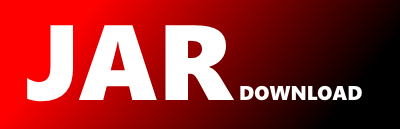
io.vertx.reactivex.redis.RedisTransaction Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.reactivex.redis;
import java.util.Map;
import io.reactivex.Observable;
import io.reactivex.Flowable;
import io.reactivex.Single;
import io.reactivex.Completable;
import io.reactivex.Maybe;
import io.vertx.redis.op.BitOperation;
import io.vertx.redis.op.ResetOptions;
import io.vertx.redis.op.ObjectCmd;
import io.vertx.redis.op.KillFilter;
import java.util.Map;
import io.vertx.redis.op.GeoUnit;
import io.vertx.core.json.JsonObject;
import io.vertx.core.AsyncResult;
import io.vertx.redis.op.RangeOptions;
import io.vertx.redis.op.GeoMember;
import io.vertx.redis.op.GeoRadiusOptions;
import io.vertx.redis.op.InsertOptions;
import io.vertx.redis.op.AggregateOptions;
import io.vertx.redis.op.SetOptions;
import io.vertx.redis.op.SortOptions;
import io.vertx.redis.op.MigrateOptions;
import io.vertx.redis.op.ScanOptions;
import io.vertx.redis.op.FailoverOptions;
import io.vertx.redis.op.SlotCmd;
import io.vertx.redis.op.RangeLimitOptions;
import io.vertx.redis.op.LimitOptions;
import io.vertx.core.json.JsonArray;
import java.util.List;
import io.vertx.reactivex.core.buffer.Buffer;
import io.vertx.core.Handler;
/**
* This Interface represents a TX
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.redis.RedisTransaction original} non RX-ified interface using Vert.x codegen.
*/
@io.vertx.lang.reactivex.RxGen(io.vertx.redis.RedisTransaction.class)
public class RedisTransaction {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
RedisTransaction that = (RedisTransaction) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final io.vertx.lang.reactivex.TypeArg __TYPE_ARG = new io.vertx.lang.reactivex.TypeArg<>(
obj -> new RedisTransaction((io.vertx.redis.RedisTransaction) obj),
RedisTransaction::getDelegate
);
private final io.vertx.redis.RedisTransaction delegate;
public RedisTransaction(io.vertx.redis.RedisTransaction delegate) {
this.delegate = delegate;
}
public io.vertx.redis.RedisTransaction getDelegate() {
return delegate;
}
/**
* Close the client - when it is fully closed the handler will be called.
* @param handler
*/
public void close(Handler> handler) {
delegate.close(handler);
}
/**
* Close the client - when it is fully closed the handler will be called.
* @return
*/
public Completable rxClose() {
return new io.vertx.reactivex.core.impl.AsyncResultCompletable(handler -> {
close(handler);
});
}
/**
* Append a value to a key
* @param key Key string
* @param value Value to append
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction append(String key, String value, Handler> handler) {
delegate.append(key, value, handler);
return this;
}
/**
* Append a value to a key
* @param key Key string
* @param value Value to append
* @return
*/
public Single rxAppend(String key, String value) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
append(key, value, handler);
});
}
/**
* Authenticate to the server
* @param password Password for authentication
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction auth(String password, Handler> handler) {
delegate.auth(password, handler);
return this;
}
/**
* Authenticate to the server
* @param password Password for authentication
* @return
*/
public Single rxAuth(String password) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
auth(password, handler);
});
}
/**
* Asynchronously rewrite the append-only file
* @param handler
* @return
*/
public RedisTransaction bgrewriteaof(Handler> handler) {
delegate.bgrewriteaof(handler);
return this;
}
/**
* Asynchronously rewrite the append-only file
* @return
*/
public Single rxBgrewriteaof() {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
bgrewriteaof(handler);
});
}
/**
* Asynchronously save the dataset to disk
* @param handler
* @return
*/
public RedisTransaction bgsave(Handler> handler) {
delegate.bgsave(handler);
return this;
}
/**
* Asynchronously save the dataset to disk
* @return
*/
public Single rxBgsave() {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
bgsave(handler);
});
}
/**
* Count set bits in a string
* @param key Key string
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction bitcount(String key, Handler> handler) {
delegate.bitcount(key, handler);
return this;
}
/**
* Count set bits in a string
* @param key Key string
* @return
*/
public Single rxBitcount(String key) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
bitcount(key, handler);
});
}
/**
* Count set bits in a string
* @param key Key string
* @param start Start index
* @param end End index
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction bitcountRange(String key, long start, long end, Handler> handler) {
delegate.bitcountRange(key, start, end, handler);
return this;
}
/**
* Count set bits in a string
* @param key Key string
* @param start Start index
* @param end End index
* @return
*/
public Single rxBitcountRange(String key, long start, long end) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
bitcountRange(key, start, end, handler);
});
}
/**
* Perform bitwise operations between strings
* @param operation Bitwise operation to perform
* @param destkey Destination key where result is stored
* @param keys List of keys on which to perform the operation
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction bitop(BitOperation operation, String destkey, List keys, Handler> handler) {
delegate.bitop(operation, destkey, keys, handler);
return this;
}
/**
* Perform bitwise operations between strings
* @param operation Bitwise operation to perform
* @param destkey Destination key where result is stored
* @param keys List of keys on which to perform the operation
* @return
*/
public Single rxBitop(BitOperation operation, String destkey, List keys) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
bitop(operation, destkey, keys, handler);
});
}
/**
* Find first bit set or clear in a string
* @param key Key string
* @param bit What bit value to look for - must be 1, or 0
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction bitpos(String key, int bit, Handler> handler) {
delegate.bitpos(key, bit, handler);
return this;
}
/**
* Find first bit set or clear in a string
* @param key Key string
* @param bit What bit value to look for - must be 1, or 0
* @return
*/
public Single rxBitpos(String key, int bit) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
bitpos(key, bit, handler);
});
}
/**
* Find first bit set or clear in a string
*
* See also bitposRange() method, which takes start, and stop offset.
* @param key Key string
* @param bit What bit value to look for - must be 1, or 0
* @param start Start offset
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction bitposFrom(String key, int bit, int start, Handler> handler) {
delegate.bitposFrom(key, bit, start, handler);
return this;
}
/**
* Find first bit set or clear in a string
*
* See also bitposRange() method, which takes start, and stop offset.
* @param key Key string
* @param bit What bit value to look for - must be 1, or 0
* @param start Start offset
* @return
*/
public Single rxBitposFrom(String key, int bit, int start) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
bitposFrom(key, bit, start, handler);
});
}
/**
* Find first bit set or clear in a string
*
* Note: when both start, and stop offsets are specified,
* behaviour is slightly different than if only start is specified
* @param key Key string
* @param bit What bit value to look for - must be 1, or 0
* @param start Start offset
* @param stop End offset - inclusive
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction bitposRange(String key, int bit, int start, int stop, Handler> handler) {
delegate.bitposRange(key, bit, start, stop, handler);
return this;
}
/**
* Find first bit set or clear in a string
*
* Note: when both start, and stop offsets are specified,
* behaviour is slightly different than if only start is specified
* @param key Key string
* @param bit What bit value to look for - must be 1, or 0
* @param start Start offset
* @param stop End offset - inclusive
* @return
*/
public Single rxBitposRange(String key, int bit, int start, int stop) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
bitposRange(key, bit, start, stop, handler);
});
}
/**
* Remove and get the first element in a list, or block until one is available
* @param key Key string identifying a list to watch
* @param seconds Timeout in seconds
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction blpop(String key, int seconds, Handler> handler) {
delegate.blpop(key, seconds, handler);
return this;
}
/**
* Remove and get the first element in a list, or block until one is available
* @param key Key string identifying a list to watch
* @param seconds Timeout in seconds
* @return
*/
public Single rxBlpop(String key, int seconds) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
blpop(key, seconds, handler);
});
}
/**
* Remove and get the first element in any of the lists, or block until one is available
* @param keys List of key strings identifying lists to watch
* @param seconds Timeout in seconds
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction blpopMany(List keys, int seconds, Handler> handler) {
delegate.blpopMany(keys, seconds, handler);
return this;
}
/**
* Remove and get the first element in any of the lists, or block until one is available
* @param keys List of key strings identifying lists to watch
* @param seconds Timeout in seconds
* @return
*/
public Single rxBlpopMany(List keys, int seconds) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
blpopMany(keys, seconds, handler);
});
}
/**
* Remove and get the last element in a list, or block until one is available
* @param key Key string identifying a list to watch
* @param seconds Timeout in seconds
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction brpop(String key, int seconds, Handler> handler) {
delegate.brpop(key, seconds, handler);
return this;
}
/**
* Remove and get the last element in a list, or block until one is available
* @param key Key string identifying a list to watch
* @param seconds Timeout in seconds
* @return
*/
public Single rxBrpop(String key, int seconds) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
brpop(key, seconds, handler);
});
}
/**
* Remove and get the last element in any of the lists, or block until one is available
* @param keys List of key strings identifying lists to watch
* @param seconds Timeout in seconds
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction brpopMany(List keys, int seconds, Handler> handler) {
delegate.brpopMany(keys, seconds, handler);
return this;
}
/**
* Remove and get the last element in any of the lists, or block until one is available
* @param keys List of key strings identifying lists to watch
* @param seconds Timeout in seconds
* @return
*/
public Single rxBrpopMany(List keys, int seconds) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
brpopMany(keys, seconds, handler);
});
}
/**
* Pop a value from a list, push it to another list and return it; or block until one is available
* @param key Key string identifying the source list
* @param destkey Key string identifying the destination list
* @param seconds Timeout in seconds
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction brpoplpush(String key, String destkey, int seconds, Handler> handler) {
delegate.brpoplpush(key, destkey, seconds, handler);
return this;
}
/**
* Pop a value from a list, push it to another list and return it; or block until one is available
* @param key Key string identifying the source list
* @param destkey Key string identifying the destination list
* @param seconds Timeout in seconds
* @return
*/
public Single rxBrpoplpush(String key, String destkey, int seconds) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
brpoplpush(key, destkey, seconds, handler);
});
}
/**
* Kill the connection of a client
* @param filter Filter options
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction clientKill(KillFilter filter, Handler> handler) {
delegate.clientKill(filter, handler);
return this;
}
/**
* Kill the connection of a client
* @param filter Filter options
* @return
*/
public Single rxClientKill(KillFilter filter) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
clientKill(filter, handler);
});
}
/**
* Get the list of client connections
* @param handler
* @return
*/
public RedisTransaction clientList(Handler> handler) {
delegate.clientList(handler);
return this;
}
/**
* Get the list of client connections
* @return
*/
public Single rxClientList() {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
clientList(handler);
});
}
/**
* Get the current connection name
* @param handler
* @return
*/
public RedisTransaction clientGetname(Handler> handler) {
delegate.clientGetname(handler);
return this;
}
/**
* Get the current connection name
* @return
*/
public Single rxClientGetname() {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
clientGetname(handler);
});
}
/**
* Stop processing commands from clients for some time
* @param millis Pause time in milliseconds
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction clientPause(long millis, Handler> handler) {
delegate.clientPause(millis, handler);
return this;
}
/**
* Stop processing commands from clients for some time
* @param millis Pause time in milliseconds
* @return
*/
public Single rxClientPause(long millis) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
clientPause(millis, handler);
});
}
/**
* Set the current connection name
* @param name New name for current connection
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction clientSetname(String name, Handler> handler) {
delegate.clientSetname(name, handler);
return this;
}
/**
* Set the current connection name
* @param name New name for current connection
* @return
*/
public Single rxClientSetname(String name) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
clientSetname(name, handler);
});
}
/**
* Assign new hash slots to receiving node.
* @param slots
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction clusterAddslots(List slots, Handler> handler) {
delegate.clusterAddslots(slots, handler);
return this;
}
/**
* Assign new hash slots to receiving node.
* @param slots
* @return
*/
public Single rxClusterAddslots(List slots) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
clusterAddslots(slots, handler);
});
}
/**
* Return the number of failure reports active for a given node.
* @param nodeId
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction clusterCountFailureReports(String nodeId, Handler> handler) {
delegate.clusterCountFailureReports(nodeId, handler);
return this;
}
/**
* Return the number of failure reports active for a given node.
* @param nodeId
* @return
*/
public Single rxClusterCountFailureReports(String nodeId) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
clusterCountFailureReports(nodeId, handler);
});
}
/**
* Return the number of local keys in the specified hash slot.
* @param slot
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction clusterCountkeysinslot(long slot, Handler> handler) {
delegate.clusterCountkeysinslot(slot, handler);
return this;
}
/**
* Return the number of local keys in the specified hash slot.
* @param slot
* @return
*/
public Single rxClusterCountkeysinslot(long slot) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
clusterCountkeysinslot(slot, handler);
});
}
/**
* Set hash slots as unbound in receiving node.
* @param slot
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction clusterDelslots(long slot, Handler> handler) {
delegate.clusterDelslots(slot, handler);
return this;
}
/**
* Set hash slots as unbound in receiving node.
* @param slot
* @return
*/
public Single rxClusterDelslots(long slot) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
clusterDelslots(slot, handler);
});
}
/**
* Set hash slots as unbound in receiving node.
* @param slots
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction clusterDelslotsMany(List slots, Handler> handler) {
delegate.clusterDelslotsMany(slots, handler);
return this;
}
/**
* Set hash slots as unbound in receiving node.
* @param slots
* @return
*/
public Single rxClusterDelslotsMany(List slots) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
clusterDelslotsMany(slots, handler);
});
}
/**
* Forces a slave to perform a manual failover of its master.
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction clusterFailover(Handler> handler) {
delegate.clusterFailover(handler);
return this;
}
/**
* Forces a slave to perform a manual failover of its master.
* @return
*/
public Single rxClusterFailover() {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
clusterFailover(handler);
});
}
/**
* Forces a slave to perform a manual failover of its master.
* @param options
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction clusterFailOverWithOptions(FailoverOptions options, Handler> handler) {
delegate.clusterFailOverWithOptions(options, handler);
return this;
}
/**
* Forces a slave to perform a manual failover of its master.
* @param options
* @return
*/
public Single rxClusterFailOverWithOptions(FailoverOptions options) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
clusterFailOverWithOptions(options, handler);
});
}
/**
* Remove a node from the nodes table.
* @param nodeId
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction clusterForget(String nodeId, Handler> handler) {
delegate.clusterForget(nodeId, handler);
return this;
}
/**
* Remove a node from the nodes table.
* @param nodeId
* @return
*/
public Single rxClusterForget(String nodeId) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
clusterForget(nodeId, handler);
});
}
/**
* Return local key names in the specified hash slot.
* @param slot
* @param count
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction clusterGetkeysinslot(long slot, long count, Handler> handler) {
delegate.clusterGetkeysinslot(slot, count, handler);
return this;
}
/**
* Return local key names in the specified hash slot.
* @param slot
* @param count
* @return
*/
public Single rxClusterGetkeysinslot(long slot, long count) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
clusterGetkeysinslot(slot, count, handler);
});
}
/**
* Provides info about Redis Cluster node state.
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction clusterInfo(Handler> handler) {
delegate.clusterInfo(handler);
return this;
}
/**
* Provides info about Redis Cluster node state.
* @return
*/
public Single rxClusterInfo() {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
clusterInfo(handler);
});
}
/**
* Returns the hash slot of the specified key.
* @param key
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction clusterKeyslot(String key, Handler> handler) {
delegate.clusterKeyslot(key, handler);
return this;
}
/**
* Returns the hash slot of the specified key.
* @param key
* @return
*/
public Single rxClusterKeyslot(String key) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
clusterKeyslot(key, handler);
});
}
/**
* Force a node cluster to handshake with another node.
* @param ip
* @param port
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction clusterMeet(String ip, long port, Handler> handler) {
delegate.clusterMeet(ip, port, handler);
return this;
}
/**
* Force a node cluster to handshake with another node.
* @param ip
* @param port
* @return
*/
public Single rxClusterMeet(String ip, long port) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
clusterMeet(ip, port, handler);
});
}
/**
* Get Cluster config for the node.
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction clusterNodes(Handler> handler) {
delegate.clusterNodes(handler);
return this;
}
/**
* Get Cluster config for the node.
* @return
*/
public Single rxClusterNodes() {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
clusterNodes(handler);
});
}
/**
* Reconfigure a node as a slave of the specified master node.
* @param nodeId
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction clusterReplicate(String nodeId, Handler> handler) {
delegate.clusterReplicate(nodeId, handler);
return this;
}
/**
* Reconfigure a node as a slave of the specified master node.
* @param nodeId
* @return
*/
public Single rxClusterReplicate(String nodeId) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
clusterReplicate(nodeId, handler);
});
}
/**
* Reset a Redis Cluster node.
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction clusterReset(Handler> handler) {
delegate.clusterReset(handler);
return this;
}
/**
* Reset a Redis Cluster node.
* @return
*/
public Single rxClusterReset() {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
clusterReset(handler);
});
}
/**
* Reset a Redis Cluster node.
* @param options
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction clusterResetWithOptions(ResetOptions options, Handler> handler) {
delegate.clusterResetWithOptions(options, handler);
return this;
}
/**
* Reset a Redis Cluster node.
* @param options
* @return
*/
public Single rxClusterResetWithOptions(ResetOptions options) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
clusterResetWithOptions(options, handler);
});
}
/**
* Forces the node to save cluster state on disk.
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction clusterSaveconfig(Handler> handler) {
delegate.clusterSaveconfig(handler);
return this;
}
/**
* Forces the node to save cluster state on disk.
* @return
*/
public Single rxClusterSaveconfig() {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
clusterSaveconfig(handler);
});
}
/**
* Set the configuration epoch in a new node.
* @param epoch
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction clusterSetConfigEpoch(long epoch, Handler> handler) {
delegate.clusterSetConfigEpoch(epoch, handler);
return this;
}
/**
* Set the configuration epoch in a new node.
* @param epoch
* @return
*/
public Single rxClusterSetConfigEpoch(long epoch) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
clusterSetConfigEpoch(epoch, handler);
});
}
/**
* Bind an hash slot to a specific node.
* @param slot
* @param subcommand
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction clusterSetslot(long slot, SlotCmd subcommand, Handler> handler) {
delegate.clusterSetslot(slot, subcommand, handler);
return this;
}
/**
* Bind an hash slot to a specific node.
* @param slot
* @param subcommand
* @return
*/
public Single rxClusterSetslot(long slot, SlotCmd subcommand) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
clusterSetslot(slot, subcommand, handler);
});
}
/**
* Bind an hash slot to a specific node.
* @param slot
* @param subcommand
* @param nodeId
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction clusterSetslotWithNode(long slot, SlotCmd subcommand, String nodeId, Handler> handler) {
delegate.clusterSetslotWithNode(slot, subcommand, nodeId, handler);
return this;
}
/**
* Bind an hash slot to a specific node.
* @param slot
* @param subcommand
* @param nodeId
* @return
*/
public Single rxClusterSetslotWithNode(long slot, SlotCmd subcommand, String nodeId) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
clusterSetslotWithNode(slot, subcommand, nodeId, handler);
});
}
/**
* List slave nodes of the specified master node.
* @param nodeId
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction clusterSlaves(String nodeId, Handler> handler) {
delegate.clusterSlaves(nodeId, handler);
return this;
}
/**
* List slave nodes of the specified master node.
* @param nodeId
* @return
*/
public Single rxClusterSlaves(String nodeId) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
clusterSlaves(nodeId, handler);
});
}
/**
* Get array of Cluster slot to node mappings
* @param handler
* @return
*/
public RedisTransaction clusterSlots(Handler> handler) {
delegate.clusterSlots(handler);
return this;
}
/**
* Get array of Cluster slot to node mappings
* @return
*/
public Single rxClusterSlots() {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
clusterSlots(handler);
});
}
/**
* Get array of Redis command details
* @param handler
* @return
*/
public RedisTransaction command(Handler> handler) {
delegate.command(handler);
return this;
}
/**
* Get array of Redis command details
* @return
*/
public Single rxCommand() {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
command(handler);
});
}
/**
* Get total number of Redis commands
* @param handler
* @return
*/
public RedisTransaction commandCount(Handler> handler) {
delegate.commandCount(handler);
return this;
}
/**
* Get total number of Redis commands
* @return
*/
public Single rxCommandCount() {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
commandCount(handler);
});
}
/**
* Extract keys given a full Redis command
* @param handler
* @return
*/
public RedisTransaction commandGetkeys(Handler> handler) {
delegate.commandGetkeys(handler);
return this;
}
/**
* Extract keys given a full Redis command
* @return
*/
public Single rxCommandGetkeys() {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
commandGetkeys(handler);
});
}
/**
* Get array of specific Redis command details
* @param commands List of commands to get info for
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction commandInfo(List commands, Handler> handler) {
delegate.commandInfo(commands, handler);
return this;
}
/**
* Get array of specific Redis command details
* @param commands List of commands to get info for
* @return
*/
public Single rxCommandInfo(List commands) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
commandInfo(commands, handler);
});
}
/**
* Get the value of a configuration parameter
* @param parameter Configuration parameter
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction configGet(String parameter, Handler> handler) {
delegate.configGet(parameter, handler);
return this;
}
/**
* Get the value of a configuration parameter
* @param parameter Configuration parameter
* @return
*/
public Single rxConfigGet(String parameter) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
configGet(parameter, handler);
});
}
/**
* Rewrite the configuration file with the in memory configuration
* @param handler
* @return
*/
public RedisTransaction configRewrite(Handler> handler) {
delegate.configRewrite(handler);
return this;
}
/**
* Rewrite the configuration file with the in memory configuration
* @return
*/
public Single rxConfigRewrite() {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
configRewrite(handler);
});
}
/**
* Set a configuration parameter to the given value
* @param parameter Configuration parameter
* @param value New value
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction configSet(String parameter, String value, Handler> handler) {
delegate.configSet(parameter, value, handler);
return this;
}
/**
* Set a configuration parameter to the given value
* @param parameter Configuration parameter
* @param value New value
* @return
*/
public Single rxConfigSet(String parameter, String value) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
configSet(parameter, value, handler);
});
}
/**
* Reset the stats returned by INFO
* @param handler
* @return
*/
public RedisTransaction configResetstat(Handler> handler) {
delegate.configResetstat(handler);
return this;
}
/**
* Reset the stats returned by INFO
* @return
*/
public Single rxConfigResetstat() {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
configResetstat(handler);
});
}
/**
* Return the number of keys in the selected database
* @param handler
* @return
*/
public RedisTransaction dbsize(Handler> handler) {
delegate.dbsize(handler);
return this;
}
/**
* Return the number of keys in the selected database
* @return
*/
public Single rxDbsize() {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
dbsize(handler);
});
}
/**
* Get debugging information about a key
* @param key Key string
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction debugObject(String key, Handler> handler) {
delegate.debugObject(key, handler);
return this;
}
/**
* Get debugging information about a key
* @param key Key string
* @return
*/
public Single rxDebugObject(String key) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
debugObject(key, handler);
});
}
/**
* Make the server crash
* @param handler
* @return
*/
public RedisTransaction debugSegfault(Handler> handler) {
delegate.debugSegfault(handler);
return this;
}
/**
* Make the server crash
* @return
*/
public Single rxDebugSegfault() {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
debugSegfault(handler);
});
}
/**
* Decrement the integer value of a key by one
* @param key Key string
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction decr(String key, Handler> handler) {
delegate.decr(key, handler);
return this;
}
/**
* Decrement the integer value of a key by one
* @param key Key string
* @return
*/
public Single rxDecr(String key) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
decr(key, handler);
});
}
/**
* Decrement the integer value of a key by the given number
* @param key Key string
* @param decrement Value by which to decrement
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction decrby(String key, long decrement, Handler> handler) {
delegate.decrby(key, decrement, handler);
return this;
}
/**
* Decrement the integer value of a key by the given number
* @param key Key string
* @param decrement Value by which to decrement
* @return
*/
public Single rxDecrby(String key, long decrement) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
decrby(key, decrement, handler);
});
}
/**
* Delete a key
* @param key Keys to delete
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction del(String key, Handler> handler) {
delegate.del(key, handler);
return this;
}
/**
* Delete a key
* @param key Keys to delete
* @return
*/
public Single rxDel(String key) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
del(key, handler);
});
}
/**
* Delete many keys
* @param keys List of keys to delete
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction delMany(List keys, Handler> handler) {
delegate.delMany(keys, handler);
return this;
}
/**
* Delete many keys
* @param keys List of keys to delete
* @return
*/
public Single rxDelMany(List keys) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
delMany(keys, handler);
});
}
/**
* Discard all commands issued after MULTI
* @param handler
* @return
*/
public RedisTransaction discard(Handler> handler) {
delegate.discard(handler);
return this;
}
/**
* Discard all commands issued after MULTI
* @return
*/
public Single rxDiscard() {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
discard(handler);
});
}
/**
* Return a serialized version of the value stored at the specified key.
* @param key Key string
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction dump(String key, Handler> handler) {
delegate.dump(key, handler);
return this;
}
/**
* Return a serialized version of the value stored at the specified key.
* @param key Key string
* @return
*/
public Single rxDump(String key) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
dump(key, handler);
});
}
/**
* Echo the given string
* @param message String to echo
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction echo(String message, Handler> handler) {
delegate.echo(message, handler);
return this;
}
/**
* Echo the given string
* @param message String to echo
* @return
*/
public Single rxEcho(String message) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
echo(message, handler);
});
}
/**
* Execute a Lua script server side. Due to the dynamic nature of this command any response type could be returned
* for This reason and to ensure type safety the reply is always guaranteed to be a JsonArray.
*
* When a reply if for example a String the handler will be called with a JsonArray with a single element containing
* the String.
* @param script Lua script to evaluate
* @param keys List of keys
* @param args List of argument values
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction eval(String script, List keys, List args, Handler> handler) {
delegate.eval(script, keys, args, handler);
return this;
}
/**
* Execute a Lua script server side. Due to the dynamic nature of this command any response type could be returned
* for This reason and to ensure type safety the reply is always guaranteed to be a JsonArray.
*
* When a reply if for example a String the handler will be called with a JsonArray with a single element containing
* the String.
* @param script Lua script to evaluate
* @param keys List of keys
* @param args List of argument values
* @return
*/
public Single rxEval(String script, List keys, List args) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
eval(script, keys, args, handler);
});
}
/**
* Execute a Lua script server side. Due to the dynamic nature of this command any response type could be returned
* for This reason and to ensure type safety the reply is always guaranteed to be a JsonArray.
*
* When a reply if for example a String the handler will be called with a JsonArray with a single element containing
* the String.
* @param sha1 SHA1 digest of the script cached on the server
* @param keys List of keys
* @param values List of values
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction evalsha(String sha1, List keys, List values, Handler> handler) {
delegate.evalsha(sha1, keys, values, handler);
return this;
}
/**
* Execute a Lua script server side. Due to the dynamic nature of this command any response type could be returned
* for This reason and to ensure type safety the reply is always guaranteed to be a JsonArray.
*
* When a reply if for example a String the handler will be called with a JsonArray with a single element containing
* the String.
* @param sha1 SHA1 digest of the script cached on the server
* @param keys List of keys
* @param values List of values
* @return
*/
public Single rxEvalsha(String sha1, List keys, List values) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
evalsha(sha1, keys, values, handler);
});
}
/**
* Execute all commands issued after MULTI
* @param handler
* @return
*/
public RedisTransaction exec(Handler> handler) {
delegate.exec(handler);
return this;
}
/**
* Execute all commands issued after MULTI
* @return
*/
public Single rxExec() {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
exec(handler);
});
}
/**
* Determine if a key exists
* @param key Key string
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction exists(String key, Handler> handler) {
delegate.exists(key, handler);
return this;
}
/**
* Determine if a key exists
* @param key Key string
* @return
*/
public Single rxExists(String key) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
exists(key, handler);
});
}
/**
* Determine if one or many keys exist
* @param keys List of key strings
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction existsMany(List keys, Handler> handler) {
delegate.existsMany(keys, handler);
return this;
}
/**
* Determine if one or many keys exist
* @param keys List of key strings
* @return
*/
public Single rxExistsMany(List keys) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
existsMany(keys, handler);
});
}
/**
* Set a key's time to live in seconds
* @param key Key string
* @param seconds Time to live in seconds
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction expire(String key, int seconds, Handler> handler) {
delegate.expire(key, seconds, handler);
return this;
}
/**
* Set a key's time to live in seconds
* @param key Key string
* @param seconds Time to live in seconds
* @return
*/
public Single rxExpire(String key, int seconds) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
expire(key, seconds, handler);
});
}
/**
* Set the expiration for a key as a UNIX timestamp
* @param key Key string
* @param seconds Expiry time as Unix timestamp in seconds
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction expireat(String key, long seconds, Handler> handler) {
delegate.expireat(key, seconds, handler);
return this;
}
/**
* Set the expiration for a key as a UNIX timestamp
* @param key Key string
* @param seconds Expiry time as Unix timestamp in seconds
* @return
*/
public Single rxExpireat(String key, long seconds) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
expireat(key, seconds, handler);
});
}
/**
* Remove all keys from all databases
* @param handler
* @return
*/
public RedisTransaction flushall(Handler> handler) {
delegate.flushall(handler);
return this;
}
/**
* Remove all keys from all databases
* @return
*/
public Single rxFlushall() {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
flushall(handler);
});
}
/**
* Remove all keys from the current database
* @param handler
* @return
*/
public RedisTransaction flushdb(Handler> handler) {
delegate.flushdb(handler);
return this;
}
/**
* Remove all keys from the current database
* @return
*/
public Single rxFlushdb() {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
flushdb(handler);
});
}
/**
* Get the value of a key
* @param key Key string
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction get(String key, Handler> handler) {
delegate.get(key, handler);
return this;
}
/**
* Get the value of a key
* @param key Key string
* @return
*/
public Single rxGet(String key) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
get(key, handler);
});
}
/**
* Get the value of a key - without decoding as utf-8
* @param key Key string
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction getBinary(String key, Handler> handler) {
delegate.getBinary(key, new Handler>() {
public void handle(AsyncResult ar) {
if (ar.succeeded()) {
handler.handle(io.vertx.core.Future.succeededFuture(Buffer.newInstance(ar.result())));
} else {
handler.handle(io.vertx.core.Future.failedFuture(ar.cause()));
}
}
});
return this;
}
/**
* Get the value of a key - without decoding as utf-8
* @param key Key string
* @return
*/
public Single rxGetBinary(String key) {
return new io.vertx.reactivex.core.impl.AsyncResultSingle(handler -> {
getBinary(key, handler);
});
}
/**
* Returns the bit value at offset in the string value stored at key
* @param key Key string
* @param offset Offset in bits
* @param handler Handler for the result of this call.
* @return
*/
public RedisTransaction getbit(String key, long offset, Handler> handler) {
delegate.getbit(key, offset, handler);
return this;
}
/**
* Returns the bit value at offset in the string value stored at key
* @param key Key string
* @param offset Offset in bits
* @return
*/
public Single