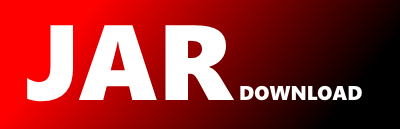
com.yoti.api.client.spi.remote.AttributeConverter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yoti-sdk-api Show documentation
Show all versions of yoti-sdk-api Show documentation
Java SDK for simple integration with the Yoti platform
The newest version!
package com.yoti.api.client.spi.remote;
import static com.yoti.api.client.spi.remote.call.YotiConstants.DEFAULT_CHARSET;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.text.ParseException;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import com.yoti.api.attributes.AttributeConstants;
import com.yoti.api.client.Anchor;
import com.yoti.api.client.Attribute;
import com.yoti.api.client.Date;
import com.yoti.api.client.Image;
import com.yoti.api.client.spi.remote.proto.AttrProto;
import com.yoti.api.client.spi.remote.proto.ContentTypeProto;
import com.yoti.api.client.spi.remote.util.AnchorType;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.google.protobuf.ByteString;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
class AttributeConverter {
private static final ObjectMapper JSON_MAPPER = new ObjectMapper();
private static final Logger LOG = LoggerFactory.getLogger(AttributeConverter.class);
private final DocumentDetailsAttributeParser documentDetailsAttributeParser;
private final AnchorConverter anchorConverter;
private AttributeConverter(DocumentDetailsAttributeParser documentDetailsAttributeParser, AnchorConverter anchorConverter) {
this.documentDetailsAttributeParser = documentDetailsAttributeParser;
this.anchorConverter = anchorConverter;
}
static AttributeConverter newInstance() {
return new AttributeConverter(new DocumentDetailsAttributeParser(), new AnchorConverter());
}
Attribute convertAttribute(AttrProto.Attribute attribute) throws ParseException, IOException {
Object value = convertValueFromProto(attribute);
value = convertSpecialType(attribute, value);
List anchors = convertAnchors(attribute);
List sources = filterAnchors(anchors, AnchorType.SOURCE.name());
List verifiers = filterAnchors(anchors, AnchorType.VERIFIER.name());
return new Attribute(emptyToNull(attribute.getEphemeralId()), attribute.getName(), value, sources, verifiers, anchors);
}
private static String emptyToNull(String value) {
return value == null || value.isEmpty() ? null : value;
}
private Object convertValueFromProto(AttrProto.Attribute attribute) throws ParseException, IOException {
return convertValue(attribute.getContentType(), attribute.getValue());
}
private Object convertValue(ContentTypeProto.ContentType contentType, ByteString value) throws ParseException, IOException {
boolean isInvalid = (contentType != ContentTypeProto.ContentType.STRING && value.isEmpty());
if (isInvalid) {
throw new ParseException("Only STRING attributes can have an empty value", 0);
}
switch (contentType) {
case STRING:
return value.toString(DEFAULT_CHARSET);
case DATE:
return Date.parseFrom(value.toByteArray());
case JPEG:
return new JpegAttributeValue(value.toByteArray());
case PNG:
return new PngAttributeValue(value.toByteArray());
case JSON:
return JSON_MAPPER.readValue(value.toString(DEFAULT_CHARSET), Map.class);
case MULTI_VALUE:
return convertMultiValue(value);
case INT:
return Integer.parseInt(value.toString(DEFAULT_CHARSET));
default:
LOG.warn("Unknown type '{}', attempting to parse it as a String", contentType);
return value.toString(DEFAULT_CHARSET);
}
}
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy