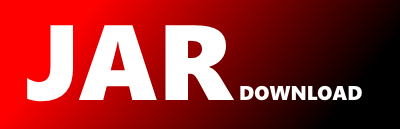
com.yoti.crypto.Crypto Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yoti-sdk-api Show documentation
Show all versions of yoti-sdk-api Show documentation
Java SDK for simple integration with the Yoti platform
The newest version!
package com.yoti.crypto;
import java.security.Key;
import java.security.PrivateKey;
import java.util.function.Supplier;
import javax.crypto.Cipher;
import javax.crypto.spec.IvParameterSpec;
import javax.crypto.spec.SecretKeySpec;
public final class Crypto {
private static final String DECRYPT_ERROR_TEMPLATE = "Failed to decrypt message using '%s' transformation";
private Crypto() { }
public static byte[] decrypt(byte[] msg, PrivateKey key, CipherType transformation) {
return wrapOnException(
() -> {
try {
return doCipher(msg, key, transformation);
} catch (Exception ex) {
throw new CryptoException(ex);
}
},
String.format(DECRYPT_ERROR_TEMPLATE, transformation.value())
);
}
private static byte[] doCipher(byte[] msg, Key key, CipherType transformation) throws Exception {
Cipher cipher = transformation.getInstance();
cipher.init(Cipher.DECRYPT_MODE, key);
return cipher.doFinal(msg);
}
public static byte[] decrypt(byte[] msg, byte[] key, IvParameterSpec iv, CipherType transformation) {
return decrypt(msg, new SecretKeySpec(key, Algorithm.AES.value()), iv, transformation);
}
public static byte[] decrypt(byte[] msg, SecretKeySpec key, IvParameterSpec iv, CipherType transformation) {
return wrapOnException(
() -> {
try {
return doCipher(msg, key, iv, transformation);
} catch (Exception ex) {
throw new CryptoException(ex);
}
},
String.format(DECRYPT_ERROR_TEMPLATE, transformation.value())
);
}
private static byte[] doCipher(byte[] msg, SecretKeySpec key, IvParameterSpec iv, CipherType transformation)
throws Exception {
Cipher cipher = transformation.getInstance();
cipher.init(Cipher.DECRYPT_MODE, key, iv);
return cipher.doFinal(msg);
}
private static T wrapOnException(Supplier op, String msg) {
try {
return op.get();
} catch (Exception ex) {
throw new CryptoException(msg, ex);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy