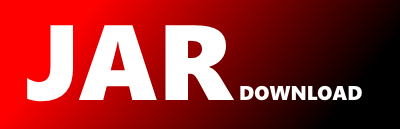
com.yodlee.api.model.account.PaymentProfile Maven / Gradle / Ivy
/**
* Copyright (c) 2019 Yodlee, Inc. All Rights Reserved.
*
* Licensed under the MIT License. See LICENSE file in the project root for license information.
*/
package com.yodlee.api.model.account;
import java.util.Collections;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.yodlee.api.model.AbstractModelComponent;
import io.swagger.annotations.ApiModelProperty;
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({"address", "identifier", "paymentBankTransferCode"})
public class PaymentProfile extends AbstractModelComponent {
@ApiModelProperty(value = "The address of the lender to which the monthly payments or the loan payoff amount should be paid. "
+ "
Additional Details:"//
+ "The address field applies only to the student loan account type.
"//
+ "Account Type: Aggregated
"//
+ "Applicable containers: loan
"//
+ "Endpoints:
"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "
")
@JsonProperty("address")
private List addresses;
@ApiModelProperty(value = "The additional information such as platform code or payment reference number that is required to make payments."//
+ "
Additional Details:" + "The identifier field applies only to the student loan account type."//
+ "
"//
+ "Account Type: Aggregated
"//
+ "Applicable containers: loan
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "
")
@JsonProperty("identifier")
private PaymentIdentifier identifier;
@ApiModelProperty(value = "The additional information for payment bank transfer code."//
+ "
"//
+ "Applicable containers: loan
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "
")
@JsonProperty("paymentBankTransferCode")
private PaymentBankTransferCode paymentBankTransferCode;
/**
* The additional information for payment bank transfer code.
* Applicable containers: loan
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return paymentBankTransferCode
*/
public PaymentBankTransferCode getPaymentBankTransferCode() {
return paymentBankTransferCode;
}
/**
* The address of the lender to which the monthly payments or the loan payoff amount should be paid.
* Additional Details: The address field applies only to the student loan account type.
* Account Type: Aggregated
* Applicable containers: loan
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return address
*/
@JsonProperty("address")
public List getAddress() {
return addresses == null ? null : Collections.unmodifiableList(addresses);
}
/**
* The additional information such as platform code or payment reference number that is required to make payments.
*
* Additional Details: * The identifier field applies only to the student loan account type.
*
* Account Type: Aggregated
* Applicable containers: loan
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return identifier
*/
public PaymentIdentifier getIdentifier() {
return identifier;
}
@Override
public String toString() {
return "PaymentProfile [addresses=" + addresses + ", identifier=" + identifier + ", paymentBankTransferCode="
+ paymentBankTransferCode + "]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy