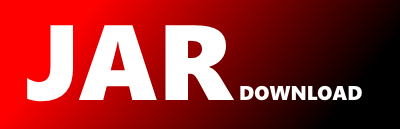
com.yoti.api.client.HumanProfile Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yoti-sdk-api Show documentation
Show all versions of yoti-sdk-api Show documentation
Java SDK for simple integration with the Yoti platform
The newest version!
package com.yoti.api.client;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.yoti.api.attributes.AttributeConstants;
public class HumanProfile extends Profile {
public static final String GENDER_MALE = "MALE";
public static final String GENDER_FEMALE = "FEMALE";
public static final String GENDER_TRANSGENDER = "TRANSGENDER";
public static final String GENDER_OTHER = "OTHER";
private Map verificationsMap;
/**
* Create a new Human profile based on a list of attributes
*
* @param attributeList list containing the attributes for this profile
*/
public HumanProfile(List> attributeList) {
super(attributeList);
}
/**
* Corresponds to primary name in passport, and surname in English.
*
* @return the family name
*/
public Attribute getFamilyName() {
return getAttribute(AttributeConstants.HumanProfileAttributes.FAMILY_NAME, String.class);
}
/**
* Corresponds to secondary names in passport, and first/middle names in English.
*
* @return the given names
*/
public Attribute getGivenNames() {
return getAttribute(AttributeConstants.HumanProfileAttributes.GIVEN_NAMES, String.class);
}
/**
* The full name attribute.
*
* @return the full name
*/
public Attribute getFullName() {
return getAttribute(AttributeConstants.HumanProfileAttributes.FULL_NAME, String.class);
}
/**
* Date of birth
*
* @return Date of birth
*/
public Attribute getDateOfBirth() {
return getAttribute(AttributeConstants.HumanProfileAttributes.DATE_OF_BIRTH, Date.class);
}
/**
* Finds all the 'Age Over' and 'Age Under' derived attributes returned with the profile, and returns them wrapped in {@link AgeVerification}
* objects
*
* @return A list of all the {@link AgeVerification} objects returned for this profile
*/
public List getAgeVerifications() {
findAllAgeVerifications();
return Collections.unmodifiableList(new ArrayList<>(verificationsMap.values()));
}
/**
* Searches for an {@link AgeVerification} corresponding to an 'Age Over' check for the given age
*
* @param age the age the search over
* @return age_over {@link AgeVerification} for the given age, or null
if no match was found
*/
public AgeVerification findAgeOverVerification(int age) {
findAllAgeVerifications();
return verificationsMap.get(AttributeConstants.HumanProfileAttributes.AGE_OVER + age);
}
/**
* Searches for an {@link AgeVerification} corresponding to an 'Age Under' check for the given age
*
* @param age the age to search under
* @return age_under {@link AgeVerification} for the given age, or null
if no match was found
*/
public AgeVerification findAgeUnderVerification(int age) {
findAllAgeVerifications();
return verificationsMap.get(AttributeConstants.HumanProfileAttributes.AGE_UNDER + age);
}
private void findAllAgeVerifications() {
if (verificationsMap == null) {
Map verifications = new HashMap<>();
for (Attribute ageOver : findAttributesStartingWith(AttributeConstants.HumanProfileAttributes.AGE_OVER, String.class)) {
verifications.put(ageOver.getName(), new AgeVerification(ageOver));
}
for (Attribute ageUnder : findAttributesStartingWith(AttributeConstants.HumanProfileAttributes.AGE_UNDER, String.class)) {
verifications.put(ageUnder.getName(), new AgeVerification(ageUnder));
}
this.verificationsMap = verifications;
}
}
/**
* Corresponds to the gender in the passport; will be one of the strings "MALE", "FEMALE", "TRANSGENDER" or "OTHER".
*
* @return the gender
*/
public Attribute getGender() {
return getAttribute(AttributeConstants.HumanProfileAttributes.GENDER, String.class);
}
/**
* The user's postal address as a String
*
* @return the postal address
*/
public Attribute getPostalAddress() {
return getAttribute(AttributeConstants.HumanProfileAttributes.POSTAL_ADDRESS, String.class);
}
/**
* The user's structured postal address as a Json
*
* @return the postal address
*/
@SuppressWarnings("unchecked")
public Attribute
© 2015 - 2025 Weber Informatics LLC | Privacy Policy