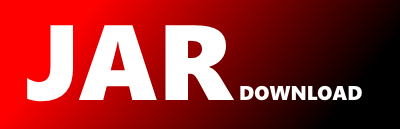
com.yoti.api.client.Attribute Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yoti-sdk-api Show documentation
Show all versions of yoti-sdk-api Show documentation
Java SDK for simple integration with the Yoti platform
The newest version!
package com.yoti.api.client;
import static com.yoti.api.client.spi.remote.util.Validation.notNull;
import java.util.Collections;
import java.util.List;
/**
* Represents a generic, typed key/value pair, a basic building block of
* {@link Profile} instances.
*/
public class Attribute {
private final String id;
private final String name;
private final T value;
private final List sources;
private final List verifiers;
private final List allAnchors;
public Attribute(String name, T value) {
this(name, value, Collections.emptyList(), Collections.emptyList(), Collections.emptyList());
}
public Attribute(String id, String name, T value) {
this(id, name, value, Collections.emptyList(), Collections.emptyList(), Collections.emptyList());
}
public Attribute(String name, T value, List sources, List verifiers, List allAnchors) {
this.id = null;
this.name = name;
this.value = value;
this.sources = notNull(sources, "sources");
this.verifiers = notNull(verifiers, "verifiers");
this.allAnchors = notNull(allAnchors, "allAnchors");
}
public Attribute(String id, String name, T value, List sources, List verifiers, List allAnchors) {
this.id = id;
this.name = name;
this.value = value;
this.sources = notNull(sources, "sources");
this.verifiers = notNull(verifiers, "verifiers");
this.allAnchors = notNull(allAnchors, "allAnchors");
}
/**
* The ID of the attribute
*
* @return attribute ID
*/
public String getId() {
return id;
}
/**
* The name of the attribute
*
* @return name of the attribute
*/
public String getName() {
return name;
}
/**
* Value of the attribute
*
* @return attribute value
*/
public T getValue() {
return value;
}
/**
* Source {@link Anchor}s identify how and when an attribute value was acquired
*
* @return List of sources for the attribute value
*/
public List getSources() {
return sources;
}
/**
* Verifier {@link Anchor}s identify how and when an attribute value was verified by another provider
*
* @return List of verifiers for the attribute value
*/
public List getVerifiers() {
return verifiers;
}
/**
* All known {@link Anchor}s for this attribute. Includes SOURCE and VERIFIER Anchors, in future may provide other types too
*
* @return All anchors returned with the attribute
*/
public List getAnchors() {
return allAnchors;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy