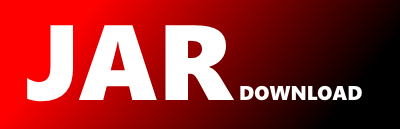
com.yoti.validation.Validation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yoti-sdk-api Show documentation
Show all versions of yoti-sdk-api Show documentation
Java SDK for simple integration with the Yoti platform
The newest version!
package com.yoti.validation;
import static java.lang.String.format;
import java.util.Collection;
import java.util.List;
public final class Validation {
private Validation() { }
public static T notNull(T value, String name) {
if (value == null) {
throw new IllegalArgumentException(format("'%s' must not be null", name));
}
return value;
}
public static boolean isNullOrEmpty(String value) {
return value == null || value.isEmpty();
}
public static void notNullOrEmpty(String value, String name) {
if (isNullOrEmpty(value)) {
throw new IllegalArgumentException(format("'%s' must not be empty or null", name));
}
}
public static void notNullOrEmpty(Collection value, String name) {
if (value == null || value.isEmpty()) {
throw new IllegalArgumentException(format("'%s' must not be empty or null", name));
}
}
public static void notEqualTo(Object value, Object notAllowed, String name) {
if (notEqualTo(value, notAllowed)) {
throw new IllegalArgumentException(format("'%s' value may not be equalTo '%s'", name, notAllowed));
}
}
private static boolean notEqualTo(Object value, Object notAllowed) {
if (notAllowed == null) {
return value == null;
}
return notAllowed.equals(value);
}
public static void notGreaterThan(T value, T limit, String name) {
if (value.compareTo(limit) > 0) {
throw new IllegalArgumentException(format("'%s' value '%s' is greater than '%s'", name, value, limit));
}
}
public static void notLessThan(T value, T limit, String name) {
if (value.compareTo(limit) < 0) {
throw new IllegalArgumentException(format("'%s' value '%s' is less than '%s'", name, value, limit));
}
}
public static void withinRange(T value, T minLimit, T maxLimit, String name) {
notLessThan(value, minLimit, name);
notGreaterThan(value, maxLimit, name);
}
public static void matchesPattern(String value, String regex, String name) {
if (value == null || ! value.matches(regex)) {
throw new IllegalArgumentException(format("'%s' value '%s' does not match format '%s'", name, value, regex));
}
}
public static void withinList(T value, List allowedValues) {
if (!allowedValues.contains(value)) {
throw new IllegalArgumentException(format("value '%s' is not in the list '%s'", value, allowedValues));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy