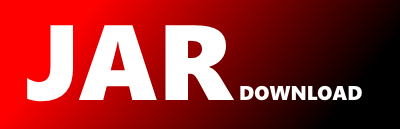
com.yodlee.sdk.api.exception.ApiException Maven / Gradle / Ivy
/**
* Copyright (c) 2019 Yodlee, Inc. All Rights Reserved.
*
* Licensed under the MIT License. See LICENSE file in the project root for license information.
*/
package com.yodlee.sdk.api.exception;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.yodlee.api.model.YodleeError;
import com.yodlee.api.model.validator.Problem;
public class ApiException extends Exception {
private static final long serialVersionUID = 755063301177528109L;
private static final Logger LOGGER = LoggerFactory.getLogger(ApiException.class);
private final boolean isClientSideError;
private final int httpStatus;
private final String responseBody;
private final transient YodleeError serverError;
private final transient List problems = new ArrayList<>();
private final transient Map> responseHeaders = new HashMap<>();
public ApiException(Throwable throwable) {
this(throwable.getMessage(), Collections.emptyList(), throwable, 0,
Collections.>emptyMap(), null, false);
}
public ApiException(String message) {
this(message, Collections.emptyList(), (Throwable) null, 0,
Collections.>emptyMap(), null, false);
}
public ApiException(List problems) {
this("Validation failed.", problems, (Throwable) null, 0, Collections.>emptyMap(), null,
true);
}
public ApiException(List problems, String message, boolean isClientSideError) {
this(message, problems, (Throwable) null, 0, null, null, isClientSideError);
}
public ApiException(String message, int httpStatus, Map> responseHeaders,
String responseBody) {
this(message, Collections.emptyList(), (Throwable) null, httpStatus, responseHeaders, responseBody,
false);
}
public ApiException(String message, Throwable throwable, int httpStatus,
Map> responseHeaders) {
this(message, Collections.emptyList(), throwable, httpStatus, responseHeaders, null, false);
}
public ApiException(String message, List problems, Throwable throwable, int httpStatus,
Map> responseHeaders, String responseBody, boolean isClientSideError) {
super(message, throwable);
this.httpStatus = httpStatus;
this.problems.addAll(problems);
this.responseHeaders.putAll(responseHeaders);
this.responseBody = responseBody;
this.isClientSideError = isClientSideError;
YodleeError yodleeError = null;
if (responseBody != null) {
ObjectMapper mapper = new ObjectMapper();
try {
yodleeError = mapper.readValue(responseBody, YodleeError.class);
} catch (IOException e) {
LOGGER.error("Exception occurred.", e);
}
}
serverError = yodleeError == null ? null : yodleeError;
}
public List getProblems() {
return Collections.unmodifiableList(problems);
}
public boolean isClientSideError() {
return isClientSideError;
}
public int getHttpStatus() {
return httpStatus;
}
public Map> getResponseHeaders() {
return Collections.unmodifiableMap(responseHeaders);
}
public String getResponseBody() {
return responseBody;
}
public YodleeError getServerError() {
return serverError;
}
private String isHttpCodeExist(int httpStatus) {
return httpStatus == 0 ? "" : (", httpStatus=" + httpStatus);
}
@Override
public String toString() {
return "ApiException [problems=" + problems + ", isClientSideError=" + isClientSideError
+ isHttpCodeExist(httpStatus) + ", responseHeaders=" + responseHeaders + ", responseBody="
+ responseBody + ", message=" + getMessage() + "]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy