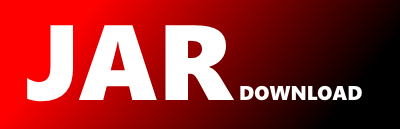
com.yodlee.api.model.account.RewardBalance Maven / Gradle / Ivy
/**
* Copyright (c) 2019 Yodlee, Inc. All Rights Reserved.
*
* Licensed under the MIT License. See LICENSE file in the project root for license information.
*/
package com.yodlee.api.model.account;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.yodlee.api.model.AbstractModelComponent;
import com.yodlee.api.model.account.enums.RewardBalanceType;
import io.swagger.annotations.ApiModelProperty;
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({"description", "balance", "units", "balanceType", "expiryDate", "balanceToLevel",
"balanceToReward"})
public class RewardBalance extends AbstractModelComponent {
@ApiModelProperty(readOnly = true,
value = "The description for the reward balance as available at provider source."//
+ "
"//
+ "Account Type: Aggregated
"//
+ "Applicable containers: reward
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("description")
private String description;
@ApiModelProperty(readOnly = true,
value = "The actual reward balance."//
+ "
"//
+ "Account Type: Aggregated
"//
+ "Applicable containers: reward
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("balance")
private Double balance;
@ApiModelProperty(readOnly = true,
value = "Unit of reward balance - miles, points, segments, dollars, credits."//
+ "
"//
+ "Account Type: Aggregated
"//
+ "Applicable containers: reward
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("units")
private String units;
@ApiModelProperty(readOnly = true,
value = "The type of reward balance."//
+ "
"//
+ "Account Type: Aggregated
"//
+ "Applicable containers: reward
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
"//
+ "Applicable Values
"//
)
@JsonProperty("balanceType")
private RewardBalanceType balanceType;
@ApiModelProperty(readOnly = true,
value = "The date on which the balance expires."//
+ "
"//
+ "Account Type: Aggregated
"//
+ "Applicable containers: reward
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("expiryDate")
private String expiryDate;
@ApiModelProperty(readOnly = true,
value = "The balance required to reach a reward level."//
+ "
"//
+ "Account Type: Aggregated
"//
+ "Applicable containers: reward
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("balanceToLevel")
private String balanceToLevel;
@ApiModelProperty(readOnly = true,
value = "The balance required to qualify for a reward such as retaining membership, business reward, etc."//
+ "
"//
+ "Account Type: Aggregated
"//
+ "Applicable containers: reward
"//
+ "Endpoints:"//
+ ""//
+ "- GET accounts
"//
+ "- GET accounts/{accountId}
"//
+ "- GET dataExtracts/userData
"//
+ "
")
@JsonProperty("balanceToReward")
private String balanceToReward;
/**
* The description for the reward balance as available at provider source.
*
* Account Type: Aggregated
* Applicable containers: reward
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return description
*/
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
/**
* The actual reward balance.
*
* Account Type: Aggregated
* Applicable containers: reward
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return balance
*/
public Double getBalance() {
return balance;
}
public void setBalance(Double balance) {
this.balance = balance;
}
/**
* Unit of reward balance - miles, points, segments, dollars, credits.
*
* Account Type: Aggregated
* Applicable containers: reward
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return units
*/
public String getUnits() {
return units;
}
public void setUnits(String units) {
this.units = units;
}
/**
* The type of reward balance.
*
* Account Type: Aggregated
* Applicable containers: reward
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
* Applicable Values
*
* @return balanceType
*/
public RewardBalanceType getBalanceType() {
return balanceType;
}
public void setBalanceType(RewardBalanceType balanceType) {
this.balanceType = balanceType;
}
/**
* The date on which the balance expires.
*
* Account Type: Aggregated
* Applicable containers: reward
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return expiryDate
*/
public String getExpiryDate() {
return expiryDate;
}
public void setExpiryDate(String expiryDate) {
this.expiryDate = expiryDate;
}
/**
* The balance required to reach a reward level.
*
* Account Type: Aggregated
* Applicable containers: reward
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return balanceToLevel
*/
public String getBalanceToLevel() {
return balanceToLevel;
}
public void setBalanceToLevel(String balanceToLevel) {
this.balanceToLevel = balanceToLevel;
}
/**
* The balance required to qualify for a reward such as retaining membership, business reward, etc.
*
* Account Type: Aggregated
* Applicable containers: reward
* Endpoints:
*
* - GET accounts
* - GET accounts/{accountId}
*
*
* @return balanceToReward
*/
public String getBalanceToReward() {
return balanceToReward;
}
public void setBalanceToReward(String balanceToReward) {
this.balanceToReward = balanceToReward;
}
@Override
public String toString() {
return "RewardBalance [description=" + description + ", balance=" + balance + ", units=" + units
+ ", balanceType=" + balanceType + ", expiryDate=" + expiryDate + ", balanceToLevel=" + balanceToLevel
+ ", balanceToReward=" + balanceToReward + "]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy