401k, equity awards, etc."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: investment
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("totalVestedBalance")
protected Money totalVestedBalance;
@ApiModelProperty(readOnly = true,
value = "The amount a mortgage company holds to pay a consumer's non-mortgage related expenses like insurance and property taxes. "
+ "
Additional Details:"//
+ "
Note: The escrow balance field is only applicable to the mortgage account type."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: loan
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("escrowBalance")
protected Money escrowBalance;
@ApiModelProperty(readOnly = true,
value = "Type of home insurance, like -"//
+ "- HOME_OWNER
"//
+ "- RENTAL
"//
+ "- RENTER
"//
+ "- etc..
"//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: insurance
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
"//
+ "Applicable Values
"//
)
@JsonProperty("homeInsuranceType")
protected HomeInsuranceType homeInsuranceType;
@ApiModelProperty(readOnly = true,
value = "Type of life insurance."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: insurance
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
"//
+ "Applicable Values
"//
)
@JsonProperty("lifeInsuranceType")
protected LifeInsuranceType lifeInsuranceType;
@ApiModelProperty(readOnly = true,
value = "The amount of loan that the lender has provided."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: loan
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("originalLoanAmount")
protected Money originalLoanAmount;
@ApiModelProperty(readOnly = true,
value = "The principal or loan balance is the outstanding balance on a loan account, excluding the interest and fees. "
+ "The principal balance is the original borrowed amount plus any applicable loan fees, minus any principal payments."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: loan
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("principalBalance")
protected Money principalBalance;
@ApiModelProperty(readOnly = true,
value = "The number of years for which premium payments have to be made in a policy."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: insurance
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("premiumPaymentTerm")
protected String premiumPaymentTerm;
@ApiModelProperty(readOnly = true,
value = "The monthly or periodic payment on a loan that is recurring in nature. The recurring payment "
+ "amount is usually same as the amount due, unless late fees or other charges are added eventually "
+ "changing the amount due for a particular month."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: loan
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("recurringPayment")
protected Money recurringPayment;
@ApiModelProperty(readOnly = true,
value = "The tenure for which the CD account is valid or in case of loan, the number"
+ " of years/months over which the loan amount has to be repaid. "//
+ "
Additional Details:
"
+ "Bank: The Term field is only applicable for the account type CD."
+ "Loan: The period for which the loan agreement is in force. The period, before or at the "
+ "end of which, the loan should either be repaid or renegotiated for another term."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: bank, loan
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("term")
protected String term;
@ApiModelProperty(readOnly = true,
value = "The maximum amount of credit a financial institution extends to a consumer "
+ "through a line of credit or a revolving loan like HELOC. "//
+ "
Additional Details:"//
+ "
Note: The credit limit field is applicable only to LOC and HELOC account types."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: loan
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("totalCreditLimit")
protected Money totalCreditLimit;
@ApiModelProperty(readOnly = true,
value = "Date on which the user is enrolled on the rewards program."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: reward
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("enrollmentDate")
protected String enrollmentDate;
@ApiModelProperty(readOnly = true,
value = "Primary reward unit for this reward program. E.g. miles, points, etc."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: reward
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("primaryRewardUnit")
protected String primaryRewardUnit;
@ApiModelProperty(readOnly = true,
value = "Current level of the reward program the user is associated with. E.g. Silver, Jade etc."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: reward
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("currentLevel")
protected String currentLevel;
@ApiModelProperty(readOnly = true,
value = "The eligible next level of the rewards program."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: reward
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("nextLevel")
protected String nextLevel;
@ApiModelProperty(readOnly = true,
value = "The sum of the current market values of short positions held in a brokerage account."
+ "
Note: The short balance balance field is only applicable to brokerage related accounts."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: investment
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("shortBalance")
protected Money shortBalance;
@ApiModelProperty(readOnly = true,
value = "Indicates the last amount contributed by the employee to the 401k account."
+ "
Note: The last employee contribution amount field is derived from the transaction"
+ " data and not aggregated from the FI site. The field is only applicable to the 401k account type."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: investment
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("lastEmployeeContributionAmount")
protected Money lastEmployeeContributionAmount;
@ApiModelProperty(readOnly = true,
value = "The date on which the last employee contribution was made to the 401k account."
+ "
Note: The last employee contribution date field is derived from the transaction data "
+ "and not aggregated from the FI site. The field is only applicable to the 401k account type."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: investment
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("lastEmployeeContributionDate")
protected String lastEmployeeContributionDate;
@ApiModelProperty(readOnly = true,
value = "The additional description or notes given by the user."//
+ "
"//
+ "Aggregated / Manual: Both
"//
+ "Applicable containers: All containers
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("memo")
protected String memo;
@ApiModelProperty(readOnly = true,
value = "The date on which the loan is disbursed."//
+ "
"//
+ "Aggregated / Manual: Both
"//
+ "Applicable containers: loan
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("originationDate")
protected String originationDate;
@ApiModelProperty(readOnly = true,
value = "The overdraft limit for the account."
+ "
Note: The overdraft limit is provided only for AUS, INDIA, UK, NZ locales."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: bank
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("overDraftLimit")
protected Money overDraftLimit;
@ApiModelProperty(readOnly = true,
value = "The valuation type indicates whether the home value is calculated either manually or by Yodlee Partners."//
+ "
"//
+ "Aggregated / Manual: Manual
"//
+ "Applicable containers: realEstate
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
"//
+ "Applicable Values
"//
)
@JsonProperty("valuationType")
protected ValuationType valuationType;
@ApiModelProperty(readOnly = true,
value = "The home value of the real estate account."//
+ "
"//
+ "Aggregated / Manual: Manual
"//
+ "Applicable containers: realEstate
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("homeValue")
protected Money homeValue;
@ApiModelProperty(readOnly = true,
value = "The date on which the home value was estimated."//
+ "
"//
+ "Aggregated / Manual: Manual
"//
+ "Applicable containers: realEstate
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("estimatedDate")
protected String estimatedDate;
@ApiModelProperty(readOnly = true,
value = "The home address of the real estate account. The address entity for home address consists of state, zip and city only"//
+ "
"//
+ "Aggregated / Manual: Manual
"//
+ "Applicable containers: realEstate
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("address")
protected AccountAddress address;
@ApiModelProperty(readOnly = true,
value = "The amount to be paid to close the loan account, i.e., the total amount "
+ "required to meet a borrower's obligation on a loan."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: loan
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("loanPayoffAmount")
protected Money loanPayoffAmount;
@ApiModelProperty(readOnly = true,
value = "The date by which the payoff amount should be paid."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: loan
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("loanPayByDate")
protected String loanPayByDate;
@ApiModelProperty(readOnly = true,
value = "Bank and branch identification information.
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: bank, investment, loan
"//
+ "Endpoints:
"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
"//
)
@JsonProperty("bankTransferCode")
protected List bankTransferCode;
@ApiModelProperty(readOnly = true,
value = "Information of different reward balances associated with the account."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: reward
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("rewardBalance")
protected List rewardBalance;
@ApiModelProperty(readOnly = true,
value = "The frequency of the billing cycle of the account in case of card. "
+ "The frequency in which premiums are paid in an "
+ "insurance policy such as monthly, quarterly, and annually. "
+ "The frequency in which due amounts are paid in a loan account."//
+ "
"//
+ "Aggregated / Manual: Both
"//
+ "Applicable containers: creditCard, insurance, loan
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
"//
+ "Applicable Values
"//
)
@JsonProperty("frequency")
protected FrequencyType frequency;
@ApiModelProperty(readOnly = true,
value = "The amount due to be paid for the account.
"//
+ "Additional Details:"
+ "Credit Card: The total amount due for the purchase of goods or services that must be paid by the due date.
"
+ "Loan: The amount due to be paid on the due date.
"
+ "Note: The amount due at the account-level can differ from the amount due at the statement-level, as the information in the aggregated card account data provides more up-to-date information.
"//
+ "Applicable containers: creditCard, loan, insurance
"//
+ "Aggregated / Manual: Both
"//
+ "Endpoints:
"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("amountDue")
protected Money amountDue;
@ApiModelProperty(readOnly = true,
value = "The minimum amount due is the lowest amount of money that a consumer is required to pay each month."
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: creditCard, insurance, loan
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("minimumAmountDue")
protected Money minimumAmountDue;
@ApiModelProperty(readOnly = true,
value = "Logical grouping of dataset attributes into datasets such as Basic Aggregation Data, Account Profile and Documents."
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: All containers
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("dataset")
protected List datasets;
////
@ApiModelProperty(readOnly = true,
value = "The providerAccountIds that share the account with the primary providerAccountId that was created when the user had added the account for the first time."//
+ "
Additional Details: This attribute is returned in the response only if the account deduplication feature is enabled and the same account is mapped to more than one provider account IDs indicating the account is owned by more than one user, for example, joint accounts."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: All Containers
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("associatedProviderAccountId")
protected List associatedProviderAccountIds;
@ApiModelProperty(readOnly = true,
value = "The loan payoff details such as date by which the payoff amount should be paid, loan payoff amount, and the outstanding balance on the loan account."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: loan
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("loanPayoffDetails")
protected LoanPayoffDetails loanPayOffDetails;
@ApiModelProperty(readOnly = true,
value = "The coverage-related details of the account."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: insurance,investment
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("coverage")
protected List coverage;
@ApiModelProperty(readOnly = true,
value = "Indicates the status of the loan account. "//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: loan
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
"//
+ "Applicable Values:
")
@JsonProperty("sourceAccountStatus")
protected SourceAccountStatus sourceAccountStatus;
@ApiModelProperty(readOnly = true,
value = "The type of repayment plan that the borrower prefers to repay the loan. "//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: loan
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
"//
+ "Applicable Values:
")
@JsonProperty("repaymentPlanType")
protected LoanRepaymentPlanType repaymentPlanType;
@ApiModelProperty(readOnly = true,
value = "A nonprofit or state organization that works with lender, servicer, school, and the Department of Education to help successfully repay Federal Family Education Loan Program (FFELP) loans. If FFELP student loans default, the guarantor takes ownership of them."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: loan
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("guarantor")
protected String guarantor;
@ApiModelProperty(readOnly = true,
value = "The financial institution that provides the loan."//
+ "
"//
+ "Aggregated / Manual: Aggregated
"//
+ "Applicable containers: loan
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("lender")
protected String lender;
@ApiModelProperty(readOnly = true,
value = "The type of account that is aggregated.")
@JsonProperty("aggregatedAccountType")
protected String aggregatedAccountType;
/**
* The type of account that is aggregated.
*
* Aggregated / Manual: Aggregated
* Applicable containers: All Containers
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return aggregatedAccountType
*/
public String getAggregatedAccountType() {
return aggregatedAccountType;
}
/**
* The loan payoff details such as date by which the payoff amount should be paid, loan payoff amount, and the
* outstanding balance on the loan account.
*
* Aggregated / Manual: Aggregated
* Applicable containers: loan
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return loanPayOffDetails
*/
@JsonProperty("loanPayoffDetails")
public LoanPayoffDetails getLoanPayOffDetails() {
return loanPayOffDetails;
}
/**
* The providerAccountIds that share the account with the primary providerAccountId that was created when the user
* had added the account for the first time.
* Additional Details: This attribute is returned in the response only if the account deduplication feature
* is enabled and the same account is mapped to more than one provider account IDs indicating the account is owned
* by more than one user, for example, joint accounts.
*
* Aggregated / Manual: Aggregated
* Applicable containers: All Containers
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return associatedProviderAccountId
*/
@JsonProperty("associatedProviderAccountId")
public List getAssociatedProviderAccountId() {
return associatedProviderAccountIds == null ? null : Collections.unmodifiableList(associatedProviderAccountIds);
}
/**
* The date on which the due amount has to be paid. *
* Additional Details:
* Credit Card: The monthly date by when the minimum payment is due to be paid on the credit card account.
*
* Loan: The date on or before which the due amount should be paid.
* Note: The due date at the account-level can differ from the due date field at the statement-level, as the
* information in the aggregated card account data provides an up-to-date information to the consumer.
*
* Aggregated / Manual: Aggregated
* Applicable containers: creditCard, loan, insurance
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return dueDate
*/
public String getDueDate() {
return dueDate;
}
/**
* The frequency of the billing cycle of the account in case of card. The frequency in which premiums are
* paid in an insurance policy such as monthly, quarterly, and annually. The frequency in which due amounts are paid
* in a loan account.
*
* Aggregated / Manual: Both
* Applicable containers: creditCard, insurance, loan
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
* Applicable Values
*
* @return frequency
*/
public FrequencyType getFrequency() {
return frequency;
}
/**
* The amount due to be paid for the account.
* Additional Details: Credit Card: The total amount due for the purchase of goods or services that
* must be paid by the due date.
* Loan: The amount due to be paid on the due date.
* Note: The amount due at the account-level can differ from the amount due at the statement-level, as the
* information in the aggregated card account data provides more up-to-date information.
* Applicable containers: creditCard, loan, insurance
* Aggregated / Manual: Both
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return amountDue
*/
public Money getAmountDue() {
return amountDue;
}
/**
* The minimum amount due is the lowest amount of money that a consumer is required to pay each month.
*
* Aggregated / Manual: Aggregated
* Applicable containers: creditCard, insurance, loan
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return minimumAmountDue
*/
public Money getMinimumAmountDue() {
return minimumAmountDue;
}
/**
* The type of service. E.g., Bank, Credit Card, Investment, Insurance, etc.
*
* Aggregated / Manual: Aggregated
* Applicable containers: All containers
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
* Applicable Values
*
* @return CONTAINER
*/
@JsonProperty("CONTAINER")
public Container getContainer() {
return container;
}
/**
* The primary key of the provider account resource.
*
* Aggregated / Manual: Both
* Applicable containers: All containers
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return providerAccountId
*/
public Long getProviderAccountId() {
return providerAccountId;
}
/**
* The status of the account that is updated by the consumer through an application or an API. Valid Values:
* AccountStatus
* Additional Details:
* ACTIVE: All the added manual and aggregated accounts status will be made \ACTIVE\ by default.
* TO_BE_CLOSED: If the aggregated accounts are not found or closed in the data provider site, Yodlee system
* marks the status as TO_BE_CLOSED
* INACTIVE: Users can update the status as INACTIVE to stop updating and to stop considering the account in
* other services
* CLOSED: Users can update the status as CLOSED, if the account is closed with the provider.
*
* Aggregated / Manual: Both
* Applicable containers: All containers
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
* Applicable Values
*
* @return accountStatus
*/
public ItemAccountStatus getAccountStatus() {
return accountStatus;
}
/**
* The account number as it appears on the site. (The POST accounts service response return this field as
* number)
* Additional Details: Bank/ Loan/ Insurance/ Investment:
* The account number for the bank account as it appears at the site.
* Credit Card: The account number of the card account as it appears at the site,
* i.e., the card number.The account number can be full or partial based on how it is displayed in the account
* summary page of the site. In most cases, the site does not display the full account number in the account summary
* page and additional navigation is required to aggregate it.
* Applicable containers: All Containers
* Aggregated / Manual: Both
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
* - POST accounts
*
*
* @return accountNumber
*/
public String getAccountNumber() {
return accountNumber;
}
/**
* The source through which the account(s) are added in the system.
* Valid Values: SYSTEM, USER
* Applicable containers: All Containers
* Aggregated / Manual: Both
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
* Applicable Values
*
* @return aggregationSource
*/
public AggregationSource getAggregationSource() {
return aggregationSource;
}
/**
* The account to be considered as an asset or liability.
* Applicable containers: All Containers
* Aggregated / Manual: Both
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return isAsset
*/
public Boolean getIsAsset() {
return isAsset;
}
/**
* The total account value. *
* Additional Details:
* Bank: available balance or current balance.
* Credit Card: running Balance.
* Investment: The total balance of all the investment account, as it appears on the FI site.
* Insurance: CashValue or amountDue *
* Loan: principalBalance
* Applicable containers: bank, creditCard, investment, insurance, loan, otherAssets, otherLiabilities,
* realEstate
* Aggregated / Manual: Both
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return balance
*/
public Money getBalance() {
return balance;
}
/**
* The primary key of the account resource and the unique identifier for the account.
*
* Aggregated / Manual: Both
* Applicable containers: All containers
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
* - GET investmentOptions
* - GET accounts/historicalBalances
* - POST accounts
*
*
* @return id
*/
public Long getId() {
return id;
}
/**
* The date time the account information was last retrieved from the provider site and updated in the Yodlee
* system.
*
* Aggregated / Manual: Both
* Applicable containers: All containers
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return lastUpdated
*/
public String getLastUpdated() {
return lastUpdated;
}
/**
*
* Applicable containers: reward, bank, creditCard, investment, loan, insurance, realEstate,
* otherLiabilities
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
* - POST accounts
*
* Applicable Values
*
* @return userClassification
*/
public UserClassification getUserClassification() {
return userClassification;
}
/**
* Used to determine whether an account to be considered in the networth calculation.
*
* Aggregated / Manual: Aggregated
* Applicable containers:
* bank,creditCard,loan,investment,insurance,realEstate,otherAssets,otherLiabilities
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return includeInNetWorth
*/
public Boolean getIncludeInNetWorth() {
return includeInNetWorth;
}
/**
* Service provider or institution name where the account originates. This belongs to the provider resource.
*
* Aggregated / Manual: Both
* Applicable containers: All containers
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return providerName
*/
public String getProviderName() {
return providerName;
}
/**
* Identifier of the provider site. The primary key of provider resource.
*
* Aggregated / Manual: Both
* Applicable containers: All containers
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return providerId
*/
public String getProviderId() {
return providerId;
}
/**
* Indicates if an account is aggregated from a site or it is a manual account i.e. account information manually
* provided by the user.
* Aggregated / Manual: Both
* Applicable containers: All containers
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return isManual
*/
public boolean getIsManual() {
return isManual;
}
/**
* The balance in the account that is available for spending. For checking accounts with overdraft, available
* balance may include overdraft amount, if end site adds overdraft balance to available balance.
* Applicable containers: bank, investment
* Aggregated / Manual: Aggregated
* Endpoints:
* GET accounts/{accountId}
*
* @return availableBalance
*/
public Money getAvailableBalance() {
return availableBalance;
}
/**
* The balance in the account that is available at the beginning of the business day; it is equal to the ledger
* balance of the account.
*
* Aggregated / Manual: Aggregated
* Applicable containers: bank
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return currentBalance
*/
public Money getCurrentBalance() {
return currentBalance;
}
/**
* The type of account that is aggregated, i.e., savings, checking, credit card, charge, HELOC, etc. The account
* type is derived based on the attributes of the account.
* Valid Values:
* Aggregated Account Type
* bank
*
* - CHECKING
* - SAVINGS
* - CD
* - PPF
* - RECURRING_DEPOSIT
* - FSA
* - MONEY_MARKET
* - IRA
* - PREPAID
*
* creditCard
*
* - OTHER
* - CREDIT
* - STORE
* - CHARGE
* - OTHER
*
* investment (SN 1.0)
*
*
* - BROKERAGE_MARGIN
* - HSA
* - IRA
* - BROKERAGE_CASH
* - 401K
* - 403B
* - TRUST
* - ANNUITY
* - SIMPLE
* - CUSTODIAL
* - BROKERAGE_CASH_OPTION
* - BROKERAGE_MARGIN_OPTION
* - INDIVIDUAL
* - CORPORATE
* - JTTIC
* - JTWROS
* - COMMUNITY_PROPERTY
* - JOINT_BY_ENTIRETY
* - CONSERVATORSHIP
* - ROTH
* - ROTH_CONVERSION
* - ROLLOVER
* - EDUCATIONAL
* - 529_PLAN
* - 457_DEFERRED_COMPENSATION
* - 401A
* - PSP
* - MPP
* - STOCK_BASKET
* - LIVING_TRUST
* - REVOCABLE_TRUST
* - IRREVOCABLE_TRUST
* - CHARITABLE_REMAINDER
* - CHARITABLE_LEAD
* - CHARITABLE_GIFT_ACCOUNT
* - SEP
* - UTMA
* - UGMA
* - ESOPP
* - ADMINISTRATOR
* - EXECUTOR
* - PARTNERSHIP
* - SOLE_PROPRIETORSHIP
* - CHURCH
* - INVESTMENT_CLUB
* - RESTRICTED_STOCK_AWARD
* - CMA
* - EMPLOYEE_STOCK_PURCHASE_PLAN
* - PERFORMANCE_PLAN
* - BROKERAGE_LINK_ACCOUNT
* - MONEY_MARKET
* - SUPER_ANNUATION
* - REGISTERED_RETIREMENT_SAVINGS_PLAN
* - SPOUSAL_RETIREMENT_SAVINGS_PLAN
* - DEFERRED_PROFIT_SHARING_PLAN
* - NON_REGISTERED_SAVINGS_PLAN
* - REGISTERED_EDUCATION_SAVINGS_PLAN
* - GROUP_RETIREMENT_SAVINGS_PLAN
* - LOCKED_IN_RETIREMENT_SAVINGS_PLAN
* - RESTRICTED_LOCKED_IN_SAVINGS_PLAN
* - LOCKED_IN_RETIREMENT_ACCOUNT
* - REGISTERED_PENSION_PLAN
* - TAX_FREE_SAVINGS_ACCOUNT
* - LIFE_INCOME_FUND
* - REGISTERED_RETIREMENT_INCOME_FUND
* - SPOUSAL_RETIREMENT_INCOME_FUND
* - LOCKED_IN_REGISTERED_INVESTMENT_FUND
* - PRESCRIBED_REGISTERED_RETIREMENT_INCOME_FUND
* - GUARANTEED_INVESTMENT_CERTIFICATES
* - REGISTERED_DISABILITY_SAVINGS_PLAN
* - DIGITAL_WALLET
* - OTHER
*
* investment (SN 2.0)
*
* - BROKERAGE_CASH
* - BROKERAGE_MARGIN
* - INDIVIDUAL_RETIREMENT_ACCOUNT_IRA
* - EMPLOYEE_RETIREMENT_ACCOUNT_401K
* - EMPLOYEE_RETIREMENT_SAVINGS_PLAN_403B
* - TRUST
* - ANNUITY
* - SIMPLE_IRA
* - CUSTODIAL_ACCOUNT
* - BROKERAGE_CASH_OPTION
* - BROKERAGE_MARGIN_OPTION
* - INDIVIDUAL
* - CORPORATE_INVESTMENT_ACCOUNT
* - JOINT_TENANTS_TENANCY_IN_COMMON_JTIC
* - JOINT_TENANTS_WITH_RIGHTS_OF_SURVIVORSHIP_JTWROS
* - JOINT_TENANTS_COMMUNITY_PROPERTY
* - JOINT_TENANTS_TENANTS_BY_ENTIRETY
* - CONSERVATOR
* - ROTH_IRA
* - ROTH_CONVERSION
* - ROLLOVER_IRA
* - EDUCATIONAL
* - EDUCATIONAL_SAVINGS_PLAN_529
* - DEFERRED_COMPENSATION_PLAN_457
* - MONEY_PURCHASE_RETIREMENT_PLAN_401A
* - PROFIT_SHARING_PLAN
* - MONEY_PURCHASE_PLAN
* - STOCK_BASKET_ACCOUNT
* - LIVING_TRUST
* - REVOCABLE_TRUST
* - IRREVOCABLE_TRUST
* - CHARITABLE_REMAINDER_TRUST
* - CHARITABLE_LEAD_TRUST
* - CHARITABLE_GIFT_ACCOUNT
* - SEP_IRA
* - UNIFORM_TRANSFER_TO_MINORS_ACT_UTMA
* - UNIFORM_GIFT_TO_MINORS_ACT_UGMA
* - EMPLOYEE_STOCK_OWNERSHIP_PLAN_ESOP
* - ADMINISTRATOR
* - EXECUTOR
* - PARTNERSHIP
* - PROPRIETORSHIP
* - CHURCH_ACCOUNT
* - INVESTMENT_CLUB
* - RESTRICTED_STOCK_AWARD
* - CASH_MANAGEMENT_ACCOUNT
* - EMPLOYEE_STOCK_PURCHASE_PLAN_ESPP
* - PERFORMANCE_PLAN
* - BROKERAGE_LINK_ACCOUNT
* - MONEY_MARKET_ACCOUNT
* - SUPERANNUATION
* - REGISTERED_RETIREMENT_SAVINGS_PLAN_RRSP
* - SPOUSAL_RETIREMENT_SAVINGS_PLAN_SRSP
* - DEFERRED_PROFIT_SHARING_PLAN_DPSP
* - NON_REGISTERED_SAVINGS_PLAN_NRSP
* - REGISTERED_EDUCATION_SAVINGS_PLAN_RESP
* - GROUP_RETIREMENT_SAVINGS_PLAN_GRSP
* - LOCKED_IN_RETIREMENT_SAVINGS_PLAN_LRSP
* - RESTRICTED_LOCKED_IN_SAVINGS_PLAN_RLSP
* - LOCKED_IN_RETIREMENT_ACCOUNT_LIRA
* - REGISTERED_PENSION_PLAN_RPP
* - TAX_FREE_SAVINGS_ACCOUNT_TFSA
* - LIFE_INCOME_FUND_LIF
* - REGISTERED_RETIREMENT_INCOME_FUND_RIF
* - SPOUSAL_RETIREMENT_INCOME_FUND_SRIF
* - LOCKED_IN_REGISTERED_INVESTMENT_FUND_LRIF
* - PRESCRIBED_REGISTERED_RETIREMENT_INCOME_FUND_PRIF
* - GUARANTEED_INVESTMENT_CERTIFICATES_GIC
* - REGISTERED_DISABILITY_SAVINGS_PLAN_RDSP
* - DEFINED_CONTRIBUTION_PLAN
* - DEFINED_BENEFIT_PLAN
* - EMPLOYEE_STOCK_OPTION_PLAN
* - NONQUALIFIED_DEFERRED_COMPENSATION_PLAN_409A
* - KEOGH_PLAN
* - EMPLOYEE_RETIREMENT_ACCOUNT_ROTH_401K
* - DEFERRED_CONTINGENT_CAPITAL_PLAN_DCCP
* - EMPLOYEE_BENEFIT_PLAN
* - EMPLOYEE_SAVINGS_PLAN
* - HEALTH_SAVINGS_ACCOUNT_HSA
* - COVERDELL_EDUCATION_SAVINGS_ACCOUNT_ESA
* - TESTAMENTARY_TRUST
* - ESTATE
* - GRANTOR_RETAINED_ANNUITY_TRUST_GRAT
* - ADVISORY_ACCOUNT
* - NON_PROFIT_ORGANIZATION_501C
* - HEALTH_REIMBURSEMENT_ARRANGEMENT_HRA
* - INDIVIDUAL_SAVINGS_ACCOUNT_ISA
* - CASH_ISA
* - STOCKS_AND_SHARES_ISA
* - INNOVATIVE_FINANCE_ISA
* - JUNIOR_ISA
* - EMPLOYEES_PROVIDENT_FUND_ORGANIZATION_EPFO
* - PUBLIC_PROVIDENT_FUND_PPF
* - EMPLOYEES_PENSION_SCHEME_EPS
* - NATIONAL_PENSION_SYSTEM_NPS
* - INDEXED_ANNUITY
* - ANNUITIZED_ANNUITY
* - VARIABLE_ANNUITY
* - ROTH_403B
* - SPOUSAL_IRA
* - SPOUSAL_ROTH_IRA
* - SARSEP_IRA
* - SUBSTANTIALLY_EQUAL_PERIODIC_PAYMENTS_SEPP
* - OFFSHORE_TRUST
* - IRREVOCABLE_LIFE_INSURANCE_TRUST
* - INTERNATIONAL_TRUST
* - LIFE_INTEREST_TRUST
* - EMPLOYEE_BENEFIT_TRUST
* - PRECIOUS_METAL_ACCOUNT
* - INVESTMENT_LOAN_ACCOUNT
* - GRANTOR_RETAINED_INCOME_TRUST
* - PENSION_PLAN
* - DIGITAL_WALLET
* - OTHER
*
* loan
*
* - MORTGAGE
* - INSTALLMENT_LOAN
* - PERSONAL_LOAN
* - HOME_EQUITY_LINE_OF_CREDIT
* - LINE_OF_CREDIT
* - AUTO_LOAN
* - STUDENT_LOAN
* - HOME_LOAN
*
* insurance
*
* - AUTO_INSURANCE
* - HEALTH_INSURANCE
* - HOME_INSURANCE
* - LIFE_INSURANCE
* - ANNUITY
* - TRAVEL_INSURANCE
* - INSURANCE
*
* realEstate
*
*
* - REAL_ESTATE
*
* reward
*
* - REWARD_POINTS
*
* Manual Account Type
* bank
*
* - CHECKING
* - SAVINGS
* - CD
* - PREPAID
*
* credit
*
*
* - CREDIT
*
* loan
*
*
* - PERSONAL_LOAN
* - HOME_LOAN
*
* insurance
*
* - INSURANCE
* - ANNUITY
*
* investment
*
* - BROKERAGE_CASH
*
*
*
* Aggregated / Manual: Both
* Applicable containers: All containers
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return accountType
*/
public String getAccountType() {
return accountType;
}
/**
* The name or identification of the account owner, as it appears at the FI site.
* Note: The account holder name can be full or partial based on how it is displayed in the account summary
* page of the FI site. In most cases, the FI site does not display the full account holder name in the account
* summary page.
*
* Aggregated / Manual: Aggregated
* Applicable containers: bank, creditCard, investment, insurance, loan, reward
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return displayedName
*/
public String getDisplayedName() {
return displayedName;
}
/**
* The date on which the account is created in the Yodlee system.
* Additional Details: It is the date when the user links or aggregates the account(s) that are held with the
* provider to the Yodlee system.
*
* Aggregated / Manual: Both
* Applicable containers: All containers
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return createdDate
*/
public String getCreatedDate() {
return createdDate;
}
/**
* A unique ID that the provider site has assigned to the account. The source ID is only available for the HELD
* accounts.
*
* Applicable containers: bank, creditCard, investment, insurance, loan, reward
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
* - GET dataExtracts/userData
*
*
* @return the sourceId
*/
public String getSourceId() {
return sourceId;
}
/**
* Logical grouping of dataset attributes into datasets such as Basic Aggregation Data, Account Profile and
* Documents.
*
* Aggregated / Manual: Aggregated
* Applicable containers: All containers
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return dataset
*/
@JsonProperty("dataset")
public List getDatasets() {
return datasets == null ? null : Collections.unmodifiableList(datasets);
}
/**
* The amount borrowed from the 401k account.
* Note: The 401k loan field is only applicable to the 401k account type.
* Applicable containers: investment
* Aggregated / Manual: Aggregated
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return 401kLoan
*/
@JsonProperty("401kLoan")
public Money getLoan401k() {
return loan401k;
}
/**
* Indicates the contract value of the annuity.
* Note: The annuity balance field is applicable only to annuities.
* Applicable containers: insurance, investment
* Aggregated / Manual: Both
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return annuityBalance
*/
public Money getAnnuityBalance() {
return annuityBalance;
}
/**
* Interest paid from the start of the year to date.
* Applicable containers: loan
* Aggregated / Manual: Aggregated
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return interestPaidYTD
*/
public Money getInterestPaidYTD() {
return interestPaidYTD;
}
/**
* Interest paid in last calendar year.
* Applicable containers: loan
* Aggregated / Manual: Aggregated
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return interestPaidLastYear
*/
public Money getInterestPaidLastYear() {
return interestPaidLastYear;
}
/**
* The type of the interest rate, for example, fixed or variable.
* Applicable containers: loan
* Aggregated / Manual: Aggregated
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
* Applicable Values
*
* @return interestRateType
*/
public LoanInterestRateType getInterestRateType() {
return interestRateType;
}
/**
* Property or possession offered to support a loan that can be seized on a default.
* Applicable containers: loan
* Aggregated / Manual: Aggregated
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return collateral
*/
public String getCollateral() {
return collateral;
}
/**
* Annual percentage yield (APY) is a normalized representation of an interest rate, based on a compounding period
* of one year. APY generally refers to the rate paid to a depositor by a financial institution on an account.
* Applicable containers: bank
* Aggregated / Manual: Aggregated
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return annualPercentageYield
*/
public Double getAnnualPercentageYield() {
return annualPercentageYield;
}
/**
* The financial cost that the policyholder pays to the insurance company to obtain an insurance cover. The premium
* is paid as a lump sum or in installments during the duration of the policy.
* Applicable containers: insurance
* Aggregated / Manual: Aggregated
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return premium
*/
public Money getPremium() {
return premium;
}
/**
* The sum of the future payments due to be paid to the insurance company during a policy year. It is the policy
* rate minus the payments made till date.
* Note: The remaining balance field is applicable only to auto insurance and home insurance.
* Applicable containers: insurance
* Aggregated / Manual: Aggregated
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return remainingBalance
*/
public Money getRemainingBalance() {
return remainingBalance;
}
/**
* The date on which the insurance policy coverage commences.
* Applicable containers: insurance
* Aggregated / Manual: Aggregated
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return policyEffectiveDate
*/
public String getPolicyEffectiveDate() {
return policyEffectiveDate;
}
/**
* The date the insurance policy began.
* Aggregated / Manual: Aggregated
* Applicable containers: insurance
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return policyFromDate
*/
public String getPolicyFromDate() {
return policyFromDate;
}
/**
* The date to which the policy exists.
* Aggregated / Manual: Aggregated
* Applicable containers: insurance
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return policyToDate
*/
public String getPolicyToDate() {
return policyToDate;
}
/**
* The death benefit amount on a life insurance policy and annuity. It is usually equal to the face amount of the
* policy, but sometimes can vary for a whole life and universal life insurance policies.
* Note: The death benefit amount field is applicable only to annuities and life insurance.
* Aggregated / Manual: Aggregated
* Applicable containers: insurance
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return deathBenefit
*/
public Money getDeathBenefit() {
return deathBenefit;
}
/**
* The duration for which the policy is valid or in effect. For example, one year, five years, etc.
* Aggregated / Manual: Aggregated
* Applicable containers: insurance
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return policyTerm
*/
public String getPolicyTerm() {
return policyTerm;
}
/**
* The status of the policy.
* Aggregated / Manual: Aggregated
* Applicable containers: insurance
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
* Applicable Values
*
* @return policyStatus
*/
public PolicyStatus getPolicyStatus() {
return policyStatus;
}
/**
* The annual percentage rate (APR) is the yearly rate of interest on the credit card account.
* Additional Details: The yearly percentage rate charged when a balance is held on a credit card. This rate
* of interest is applied every month on the outstanding credit card balance.
* Aggregated / Manual: Aggregated
* Applicable containers: creditCard
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return apr
*/
public Double getApr() {
return apr;
}
/**
* Derived APR will be an estimated purchase APR based on consumers credit card transactions and credit card
* purchase.
* Aggregated / Manual / Derived: Derived
* Applicable containers: creditCard
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
* - GET dataExtracts/userData
*
*
* @return derivedApr
*/
public Double getDerivedApr() {
return derivedApr;
}
/**
* Annual percentage rate applied to cash withdrawals on the card.
*
* Account Type: Aggregated
* Applicable containers: creditCard
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
* - GET dataExtracts/userData
*
*
* @return cashApr
*/
public Double getCashApr() {
return cashApr;
}
/**
* The amount that is available for an ATM withdrawal, i.e., the cash available after deducting the amount that is
* already withdrawn from the total cash limit. (totalCashLimit-cashAdvance= availableCash)
* Additional Details: The available cash amount at the account-level can differ from the available cash at
* the statement-level, as the information in the aggregated card account data provides more up-to-date
* information.
* Aggregated / Manual: Aggregated
* Applicable containers: creditCard
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return availableCash
*/
public Money getAvailableCash() {
return availableCash;
}
/**
*
* Credit Card: Amount that is available to spend on the credit card. It is usually the Total credit line-
* Running balance- pending charges.
* Loan: The unused portion of line of credit, on a revolving loan (such as a home-equity line of credit).
*
* Additional Details:
* Note: The available credit amount at the account-level can differ from the available credit field at the
* statement-level, as the information in the aggregated card account data provides more up-to-date information.
*
* Aggregated / Manual: Aggregated
* Applicable containers: creditCard, loan
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return availableCredit
*/
public Money getAvailableCredit() {
return availableCredit;
}
/**
* The amount that is available for immediate withdrawal or the total amount available to purchase securities in a
* brokerage or investment account.
* Note: The cash balance field is only applicable to brokerage related accounts.
*
* Aggregated / Manual: Aggregated
* Applicable containers: investment
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return cash
*/
public Money getCash() {
return cash;
}
/**
* The amount of cash value available in the consumer's life insurance policy account - except for term insurance
* policy - for withdrawals, loans, etc. This field is also used to capture the cash value on the home insurance
* policy.It is the standard that the insurance company generally prefer to reimburse the policyholder for his or
* her loss, i.e., the cash value is equal to the replacement cost minus depreciation. The cash value is also
* referred to as surrender value in India for life insurance policies.
* Note: The cash value field is applicable to all types of life insurance (except for term life) and home
* insurance.
*
* Aggregated / Manual: Aggregated
* Applicable containers: insurance
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return cashValue
*/
public Money getCashValue() {
return cashValue;
}
/**
* The classification of the account such as personal, corporate, etc.
*
* Aggregated / Manual: Aggregated
* Applicable containers: bank, creditCard, investment, reward, loan, insurance
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
* Applicable Values
*
* @return classification
*/
public AccountClassification getClassification() {
return classification;
}
/**
* The date on which the insurance policy expires or matures.
* Additional Details: The due date at the account-level can differ from the due date field at the
* statement-level, as the information in the aggregated card account data provides an up-to-date information to the
* consumer.
*
* Aggregated / Manual: Aggregated
* Applicable containers: insurance
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return expirationDate
*/
public String getExpirationDate() {
return expirationDate;
}
/**
* The amount stated on the face of a consumer's policy that will be paid in the event of his or her death or any
* other event as stated in the insurance policy. The face amount is also referred to as the sum insured or maturity
* value in India.
* Note: The face amount field is applicable only to life insurance.
*
* Aggregated / Manual: Aggregated
* Applicable containers: insurance
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return faceAmount
*/
public Money getFaceAmount() {
return faceAmount;
}
/**
*
* Bank: The interest rate offered by a FI to its depositors on a bank account.
* Loan: Interest rate applied on the loan. *
* Additional Details:
* Note: The Interest Rate field is only applicable for the following account types: savings, checking, money
* market, and certificate of deposit.
*
* Aggregated / Manual: Aggregated
* Applicable containers: bank, loan
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return interestRate
*/
public Double getInterestRate() {
return interestRate;
}
/**
* Last/Previous payment amount on the account. Portion of the principal and interest paid on previous month or
* period to satisfy a loan.
* Additional Details: If the payment is already done for the current billing cycle, then the field indicates
* the payment of the current billing cycle. If payment is yet to be done for the current billing cycle, then the
* field indicates the payment that was made for any of the previous billing cycles.
*
* Aggregated / Manual: Aggregated
* Applicable containers: creditCard, loan, insurance
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return lastPaymentAmount
*/
public Money getLastPaymentAmount() {
return lastPaymentAmount;
}
/**
* The date on which the payment for the previous or current billing cycle is done.
* Additional Details: If the payment is already done for the current billing cycle, then the field indicates
* the payment date of the current billing cycle. If payment is yet to be done for the current billing cycle, then
* the field indicates the date on which the payment was made for any of the previous billing cycles. The last
* payment date at the account-level can differ from the last payment date at the statement-level, as the
* information in the aggregated card account data provides an up-to-date information to the consumer.
*
* Aggregated / Manual: Aggregated
* Applicable containers: creditCard, loan, insurance
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return lastPaymentDate
*/
public String getLastPaymentDate() {
return lastPaymentDate;
}
/**
* The amount of borrowed funds used to purchase securities.
* Note: Margin balance is displayed only if the brokerage account is approved for margin. The margin balance
* field is only applicable to brokerage related accounts.
*
* Aggregated / Manual: Aggregated
* Applicable containers: investment
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return marginBalance
*/
public Money getMarginBalance() {
return marginBalance;
}
/**
* The maturity amount on the CD is the amount(principal and interest) paid on or after the maturity date.
* Additional Details: The Maturity Amount field is only applicable for the account type CD(Fixed Deposits).
*
*
* Aggregated / Manual: Aggregated
* Applicable containers: bank
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return maturityAmount
*/
public Money getMaturityAmount() {
return maturityAmount;
}
/**
* The date when a certificate of deposit (CD/FD) matures or the final payment date of a loan at which point the
* principal amount (including pending interest) is due to be paid.
* Additional Details: The date when a certificate of deposit (CD) matures, i.e., the money in the CD can be
* withdrawn without paying an early withdrawal penalty. The final payment date of a loan, i.e., the principal
* amount (including pending interest) is due to be paid.
*
* Aggregated / Manual: Aggregated
* Applicable containers: bank, loan
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return maturityDate
*/
public String getMaturityDate() {
return maturityDate;
}
/**
* The amount in the money market fund or its equivalent such as bank deposit programs.
* Note: The money market balance field is only applicable to brokerage related accounts.
*
* Aggregated / Manual: Aggregated
* Applicable containers: investment
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return moneyMarketBalance
*/
public Money getMoneyMarketBalance() {
return moneyMarketBalance;
}
/**
* The nickname of the account as provided by the consumer to identify an account. The account nickname can be used
* instead of the account name. *
*
* Aggregated / Manual: Both
* Applicable containers: All containers
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return nickname
*/
public String getNickname() {
return nickname;
}
/**
* The amount that is currently owed on the credit card account.
*
* Aggregated / Manual: Aggregated
* Applicable containers: creditCard
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return runningBalance
*/
public Money getRunningBalance() {
return runningBalance;
}
/**
* The maximum amount that can be withdrawn from an ATM using the credit card. Credit cards issuer allow cardholders
* to withdraw cash using their cards - the cash limit is a percent of the overall credit limit.
*
* Aggregated / Manual: Aggregated
* Applicable containers: creditCard
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return totalCashLimit
*/
public Money getTotalCashLimit() {
return totalCashLimit;
}
/**
* Total credit line is the amount of money that can be charged to a credit card. If credit limit of $5,000 is
* issued on a credit card, the total charges on the card cannot exceed this amount.
*
* Aggregated / Manual: Aggregated
* Applicable containers: creditCard
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return totalCreditLine
*/
public Money getTotalCreditLine() {
return totalCreditLine;
}
/**
* The total unvested balance that appears in an investment account. Such as the 401k account or the equity award
* account that includes employer provided funding.
* Note: The amount the employer contributes is generally subject to vesting and remain unvested for a
* specific period of time or until fulfillment of certain conditions. The total unvested balance field is only
* applicable to the retirement related accounts such as 401k, equity awards, etc.
*
* Aggregated / Manual: Aggregated
* Applicable containers: investment
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return totalUnvestedBalance
*/
public Money getTotalUnvestedBalance() {
return totalUnvestedBalance;
}
/**
* The total vested balance that appears in an investment account. Such as the 401k account or the equity award
* account that includes employer provided funding.
* Note: The amount an employee can claim after he or she leaves the organization. The total vested balance
* field is only applicable to the retirement related accounts such as 401k, equity awards, etc.
*
* Aggregated / Manual: Aggregated
* Applicable containers: investment
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return totalVestedBalance
*/
public Money getTotalVestedBalance() {
return totalVestedBalance;
}
/**
* The amount a mortgage company holds to pay a consumer's non-mortgage related expenses like insurance and property
* taxes.
* Additional Details:
* Note: The escrow balance field is only applicable to the mortgage account type.
*
* Aggregated / Manual: Aggregated
* Applicable containers: loan
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return escrowBalance
*/
public Money getEscrowBalance() {
return escrowBalance;
}
/**
* Type of home insurance, like -
*
* - HOME_OWNER
* - RENTAL
* - RENTER
* - etc..
*
* Aggregated / Manual: Aggregated
* Applicable containers: insurance
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
* Applicable Values
*
* @return homeInsuranceType
*/
public HomeInsuranceType getHomeInsuranceType() {
return homeInsuranceType;
}
/**
* Type of life insurance.
*
* Aggregated / Manual: Aggregated
* Applicable containers: insurance
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
* Applicable Values
*
* @return lifeInsuranceType
*/
public LifeInsuranceType getLifeInsuranceType() {
return lifeInsuranceType;
}
/**
* The amount of loan that the lender has provided.
*
* Aggregated / Manual: Aggregated
* Applicable containers: loan
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return originalLoanAmount
*/
public Money getOriginalLoanAmount() {
return originalLoanAmount;
}
/**
* The principal or loan balance is the outstanding balance on a loan account, excluding the interest and fees. The
* principal balance is the original borrowed amount plus any applicable loan fees, minus any principal payments.
*
*
* Aggregated / Manual: Aggregated
* Applicable containers: loan
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return principalBalance
*/
public Money getPrincipalBalance() {
return principalBalance;
}
/**
* The number of years for which premium payments have to be made in a policy.
*
* Aggregated / Manual: Aggregated
* Applicable containers: insurance
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return premiumPaymentTerm
*/
public String getPremiumPaymentTerm() {
return premiumPaymentTerm;
}
/**
* The monthly or periodic payment on a loan that is recurring in nature. The recurring payment amount is usually
* same as the amount due, unless late fees or other charges are added eventually changing the amount due for a
* particular month.
*
* Aggregated / Manual: Aggregated
* Applicable containers: loan
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return recurringPayment
*/
public Money getRecurringPayment() {
return recurringPayment;
}
/**
* The tenure for which the CD account is valid or in case of loan, the number of years/months over which the loan
* amount has to be repaid.
* Additional Details:
* Bank: The Term field is only applicable for the account type CD. Loan: The period for which the loan agreement is
* in force. The period, before or at the end of which, the loan should either be repaid or renegotiated for another
* term.
*
* Aggregated / Manual: Aggregated
* Applicable containers: bank, loan
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return term
*/
public String getTerm() {
return term;
}
/**
* The maximum amount of credit a financial institution extends to a consumer through a line of credit or a
* revolving loan like HELOC.
* Additional Details:
* Note: The credit limit field is applicable only to LOC and HELOC account types.
*
* Aggregated / Manual: Aggregated
* Applicable containers: loan
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return totalCreditLimit
*/
public Money getTotalCreditLimit() {
return totalCreditLimit;
}
/**
* Date on which the user is enrolled on the rewards program.
*
* Aggregated / Manual: Aggregated
* Applicable containers: reward
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return enrollmentDate
*/
public String getEnrollmentDate() {
return enrollmentDate;
}
/**
* Primary reward unit for this reward program. E.g. miles, points, etc.
*
* Aggregated / Manual: Aggregated
* Applicable containers: reward
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return primaryRewardUnit
*/
public String getPrimaryRewardUnit() {
return primaryRewardUnit;
}
/**
* Current level of the reward program the user is associated with. E.g. Silver, Jade etc.
*
* Aggregated / Manual: Aggregated
* Applicable containers: reward
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return currentLevel
*/
public String getCurrentLevel() {
return currentLevel;
}
/**
* The eligible next level of the rewards program.
*
* Aggregated / Manual: Aggregated
* Applicable containers: reward
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return nextLevel
*/
public String getNextLevel() {
return nextLevel;
}
/**
* The sum of the current market values of short positions held in a brokerage account.
* Note: The short balance balance field is only applicable to brokerage related accounts.
*
* Aggregated / Manual: Aggregated
* Applicable containers: investment
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return shortBalance
*/
public Money getShortBalance() {
return shortBalance;
}
/**
* Indicates the last amount contributed by the employee to the 401k account.
* Note: The last employee contribution amount field is derived from the transaction data and not aggregated
* from the FI site. The field is only applicable to the 401k account type.
*
* Aggregated / Manual: Aggregated
* Applicable containers: investment
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return lastEmployeeContributionAmount
*/
public Money getLastEmployeeContributionAmount() {
return lastEmployeeContributionAmount;
}
/**
* The date on which the last employee contribution was made to the 401k account.
* Note: The last employee contribution date field is derived from the transaction data and not aggregated
* from the FI site. The field is only applicable to the 401k account type.
*
* Aggregated / Manual: Aggregated
* Applicable containers: investment
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return lastEmployeeContributionDate
*/
public String getLastEmployeeContributionDate() {
return lastEmployeeContributionDate;
}
/**
* The additional description or notes given by the user.
*
* Aggregated / Manual: Both
* Applicable containers: All containers
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return memo
*/
public String getMemo() {
return memo;
}
/**
* The date on which the loan is disbursed.
*
* Aggregated / Manual: Both
* Applicable containers: loan
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return originationDate
*/
public String getOriginationDate() {
return originationDate;
}
/**
* The overdraft Limit for the account.
* Note: The overdraft Limit is provided only for AUS, INDIA, UK, NZ locales.
*
* Aggregated / Manual: Aggregated
* Applicable containers: bank
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return overDraftLimit
*/
public Money getOverDraftLimit() {
return overDraftLimit;
}
/**
* The valuation Type indicates whether the home value is calculated either manually or by Yodlee Partners.
*
* Aggregated / Manual: Manual
* Applicable containers: realEstate
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
* Applicable Values
*
* @return valuationType
*/
public ValuationType getValuationType() {
return valuationType;
}
/**
* The home value of the real estate account.
*
* Aggregated / Manual: Manual
* Applicable containers: realEstate
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return homeValue
*/
public Money getHomeValue() {
return homeValue;
}
/**
* The date on which the home value was estimated.
*
* Aggregated / Manual: Manual
* Applicable containers: realEstate
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return estimatedDate
*/
public String getEstimatedDate() {
return estimatedDate;
}
/**
* The home address of the real estate account. The address entity for home address consists of state, zip and city
* only
*
* Aggregated / Manual: Manual
* Applicable containers: realEstate
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return address
*/
public AccountAddress getAddress() {
return address;
}
/**
* The amount to be paid to close the loan account, i.e., the total amount required to meet a borrower's obligation
* on a loan.
*
* Aggregated / Manual: Aggregated
* Applicable containers: loan
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return loanPayoffAmount
*/
public Money getLoanPayoffAmount() {
return loanPayoffAmount;
}
/**
* Bank and branch identification information.
* Aggregated / Manual: Aggregated
* Applicable containers: bank, investment, loan
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return bankTransferCode
*/
@JsonProperty("bankTransferCode")
public List getBankTransferCode() {
return bankTransferCode == null ? null : Collections.unmodifiableList(bankTransferCode);
}
/**
* Information of different reward balances associated with the account.
*
* Aggregated / Manual: Aggregated
* Applicable containers: reward
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return rewardBalance
*/
public List getRewardBalance() {
return rewardBalance == null ? null : Collections.unmodifiableList(rewardBalance);
}
/**
* The date by which the payoff amount should be paid.
*
* Aggregated / Manual: Aggregated
* Applicable containers: loan
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return loanPayByDate
*/
public String getLoanPayByDate() {
return loanPayByDate;
}
/**
* The coverage-related details of the account.
*
* Aggregated / Manual: Aggregated
* Applicable containers: insurance,investment
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return coverage
*/
public List getCoverage() {
return coverage == null ? null : Collections.unmodifiableList(coverage);
}
/**
* The account name as it appears at the site.
* (The POST accounts service response return this field as name)
* Applicable containers: All Containers
* Aggregated / Manual: Both
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return accountName
*/
public String getAccountName() {
return accountName;
}
/**
* The last payment made for the account.
* Aggregated / Manual: Aggregated
* Applicable containers: bill
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return lastPayment
*/
public Money getLastPayment() {
return lastPayment;
}
/**
* Indicates the status of the loan account.
*
* Aggregated / Manual: Aggregated
* Applicable containers: loan
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
* Applicable Values:
*
* @return sourceAccountStatus
*/
public SourceAccountStatus getSourceAccountStatus() {
return sourceAccountStatus;
}
/**
* The type of repayment plan that the borrower prefers to repay the loan.
*
* Aggregated / Manual: Aggregated
* Applicable containers: loan
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
* Applicable Values:
*
* @return repaymentPlanType
*/
public LoanRepaymentPlanType getRepaymentPlanType() {
return repaymentPlanType;
}
/**
* A nonprofit or state organization that works with lender, servicer, school, and the Department of Education to
* help successfully repay Federal Family Education Loan Program (FFELP) loans. If FFELP student loans default, the
* guarantor takes ownership of them.
*
* Aggregated / Manual: Aggregated
* Applicable containers: loan
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return guarantor
*/
public String getGuarantor() {
return guarantor;
}
/**
* The financial institution that provides the loan.
*
* Aggregated / Manual: Aggregated
* Applicable containers: loan
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return lender
*/
public String getLender() {
return lender;
}
@ApiModelProperty(readOnly = true,
value = "Indicates the migration status of the account from screen-scraping provider to the Open Banking provider. "//
+ "
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("oauthMigrationStatus")
protected OpenBankingMigrationStatusType openBankingMigrationStatusType;
/**
* Indicates the migration status of the account from screen-scraping provider to the Open Banking provider.
*
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
* - GET dataExtracts/userData
*
*
* @return OpenBankingMigrationStatusType
*/
@JsonProperty("oauthMigrationStatus")
public OpenBankingMigrationStatusType getOpenBankingMigrationStatusType() {
return openBankingMigrationStatusType;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy